Unlocking Learning: The Challenges of Static Analysis Education
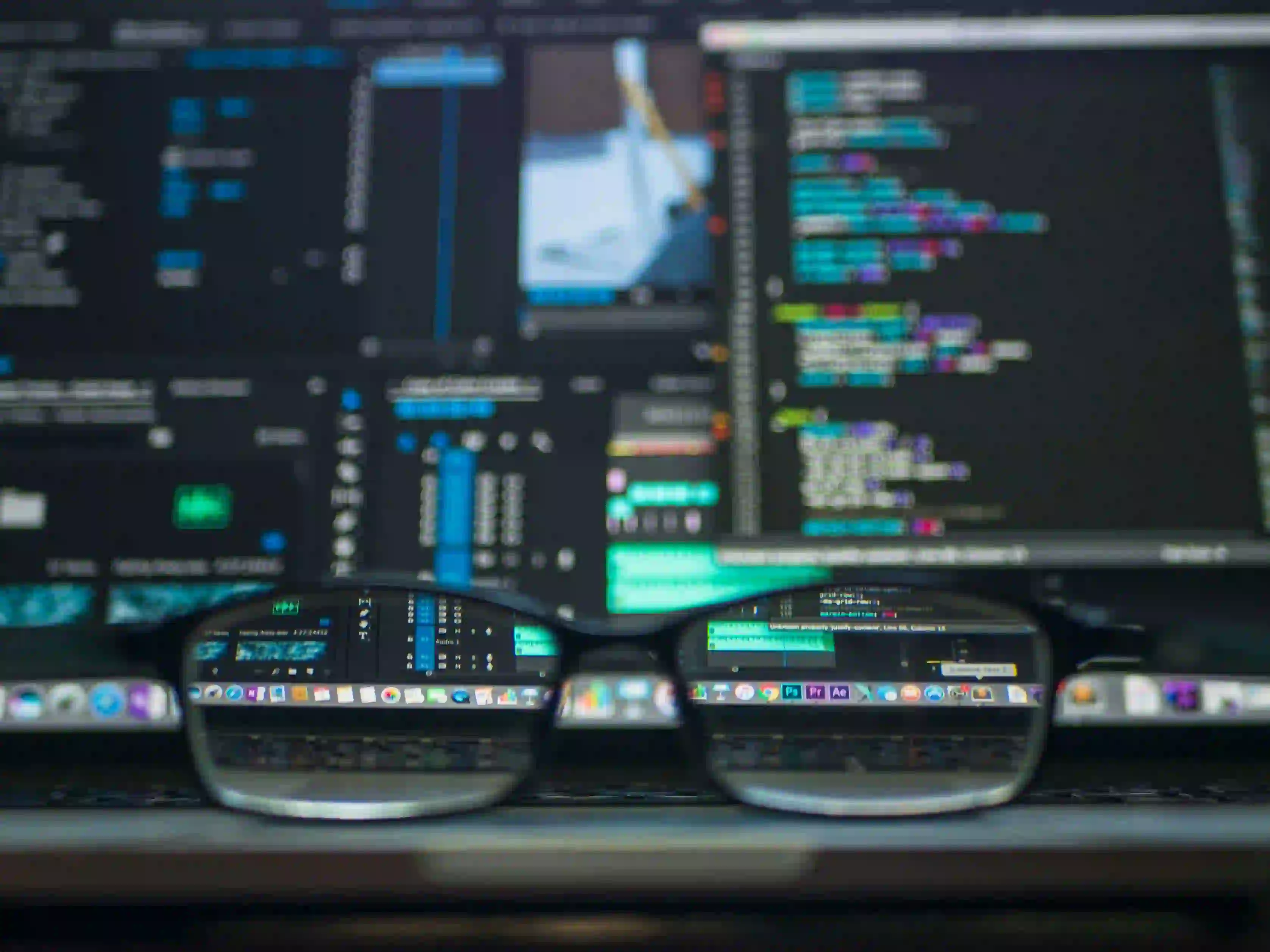
Unlocking Learning: The Challenges of Static Analysis Education
Static analysis is a crucial component of software development, offering a method to analyze code for potential errors without executing it. As we dive into the intricacies of static analysis education, we uncover several challenges that educators and learners encounter. This blog post aims to explore these challenges, providing insights and potential solutions for effective static analysis learning.
Understanding Static Analysis
Static analysis tools review source code for compliance with best practices, code standards, vulnerabilities, and various other metrics. Tools like SonarQube, ESLint, and PMD help developers identify issues early in the development process, leading to higher code quality and reduced bugs.
However, educating aspiring developers about static analysis presents unique hurdles. Let's delve into these challenges.
Challenge #1: Complexity of Concepts
The Problem
Static analysis encompasses several layers of complexity, from understanding abstract syntax trees to grasping various heuristics for detecting anomalies. For many students, especially those new to programming, these concepts can be daunting.
Solutions
-
Breaking Down Topics:
- Start with foundational principles in computer science before diving into static analysis specifics. Concepts such as syntax trees or control flow can be discussed in simpler terms.
-
Real-world Examples:
- Use practical examples to demonstrate the value of static analysis. Highlight cases where static analysis has caught significant bugs or vulnerabilities, such as in high-stakes applications like healthcare software.
Example Code
Here’s a simple code snippet to demonstrate static analysis in Java:
public class Example {
public static void main(String[] args) {
// Example of a potential null pointer dereference
String str = null;
System.out.println(str.length());
}
}
This code snippet can generate a warning during static analysis because it attempts to call the length()
method on a null reference, potentially leading to a runtime exception. Educators can use such examples to explain the importance of checking for null values.
Challenge #2: Diverse Tooling and Techniques
The Problem
With a plethora of static analysis tools available, each with their own intricacies, the learning curve can become steep. Students may feel overwhelmed by the differences between tools like FindBugs, SonarQube, and Checkstyle.
Solutions
-
Tool Selection:
- Choose one or two popular tools and delve deeper into their usage rather than trying to cover everything. For instance, SonarQube can serve as a comprehensive gateway to understanding static analysis.
-
Tool Comparisons:
- Provide comparisons between tools, discussing their strengths and weaknesses. This contextualizes why certain tools are preferred for specific tasks or environments.
Example Snippet Using Checkstyle
Below is a brief introduction to Checkstyle, a static analysis tool that checks Java code for adherence to coding standards:
<module name="Checker">
<module name="TreeWalker">
<module name="MagicNumber"/>
<module name="FinalLocalVariable"/>
</module>
</module>
This Checkstyle
configuration checks for the use of magic numbers and ensures all local variables are declared as final
whenever applicable. By emphasizing coding standards, educators can stress the importance of maintainability and readability in code.
Challenge #3: Balancing Theory and Practice
The Problem
Static analysis education often heavily leans towards theoretical knowledge—a focus on theory without practice leaves students unprepared for real-world applications.
Solutions
-
Hands-On Workshops:
- Organize workshops where students can apply static analysis tools to their projects. This practical approach reinforces theoretical concepts.
-
Collaborative Coding:
- Encourage collaboration through peer review sessions where students can use static analysis tools on each other's code, fostering a culture of quality improvement.
Example of a Hands-On Activity
A useful hands-on activity would involve students writing a small Java application, intentionally including various coding mistakes. After that, they can use tools like PMD or SonarQube to detect these issues. This exercise reinforces learning objectives:
public class Sample {
public static void main(String[] args) {
// Intentional error: Unused variable
int unusedVariable = 5;
System.out.println("Hello, World!");
}
}
When analyzed, this code should raise warnings about the unused variable, providing immediate feedback about common pitfalls in coding practices.
Challenge #4: Keeping Up with Evolving Practices
The Problem
The software development landscape is ever-changing. Static analysis practices and tools continuously evolve, making it challenging for educators to provide up-to-date knowledge.
Solutions
-
Regular Updates:
- Incorporate recent findings or tools into the curriculum. Regular updates ensure students are learning about current methodologies and best practices.
-
Industry Collaboration:
- Partner with industry professionals or organizations to provide guest lectures or workshops. This provides students with insights into real-world applications of static analysis.
To Wrap Things Up
Static analysis is an essential skill for developers, promoting code quality and reducing risks. Educators face challenges such as complex concepts, diverse tooling, the theory-practice gap, and keeping up with evolving standards. Nonetheless, through careful planning and an emphasis on practical learning, these challenges can be addressed effectively.
By overcoming these hurdles, we equip the next generation of developers with the ability to leverage static analysis tools effectively, enhancing both their skill sets and the quality of software produced.
For those interested in learning more about static analysis, check out SonarQube, FindBugs, and Checkstyle.
Stay curious, keep coding, and remember that mastery comes with practice and perseverance. Happy analyzing!