Nail Your Web Development Interview: Key Preparation Tips
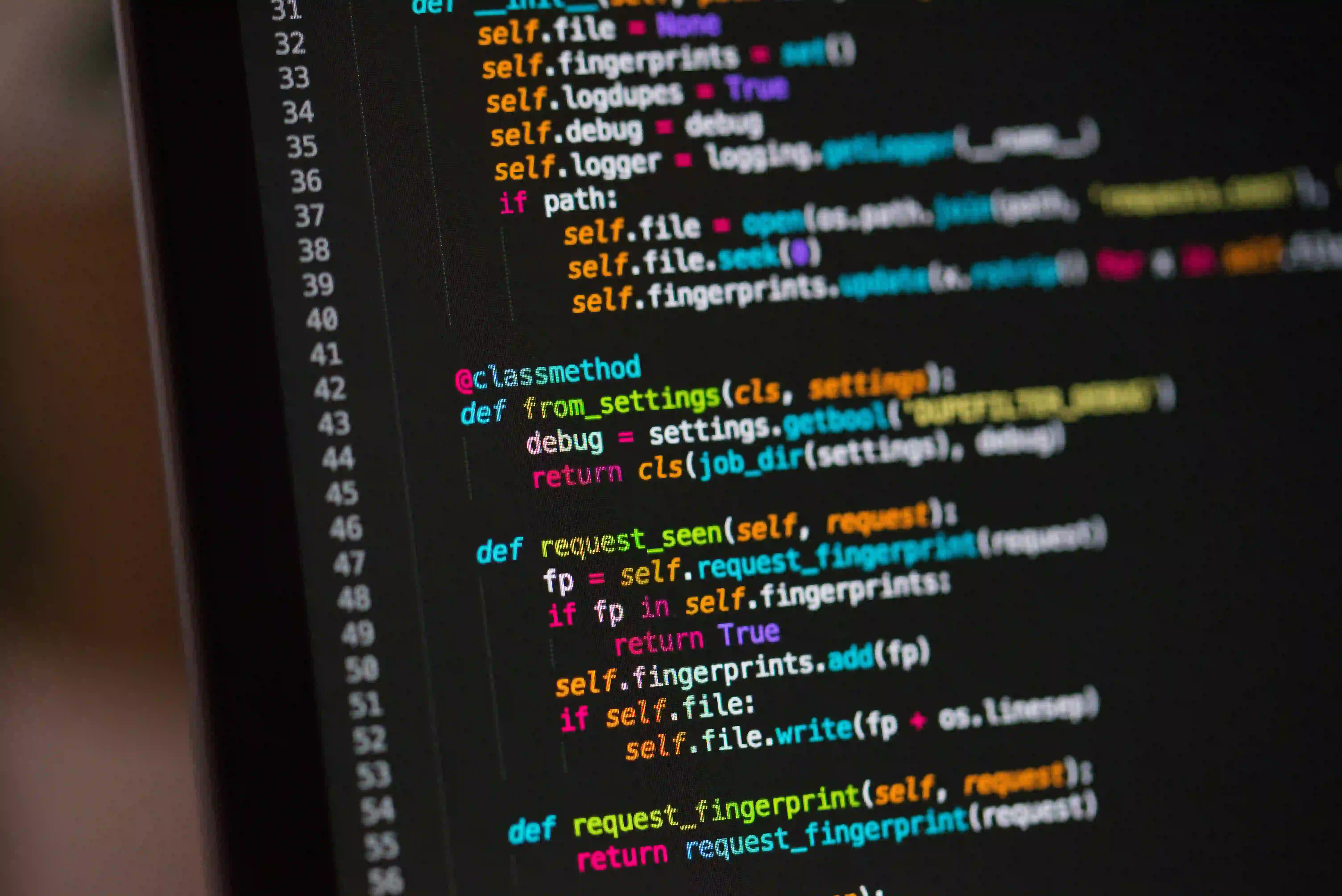
Nail Your Web Development Interview: Key Preparation Tips
Web development is a dynamic and ever-evolving field. With new technologies and frameworks emerging regularly, the demand for proficient web developers is on the rise. This guide aims to help you prepare effectively for your web development interviews, ensuring that you catch potential employers' attention and showcase your skills confidently.
Understanding the Web Development Landscape
Before diving into preparation tips, it's crucial to understand what web development encompasses. Generally, web development can be broken down into three primary areas:
-
Front-End Development: This is the client-facing part of a web application. It involves design and user experience, often utilizing languages like HTML, CSS, and JavaScript.
-
Back-End Development: This involves server-side logic, databases, and APIs that communicate data between the server and the client. Languages such as PHP, Ruby, Python, Java, and Node.js are commonly used.
-
Full-Stack Development: This combines both front-end and back-end development, enabling a developer to work on both sides of the application.
Having a clear understanding of these aspects will help you articulate your skills during the interview.
Key Preparation Tips
Now, let’s dive into the essential tips for preparing for your web development interview.
1. Master the Fundamentals
Why This Matters: Employers value solid foundational knowledge as it translates to better problem-solving skills and adaptability in using new technologies.
Actionable Steps:
- Review core concepts of HTML, CSS, and JavaScript.
- Familiarize yourself with key principles of responsive design and accessibility.
Code Snippet Example: A Basic Responsive Layout
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="styles.css">
<title>Responsive Design Example</title>
</head>
<body>
<header>
<h1>My Responsive Website</h1>
</header>
<main>
<section>
<p>This layout adjusts based on screen size!</p>
</section>
</main>
</body>
</html>
The above HTML structure is simple yet effective for showcasing a responsive website. The meta
tag for the viewport is essential for making the design adaptable to various screen sizes.
2. Deepen Your Knowledge in Frameworks
Why This Matters: Frameworks streamline development processes and increase productivity. Many companies use specific frameworks, and familiarity can be a significant advantage.
Actionable Steps:
- Choose a front-end framework (like React, Angular, or Vue.js) and learn its core concepts.
- Explore back-end frameworks such as Express.js for Node.js or Django for Python.
3. Build a Portfolio
Why This Matters: A well-curated portfolio not only showcases your skills but also reflects your passion for web development.
Actionable Steps:
- Create personal projects or contribute to open-source projects on platforms like GitHub.
- Share your work on platforms such as CodePen or your own website.
Example of a Simple JavaScript Project
const tasks = [];
function addTask(task) {
tasks.push(task);
console.log(`Task added: ${task}`);
}
function displayTasks() {
tasks.forEach((task, index) => {
console.log(`${index + 1}: ${task}`);
});
}
addTask("Learn JavaScript");
addTask("Build a web app");
displayTasks();
This code snippet defines a simple task manager in JavaScript. Building practical projects will bolster your portfolio and help you become proficient.
4. Prepare for Common Interview Questions
Why This Matters: Familiarity with common interview questions allows you to respond confidently and articulate your thoughts clearly.
Common Questions:
- What is the difference between
let
,const
, andvar
in JavaScript? - Explain the concept of promises in JavaScript.
- What are the key differences between REST and GraphQL?
Utilize resources like LeetCode or HackerRank to practice coding questions and algorithm challenges.
5. Familiarize Yourself with Version Control
Why This Matters: Most development teams use version control systems like Git for collaboration. Understanding Git is often a prerequisite.
Actionable Steps:
- Learn basic Git commands:
clone
,commit
,push
,pull
, andbranch
. - Understand how to work with branches and resolve merge conflicts.
Example of Basic Git Commands
# Initializing a new repository
git init
# Adding changes to the staging area
git add .
# Committing changes
git commit -m "Initial commit"
# Pushing to a remote repository
git push origin master
Familiarity with Git commands enhances collaboration and keeps your codebases organized.
6. Practice Problem-Solving Skills
Why This Matters: Many technical interviews involve solving coding problems on the spot. Practicing these will improve your algorithmic thinking.
Actionable Steps:
- Use platforms like Coderbyte or CodeSignal for coding challenges.
- Join community challenges like Advent of Code to sharpen your skills.
7. Research the Company
Why This Matters: Tailoring your responses and demonstrating knowledge about the company can set you apart from other candidates.
Actionable Steps:
- Review the company’s website, recent projects, and technologies they use.
- Prepare questions to ask about their tech stack and team dynamics.
Wrapping Up
Interview preparation can be daunting, but with the right strategy and a focused approach, you can navigate through successfully. By mastering the fundamentals, familiarizing yourself with frameworks, building a portfolio, and practicing problem-solving, you’ll enhance your prospects of landing that dream web development role.
Stay consistent in your preparation, and remember that every interview is a learning experience. Good luck with your preparation!
Further Resources
- FreeCodeCamp
- MDN Web Docs for HTML/CSS/JavaScript
- Codecademy
Crafting your skills and knowledge with care will surely help you nail your web development interview!