Thread Pool Mismanagement: Common Java Executor Pitfalls
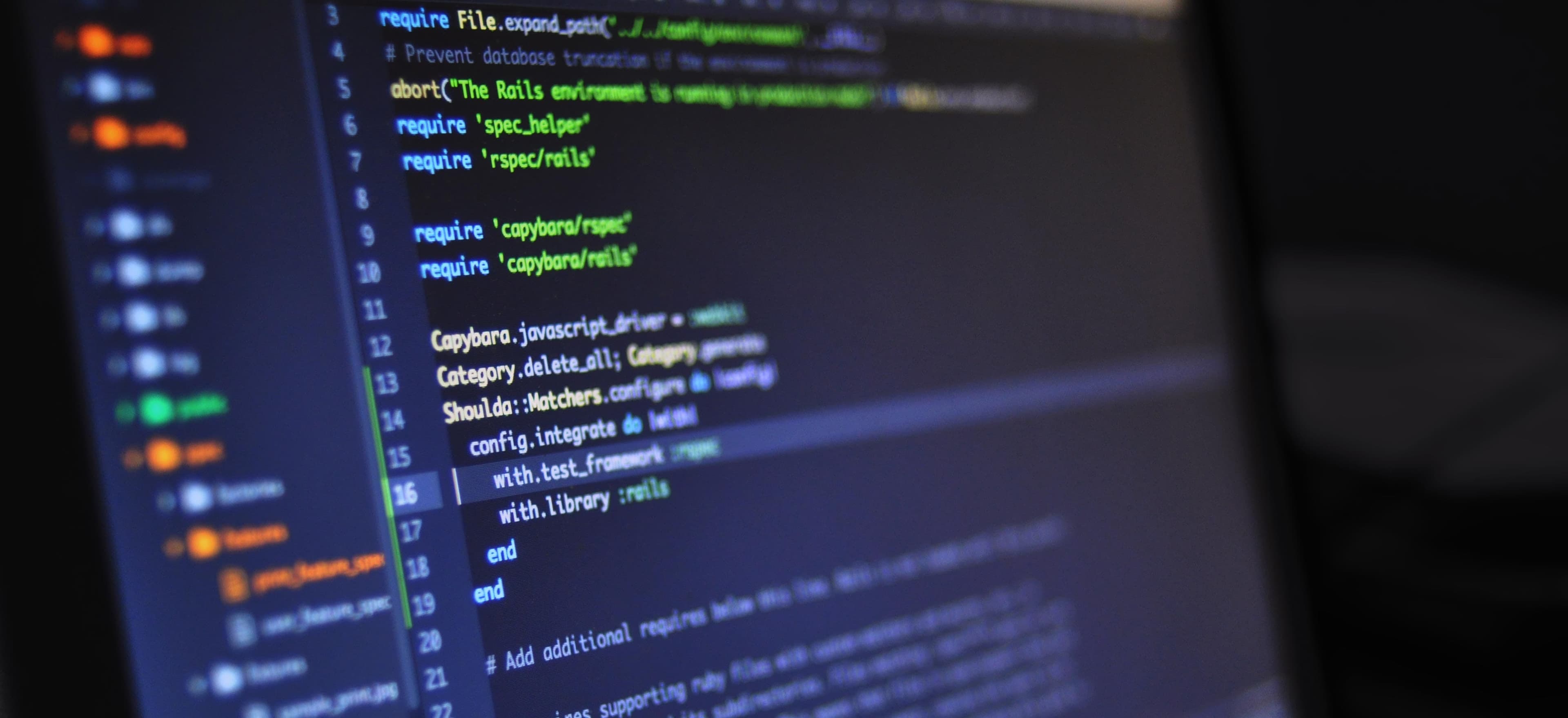
- Published on
Thread Pool Mismanagement: Common Java Executor Pitfalls
When it comes to writing multithreaded applications in Java, the Executor framework provides a robust way to manage threads. However, dealing with thread pools can be tricky, and mismanagement can lead to performance bottlenecks and unexpected behavior in your applications. This article delves into the common pitfalls encountered while managing Java Executors, how to avoid them, and best practices for optimal performance.
The Importance of Thread Pool Management
Thread pools serve as a means of efficiently reusing threads for executing tasks. Rather than creating and destroying threads on-the-fly, which is resource-intensive, a thread pool maintains a set of active threads and manages the execution of tasks through these threads.
The Java Executor framework, introduced in Java 5, encapsulates this thread management in an elegant API. However, misuse could turn these powerful features into a source of chaos rather than order.
Common Pitfalls in Thread Pool Mismanagement
1. Not Configuring Thread Pool Size Properly
One of the most common mistakes developers make is failing to configure thread pool size appropriately.
Impact: Setting the size too low can result in tasks waiting to be executed, leading to increased response times and potential application bottlenecks. On the other hand, setting it too high can lead to resource exhaustion and can degrade performance due to context switching.
Solution: Use the properties of your application and server environment to inform the configuration. The best practice is to set the thread pool size based on core CPU count, application load, and task characteristics.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
public static void main(String[] args) {
// Fetch the number of available processors
int coreCount = Runtime.getRuntime().availableProcessors();
// Creating a fixed thread pool based on the number of processors
ExecutorService executorService = Executors.newFixedThreadPool(coreCount);
// Submit tasks to the executor
for (int i = 0; i < 100; i++) {
executorService.submit(new Task(i));
}
}
static class Task implements Runnable {
private final int taskId;
Task(int taskId) {
this.taskId = taskId;
}
@Override
public void run() {
System.out.println("Executing Task ID: " + taskId);
// Simulate task execution
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
System.out.println("Task interrupted: " + taskId);
}
}
}
}
Key Takeaway: Monitor your thread pool performance using Java Management Extensions (JMX) or other profiling tools. This real-time data will inform adjustments to pool configuration.
2. Using the Wrong Type of Executor
Java provides various types of Executor implementations. Choosing the wrong one can lead to significant increases in latency and the inability to handle all submitted tasks.
Impact: Failing to assess your application needs can lead to using newCachedThreadPool()
for tasks that may stay in the system longer than anticipated, leading to resource leaks.
Solution: Evaluate the nature of your tasks. For long-running tasks, prefer newFixedThreadPool()
. If tasks are of unpredictable duration, newCachedThreadPool()
may be more appropriate.
// Example of using a cached thread pool
ExecutorService cachedThreadPool = Executors.newCachedThreadPool();
for (int i = 0; i < 50; i++) {
cachedThreadPool.submit(new Task(i));
}
Key Takeaway: Understand your workload. A short-lived, sporadic task model suits a cached pool, whereas a more predictable workload demands fixed or scheduled pools.
3. Failing to Shutdown Executors
When finishing the lifecycle of an application, it’s vital to shut down the Executor services. Neglecting this can result in memory leaks, as application threads linger unnecessarily.
Impact: Leaving threads active can prevent the JVM from exiting, causing wasted resources.
Solution: Always invoke shutdown()
or shutdownNow()
on your Executors when they are no longer needed.
// Shutdown Example
class Application {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(5);
// Execute tasks...
// Proper shutdown
executor.shutdown();
try {
if (!executor.awaitTermination(60, TimeUnit.SECONDS)) {
executor.shutdownNow(); // Force shutdown if necessary
}
} catch (InterruptedException ex) {
executor.shutdownNow();
}
}
}
Key Takeaway: Always ensure proper shutdown in a finally
block or use try-with-resources if applicable to guarantee clean termination of resources.
4. Not Handling Exceptions in Tasks
A common oversight in task management is the failure to handle exceptions properly. Uncaught exceptions can terminate threads unexpectedly, leading to incomplete task execution.
Impact: If a thread in a fixed thread pool encounters an exception, it will terminate, and the pool will not recover from it. Tasks assigned to that thread will thus never be executed.
Solution: Use Future
and handle exceptions gracefully. Implement robust error handling to log errors or retry tasks as necessary.
ExecutorService executorService = Executors.newFixedThreadPool(3);
Future<?> future = executorService.submit(() -> {
// Task code that might throw an exception
throw new RuntimeException("Simulated Exception");
});
try {
future.get();
} catch (ExecutionException e) {
System.out.println("Error during Task Execution: " + e.getCause());
} catch (InterruptedException e) {
Thread.currentThread().interrupt(); // Restore the interrupt status
}
Key Takeaway: Make use of the Future
API to regain control over task execution and properly manage exceptions.
5. Ignoring Task Queues
Each Executor can be customized with different types of task queues, allowing finer control over how tasks are submitted and executed.
Impact: Mismanagement of task queues can lead to overflow, resulting in application slowdown and unresponsive behavior.
Solution: Consider using bounded queues where necessary, like a LinkedBlockingQueue
, to limit the number of tasks waiting in the queue.
BlockingQueue<Runnable> queue = new LinkedBlockingQueue<>(50);
ExecutorService executorService = new ThreadPoolExecutor(5, 10,
60L, TimeUnit.SECONDS, queue);
Key Takeaway: Understanding queues and their characteristics helps mitigate situations where the system can be overwhelmed by excessive task submissions.
Key Takeaways
Effective management of Java Executors is crucial for creating responsive, high-performance applications. By avoiding the common pitfalls of thread pool mismanagement discussed in this article—such as improper pool configuration, choosing the wrong type of executor, neglecting shutdown procedures, inadequate exception handling, and ignoring task queues—you can significantly enhance the performance and reliability of your applications.
Always remember to assess your workload characteristics and choose the appropriate executor type, and maintain diligent monitoring and management of your thread pools. For further reading on Java Thread Pools and the Executor framework, consider checking out the official Java documentation.
By following these best practices, you will pave the way for improved concurrency management within your Java applications, unlocking the power of multithreading in an efficient manner. Happy coding!
Checkout our other articles