Top Debugging Mistakes Java Developers Make in Production
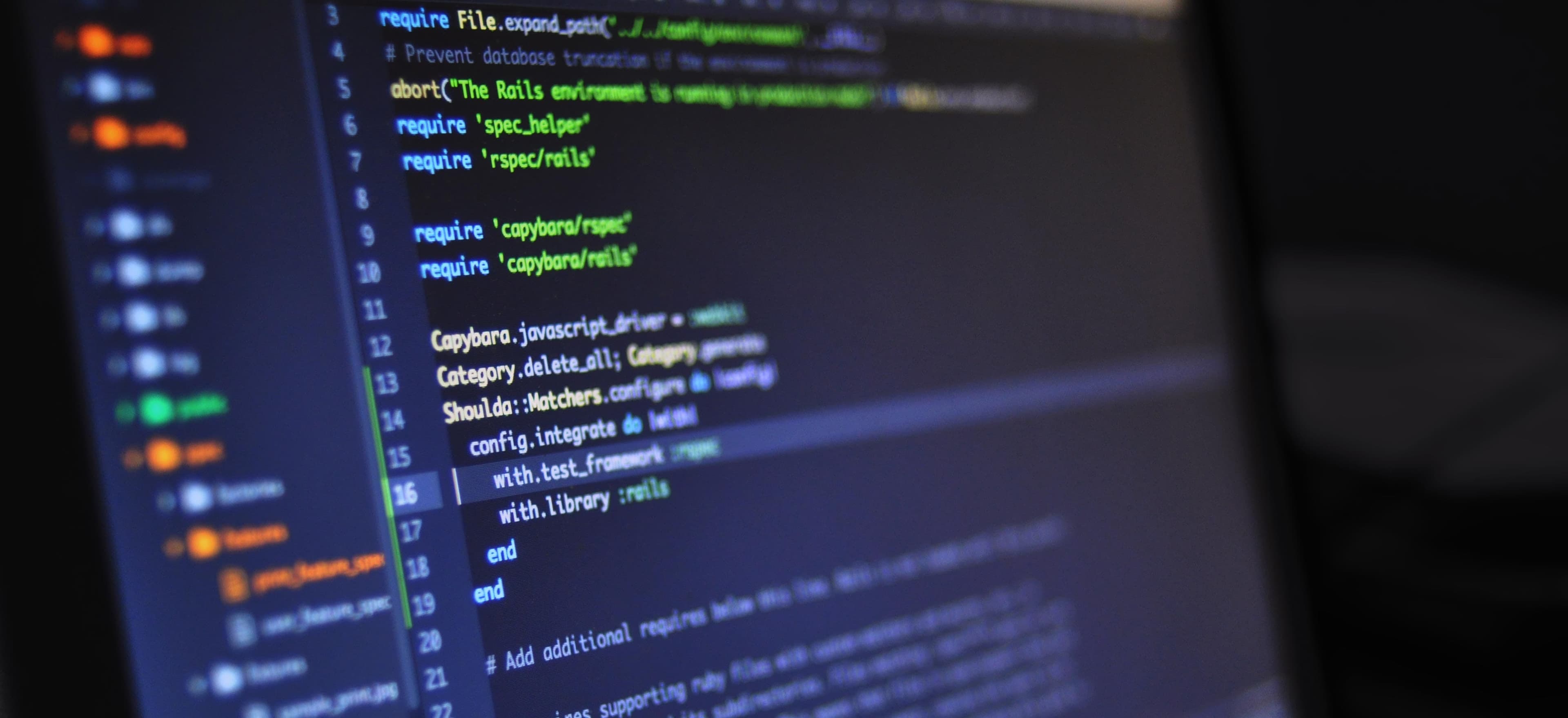
- Published on
Top Debugging Mistakes Java Developers Make in Production
Debugging is an integral part of the software development lifecycle, and Java developers are no exception to the importance of efficient debugging practices. When your application goes live, the stakes are higher, and mistakes can lead to downtime, customer dissatisfaction, or even data loss. In this blog post, we will dive into the top debugging mistakes Java developers often make in production environments and how to avoid them.
1. Ignoring Logging Best Practices
Why Logging Matters
Logging is crucial for understanding what happens in your application, especially in production. However, many developers overlook proper logging practices, which can lead to inefficiencies in debugging when issues arise.
Common Mistakes
- Not Using Appropriate Log Levels: Using only debug or error log levels can obscure essential information.
- Logging Too Much Information: Excessive logging can lead to performance issues and cluttered logs, making it hard to trace problems.
Recommendations
Use a structured logging framework to manage log levels and message formats. For example, consider using SLF4J in conjunction with a logging implementation like Logback or Log4j.
Example Code Snippet
Here's how you can set up structured logging:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class UserService {
private static final Logger logger = LoggerFactory.getLogger(UserService.class);
public void fetchUserById(String userId) {
logger.debug("Fetching user with ID: {}", userId);
// Simulating user fetching...
logger.info("User with ID {} fetched successfully", userId);
}
}
In this snippet, using different log levels allows for both granular debugging during development and quick insights during production.
2. Bypassing Error Handling
Understanding Error Handling
Java provides several mechanisms for error handling. However, developers often neglect these or implement overly simplistic error management strategies in production.
Common Mistakes
- Ignoring Exception Types: Catching general exceptions (
Exception
orThrowable
) can obscure actual issues. - Failing to Handle Errors Gracefully: Crashing the application instead of handling exceptions can lead to a poor user experience.
Recommendations
Be specific in catching exceptions and ensure that you provide feedback or a rollback mechanism whenever an error occurs.
Example Code Snippet
Here's an example of robust error handling:
try {
// Code that might throw an exception
readFile("nonexistentfile.txt");
} catch (FileNotFoundException ex) {
logger.error("File not found: {}", ex.getMessage());
// Handle the error (e.g., notify the user)
} catch (IOException ex) {
logger.error("An IO error occurred: {}", ex.getMessage());
// Retry logic could go here
}
In this case, using specific exception handling allows you to react intelligently to different error conditions.
3. Neglecting Proper Testing
The Importance of Testing
Production code should ideally be backed by extensive testing. However, many developers skip this step or rely solely on unit tests without considering integration or system tests.
Common Mistakes
- Skipping Integration Tests: Relying only on unit tests can lead to undetected issues in the interaction between components.
- Insufficient Test Coverage: Not covering edge cases or failure scenarios can pave the way for overwhelming surprises in production.
Recommendations
Incorporate a suite of tests that include unit, integration, and end-to-end tests to ensure robust coverage and to catch unexpected behaviors.
Example Code Snippet
Here's how to implement an integration test using JUnit and Mockito:
import org.junit.jupiter.api.Test;
import static org.mockito.Mockito.*;
public class UserServiceTest {
UserRepository userRepository = mock(UserRepository.class);
UserService userService = new UserService(userRepository);
@Test
void testFetchUserById() {
when(userRepository.findById("1")).thenReturn(new User("1", "John Doe"));
User user = userService.fetchUserById("1");
assertEquals("John Doe", user.getName());
}
}
This snippet demonstrates how mocking allows you to test the interactions between components without relying on actual database access.
4. Failing to Monitor Application Health
The Role of Monitoring
Monitoring isn’t just for production; it is a proactive measure that can save you from post-deployment disasters.
Common Mistakes
- Ignoring Performance Metrics: Not tracking performance can lead to bottlenecks going unnoticed.
- Lack of Alerting Mechanisms: Without alerts, issues can escalate before developers are aware.
Recommendations
Implement application performance monitoring (APM) tools such as New Relic or AppDynamics to track metrics and set up alerts for anomalies.
5. Poor Code Quality
Understanding Code Readability
Code quality affects not only performance but also maintainability and debuggability.
Common Mistakes
- Duplicating Code: Not following DRY (Don't Repeat Yourself) principles can lead to inconsistencies.
- Inconsistent Naming Conventions: Using non-descriptive variable names can make it harder to trace bugs.
Recommendations
Adopt solid coding standards and practices, and use code review processes to boost quality.
Example Code Snippet
Here's an example of a well-structured service class:
public class OrderService {
private final OrderRepository orderRepository;
public OrderService(OrderRepository orderRepository) {
this.orderRepository = orderRepository;
}
public void processOrder(String orderId) {
// Process logic here
if (orderId == null || orderId.isEmpty()) {
throw new IllegalArgumentException("Order ID cannot be null or empty");
}
// Further processing...
}
}
In this example, clear variable naming and method documentation can make it easier for other developers to follow the logic and assist in debugging.
Lessons Learned
Debugging mistakes can be costly in production. Excessive reliance on trial-and-error methods or poor logging practices can intensify problems. By focusing on logging, error handling, testing, monitoring, and code quality, Java developers can improve their effectiveness in debugging and enhance application reliability.
Adopting best practices is a continuous effort. Stay informed and willing to adapt your strategies and tools. For further reading, check out resources from the Oracle Java Documentation and explore community-driven platforms like Stack Overflow for insights and discussions on Java debugging practices.
When you equip yourself with the right knowledge and tools, you'll be prepared to tackle whatever challenges arise in your production environments. Happy coding!
Checkout our other articles