Mastering Runtime Logging: Change Levels On-the-Fly!
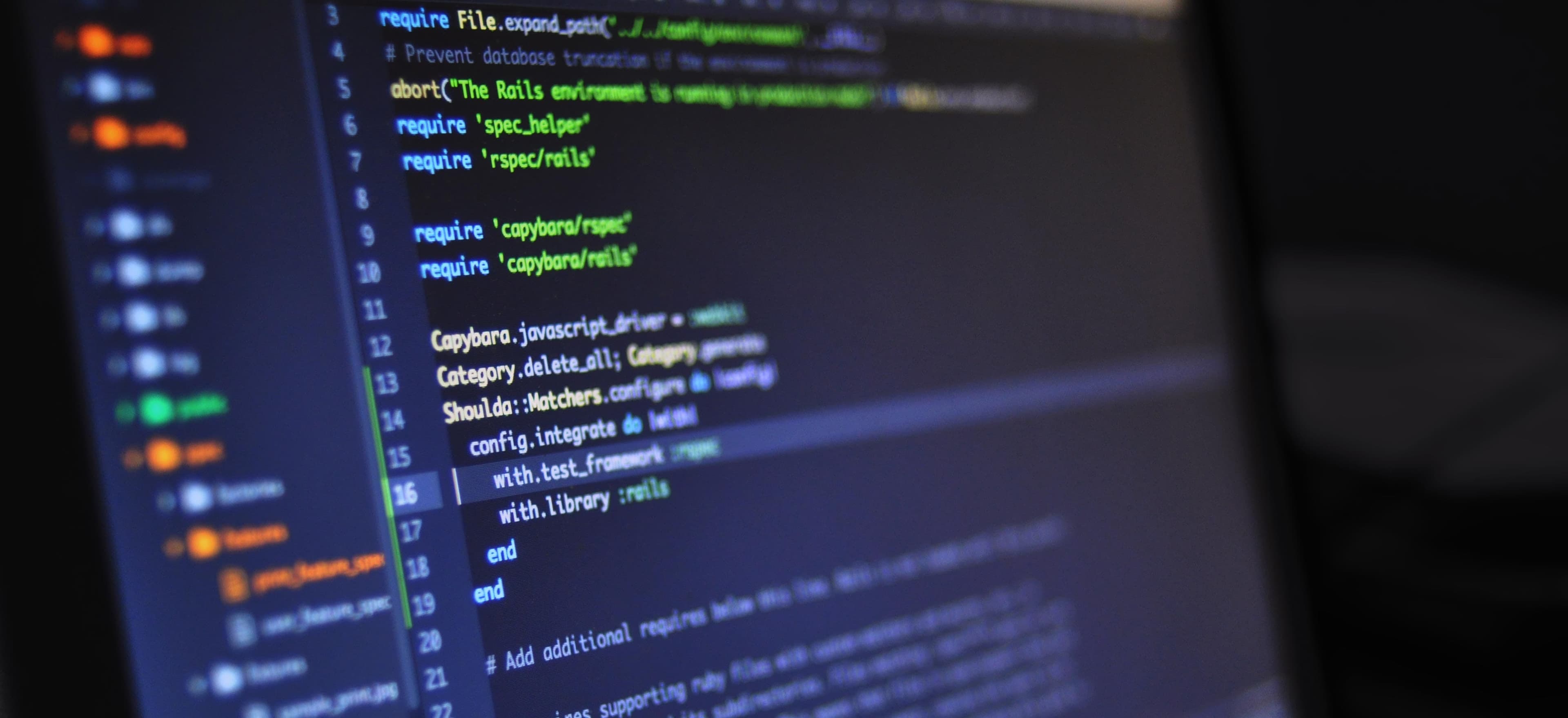
- Published on
Mastering Runtime Logging: Change Levels On-the-Fly!
When developing a robust Java application, logging is an essential aspect that often gets overlooked. Effective logging can provide insight into the runtime behavior of your application, simplify debugging, and aid in monitoring application health in production. In modern applications, runtime logging often comes with the ability to change logging levels dynamically without restarting the application. This capability is crucial for adapting the level of detail captured in logs while the application is running.
In this blog post, we will delve into the intricacies of logging in Java—specifically, how to implement runtime logging level adjustments with practical examples. By the end, you will understand why and how to utilize this feature, enhancing your application’s maintainability and debugging efficiency.
Importance of Logging Levels
Logging frameworks in Java, such as Log4j, SLF4J, and Java Util Logging, support various logging levels—INFO, DEBUG, WARN, ERROR, etc. Each level is designed for different purposes:
- DEBUG: Detailed information, typically of interest only when diagnosing problems.
- INFO: General system information and notifications.
- WARN: Indications of possible issues that are not errors yet.
- ERROR: Error events that might still allow the application to continue running.
Changing these levels on-the-fly allows developers to capture richer information during specific troubleshooting sessions without overwhelming them with data in normal operations.
Setting Up Your Logging Framework
For this tutorial, we will use Log4j 2, a widely-used logging framework that provides advanced features and fine control over logging. Ensure you include the necessary dependencies in your pom.xml
if you are using Maven:
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-api</artifactId>
<version>2.17.0</version>
</dependency>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>2.17.0</version>
</dependency>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-slf4j-impl</artifactId>
<version>2.17.0</version>
</dependency>
Configuration of Log4j 2
To initialize Log4j, create a configuration file named log4j2.xml
in the src/main/resources
directory. Here is a basic setup to get you started:
<?xml version="1.0" encoding="UTF-8"?>
<Configuration status="WARN">
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{yyyy-MM-dd HH:mm:ss} %p %c{1} - %m%n"/>
</Console>
</Appenders>
<Loggers>
<Root level="INFO">
<AppenderRef ref="Console"/>
</Root>
</Loggers>
</Configuration>
Log4j 2 API Usage
With the logging framework set up, now it's time to implement logging in your Java application. Here’s a brief yet illustrative example:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class LoggingExample {
private static final Logger logger = LogManager.getLogger(LoggingExample.class);
public void execute() {
logger.debug("This is a debug message");
logger.info("Application is starting...");
logger.warn("Warning: Something unexpected happened!");
logger.error("Error occurred!", new RuntimeException("Sample Exception"));
}
public static void main(String[] args) {
new LoggingExample().execute();
}
}
The Significance of Logging Example
In this simple class, we create a logger instance that captures various logging events. The purpose of these logs is clear—it enables us to track the state and behavior of the application. However, logging every event at the DEBUG level can clutter output and degrade performance. This is where dynamic log level changes come into play.
Changing Logging Levels at Runtime
Log4j 2 supports changing logging levels at runtime via its management API. Here’s how to leverage this functionality.
Step 1: Adding JMX Support
You can enable JMX (Java Management Extensions) in your Log4j configuration to manage logging levels dynamically. Modify log4j2.xml
as follows:
<Configuration status="WARN">
...
<Jmx enabled="true"/>
...
</Configuration>
Step 2: Sample JMX Client Code
You can access the JMX MBeans using standard JMX tooling (JConsole, VisualVM, etc.) or programmatically. Below is an example of how you might change the logging level within your application.
import javax.management.MBeanServer;
import javax.management.ObjectName;
import java.lang.management.ManagementFactory;
public class LogLevelChanger {
public static void changeLogLevel(String loggerName, String newLevel) {
try {
MBeanServer mBeanServer = ManagementFactory.getPlatformMBeanServer();
ObjectName objectName = new ObjectName("org.apache.logging.log4j2:type=Logger,name=" + loggerName);
mBeanServer.setAttribute(objectName, new javax.management.Attribute("level", newLevel));
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why Change Log Levels?
Here’s the why behind changing logging levels dynamically:
- Performance Optimization: Reduce the verbosity of logging during regular operation, switching to a more verbose level only during debugging sessions.
- Flexibility: Developers can toggle levels without needing to restart the application or redeploy code.
- Monitoring: In production environments, only capturing WARN and ERROR logs can prevent flooding the log files, but maintaining the ability to switch to DEBUG for specific modules can be invaluable.
Final Thoughts
Runtime logging and the ability to change levels dynamically are invaluable tools for application debugging and troubleshooting. They save you from restarting services for minor tweaks and can enhance the performance of your application by controlling log verbosity.
For more thorough readings on logging frameworks, refer to the official Log4j documentation and consider exploring Java Logging Basics to solidify your foundation.
By mastering runtime logging and adapting levels on-the-fly in your Java applications, you'll make significant strides in manageability and troubleshooting efficiencies. Happy logging!
Checkout our other articles