Troubleshooting Common Issues in Spring AI with MongoDB
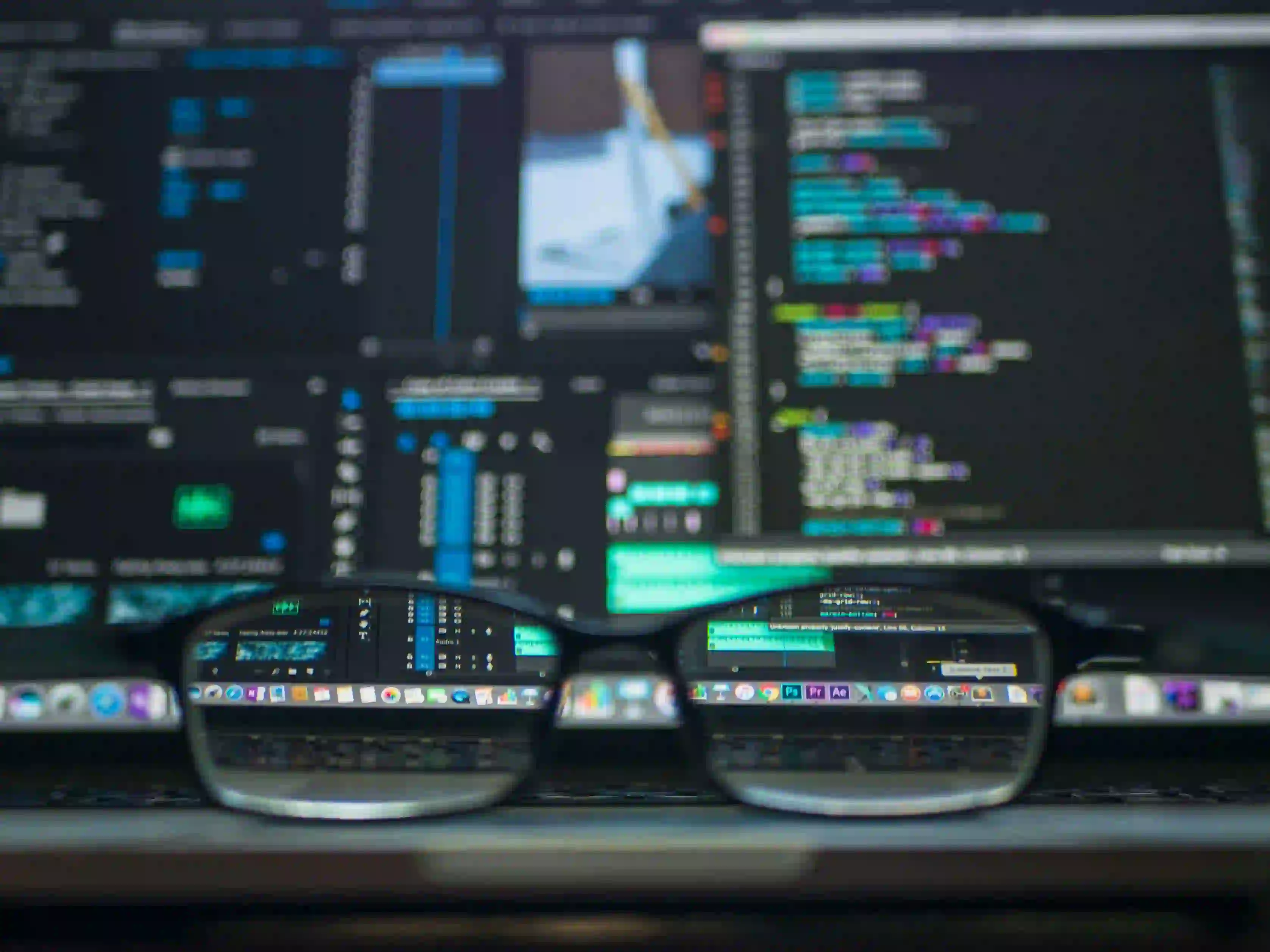
Troubleshooting Common Issues in Spring AI with MongoDB
In today's fast-paced digital world, incorporating artificial intelligence (AI) capabilities into your applications has become essential. Spring Framework, with its powerful integration of various technologies, offers a robust platform for building AI applications. MongoDB, a NoSQL database, serves as a great data store for AI projects due to its flexible schema and scalability.
However, developers may run into a variety of challenges while integrating Spring with MongoDB, especially when trying to leverage AI functionalities. In this blog post, we will explore some of the most common issues you might face using Spring AI with MongoDB, how to troubleshoot them, and best practices to keep in mind.
Table of Contents
- Understanding Spring AI and MongoDB
- Common Issues and Troubleshooting Tips
- Best Practices
- Conclusion
Understanding Spring AI and MongoDB
Before diving into troubleshooting, it’s important to understand the technologies involved.
Spring AI
Spring AI is an extension of the Spring ecosystem designed to facilitate the implementation of AI applications. It supports various machine learning frameworks and simplifies the integration and deployment of AI models in Spring applications.
MongoDB
MongoDB is a widely used NoSQL database that employs a document-oriented data model. It stores data in BSON (Binary JSON) format, allowing a flexible schema. This flexibility is particularly advantageous for AI applications that may require continuous adjustments to data structures based on the evolving nature of machine learning algorithms.
Common Issues and Troubleshooting Tips
Even with the seamless integration capabilities of Spring and MongoDB, developers may encounter issues. Here, we identify some common problems and their troubleshooting tips.
Connection Errors
Problem
A frequent issue developers encounter is the inability to connect to the MongoDB instance. This could stem from an incorrect URI, firewall settings, or incorrect configuration.
Troubleshooting Steps
-
Check Connection URI: Ensure that your MongoDB URI is correct. Here’s a common format:
☕snippet.javaspring.data.mongodb.uri=mongodb://<username>:<password>@localhost:27017/<database>
Make sure to replace
<username>
,<password>
, and<database>
with your actual data. -
Firewall Settings: Check for any firewall restrictions that might prevent access to your MongoDB instance. Ensure the default MongoDB port (27017) is open.
-
Using Docker: If you're running MongoDB in a Docker container, verify that you are using the correct IP address of the container and that networking is correctly set up.
Note: Using environment variables for sensitive data (username and password) is a good practice.
Data Consistency Issues
Problem
Data inconsistency issues may arise when multiple applications or instances of your application attempt to read and write to the database simultaneously.
Troubleshooting Steps
-
ACID Transactions: Use MongoDB's support for multi-document ACID transactions. Here is a brief snippet:
☕snippet.java@Transactional public void updateDocument(String id, String newValue) { Query query = new Query(Criteria.where("_id").is(id)); Update update = new Update().set("value", newValue); mongoTemplate.updateFirst(query, update, Document.class); }
The
@Transactional
annotation ensures all operations in the method are completed successfully or none at all. -
Optimistic Locking: Implement optimistic locking by using versioning with your entity. Here’s how you might define a document:
☕snippet.java@Document public class MyData { @Id private String id; private String value; @Version private Long version; // For optimistic locking }
Performance Bottlenecks
Problem
As your application scales, you may notice performance bottlenecks due to inefficient queries or indexing.
Troubleshooting Steps
-
Indexes: Ensure you are using indexes appropriately. Without indexing, MongoDB may perform full table scans, degrading performance.
☕snippet.java@CompoundIndex(def = "{'field1': 1, 'field2': -1}") // Example of a compound index public class MyData { ... }
-
Profiling Queries: Use MongoDB's built-in tools to profile your queries. This will help you gain insights on which queries are taking longer than expected.
Query Optimization
Problem
Inefficient queries can slow down your application, especially when dealing with large data volumes common in AI applications.
Troubleshooting Steps
-
Use Projections: Only retrieve necessary fields instead of fetching entire documents. For instance:
☕snippet.javaQuery query = new Query(); query.fields().include("field1").include("field2"); // Fetch only specific fields List<MyData> results = mongoTemplate.find(query, MyData.class);
-
Aggregation Framework: Utilize MongoDB’s aggregation capabilities to perform complex data transformations.
Handling Data Serialization
Problem
AI models often require specific data formats. Failing to correctly serialize data can lead to problems when training or making predictions.
Troubleshooting Steps
-
Custom Serializers: Implement custom serializers for your data models to ensure they match the expected format. For example:
☕snippet.java@Document public class MyModel { @Id private String id; private List<Double> features; // Example feature list for AI model ... }
-
Using BSON: Utilize BSON if needed for binary data formats, especially for large datasets like images or videos.
Best Practices
To mitigate issues in the future, consider the following best practices:
- Version Control: Use version control for your database schema and configurations.
- Regular Backups: Regularly back up your data. MongoDB Atlas provides automated backup options that are essential for disaster recovery.
- Monitoring Tools: Use monitoring tools like MongoDB Cloud Manager or third-party performance monitoring solutions to keep an eye on your application's performance.
- Test Extensively: Perform thorough testing, particularly in scenarios involving concurrent data access or complex AI processes.
To Wrap Things Up
Integrating Spring AI with MongoDB can elevate your application's capabilities, but like any development endeavor, it can come with its challenges. By following the troubleshooting tips and best practices highlighted in this post, you can effectively navigate common issues and harness the powerful combination of Spring and MongoDB.
For further resources, consider looking into the official Spring AI documentation and MongoDB documentation. Happy coding!
This blog post serves as a guide, but real-world applications will often present unique challenges. Share your experiences in the comments below, and let’s improve our Spring AI implementations together!