Securing UI Components in Spring AOP: Common Pitfalls
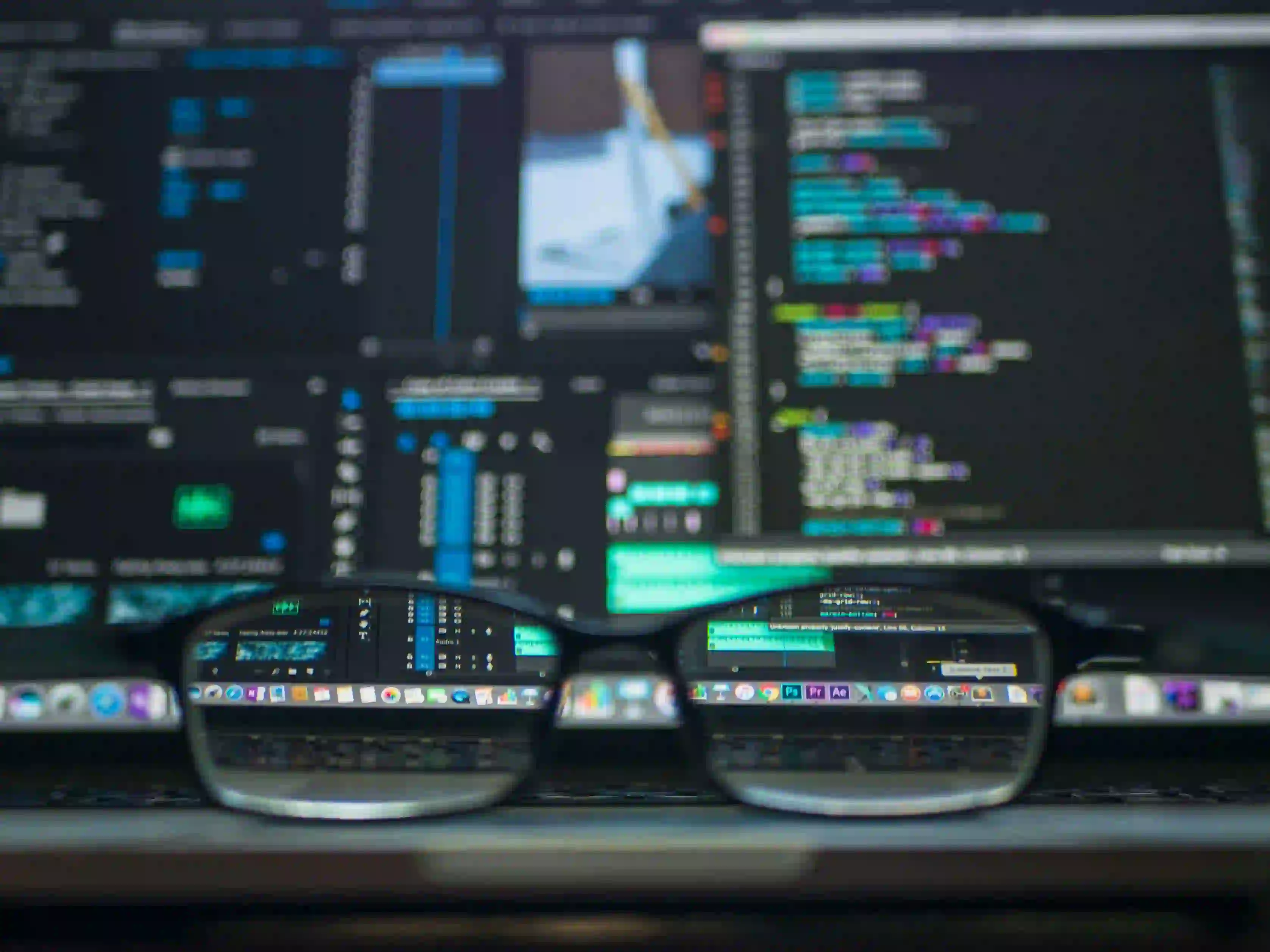
Securing UI Components in Spring AOP: Common Pitfalls
Securing UI components is a crucial aspect of building modern web applications. With Spring AOP (Aspect-Oriented Programming), developers can elegantly manage concerns like security. However, pitfalls can arise if best practices are not adhered to. In this blog post, we'll explore these common pitfalls in securing UI components using Spring AOP.
What is Spring AOP?
Spring AOP is part of the Spring Framework that provides support for aspect-oriented programming. It allows developers to define "aspects", which are reusable pieces of code that define behavior that can be applied to multiple parts of an application. The power of AOP lies in its ability to separate cross-cutting concerns, such as logging, transaction management, and security.
Why Secure UI Components?
Securing UI components is vital for several reasons:
- Protect sensitive data: Prevent unauthorized access to user information and application functionality.
- User trust: Users need to feel safe in their interactions with your application.
- Compliance: Regulations like GDPR and HIPAA require certain levels of security for handling user data.
Common Pitfalls
-
Not Defining Security Policies Clearly
One common pitfall is the lack of clearly defined security policies. Without a proper understanding of what should be secured, developers run the risk of leaving gaps in their security.
Why It Matters: Clear policies help in planning aspects appropriately.
Solution: Define a detailed security policy document that outlines what is to be guarded and how.
-
Overusing AOP for Security
A major misunderstanding is that every security concern needs an AOP solution. Developers sometimes use AOP where simpler solutions would suffice.
Why It Matters: Overhead from AOP can affect performance.
Example: If securing a single method can be done with annotation-based security like
@PreAuthorize
, then avoid adding the complexity of AOP.☕snippet.java@PreAuthorize("hasRole('ADMIN')") public void deleteUser(Long userId) { // Method to delete a user }
-
Not Using Proper Join Points and Pointcuts
Defining the right join points and pointcuts is crucial for ensuring that security aspects are applied where they are needed.
Why It Matters: Incorrectly defined join points can lead to missed security checks.
Example: Ensure that you're intercepting the right method calls. Specify pointcuts that accurately reflect your security needs.
☕snippet.java@Pointcut("execution(* com.example.service.*.*(..))") public void serviceLayer() {} @Before("serviceLayer()") public void checkUserPermissions(JoinPoint joinPoint) { // Security checks before executing service methods }
-
Ignoring the Order of AOP Aspects
AOP allows for multiple aspects to be applied. Ignoring the order can lead to security breaches since one aspect might override checks done by another.
Why It Matters: The order of aspects can change behavior fundamentally.
Example: Use
@Order
to control the execution order.☕snippet.java@Aspect @Order(1) public class SecurityAspect { // Security before method execution } @Aspect @Order(2) public class LoggingAspect { // Logging after method execution }
-
Using AOP in Insufficient Context
It’s crucial to ensure that your security context is valid within your aspects. Failing to do this can introduce vulnerabilities.
Why It Matters: An invalid security context can lead to unauthorized access.
Solution: Always verify that your security context information is current inside your aspect methods.
☕snippet.java@Before("serviceLayer()") public void checkUserAuthentication(JoinPoint joinPoint) { Authentication authentication = SecurityContextHolder.getContext().getAuthentication(); if (authentication == null || !authentication.isAuthenticated()) { throw new AccessDeniedException("User is not authenticated"); } }
-
Lack of Testing for Security Aspects
Security is frequently overlooked in testing. Automated tests that verify security annotations and aspects can catch potential vulnerabilities early in the development lifecycle.
Why It Matters: Regular security tests can prevent future breaches.
Example: Use Spring's test support to create tests that validate security aspects.
☕snippet.java@RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(classes = {AppConfig.class}) public class SecurityAspectTest { @Autowired private UserService userService; @Test(expected = AccessDeniedException.class) public void testDeleteUserWithoutPermission() { userService.deleteUser(1L); // Expect access denied here } }
Additional Resources
For further reading, you can explore the following resources:
- Spring AOP Documentation
- Spring Security Primer
- Best Practices for Implementing Security in Web Applications
The Bottom Line
Securing UI components with Spring AOP brings numerous advantages, but developers must be cognizant of common pitfalls. By defining clear policies, using AOP judiciously, ensuring appropriate join points, managing execution order, validating the security context, and emphasizing comprehensive testing, you can create a robust security framework for your web applications.
Incorporating these practices will not only protect your application but also enhance user trust, leading to a more successful software product. Embrace Spring AOP but use it wisely, and you will build a secure foundation for your UI components.
Feel free to ask questions or share your experiences in using Spring AOP for securing UI components in the comments below!