Combatting Latency Issues in Microservices Architecture
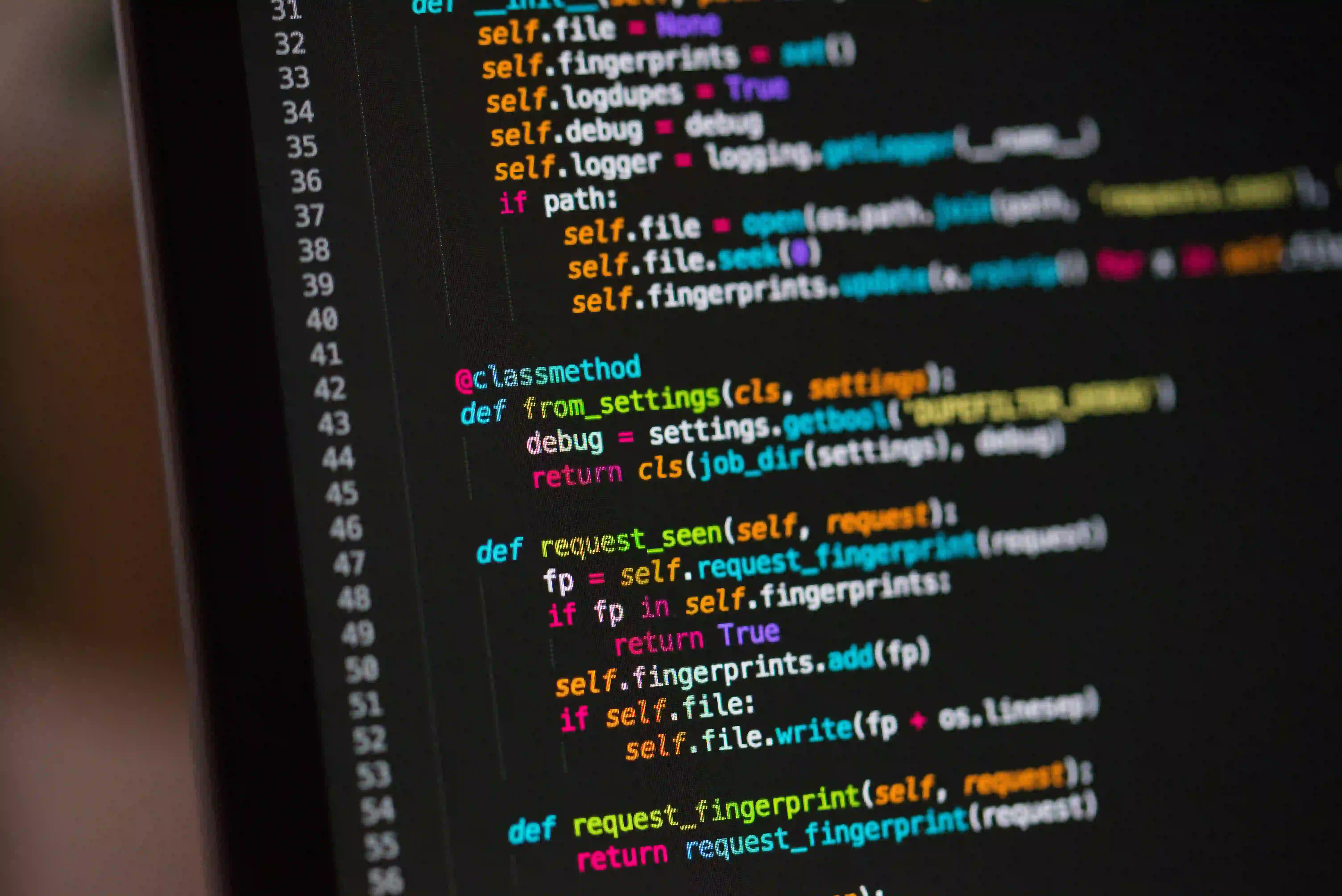
Combatting Latency Issues in Microservices Architecture
In today's digital-first world, microservices architecture has emerged as a powerful approach for software development. It allows teams to build and deploy applications as a suite of small, independent services. However, one significant challenge developers often face is latency. This blog delves into the intricacies of latency issues within microservices and discusses effective strategies for combatting them.
Understanding Latency in Microservices
Latency refers to the delay between the request for data and the delivery of that data. It's a key factor in the user experience, especially in distributed systems like microservices architectures. Given the interconnected nature of microservices, a single service's performance can significantly affect the overall latency of the application.
Common Causes of Latency
- Network Overhead: Microservices communicate over the network, and network latency is an unavoidable factor.
- Service Dependencies: A request often needs to traverse multiple services, which can increase the travel time.
- Serialization/Deserialization: Transforming data into a format suitable for transmission adds processing time.
- Database Calls: Many services depend on databases, and database latency can introduce delays.
- Heavy Payloads: Large amounts of data transfer can slow down communication.
- Cold Starts: When a service is deployed but not currently running, it can take time to start up (a common issue with serverless architectures).
Strategies to Combat Latency
Let’s explore some methodologies to reduce latency effectively while maximizing the efficiency of your microservices architecture.
1. Optimize Network Communication
Efficient network communication can significantly reduce latency.
Use Asynchronous Communication
Asynchronous communication allows the services to proceed without waiting for the responses of others. For example, using message queues like RabbitMQ or Kafka can decouple services, hence minimizing wait times.
import org.springframework.amqp.rabbit.core.RabbitTemplate;
// Beans Configuration
@Bean
public RabbitTemplate rabbitTemplate(ConnectionFactory connectionFactory) {
return new RabbitTemplate(connectionFactory);
}
// Sending Message
@Autowired
private RabbitTemplate rabbitTemplate;
public void sendMessage(String message) {
rabbitTemplate.convertAndSend("myQueue", message);
// Asynchronous as no direct wait for a response
}
Why? Asynchronous communication allows your application to remain responsive, as services can handle other requests while waiting for a response.
2. Caching Mechanisms
Implementing caching strategies helps to alleviate the burden on databases or external APIs, thereby reducing latency.
Utilize Distributed Caching
Tools like Redis or Memcached can store frequently accessed data, allowing services to retrieve it faster than querying a database.
import org.springframework.cache.annotation.Cacheable;
@Service
public class UserService {
@Cacheable("users")
public User getUserById(String userId) {
return userRepository.findById(userId).orElse(null);
}
}
Why? Caching eliminates repeated database access for commonly requested data, significantly speeding up response times.
3. Service Discovery and Load Balancing
Using service discovery tools can optimize how requests are routed to services, thereby improving response speeds.
Implement Load Balancers
Load balancers distribute incoming network traffic evenly across multiple instances of a service, preventing bottlenecks.
services:
my-service:
image: my-service-image
deploy:
replicas: 3
networks:
- my-network
networks:
my-network:
Why? Using a load balancer helps manage request loads more evenly, ensuring no single service becomes a bottleneck.
4. Microservices Design Best Practices
Designing microservices with latency in mind involves several essential practices:
Use Appropriate Database Strategies
- Read Replicas: Set up read replicas for your database to handle increased read requests separately.
- Database Sharding: Distribute data across multiple databases to spread the load.
Implement Rate Limiting
Avoid overwhelming services with too many requests, as this leads to higher latency. Tools like Sentinel or Histrix can be useful.
5. Monitor and Analyze Performance
Regularly monitoring your services can help identify latency issues effectively.
Distributed Tracing
Tools like Jaeger or Zipkin allow tracking requests as they traverse through various services, making it easier to pinpoint where latency arises.
Why? Tracking the flow helps teams immediately address problem areas, improving overall system performance.
The Closing Argument
In a microservices architecture, latency can be a persistent challenge. By understanding its causes, leveraging strategies such as efficient communication, caching, load balancing, and adopting best design practices, you can significantly mitigate latency issues.
Microservices offer an agile approach to software development, but without addressing latency, you risk degrading the user experience. As you optimize your architecture, remember that regular monitoring and performance analysis are key to maintaining responsiveness.
For further reading, you may find the following resources insightful:
- Microservices Performance Challenges
- Caching Strategies in Microservices
By adhering to these principles, you’ll ensure that latency won’t be an Achilles' heel for your microservices architecture. Happy coding!