Common Token Authentication Pitfalls in Java Apps
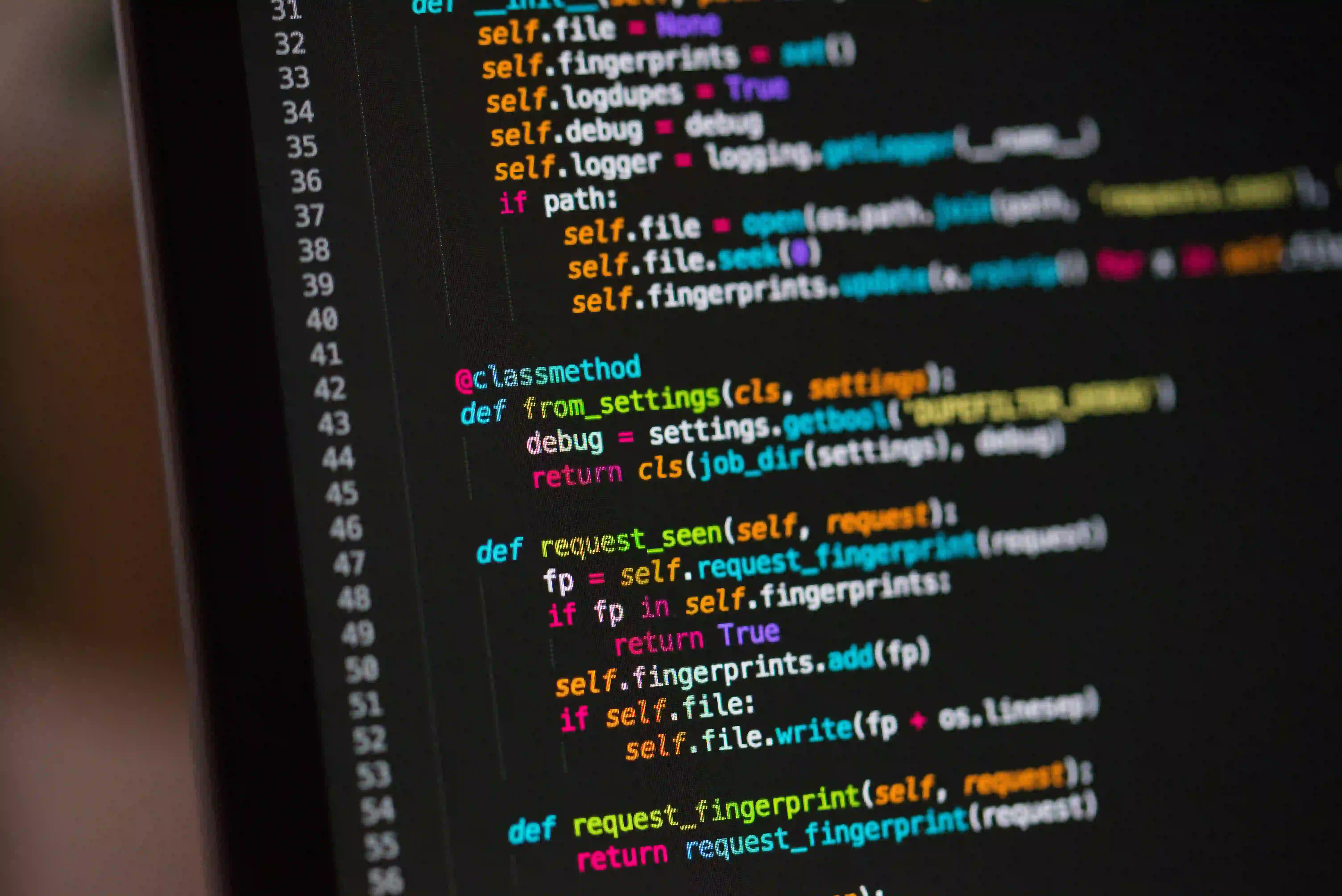
Common Token Authentication Pitfalls in Java Apps
In today's digital landscape, token authentication is a fundamental part of securing applications. Java, being one of the most popular programming languages, has a multitude of libraries and frameworks to handle authentication tokens effectively. However, even experienced developers can encounter pitfalls that can lead to security vulnerabilities or performance issues. This blog post will explore some common pitfalls, provide code snippets for better understanding, and offer strategies to avoid these issues.
Understanding Token Authentication
Token authentication is a process where a user authenticates with a server and receives a token (usually a JSON Web Token or JWT) that grants access to protected resources. This token is then sent with each subsequent request, allowing the server to verify the user's identity without needing to re-authenticate them.
Why Token Authentication?
- Statelessness: Tokens reduce server overhead since you do not need to maintain session state on the server.
- Scalability: It allows easier scaling as any node can process requests without shared session information.
- Cross-domain Support: Tokens facilitate communication across different domains.
Common Pitfalls
1. Not Validating Tokens
One of the primary pitfalls is failing to validate tokens properly. Every time a token is presented, the application must verify its authenticity, expiry, and integrity.
Example Code
Here's a basic implementation that validates a JWT token:
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
public class TokenUtil {
private static final String SECRET_KEY = "your_secret_key";
public static Claims validateToken(String token) {
try {
return Jwts.parser()
.setSigningKey(SECRET_KEY)
.parseClaimsJws(token)
.getBody();
} catch (Exception e) {
// Handle token validation error
return null;
}
}
}
Why Validation is Important
Tokens can expire or may be tampered with by malicious users. A solid validation strategy ensures that only legitimate users can access protected resources. This can significantly reduce the attack surface of your application.
2. Hardcoding Secrets
Storing secret keys or any sensitive information directly in the source code can lead to significant vulnerabilities. When the codebase is shared or accidentally exposed, attackers can easily compromise the application.
Best Practice
Instead of hardcoding secrets, store them in environment variables or a secure vault.
public class TokenUtil {
private static final String SECRET_KEY = System.getenv("SECRET_KEY");
public static String generateToken(String username) {
return Jwts.builder()
.setSubject(username)
.signWith(SignatureAlgorithm.HS256, SECRET_KEY)
.compact();
}
}
Why This Matters
Using environment variables or secure storage solutions allows for flexibility and security. You can easily change the keys without modifying your application code.
3. Long-Lived Tokens
While it might seem convenient, issuing long-lived tokens can increase the risk of compromise. If a token is intercepted, an attacker can access protected resources for an extended period.
Solution: Short-Lived Tokens and Refresh Tokens
You should implement short-lived access tokens and use refresh tokens when necessary. Here’s a simple way to issue a short-lived token:
public class TokenUtil {
private static final long ACCESS_TOKEN_VALIDITY = 1800000; // 30 minutes
public static String generateAccessToken(String username) {
return Jwts.builder()
.setSubject(username)
.setExpiration(new Date(System.currentTimeMillis() + ACCESS_TOKEN_VALIDITY))
.signWith(SignatureAlgorithm.HS256, SECRET_KEY)
.compact();
}
}
Why Short-Lived Tokens?
Short-lived tokens help mitigate risks by reducing the time window for exploitation. Refresh tokens can be implemented to allow new access tokens without requiring the user to log in each time.
4. Lack of Logout Mechanism
In many applications, once authenticated, users might not have a clear way to log out. This lack of a logout mechanism can leave the application vulnerable, especially on shared devices.
Implementing a Logout Mechanism
You can implement a simple logout feature using token blacklisting:
import java.util.HashSet;
import java.util.Set;
public class TokenBlacklist {
private static final Set<String> BLACKLIST = new HashSet<>();
public static void logout(String token) {
BLACKLIST.add(token);
}
public static boolean isBlacklisted(String token) {
return BLACKLIST.contains(token);
}
}
Why Logout is Critical
By allowing users to log out, you effectively allow them to invalidate their tokens. This is particularly important in scenarios where users share devices or access sensitive information.
5. Not Using HTTPS
Another common pitfall is neglecting to use HTTPS. Without HTTPS, tokens can easily be intercepted over unencrypted connections.
Recommended Practice
Always enforce HTTPS in production. You can do this by configuring your web server and making sure the application is accessible only over secure connections.
Why HTTPS Matters
HTTP exposes all data transmitted, making it easy for attackers to capture tokens and impersonate users. HTTPS greatly reduces this risk by encrypting data in transit.
The Closing Argument
Token-based authentication is an elegant and flexible method for securing Java web applications. However, to leverage its advantages fully, it's crucial to be aware of common pitfalls.
- Always validate tokens effectively.
- Never hardcode secrets; use secure storage alternatives.
- Consider using short-lived tokens with refresh mechanisms.
- Implement a robust logout feature.
- Always enforce HTTPS to secure data in transit.
By following these best practices, you can significantly enhance the security and performance of your token authentication implementation. For further reading on best practices for securing Java applications, you may refer to OWASP Java Security and Spring Security Documentation.
By avoiding these pitfalls, your Java web applications can remain secure and provide a better user experience without compromising on safety.