Common Issues When Integrating Twitter API in Java Apps
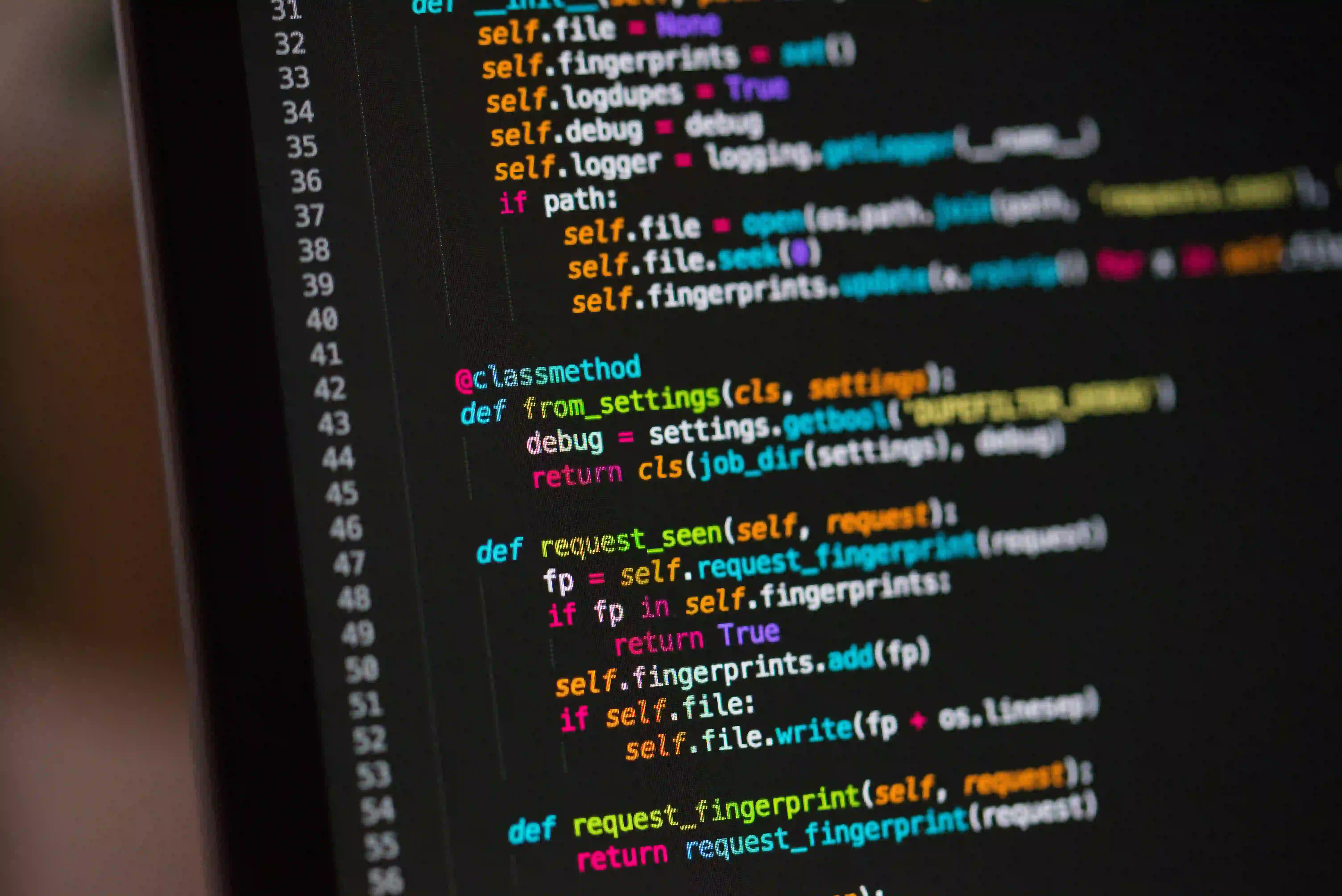
Common Issues When Integrating Twitter API in Java Apps
Integrating the Twitter API into Java applications can open a world of opportunities for real-time data access and social media engagement. However, this integration is not without its challenges. In this blog post, we will explore some common issues developers face while integrating the Twitter API in Java applications. We’ll provide you with practical solutions, tips, and code snippets to navigate these complexities effectively.
Table of Contents
- Understanding Twitter API Basics
- Common Issues in Twitter API Integration
- Authentication Problems
- Rate Limiting
- Handling JSON Responses
- Managing API Endpoints
- Best Practices for Integration
- Conclusion
Understanding Twitter API Basics
Before diving into potential issues, it's essential to understand the basics of the Twitter API. The Twitter API allows your application to interact with Twitter's platform, enabling functionalities such as posting tweets, retrieving user data, and more. By utilizing various endpoints, developers can access a wealth of information.
To start programming with the Twitter API in Java, you must obtain API keys and tokens. This includes:
- API Key
- API Secret Key
- Access Token
- Access Token Secret
These credentials authenticate your Java application with Twitter’s services.
Common Issues in Twitter API Integration
1. Authentication Problems
Authentication is perhaps the most common hurdle developers encounter when integrating the Twitter API. Ensuring that your credentials are configured correctly is vital.
Solution
Use libraries such as Twitter4J, which simplifies the authentication process. Below is a sample code snippet demonstrating how to set up authentication:
import twitter4j.conf.ConfigurationBuilder;
import twitter4j.Twitter;
import twitter4j.TwitterFactory;
public class TwitterAuth {
public static Twitter getTwitterInstance() {
ConfigurationBuilder cb = new ConfigurationBuilder();
cb.setDebugEnabled(true)
.setOAuthConsumerKey("YOUR_API_KEY")
.setOAuthConsumerSecret("YOUR_API_SECRET")
.setOAuthAccessToken("YOUR_ACCESS_TOKEN")
.setOAuthAccessTokenSecret("YOUR_ACCESS_TOKEN_SECRET");
TwitterFactory tf = new TwitterFactory(cb.build());
return tf.getInstance();
}
}
Why? This method streamlines the authentication process and reduces the likelihood of errors by using a dedicated library that handles most intricacies behind the scenes.
2. Rate Limiting
Twitter imposes rate limits on API calls to ensure fair usage. Hitting these limits can disrupt your application's functionality.
Solution
Be proactive in managing your API calls. Implement a strategy to check the rate limit status before making requests:
import twitter4j.TwitterException;
import twitter4j.Twitter;
public void checkRateLimit(Twitter twitter) throws TwitterException {
RateLimitStatus rateLimitStatus = twitter.getRateLimitStatus("application").get("/statuses/home_timeline");
System.out.println("Remaining Requests: " + rateLimitStatus.getRemaining());
}
Why? Understanding your rate limits allows you to adapt your application's request flow and avoid unexpected interruptions. For more information about Twitter's rate limits, check the Twitter Developer Documentation.
3. Handling JSON Responses
Another common issue developers face is parsing JSON responses from Twitter’s API. Often, the responses can be complex and nested, making data extraction cumbersome.
Solution
Using libraries like Jackson or Gson can simplify the JSON parsing process. Here's an example using Jackson:
import com.fasterxml.jackson.databind.ObjectMapper;
public class User {
public String name;
public String screen_name;
// Add other fields as needed
}
public User parseUserFromJson(String jsonResponse) throws IOException {
ObjectMapper objectMapper = new ObjectMapper();
return objectMapper.readValue(jsonResponse, User.class);
}
Why? Leveraging libraries for JSON parsing not only saves time but also significantly reduces the chances of manual errors while extracting data.
4. Managing API Endpoints
As Twitter updates its API and introduces new features, managing the changes in API endpoints can be a significant challenge.
Solution
Stay updated with the Twitter API changelog and consider creating a dedicated service to handle API calls. This approach makes it easy to manage endpoint changes without altering your core application logic.
import twitter4j.Status;
import twitter4j.Twitter;
import twitter4j.TwitterException;
public class TwitterService {
private Twitter twitter;
public TwitterService(Twitter twitter) {
this.twitter = twitter;
}
public List<Status> getUserTimeline(String username) throws TwitterException {
return twitter.getUserTimeline(username);
}
}
Why? By encapsulating API calls, you gain better control and adaptability, easing the transition whenever Twitter updates its API endpoints.
Best Practices for Integration
-
Error Handling: Always implement robust error handling. The Twitter API can be unpredictable; managing exceptions effectively will help maintain application stability.
-
Environment Variables: Store your API keys and tokens in environment variables instead of hardcoding them into your application. This adds an extra layer of security.
-
Testing: Use unit tests to verify API interactions. This ensures your application remains functional as you develop new features or make changes.
-
Content Guidelines: Familiarize yourself with Twitter's Content Guidelines to avoid any potential violations leading to API usage restrictions.
-
Documentation: Always refer to the Twitter API Documentation for the most up-to-date information regarding endpoints, parameters, and best practices.
Key Takeaways
Integrating the Twitter API in Java applications is undoubtedly rewarding, offering an enriched user experience through social media interactions. However, being aware of common issues—such as authentication problems, rate limiting, JSON handling, and managing API endpoints—can significantly ease your development process.
By adopting best practices and leveraging powerful libraries, you can construct seamless integrations that are as powerful as they are user-friendly. Remember, the world of social media is dynamic, and staying informed and agile is key to success.
Whether you are building a social media dashboard, a bot, or any other engaging application, embracing these challenges head-on will leave a lasting impact on your development journey. Happy coding!