Choosing the Right Java Testing Framework: JUnit vs TestNG
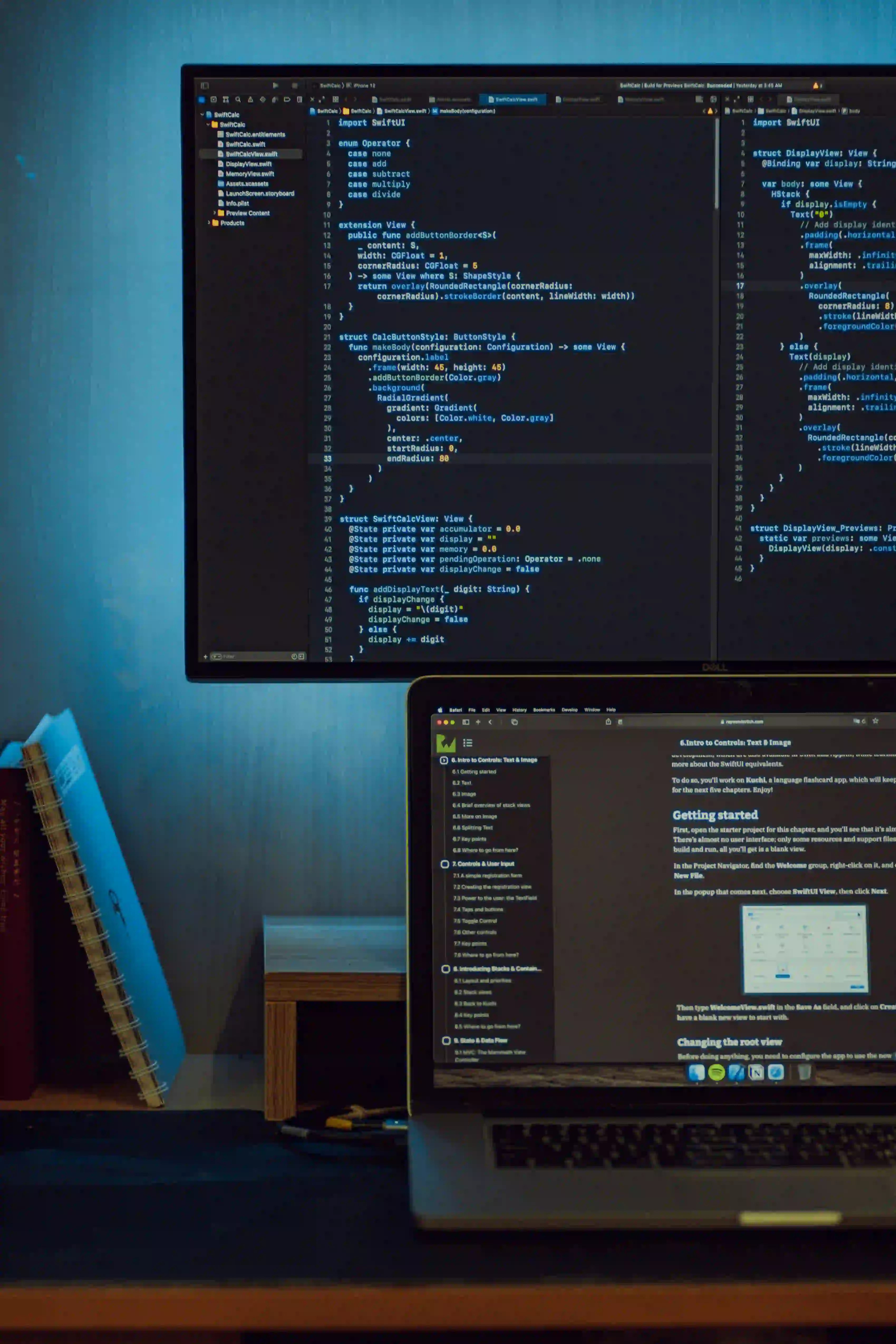
Choosing the Right Java Testing Framework: JUnit vs TestNG
In the realm of software development, testing is an integral part of the process. It ensures that your code is reliable, efficient, and bug-free. As a Java developer, you are likely to encounter various testing frameworks, each with unique features and capabilities. Two of the most popular testing frameworks are JUnit and TestNG. In this blog post, we will delve into the strengths and weaknesses of both frameworks, helping you make an informed decision for your testing needs.
What is JUnit?
JUnit is a widely used testing framework that provides a structured way to write and run tests for Java applications. It is a simple yet powerful framework that has been around since the early days of Java.
Key Features of JUnit
-
Annotation-Based Testing: JUnit uses annotations to mark test methods, making it easier to identify and categorize tests. Common annotations include
@Test
,@Before
,@After
, and@Ignore
. -
Assertion Methods: JUnit provides a suite of assertion methods that allow developers to validate test outcomes. You can assert equality, non-equality, and handle exceptions with methods like
assertEquals()
,assertNotNull()
, and others. -
Test Suites: With JUnit, you can group multiple test cases into a single test suite, running them together to save time and unitize functionality.
Here’s a simple example of a JUnit test case:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
Why this code? The test case above checks if the add
method in the Calculator
class correctly sums the numbers 2 and 3. The use of @Test
annotation indicates that this method is a test case, while assertEquals
ensures the expected output matches the actual output.
What is TestNG?
TestNG (Test Next Generation) is another powerful testing framework inspired by JUnit but designed to provide a more comprehensive testing suite. It caters to both unit and functional testing.
Key Features of TestNG
-
Flexible Configuration: TestNG allows for more flexible test configuration through XML files. You can define groups, data providers, and various execution parameters.
-
Annotations: TestNG has a broader range of annotations compared to JUnit. It offers
@BeforeClass
,@AfterClass
,@DataProvider
, and@Test(dependsOnMethods = ...)
, allowing for a more sophisticated level of control. -
Parallel Execution: One of TestNG’s standout features is its ability to execute tests in parallel. This is particularly useful for large test suites, significantly reducing the total testing time.
Here's how a simple TestNG test case looks:
import org.testng.Assert;
import org.testng.annotations.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
Assert.assertEquals(5, calculator.add(2, 3));
}
}
Why this code? Similar to the JUnit example, this TestNG test case validates the add
method. The use of Assert.assertEquals
functions similarly to JUnit's assertion, validating the functionality effectively.
Comparison: JUnit vs. TestNG
When deciding between JUnit and TestNG, it’s important to weigh their respective strengths and capabilities based on your project requirements.
Ease of Use
Both JUnit and TestNG are user-friendly. JUnit's simplicity makes it a more approachable option for developers who are new to testing. If you need straightforward unit tests, JUnit is a strong contender.
TestNG, however, provides more advanced features, beneficial for complex testing scenarios and integration testing. Developers familiar with XML configuration may appreciate the added flexibility.
Features and Functionality
-
Annotations: TestNG boasts a richer set of annotations, giving you more options for organizing and controlling the lifecycle of your tests. If you need to perform actions before or after class runs, TestNG shines here.
-
Data-Driven Testing: TestNG has built-in support for data-driven testing, allowing you to feed multiple sets of data into a single test method. This is particularly helpful in parameterized testing scenarios.
-
Parallel Execution: TestNG’s ability to run tests in parallel is a significant advantage for larger test suites, dramatically improving testing efficiency.
Community and Support
JUnit has been around longer than TestNG and boasts a larger community. Its established presence translates to abundant resources, tutorials, and documentation.
TestNG, while newer, has developed a solid community of users and contributors. It has gained traction among Selenium users, especially for automated browser testing.
When to Use JUnit
Use JUnit when:
- Your testing needs are relatively simple and straightforward.
- You require a quick setup with minimal configuration.
- You are working on small to medium-sized projects where basic unit tests suffice.
When to Use TestNG
Use TestNG when:
- You need a more flexible testing framework with advanced features.
- Your application has complex scenarios that involve integration testing or extensive data-driven testing.
- You wish to leverage parallel execution to save time.
My Closing Thoughts on the Matter
Choosing between JUnit and TestNG ultimately depends on your specific project needs and personal preferences. If you need simplicity and quick setup, JUnit is an excellent choice. However, if you require robust testing capabilities and advanced features, TestNG may be the way to go.
In modern software development, it is crucial to choose a testing framework that not only fits your immediate needs but one that also aligns with future development goals. Integrating testing as a core part of your development process is crucial for ensuring the reliability and maintainability of your Java applications.
For more detailed guides and resources, you can visit:
- JUnit Documentation
- TestNG Documentation
Decide wisely, and happy testing!