Resolving Common Pitfalls in R2DBC Async Database Access
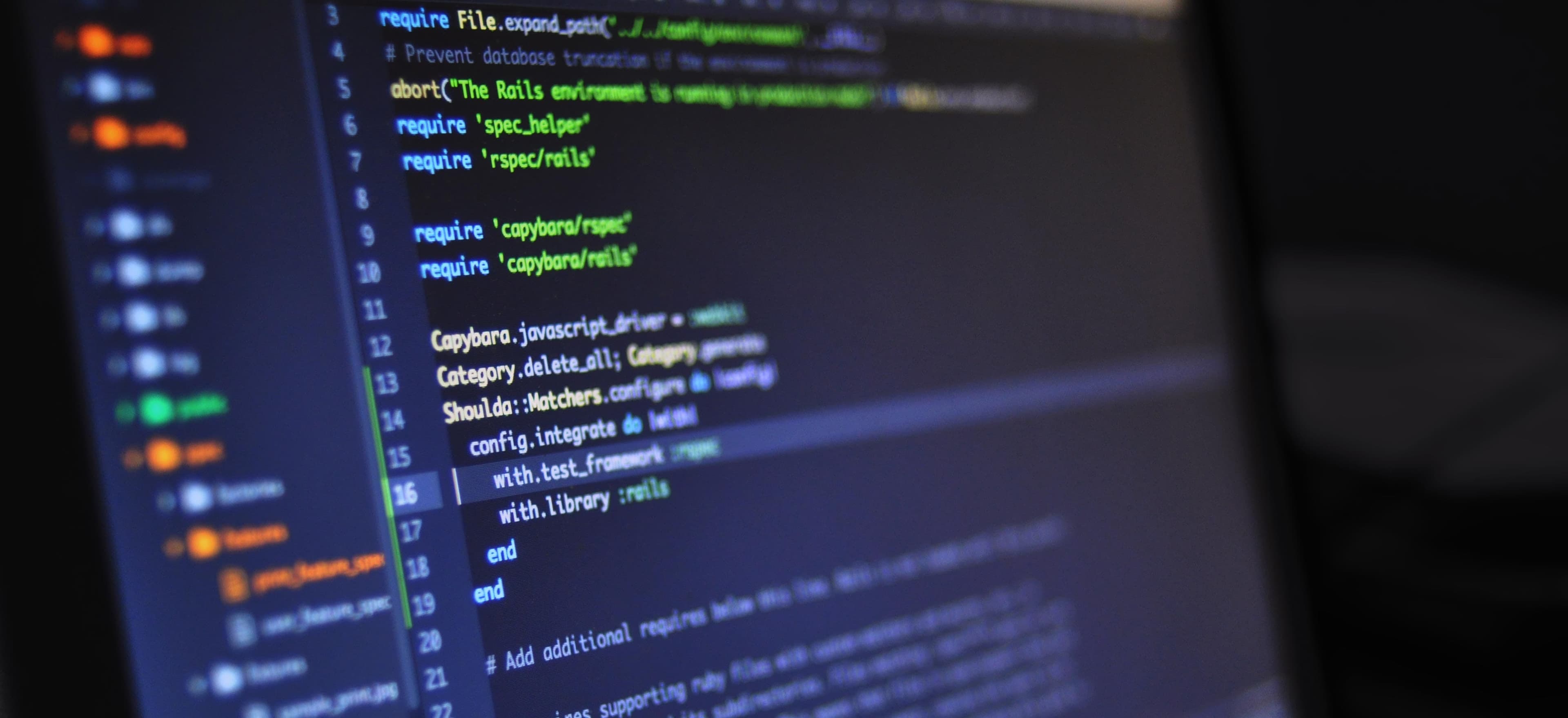
- Published on
Resolving Common Pitfalls in R2DBC Async Database Access
As the demand for responsive and efficient applications continues to grow, developers are increasingly looking for ways to enhance their database interactions. Reactive programming has become a prominent paradigm, with R2DBC (Reactive Relational Database Connectivity) stepping in to simplify the process of async database access in Java applications. However, while R2DBC promises non-blocking interactions, developers often encounter common pitfalls. In this blog post, we will explore these pitfalls and how to resolve them effectively, ensuring smoother and more efficient database interactions.
Understanding R2DBC
Before we delve into the pitfalls, let’s briefly understand R2DBC. R2DBC is a specification designed to provide a reactive programming interface for relational databases. Unlike traditional JDBC (Java Database Connectivity), which is synchronous and can lead to blocking operations, R2DBC allows for non-blocking connections. This is critical in environments where performance and scalability are paramount, such as microservices architecture.
Here’s how a basic R2DBC connection looks in code:
import io.r2dbc.spi.ConnectionFactory;
import io.r2dbc.spi.ConnectionFactoryOptions;
import io.r2dbc.spi.Row;
import reactor.core.publisher.Flux;
public class R2DBCExample {
private final ConnectionFactory connectionFactory;
public R2DBCExample() {
this.connectionFactory = ConnectionFactories.get(ConnectionFactoryOptions.parse("r2dbc:postgresql://user:password@localhost/dbname"));
}
public Flux<Row> fetchData() {
return Flux.usingWhen(
connectionFactory.create(),
connection -> connection.createStatement("SELECT * FROM my_table").execute(),
Result::getRows,
(connection, e) -> connection.close(),
connection -> connection.close()
);
}
}
Code Explanation
- ConnectionFactory: This is used to create connections to the database.
- Flux: Part of the Project Reactor, Flux represents an asynchronous sequence of 0 to N items. Here, we use it to fetch data from the database.
- usingWhen(): This method allows for safe resource management by ensuring the connection is closed after completing the operation.
This simple example illustrates the basic async interaction with a database using R2DBC, but its real power comes from effective error handling and proper resource management.
Common Pitfalls in R2DBC
1. Failing to Handle Exceptions Properly
In traditional JDBC, exceptions are often thrown directly, but in R2DBC, you must account for asynchronous error handling. Many developers overlook this, leading to silent failures or crashes.
Solution
Always define error handling in your reactive streams. Use operators like onErrorReturn
, onErrorResume
, or doOnError
.
fetchData()
.doOnError(e -> System.err.println("Database access error: " + e.getMessage()))
.onErrorReturn(Collections.emptyList()) // Return a default value on error
.subscribe(rows -> {
// Process rows
});
2. Ignoring Backpressure
When dealing with reactive streams, ignoring backpressure can lead to memory exhaustion and performance degradation. Here’s why this matters:
R2DBC handles streams of data, but if the consumer cannot keep up, data will pile up, causing out-of-memory errors.
Solution
Implement backpressure strategies. Utilize Flux
and Mono
operators that support backpressure, like limitRate()
.
fetchData()
.limitRate(2) // Limit the number of items requested
.subscribe(
row -> processRow(row),
throwable -> handleError(throwable)
);
3. Managing Database Connections Inefficiently
Creating and closing database connections can be expensive in terms of time and resources. Developers might establish new connections unnecessarily, leading to performance bottlenecks.
Solution
Use a connection pool. R2DBC implementations like R2DBC Pool provide efficient connection pooling mechanisms.
import io.r2dbc.pool.ConnectionPool;
import io.r2dbc.pool.ConnectionPoolConfiguration;
import io.r2dbc.spi.ConnectionFactory;
ConnectionPoolConfiguration config = ConnectionPoolConfiguration.builder(connectionFactory)
.maxSize(10) // Max connections in the pool
.build();
ConnectionPool connectionPool = new ConnectionPool(config);
// Usage
connectionPool.create().flatMap(connection ->
connection.createStatement("SELECT * FROM my_table").execute())
.flatMap(result -> result.map((row, metadata) -> row.get("column_name")))
.subscribe(value -> System.out.println(value));
This code sets up a connection pool, ensuring that connections are reused efficiently rather than constantly creating new ones.
4. Not Leveraging the Benefits of Reactive Programming
Many developers using R2DBC continue to write code in a blocking manner, missing out on the full benefits of reactive programming.
Solution
Embrace asynchronous programming patterns. Use flatMap
, subscribeOn
, and publishOn
to maximize performance and responsiveness.
fetchData()
.flatMap(row -> processRowAsync(row)) // Asynchronously process each row
.subscribe(result -> System.out.println("Processed data: " + result));
Here, flatMap
is applied to process each row asynchronously, taking full advantage of the reactive model.
Best Practices for R2DBC
- Use a Framework: Consider using frameworks like Spring Data R2DBC, which simplify database interactions, provide repository support, and allow you to focus on business logic.
- Error Handling: Implement global error handling strategies for your reactive streams to ensure consistency and reliability.
- Testing: Write comprehensive tests around your database interactions. Libraries like Testcontainers can help you spin up databases for testing purposes.
- Documentation: Read the official R2DBC documentation and familiarize yourself with reactive programming concepts.
For more on R2DBC best practices, you can check the R2DBC documentation.
To Wrap Things Up
While R2DBC opens up the doors to efficient and reactive database access in Java, it also brings along its own set of challenges. By understanding and addressing the common pitfalls—such as handling exceptions, managing connections effectively, and utilizing backpressure—you can significantly improve your application's performance and reliability.
As you continue your journey with R2DBC, remember to embrace the reactive paradigm fully and consider integrating frameworks that can ease your workload. Happy coding!
To deepen your knowledge, discover more about Project Reactor and its integration with R2DBC—both crucial components in the reactive ecosystem of Java applications.