Overcoming Common Pitfalls in Exploratory Programming
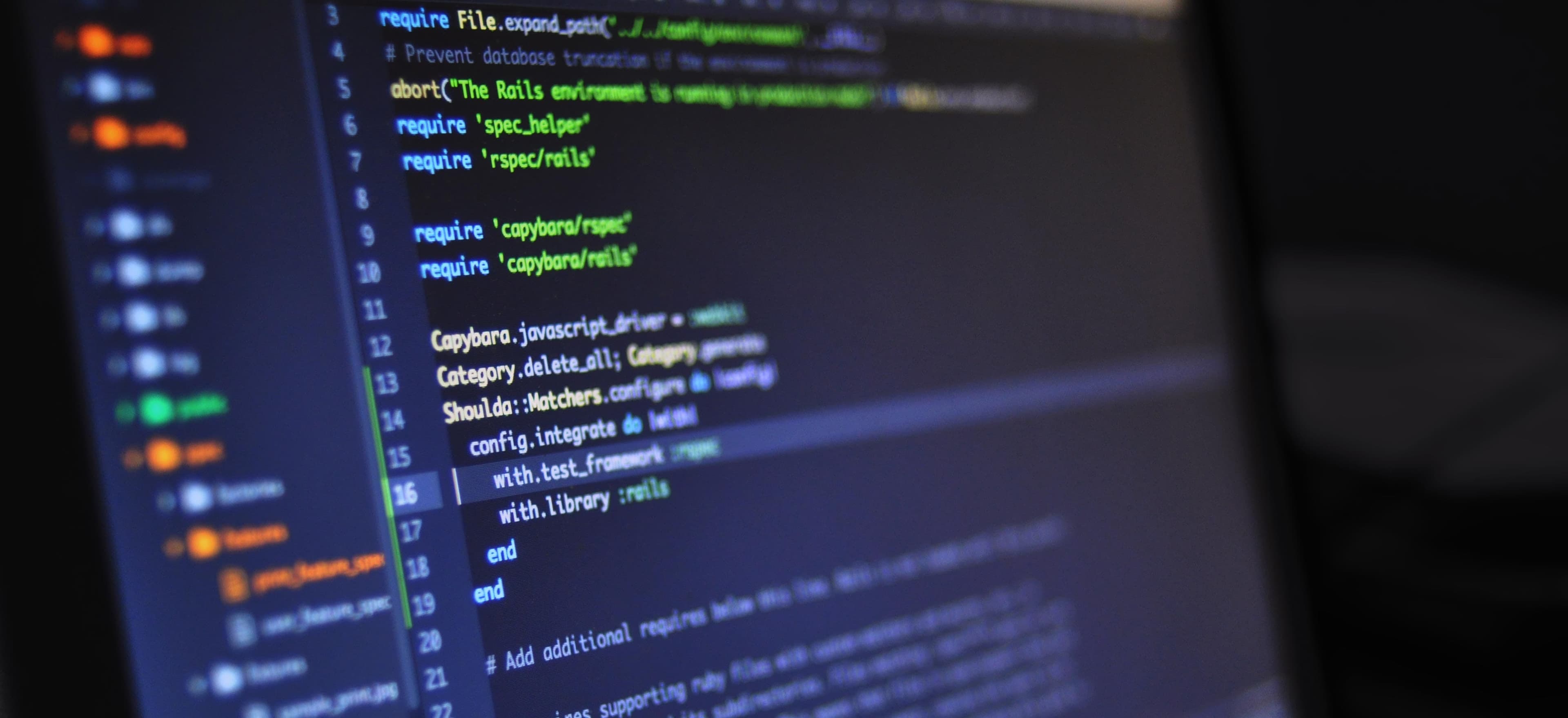
- Published on
Overcoming Common Pitfalls in Exploratory Programming
Exploratory programming can be a liberating and enjoyable experience. Unlike traditional programming, where the objectives are often rigidly defined, exploratory programming allows developers to experiment, learn, and innovate. However, with this freedom comes challenges that can lead to common pitfalls. In this post, we will discuss these pitfalls and provide strategies for overcoming them. The goal is to enhance your exploratory programming endeavors while maintaining a productive workflow.
Understanding Exploratory Programming
Before diving into the pitfalls, let’s clarify what exploratory programming entails. At its core, exploratory programming is about experimentation. Developers might work on unfamiliar problems, languages, or technologies. It's a way to understand new domains, concepts, or frameworks better.
This method can lead to serendipitous discoveries but can also cause you to stray off course if not managed properly. With this in mind, we must acknowledge the potential pitfalls you may encounter.
Common Pitfalls in Exploratory Programming
-
Lack of Clear Objectives
One of the biggest mistakes in exploratory programming is diving in without a clear goal. While exploration fosters creativity, aimlessness can lead to wasted time and effort.
Solution: Set specific objectives. For instance, instead of saying, "I want to learn Java," refine it to, "I want to learn Java’s exception handling." This clarity will guide your exploration and keep you focused.
-
Inadequate Documentation
It’s easy to forget what you’ve done when you’re experimenting. Over time, a lack of documentation can make it difficult to retrace your steps or replicate your findings.
Solution: Document as you go. Use tools like Markdown for easy formatting or platforms like GitHub to maintain a record. Here’s a simple example of how you might document a Java function:
/** * This method calculates the factorial of a number. * @param number The number to calculate factorial for. * @return The factorial of the given number. */ public static long factorial(int number) { if (number == 0) { return 1; } return number * factorial(number - 1); }
This documentation not only helps clarify the method's functionality but also provides context for any future analysis or alterations.
-
Over-Complexity
Exploratory programming can sometimes lead to overly complex solutions. As you experiment, it's easy to add layers of complexity that may not be necessary.
Solution: Keep your code simple and readable. Implement the KISS principle (Keep It Simple, Stupid). For example, if you're exploring a data structure, begin with the simplest implementation before gradually introducing complexity.
Instead of complex nested loops, consider using a Java Stream API for simplicity:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5); int sum = numbers.stream().mapToInt(Integer::intValue).sum(); System.out.println("Sum: " + sum);
This approach simplifies summing integers and makes the code more understandable.
-
Neglecting Best Practices
In the spirit of experimentation, many programmers tend to skip best practices such as code reviews and tests. While exploratory programming is inherently informal, neglecting these practices can lead to poorly written code.
Solution: Integrate best practices into your exploration process. Write unit tests for your experiments. For instance, if you develop a new functionality, ensure that you create corresponding JUnit tests:
import static org.junit.jupiter.api.Assertions.assertEquals; import org.junit.jupiter.api.Test; public class FactorialTest { @Test public void testFactorial() { assertEquals(120, factorial(5)); assertEquals(1, factorial(0)); } }
Running tests ensures that your exploratory code is robust and reliable, even if it deviates from conventional structures.
-
Failure to Iterate
After building something, many developers stop exploring further. The initial excitement can blind developers to the necessity of iteration and enhancement.
Solution: Adopt an iterative mindset. After creating an initial solution, assess its strengths and weaknesses. Seek feedback and continuously improve it. Use version control systems, such as Git, to experiment within branches without affecting the main codebase.
-
Ignoring Community Resources
Many programmers overlook existing community resources that can enhance their work. The programming community is rich with documentation, forums, and shared projects.
Solution: Engage with your community. Use platforms like Stack Overflow for questions, or explore GitHub for source code and inspiration. Leveraging these resources can spur new ideas and prevent you from reinventing the wheel.
Bringing It All Together
Exploratory programming is a valuable way to gain experience, learn about new technologies, and uncover novel solutions. However, it is crucial to acknowledge and navigate the common pitfalls that can arise during this process. By setting clear objectives, documenting your work, adhering to simplicity and best practices, iterating on solutions, and utilizing community resources, you will maximize your success in exploratory programming.
Remember, the goal is not just to write code but to create meaningful, maintainable, and innovative solutions. Happy coding!
Checkout our other articles