Mastering @InjectMocks: Common Pitfalls to Avoid in Mockito
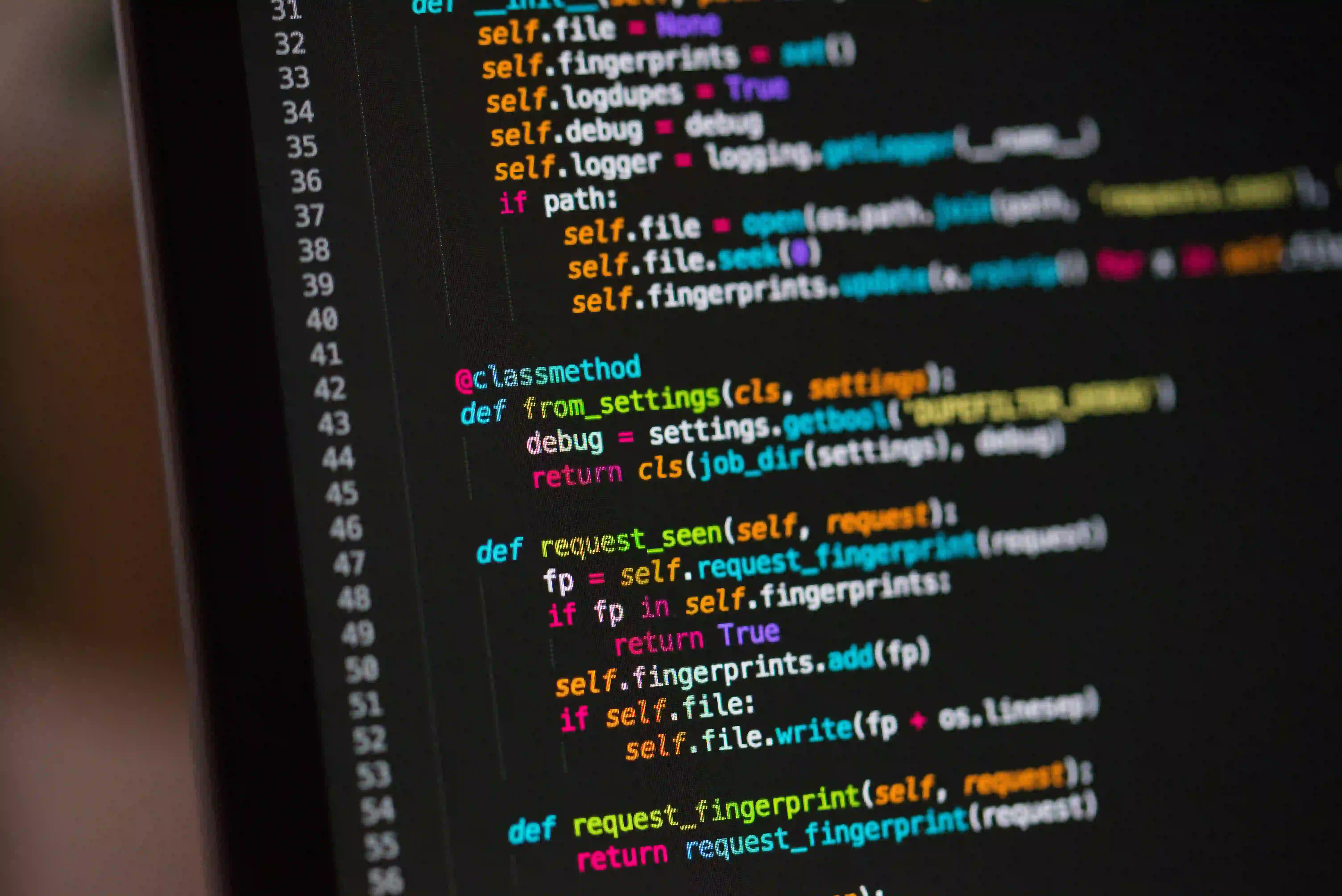
Mastering @InjectMocks: Common Pitfalls to Avoid in Mockito
Mockito is a powerful mocking framework for unit testing in Java. One of its most useful annotations is @InjectMocks
, which simplifies the creation of test doubles by automatically injecting mock dependencies into the class that you are testing. However, while @InjectMocks
can make tests concise and easier to read, it comes with its own set of common pitfalls. In this blog post, we’ll take a deep dive into @InjectMocks
, explore potential issues, and guide you on how to avoid them.
Understanding @InjectMocks
The @InjectMocks
annotation is part of the Mockito framework, a tool that allows developers to create mock objects for testing. What makes @InjectMocks
special is its ability to instantiate a class and automatically inject its dependencies using constructor injection or setter injection.
Here's how you typically use @InjectMocks
:
import static org.mockito.Mockito.*;
class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUserById(Long id) {
return userRepository.findById(id);
}
}
public class UserServiceTest {
@InjectMocks
private UserService userService;
@Mock
private UserRepository userRepository;
@Before
public void init() {
MockitoAnnotations.initMocks(this);
}
@Test
public void testGetUserById() {
User mockedUser = new User(1L, "John");
when(userRepository.findById(anyLong())).thenReturn(mockedUser);
User user = userService.getUserById(1L);
assertNotNull(user);
assertEquals("John", user.getName());
}
}
In this example, the UserService
class is tested by injecting a mock of UserRepository
. The @Mock
annotation creates a mock instance of UserRepository
that is then injected into UserService
.
Common Pitfalls When Using @InjectMocks
While @InjectMocks
simplifies dependency injection, there are several pitfalls to be aware of:
1. Non-Default Constructors
When your class under test does not have a default (no-argument) constructor, @InjectMocks
may fail to instantiate it. Instead, it will throw a MockitoException
.
Example of the Pitfall:
class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
}
Solution:
Ensure that you define a constructor that Mockito can use, or use the @InjectMocks
annotation together with relevant mock instances.
2. Misconfiguration of Mock Fields
If the fields that you want to mock are not properly annotated with @Mock
, Mockito will not inject them into your object. Your test will likely fail or return null for mock objects.
Example of the Pitfall:
public class SomeServiceTest {
@InjectMocks
private SomeService someService; // UserService requires UserRepository
@Mock
private UserRepository userRepository; // Oops! Missed this annotation
@Before
public void init() {
MockitoAnnotations.initMocks(this);
}
@Test
public void testDoSomething() {
// This could lead to NullPointerException due to missing @Mock
}
}
Solution:
Always double-check that all dependent fields are annotated with @Mock
or use MockitoAnnotations.openMocks(this)
in JUnit 5 to manage mocks more efficiently.
3. Unused Mocks Leading to Confusion
An injected mock that is never used in the test will not necessarily cause an error, but it can lead to confusion. When you have several mocks, ensure that each one actively participates in your tests.
Example of the Pitfall:
@Mock
private AnotherService anotherService; // Unused in the test
@Test
public void testAnotherMethod() {
// If you never call anotherService, it might not be clear why you included it.
}
Solution:
Use mocks in your tests or remove them to maintain a cleaner and more understandable codebase.
4. Circular Dependencies
Injecting mocks into classes that have circular dependencies can cause an error at runtime.
Example of the Pitfall:
class ClassA {
private ClassB classB;
public ClassA(ClassB classB) {
this.classB = classB;
}
}
class ClassB {
private ClassA classA;
public ClassB(ClassA classA) {
this.classA = classA;
}
}
public class CircularDependencyTest {
@InjectMocks
private ClassA classA;
@Mock
private ClassB classB; // This will cause a problem
}
Solution:
Refactor your classes to avoid circular dependencies. This often involves rethinking the design and responsibilities of your classes to ensure they are cohesive and maintainable.
5. Incorrect Usage of Argument Matchers
Using argument matchers incorrectly can lead to unexpected behavior, particularly with @InjectMocks
. For example, if you have strict matching and an actual value in a test, this can lead to failing tests.
Example of the Pitfall:
when(someService.doSomething("test")).thenReturn("mockedValue"); // Will cause an issue
Solution:
Use anyString()
or appropriate matchers instead of hardcoded values when you are uncertain about the input.
when(someService.doSomething(anyString())).thenReturn("mockedValue"); // Correct usage
To Wrap Things Up
Mastering @InjectMocks
in Mockito can significantly enhance your unit testing capability by providing a neat and efficient way to manage dependencies. However, it is essential to be aware of the common pitfalls that can arise when using this powerful feature.
By avoiding non-default constructors without proper handling, ensuring proper annotation of mock fields, removing unused mocks, handling circular dependencies with care, and avoiding incorrect usage of argument matchers, you can leverage Mockito to its fullest potential.
For more detailed insights into Mockito and its capabilities, consider checking the official Mockito documentation and exploring community discussions on best practices in software testing.
Remember, quality tests are the backbone of robust applications, and effective use of @InjectMocks
is a step towards achieving that.