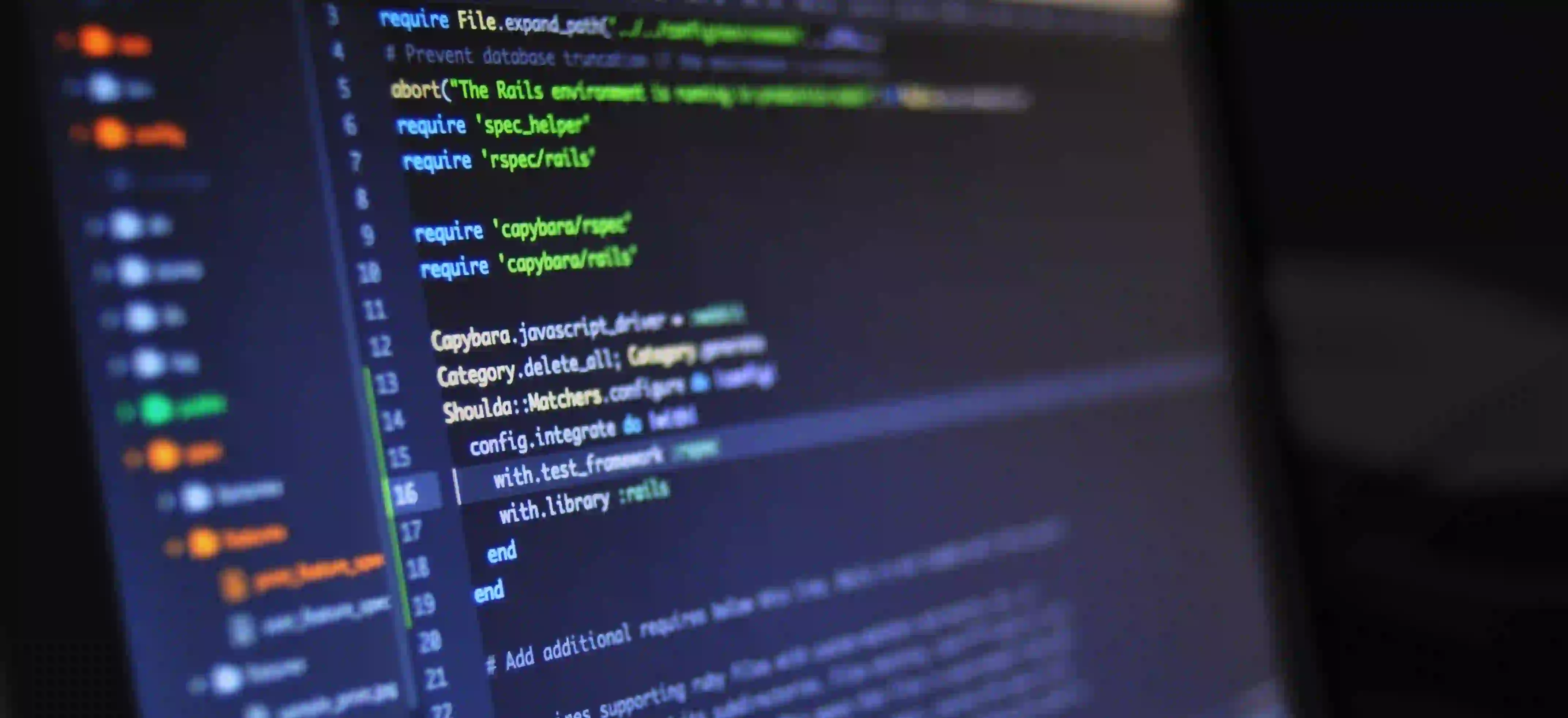
Common Pitfalls When Integrating MongoDB with Spring Boot
Spring Boot and MongoDB make for a formidable duo—bringing together ease of development and a powerful NoSQL database. However, navigating through this integration can sometimes be a rocky road. In this post, we'll explore common pitfalls developers often encounter while integrating MongoDB with Spring Boot and how to overcome these challenges.
Understanding Spring Boot and MongoDB
What is Spring Boot?
Spring Boot is an extension of the Spring framework that simplifies the process of building production-ready applications. With its various annotations and auto-configuration capabilities, it emphasizes convention over configuration, allowing developers to focus more on writing code rather than worrying about configuration details.
What is MongoDB?
MongoDB is a NoSQL database known for its high performance, scalability, and flexibility with data structures. It stores data in JSON-like documents, which makes it particularly adaptable for applications that require quick and flexible data storage solutions.
Common Pitfalls When Integrating MongoDB with Spring Boot
1. Incorrect Configuration Settings
One of the initial hurdles developers face is incorrect configuration settings. Spring Boot uses application.properties
or application.yml
files to configure the database connection.
Example Configuration
spring:
data:
mongodb:
uri: mongodb://localhost:27017/mydatabase
Ensure that your MongoDB server is running and that you provide the correct URI. Check the format meticulously because a small mistake can lead to frustrating connection errors.
2. Not Utilizing Spring Data MongoDB
Many developers transition from traditional databases and might overlook Spring Data MongoDB. This powerful framework offers easy integration with MongoDB, abstracts various operations, and supports reactive programming.
Why Use Spring Data MongoDB?
Using Spring Data simplifies CRUD operations, pagination, and sorting, making your code cleaner and easier to manage.
Example Repository
import org.springframework.data.mongodb.repository.MongoRepository;
public interface EmployeeRepository extends MongoRepository<Employee, String> {
Employee findByLastName(String lastName);
}
By extending MongoRepository
, you gain several methods without needing to implement them. This minimizes boilerplate code and enhances productivity.
3. Ignoring Exception Handling
When working with databases, exceptions are inevitable. Developers often fail to implement robust exception handling, which can lead to unresponsive applications.
Exception Handling Example
import org.springframework.dao.DataAccessException;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RestControllerAdvice;
@RestControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(DataAccessException.class)
public ResponseEntity<String> handleDataAccessException(DataAccessException ex) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR)
.body("Database error occurred: " + ex.getMessage());
}
}
By globally handling exceptions, you can provide meaningful feedback and avoid exposing stack traces to users.
4. Poor Data Modeling
When migrating from SQL to NoSQL, developers often replicate their SQL schemas directly into MongoDB. This may lead to complex data models that are difficult to maintain and inefficient to query.
A Better Approach
Instead of a highly normalized schema, consider using embedded documents and arrays. MongoDB allows for flexible JSON-like documents which are ideal for certain data relationships.
Example Model
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.mapping.Document;
@Document
public class Department {
@Id
private String id;
private String name;
private List<Employee> employees; // Embedded documents
// Getters and setters
}
5. Ignoring Reactive Programming
With the rise of asynchronous programming, developers often ignore the powerful reactive capabilities of Spring WebFlux and Spring Data MongoDB. This could be a major oversight for applications that require non-blocking I/O operations.
Why Consider Reactive Programming?
Using reactive programming allows your application to handle several requests simultaneously, thus improving responsiveness and resource utilization.
Reactive Repository Example
import org.springframework.data.mongodb.repository.ReactiveMongoRepository;
import reactor.core.publisher.Flux;
public interface ReactiveEmployeeRepository extends ReactiveMongoRepository<Employee, String> {
Flux<Employee> findByLastName(String lastName);
}
6. Lack of Indexing
Imagine querying a database without any indexes; the performance would be dismal. Yet, many developers forget to define indexes in their MongoDB collections, leading to slow queries.
Creating Indexes
You can create indexes by using the @Indexed
annotation in your entity classes.
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.index.Indexed;
import org.springframework.data.mongodb.core.mapping.Document;
@Document
public class Employee {
@Id
private String id;
@Indexed
private String lastName;
// Getters and setters
}
7. Failing to Leverage Query Derivation
Query derivation is a powerful feature that Spring Data provides allowing you to create queries by just declaring repository method names. However, many skip this feature, falling back on custom query implementations.
Example of Query Derivation
import java.util.List;
public interface EmployeeRepository extends MongoRepository<Employee, String> {
List<Employee> findByFirstNameAndLastName(String firstName, String lastName);
}
This abstraction makes it easier to write queries without getting caught up in the intricacies of query syntax.
The Closing Argument
Integrating MongoDB with Spring Boot can immensely boost application efficiency, but it is essential to navigate the common pitfalls. From ensuring correct configuration settings to leveraging features like Spring Data, each step matters for smooth integration.
Key takeaways include:
- Getting configuration right is crucial.
- Make full use of Spring Data MongoDB capabilities.
- Enforce robust exception handling mechanisms.
- Model your data in a way that leverages MongoDB's strengths.
- Explore reactive programming for enhanced performance.
- Always consider indexing for better query performance.
- Don't underestimate the power of query derivation.
For further reading, consider the official Spring Data documentation and MongoDB’s official documentation. These resources will deepen your understanding of these technologies and help you avoid common pitfalls.
By being aware of these common mistakes and learning how to prevent them, you'll smoother the path to a successful integration of MongoDB with your Spring Boot applications. Happy coding!