Unlocking Performance: Mastering Thread Dump Analysis in Java
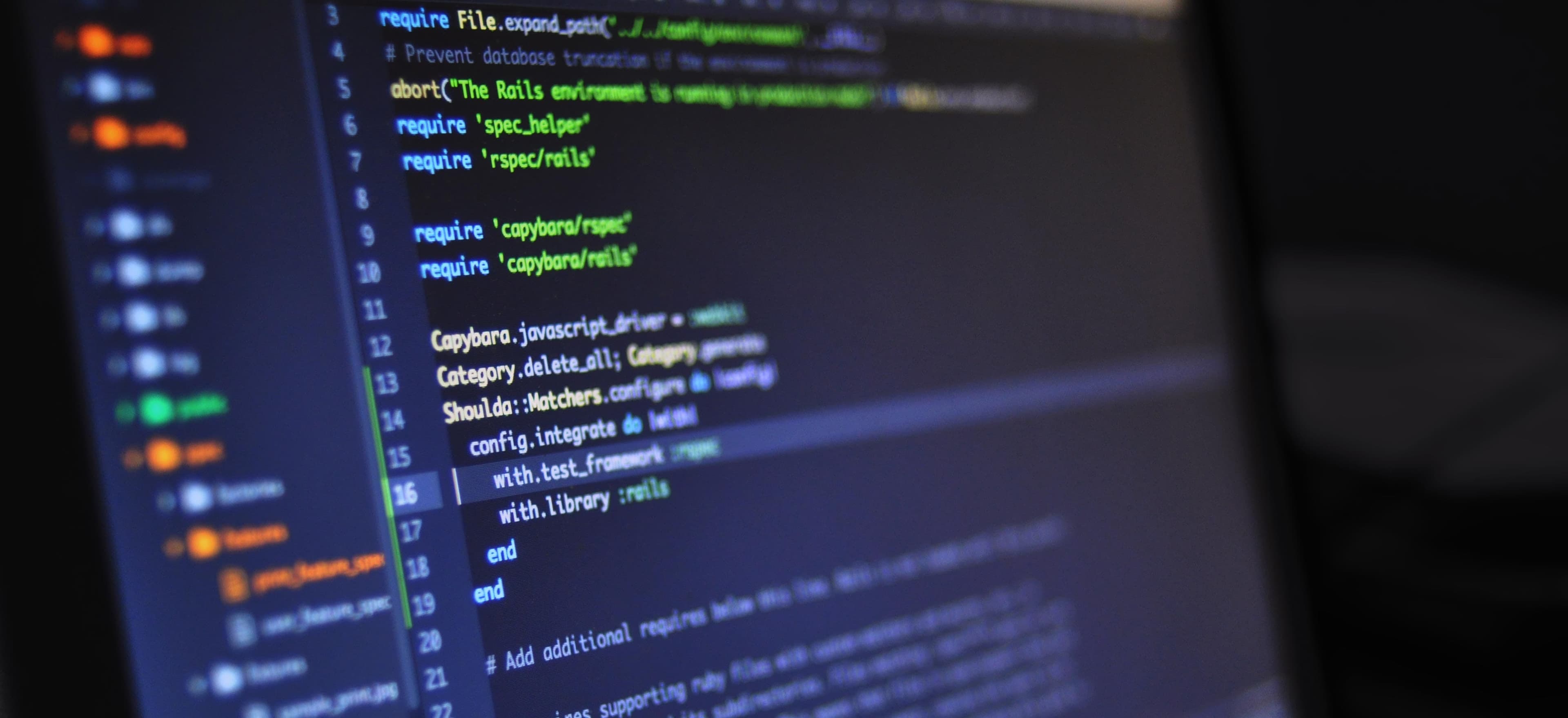
- Published on
Unlocking Performance: Mastering Thread Dump Analysis in Java
Java, as one of the most popular programming languages, powers countless applications worldwide. However, a common challenge that developers face is performance tuning. Among the various techniques available, analyzing thread dumps is one powerful approach to diagnose complex performance issues. In this post, we will explore how to analyze thread dumps effectively, providing code snippets and practical insights along the way.
What is a Thread Dump?
A thread dump is a snapshot of all the threads that are running within a Java Virtual Machine (JVM) at a specific point in time. It provides critical insights into the state of the application, including:
- The state of each thread (RUNNABLE, BLOCKED, WAITING, TERMINATED).
- Stack traces showing where each thread is executing.
- Locks held by threads, which can indicate contention issues.
Understanding thread dumps is key to optimizing the performance of Java applications.
How to Generate a Thread Dump
Before we dive into analysis, let's ensure you know how to generate a thread dump. You can create a thread dump in several ways:
-
Using JDK tools:
-
jstack
: This command-line utility is part of the Java Development Kit (JDK). You can run it as follows:jstack [pid]
Where
[pid]
is the Process ID of your Java application.
-
-
Using VisualVM:
- VisualVM is a powerful monitoring tool included in the JDK. Launch VisualVM and connect to your application. Then, navigate to the "Threads" tab and click on "Thread Dump".
-
Using kill command:
- On Unix-like systems, you can use the
kill
command (e.g.,kill -3 [pid]
). This sends a SIGQUIT signal to the Java process, spitting out a thread dump in the console.
- On Unix-like systems, you can use the
Breaking Down the Thread Dump
Once you have a thread dump, it's time to analyze it. Here's a basic structure of a thread dump:
"Thread-1" #11 prio=5 os_prio=0 tid=0x00007f997c000800 nid=0x1f6b runnable [0x00007f9977167000]
java.lang.Thread.State: RUNNABLE
at com.example.MyClass.myMethod(MyClass.java:20)
- locked <0x00000000c1fee648> (a java.lang.Object)
Key Components
- Thread Name: Identifies the thread (e.g., "Thread-1").
- Priority: The thread's priority level.
- State: Indicates the current state (RUNNABLE, BLOCKED, WAITING).
- Stack Trace: Shows method calls and line numbers.
Analyzing the State
The state of threads provides immediate insights into their behavior.
-
RUNNABLE: This means the thread is ready to execute or is currently executing. High numbers of runnable threads may indicate a CPU bottleneck.
-
BLOCKED: A thread is waiting for a monitor lock to enter a synchronized block or method. High levels of blocked threads can point to contention problems.
-
WAITING: This indicates that a thread is waiting indefinitely for another thread to perform a particular action (like
Object.wait()
).
Example: Identifying a Blocked Thread
Let's consider a hypothetical thread dump for analysis:
"Thread-2" #12 prio=5 os_prio=0 tid=0x00007f997c000800 nid=0x1f6c waiting for monitor entry [0x00007f9977156000]
java.lang.Thread.State: BLOCKED (on object monitor)
at com.example.MyClass.mySynchronizedMethod(MyClass.java:50)
- waiting to lock <0x00000000c1fee648> (a java.lang.Object)
- locked by "Thread-3" #13
What We Discover
- Thread-2 is in a BLOCKED state, trying to enter a synchronized method.
- The thread is waiting to acquire a lock held by Thread-3, which suggests that there may be a deadlock or significant contention.
This indicates a need to investigate synchronization in MyClass
. Perhaps consider refactoring the code to reduce locking contention.
public synchronized void mySynchronizedMethod() {
// Critical section
}
Possible Solutions
-
Reduce Scope of Synchronized Blocks: Only lock what is necessary within critical sections.
-
Use Reentrant Locks: These provide more sophisticated locking mechanisms, allowing threads to wait or try non-blocking attempts to acquire locks.
import java.util.concurrent.locks.ReentrantLock;
public class MyClass {
private final ReentrantLock lock = new ReentrantLock();
public void myMethod() {
lock.lock();
try {
// Critical section
} finally {
lock.unlock();
}
}
}
Tools for Thread Dump Analysis
While manual analysis is valuable, utilizing tools can expedite the process. There are various tools available:
-
FastThread: FastThread is an online thread dump analyzer that provides a detailed report, including thread states, stack traces, and lock details.
-
Samurai: Samurai is an open-source tool designed for Java thread dump analysis.
-
MAT (Memory Analyzer Tool): While predominantly used for heap dumps, MAT can also assist in analysis related to thread contention.
When to Use Thread Dumps
While thread dumps can be incredibly illuminating, they should be used judiciously. Here are some scenarios in which thread dumps are particularly effective:
- When applications freeze or are unresponsive.
- For diagnosing performance bottlenecks related to thread contention.
- When investigating stack overflow exceptions or deadlocks.
Best Practices for Thread Dumps
-
Collect Multiple Dumps: Collect thread dumps at intervals while the application experiences issues. This can show thread state changes and interactions over time.
-
Analyze with Context: Always have application context at hand. Know the expected behavior and workloads, which can guide your analysis.
-
Document Findings: Keep a record of your findings from thread dump analyses. This documentation can prove invaluable for future debugging.
-
Combine with Other Metrics: Use tools like Java Monitoring and Management Console (JMX) or APM tools like New Relic or Dynatrace for a more comprehensive understanding.
Final Thoughts
Thread dump analysis is a vital skill for any Java developer looking to optimize application performance. By understanding how to generate, read, and analyze thread dumps, you can unlock the potential for better performance, faster response times, and overall smoother user experiences.
Take the time to familiarize yourself with thread behaviors and use the resources available to continually learn and improve your analysis capabilities. Whether you're tackling a minor performance hiccup or navigating a sprawling enterprise application, thread dumps hold the keys to understanding your Java application's performance intricacies.
For further reading and exploration, consider checking out:
- Java Concurrency in Practice for an in-depth understanding of concurrency issues.
- Java Performance: The Definitive Guide for performance tuning strategies.
Happy coding and optimizing!
Checkout our other articles