Effortlessly Remove Docker Images Based on Custom Criteria
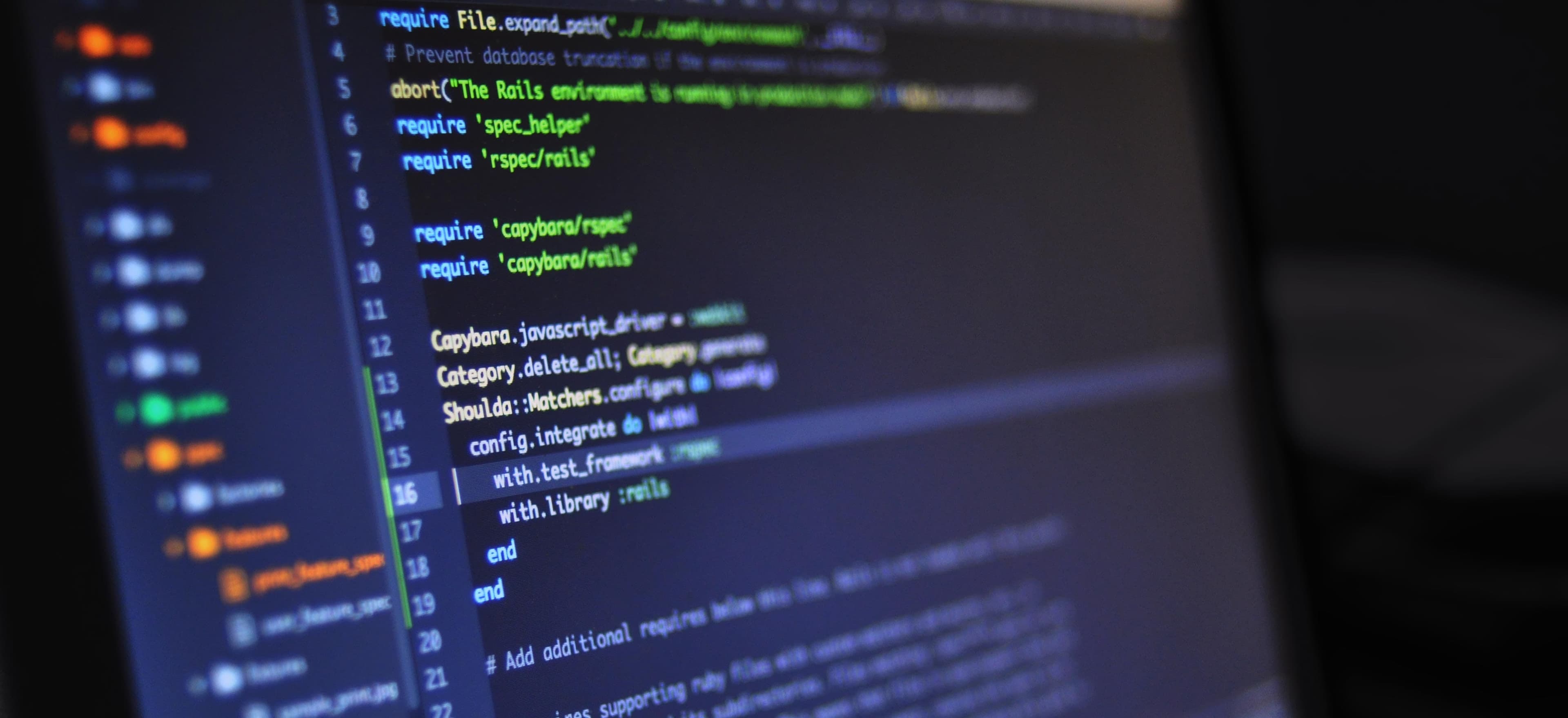
- Published on
Effortlessly Remove Docker Images Based on Custom Criteria
Docker is a powerful platform that allows developers to automate the deployment of applications within containers. However, as you use Docker, you may encounter issues with storage space due to the accumulation of unused or outdated Docker images. This blog post will guide you on how to efficiently remove Docker images based on custom criteria, ensuring your local environment remains clean without sacrificing your workflow.
Why Clean Up Docker Images?
Cleaning up Docker images is critical for several reasons:
- Storage Management: Unused images consume significant disk space, which can lead to issues with system performance.
- Performance: A cluttered Docker environment makes it difficult to find images, increase build times, and can affect the performance of Docker commands.
- Security: Old and unused images can pose security risks as they may contain vulnerabilities.
In this post, we will explore how to consolidate image management by implementing custom scripts to remove Docker images based on specified criteria like age, size, and status.
Prerequisites
To follow along with this guide, you should have:
- Docker installed on your machine. Install Docker
- Basic knowledge of Docker commands
- Familiarity with shell scripting
Understanding Docker Images
Before diving into the cleaning process, let's briefly discuss what Docker images are.
Docker images are read-only templates used to create containers. Images contain the application code, libraries, dependencies, and the environment necessary for the application to run. With each new version of your application or added library, new images can accumulate quickly.
Inspecting Docker Images
To see all Docker images present on your system, you can use the following command:
docker images
This command will return a list of all images with columns for REPOSITORY, TAG, IMAGE ID, CREATED, SIZE, and more.
Example Output
REPOSITORY TAG IMAGE ID CREATED SIZE
myapp latest 7ab7fca97368 2 weeks ago 256MB
ubuntu 20.04 a3b121130f86 3 months ago 64MB
Automating Cleanup with Custom Criteria
The crux of this post is to make removing Docker images smarter. Let's write a script to automate the cleanup process based on specific criteria.
Removing Images Older than a Certain Date
One common criterion for cleaning up images is age. For instance, you might want to delete Docker images that are older than a specific number of days. Below is a Bash script that fulfills this requirement:
#!/bin/bash
# Set the age threshold in days
DAYS_THRESHOLD=30
# Get the current date in seconds
CURRENT_DATE=$(date +%s)
# Loop through each image
docker images -q | while read -r IMAGE_ID; do
# Get the image creation date in seconds
IMAGE_DATE=$(docker inspect -f '{{.Created}}' "$IMAGE_ID")
IMAGE_DATE_SECONDS=$(date --date="$IMAGE_DATE" +%s)
# Calculate age in days
AGE=$(( (CURRENT_DATE - IMAGE_DATE_SECONDS) / 86400 ))
# Remove the image if it's older than the threshold
if [ "$AGE" -gt "$DAYS_THRESHOLD" ]; then
echo "Removing image ID $IMAGE_ID (created $AGE days ago)"
docker rmi "$IMAGE_ID"
fi
done
Why This Script Works
- Threshold Date: Setting a variable for the number of days allows you to adjust it easily.
- Looping Through Images: The script retrieves all image IDs and iterates over each one.
- Age Calculation: It converts the image creation date into seconds for simple calculation against the current date.
- Conditional Removal: If the image age exceeds the threshold, it is removed.
Removing Images Based on Size
Now, let’s consider another use case: removing images based on size. Below is a script that will help you delete images larger than a certain size (in megabytes).
#!/bin/bash
# Set the size threshold in MB
SIZE_THRESHOLD=500
# Loop through each image
docker images --format "{{.ID}} {{.Size}}" | while read -r line; do
IMAGE_ID=$(echo $line | cut -d ' ' -f 1)
IMAGE_SIZE=$(echo $line | cut -d ' ' -f 2)
# Convert the size to bytes for comparison
SIZE_UNIT=$(echo $IMAGE_SIZE | grep -o '[A-Z]*$') # Get size unit (MB/GB)
SIZE_VALUE=$(echo $IMAGE_SIZE | grep -o '^[0-9]*')
if [[ "$SIZE_UNIT" == "GB" ]]; then
SIZE_VALUE=$(( SIZE_VALUE * 1024 )) # Convert GB to MB
fi
# Remove the image if it's larger than the threshold
if [ "$SIZE_VALUE" -gt "$SIZE_THRESHOLD" ]; then
echo "Removing image ID $IMAGE_ID (size: $IMAGE_SIZE)"
docker rmi "$IMAGE_ID"
fi
done
Explanation of the Size Removal Script
- Size Formatting: The script retrieves image IDs and sizes in a formatted manner for easier parsing.
- Unit Handling: It checks the size unit (MB/GB) to conduct proper comparisons.
- Decision Making: Like the previous script, it conditionally removes images based on the specified size threshold.
Additional Cleanup Strategies
Apart from age and size, you might want to target other cleanup strategies such as:
- Removing dangling images: Images that are not tagged and not referenced by any container. You can execute the following command directly:
docker image prune
- Removing unused images: Clean up images that are not associated with any running container.
docker image prune -a
Wrapping Up
Maintaining a clean Docker environment plays a significant role in productivity, performance, and security. Automating the removal of Docker images based on custom criteria can save you time and effort, allowing you to focus on building and deploying your applications.
With the provided scripts, you can easily modify your cleanup routines based on age and size, as well as adapt them to suit additional criteria of your choice. Always test any script in a safe environment to avoid accidental loss of important images.
For further reading on Docker commands and their usage, check out the Docker Documentation.
Happy Dockering!
Checkout our other articles