How to Stay Relevant in Java Development: Key Trends 2023
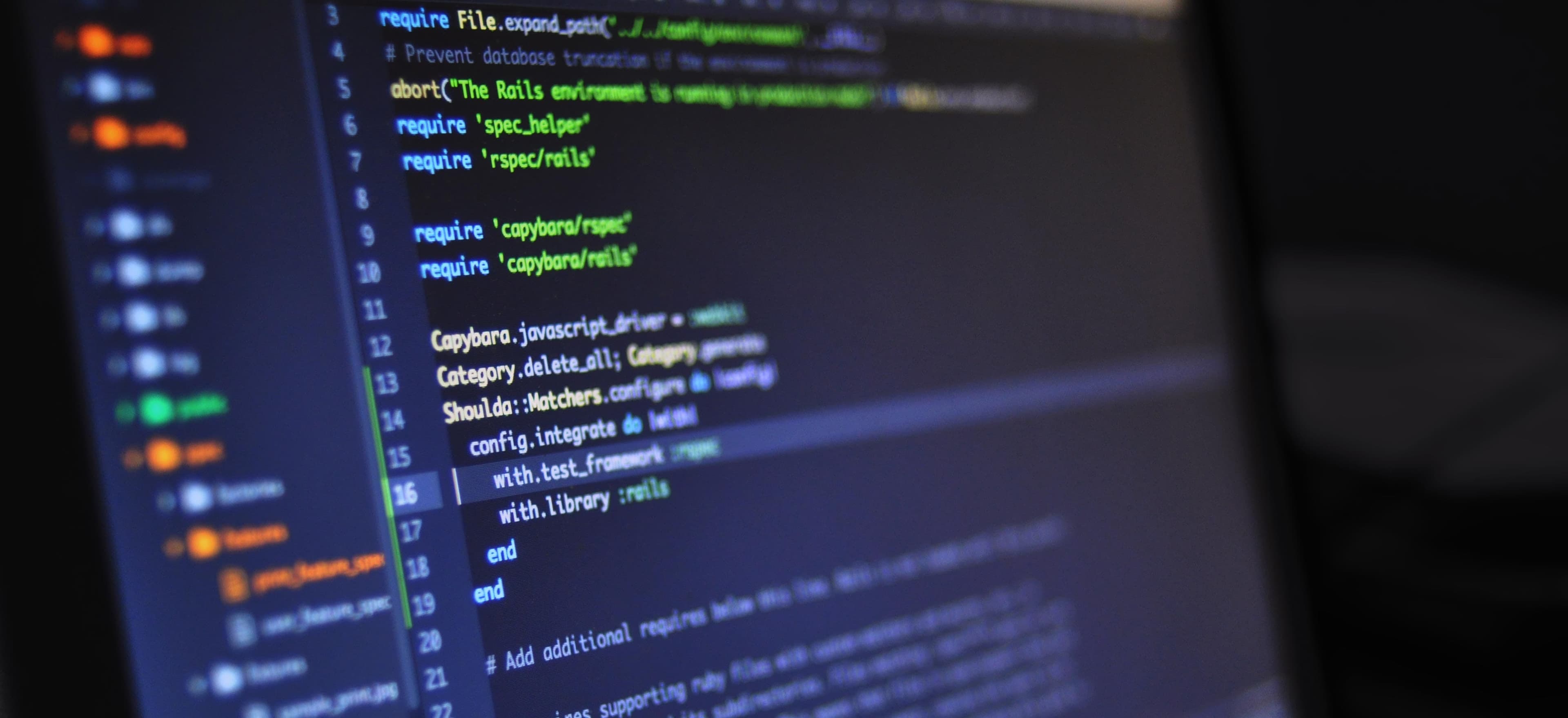
- Published on
How to Stay Relevant in Java Development: Key Trends 2023
Java has long been a stalwart in the world of programming languages, dominating areas such as enterprise applications, mobile applications, and server-side development. As we progress through 2023, Java is more relevant than ever, but to remain competitive, developers must stay abreast of the latest trends and technologies. In this article, we will discuss key trends in the Java ecosystem, important frameworks, and best practices to keep your skills sharp.
The Rise of Microservices
Microservices architecture has reshaped application design and deployment. Instead of building monolithic applications, developers have begun adopting a microservices approach for greater flexibility and scalability.
Why Microservices?
- Scalability: Each service can be scaled independently based on demand.
- Maintainability: Smaller codebases are easier to manage and debug.
- Flexibility: Different technologies can be used for different services, allowing optimization of each component.
Example Code Snippet for Microservices with Spring Boot
@RestController
@RequestMapping("/api/greetings")
public class GreetingController {
@GetMapping("/{name}")
public String greet(@PathVariable String name) {
return "Hello, " + name + "!";
}
}
Commentary: Here, we have a simple Spring Boot REST controller that acts as a microservice. By using the @RestController
annotation, we define an API endpoint that responds with personalized greetings. This microservice can operate independently of other services, making it easier to develop, deploy, and scale.
For more on microservices architecture, you can check out this comprehensive guide on Microservices.
The Continued Adoption of Cloud Technologies
Cloud computing has become a necessity rather than an option. Java developers are increasingly making use of cloud-native technologies to improve application performance and reduce infrastructure costs.
Cloud Technologies in Java
- Spring Cloud: Simplifies building cloud applications.
- Kubernetes: Manages containerized applications efficiently.
- AWS and Azure SDKs: These are essential for integrating with cloud services effectively.
Cloud Example
To utilize Spring Cloud for service discovery, you typically use Netflix Eureka:
@EnableEurekaServer
@SpringBootApplication
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
Commentary: The @EnableEurekaServer
annotation indicates that this application acts as a service registry. By allowing services to register themselves, this setup facilitates efficient routing and communication in a microservices environment. Stretch your knowledge of cloud technologies, as they are becoming a vital aspect of modern development.
Embracing Java 17 Features
Java 17, the latest Long-Term Support (LTS) release, introduces several noteworthy features that developers must utilize.
Notable Features in Java 17
- Sealed Classes: Control which classes can extend a particular class.
- Pattern Matching for
instanceof
: Simplifies type checks and casting. - New APIs: Enhancements in performance and ease-of-use with new methods in existing classes.
Example of Pattern Matching
if (obj instanceof String str) {
System.out.println("String length: " + str.length());
}
Commentary: This code snippet illustrates the new pattern matching feature in Java 17. It avoids the verbosity of traditional instanceof
checks, making the code cleaner and more readable. As Java continues to evolve, embracing the latest language features will help maintain relevance.
The Importance of DevOps Practices
With the continuous integration and continuous delivery (CI/CD) approach, Java developers must work closely with DevOps teams.
Benefits of DevOps for Java Developers
- Faster Releases: Streamlined processes lead to increased deployment frequency.
- Collaboration: Cross-functional teams promote better communication.
- Automation: Automated testing and deployment reduce human error.
Automated Testing Example with JUnit 5
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5");
}
}
Commentary: This JUnit 5 test case exemplifies automated testing in Java applications. It not only promotes code quality but also aligns with DevOps principles by ensuring that automated tests run during the CI/CD pipeline. Staying familiar with testing frameworks is critical for maintaining code robustness and reliability.
Focus on Reactive Programming
Reactive programming has gained momentum as applications need to handle numerous events and asynchronous data streams. Java's popular framework for reactive programming is Project Reactor.
Why Choose Reactive Programming?
- Responsiveness: Applications can remain responsive under high load.
- Event-driven: Easily manage streams of data and events.
- Reduced Resource Consumption: Efficiently uses resources with non-blocking I/O.
Example Code Snippet with Project Reactor
import reactor.core.publisher.Flux;
public class ReactiveExample {
public static void main(String[] args) {
Flux<String> flux = Flux.just("Java", "Spring", "Reactive");
flux.subscribe(System.out::println);
}
}
Commentary: This simple example creates a Flux
, which is a reactive data structure. By subscribing to the Flux
, we can process and consume each element asynchronously. With applications growing more complex, mastering reactive programming can be a distinct advantage.
Bringing It All Together
As Java evolves, it continues to be relevant and robust across various sectors. Staying updated with trends such as microservices, cloud technologies, Java 17 features, and DevOps practices is crucial for your development career. Through the integration of modern architectures like microservices and adopting progressive programming paradigms like reactive programming, developers can ensure they remain at the forefront of the technology landscape in 2023 and beyond.
Invest in continuous learning, practice through hands-on coding, and embrace new methodologies. Java is not just a language but a comprehensive ecosystem that demands adaptability and foresight. Make 2023 the year you enhance your skills and solidify your position as a competent Java developer.