Mastering AnchorPane Animation for Dynamic JavaFX Layouts
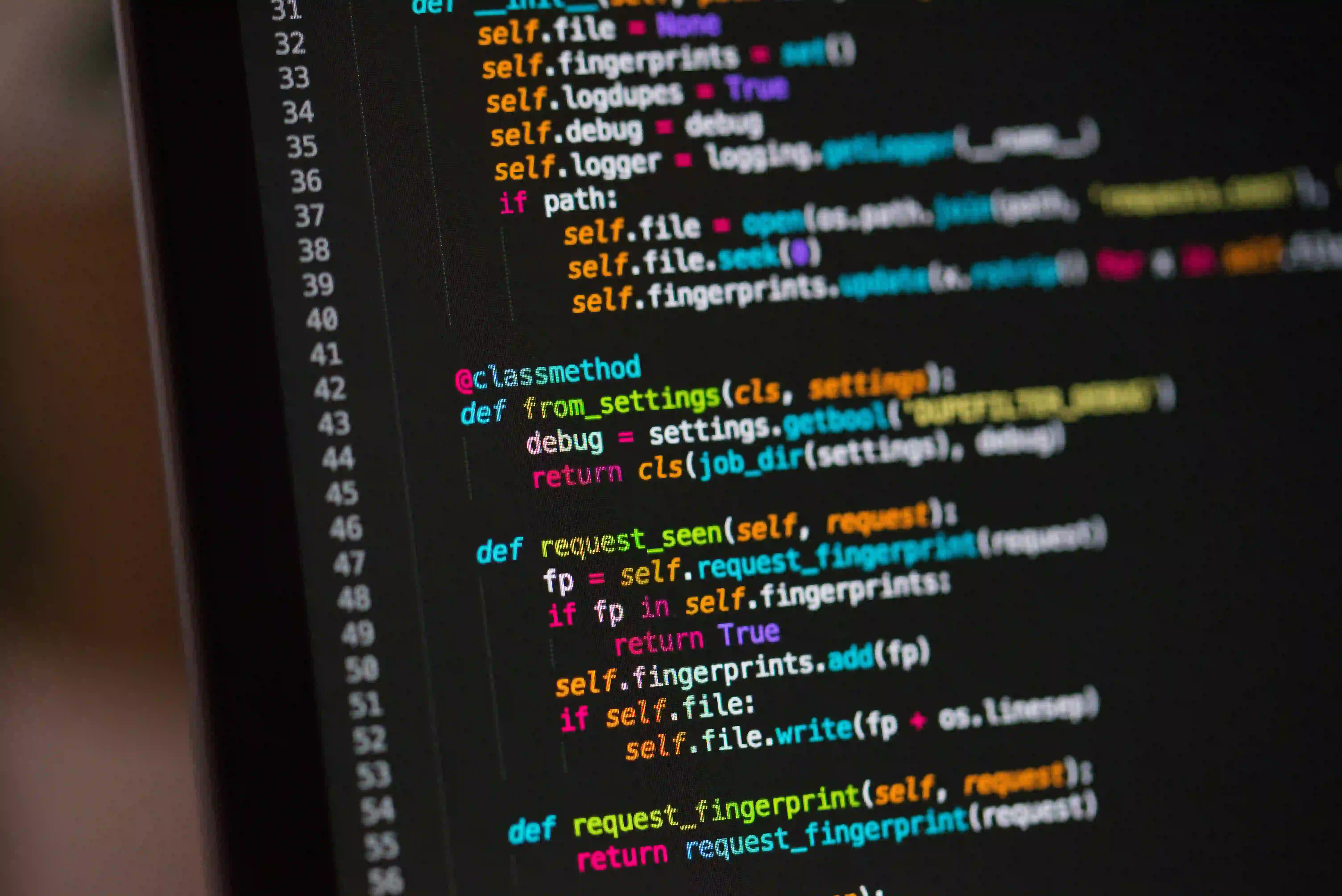
Mastering AnchorPane Animation for Dynamic JavaFX Layouts
JavaFX is a powerful framework for building sophisticated graphical user interfaces (GUIs) in Java. One of the layout containers that JavaFX provides is AnchorPane
, which offers a flexible way to position nodes based on their distance from the edges of the pane. In this article, we will dive into how to effectively animate components within an AnchorPane
, making your JavaFX applications visually appealing and dynamic.
Table of Contents
- Understanding AnchorPane
- Setting Up Your JavaFX Environment
- Basic Animation Techniques
- Creating Animations with AnchorPane
- Advanced Animation Techniques
- Conclusion
Understanding AnchorPane
The AnchorPane
layout is particularly useful because it allows you to anchor its children to the pane's edges. By setting the anchor values, you define how far the child nodes will be from the edges of the pane. This is useful for responsive design, where the layout adjusts dynamically as the window resizes.
Key Properties
- Top Anchor: The distance from the top edge of the
AnchorPane
. - Bottom Anchor: The distance from the bottom edge.
- Left Anchor: The distance from the left edge.
- Right Anchor: The distance from the right edge.
This flexibility makes AnchorPane
a favorite among developers looking to create responsive user interfaces easily.
Setting Up Your JavaFX Environment
Before jumping into animations, ensure your JavaFX environment is set up properly. Here are the basic steps:
- Install JDK: Ensure you have JDK 8 or higher installed.
- Set Up JavaFX: If you are using JDK 11 or higher, you will need to download the JavaFX SDK separately. Follow the JavaFX Installation Guide for instructions.
- Configure Your IDE: For IDEs like IntelliJ IDEA or Eclipse, make sure to add the JavaFX libraries to your project.
// Sample main application class
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.AnchorPane;
import javafx.stage.Stage;
public class AnchorPaneDemo extends Application {
@Override
public void start(Stage primaryStage) {
AnchorPane anchorPane = new AnchorPane();
Scene scene = new Scene(anchorPane, 800, 600);
primaryStage.setTitle("AnchorPane Animation Demo");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Basic Animation Techniques
In JavaFX, there are several ways to animate nodes. You can use the built-in TranslateTransition
, FadeTransition
, or other transition classes. Every transition class allows smooth and efficient animations with configurable properties such as duration and delays.
Example: Basic Fade Transition
The following snippet shows how to create a simple fade transition for a button.
import javafx.animation.FadeTransition;
import javafx.scene.control.Button;
import javafx.util.Duration;
// Create a button
Button button = new Button("Hover me");
// Create a fade transition
FadeTransition fadeTransition = new FadeTransition(Duration.seconds(1), button);
fadeTransition.setFromValue(1.0);
fadeTransition.setToValue(0.5);
fadeTransition.setCycleCount(FadeTransition.INDEFINITE);
fadeTransition.setAutoReverse(true);
// Start the animation on mouse entered event
button.setOnMouseEntered(e -> fadeTransition.play());
button.setOnMouseExited(e -> fadeTransition.stop());
Why Use Fade Transition?
Fade transitions are great for creating subtle visual feedback in your applications. They enhance user experience and indicate that an element is interactive.
Creating Animations with AnchorPane
Now that you have a basic understanding of animations, let's move on to animating children within an AnchorPane
. The following example demonstrates how to animate a rectangle moving and resizing within an AnchorPane
.
Example: Rectangle Animation
import javafx.animation.TranslateTransition;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
Rectangle rectangle = new Rectangle(100, 100, Color.BLUE);
AnchorPane.setTopAnchor(rectangle, 100.0);
AnchorPane.setLeftAnchor(rectangle, 100.0);
anchorPane.getChildren().add(rectangle);
// Create a translate transition
TranslateTransition translateTransition = new TranslateTransition(Duration.seconds(2), rectangle);
translateTransition.setFromX(0);
translateTransition.setToX(400);
translateTransition.setCycleCount(TranslateTransition.INDEFINITE);
translateTransition.setAutoReverse(true);
// Start the animation
translateTransition.play();
How It Works
- Rectangle Creation: A rectangle is created and positioned within the
AnchorPane
. - TranslateTransition: The transition moves the rectangle horizontally across the pane.
- Cycle and AutoReverse: The animation will repeat indefinitely and reverse once it reaches the end.
Advanced Animation Techniques
Once you have mastered the basics, consider using more complex animations or combining multiple transitions. This allows for visually striking effects and a more dynamic user experience.
Example: Sequential Animation
Here’s how you can use the SequentialTransition
to chain animations.
import javafx.animation.SequentialTransition;
import javafx.animation.ScaleTransition;
Rectangle square = new Rectangle(100, 100, Color.GREEN);
AnchorPane.setTopAnchor(square, 250.0);
AnchorPane.setLeftAnchor(square, 250.0);
anchorPane.getChildren().add(square);
// Create scale transition
ScaleTransition scaleTransition = new ScaleTransition(Duration.seconds(1), square);
scaleTransition.setFromX(1);
scaleTransition.setToX(1.5);
scaleTransition.setCycleCount(2);
scaleTransition.setAutoReverse(true);
// Create translate transition
TranslateTransition moveTransition = new TranslateTransition(Duration.seconds(1), square);
moveTransition.setByX(200);
moveTransition.setCycleCount(2);
moveTransition.setAutoReverse(true);
// Create sequential transition
SequentialTransition sequentialTransition = new SequentialTransition(scaleTransition, moveTransition);
sequentialTransition.setCycleCount(SequentialTransition.INDEFINITE);
sequentialTransition.play();
Explanation
- Scale Transition: This enlarges the rectangle and returns it to its original size.
- Translate Transition: This moves the rectangle back and forth horizontally.
- Sequential Transition: Combines both animations, allowing them to run in sequence, enhancing user engagement.
Lessons Learned
Animating elements in an AnchorPane
using JavaFX not only improves your application's aesthetics but also provides an interactive experience for users. By utilizing the built-in transition classes, you can create seamless animations that enrich the overall user experience.
To further explore JavaFX capabilities, consider Oracle's JavaFX Documentation for comprehensive learning. Happy coding, and may your applications impress users with their dynamic interfaces!
This engaging overview blends foundational knowledge with practical examples, urging developers to explore the vast potential of JavaFX and its animation capabilities. The further you delve, the more you'll uncover about creating dynamic, user-friendly applications.