Overcoming Common JavaFX 8 Popup Editor Challenges
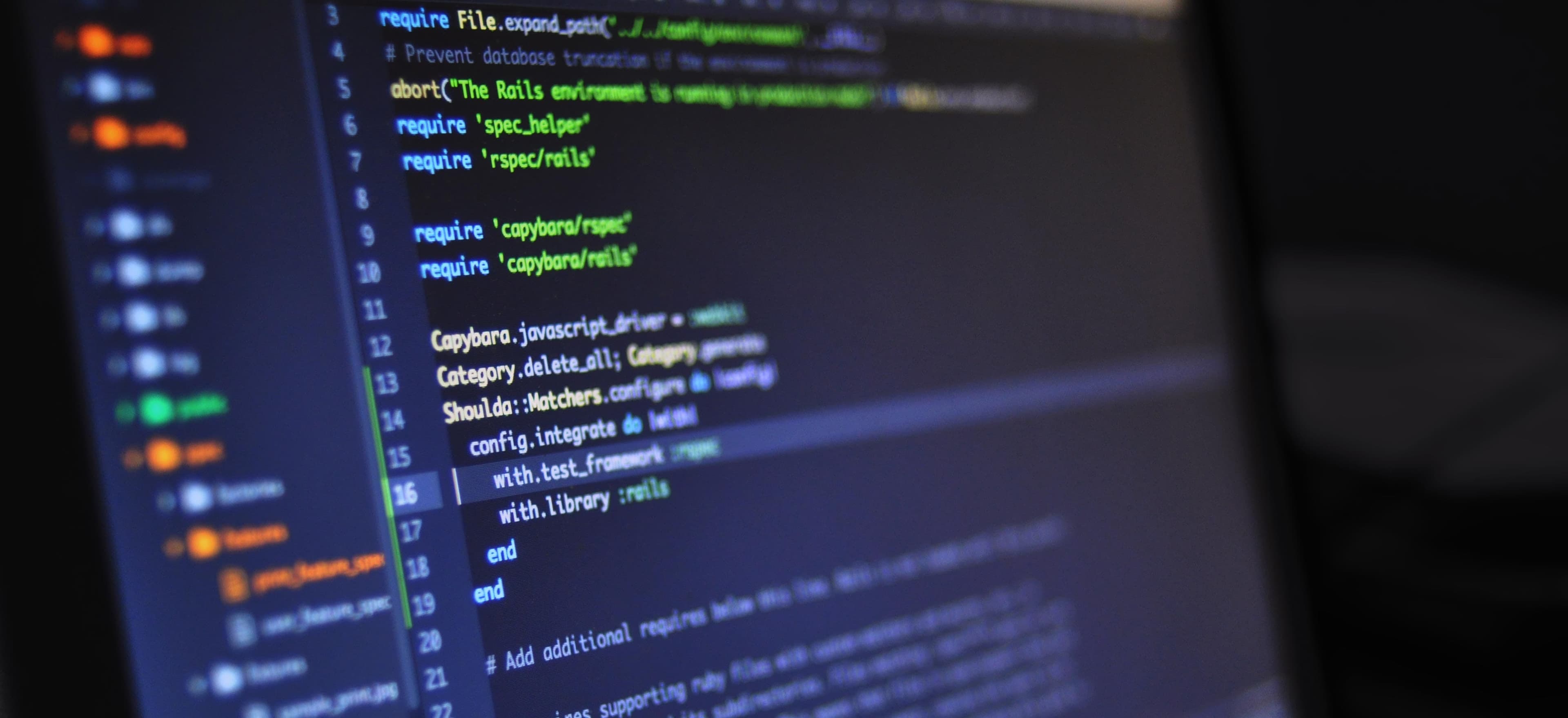
- Published on
Overcoming Common JavaFX 8 Popup Editor Challenges
JavaFX is a powerful framework for building rich desktop applications in Java. One frequent interaction in user interfaces is through popups, especially when editing or configuring data. Despite its potential, developers often face challenges when implementing JavaFX 8 Popups. In this blog post, we will discuss common challenges, provide code snippets that illustrate best practices, and explain how to effectively utilize popups in JavaFX.
Table of Contents
- What is JavaFX?
- Common Challenges with Popups
- Challenge 1: Managing Focus
- Challenge 2: Handling User Input
- Challenge 3: Styling the Popup
- Implementing a Simple Popup Editor
- Conclusion
- References
What is JavaFX?
JavaFX is a software platform for creating desktop applications using Java. It provides a rich set of UI controls and allows Java developers to build visually appealing applications with ease. JavaFX enables developers to utilize various media, graphics, and other interactive elements that enhance user experience.
Common Challenges with Popups
While working with popups in JavaFX, several challenges may impede your development process. Here are three common issues:
Challenge 1: Managing Focus
One primary challenge is controlling the focus when a popup is activated. If focus remains on the parent application window, users may unintentionally interact with it instead of the popup.
Solution: Request Focus
Use the requestFocus()
method on your Popup. Here’s how you can implement it:
// Create a new popup
Popup popup = new Popup();
TextField textField = new TextField();
// Adding the text field to the popup
popup.getContent().add(textField);
// Showing the popup at a specified location
popup.setAutoHide(true);
popup.show(stage, x, y);
// Request focus on the text field
textField.requestFocus();
Why This Works: By calling requestFocus()
, we ensure that users can start typing immediately in the text field without clicking it first.
Challenge 2: Handling User Input
Another common problem developers encounter is properly handling user input. It can be challenging to capture and process data accurately when the user interacts with the popup.
Solution: Use TextField with Event Handlers
Setting up event handlers ensures that you can capture user actions effectively.
textField.setOnKeyPressed(event -> {
if (event.getCode() == KeyCode.ENTER) {
String input = textField.getText();
System.out.println("User entered: " + input);
// Hide the popup after processing input
popup.hide();
}
});
Why This Works: With the setOnKeyPressed
event handler, we enable a quick response to user input. Users can hit the Enter key to submit their data, which enhances usability by decreasing the steps required to interact with the popup.
Challenge 3: Styling the Popup
Styling popups can be tricky. Default popups in JavaFX do not have the same styles as your application, which can lead to a disjointed user experience.
Solution: Apply CSS Styles
JavaFX allows you to apply CSS styles to your popups effectively. Here’s a quick example:
String css = " -fx-background-color: #FFC107; " +
" -fx-border-color: #000000; " +
" -fx-border-width: 2; ";
popup.setStyle(css);
Why This Works: By defining CSS directly in your code, you can customize the look and feel of popups to align the UI elements throughout your application. This adds to the aesthetic appeal and user interactivity.
Implementing a Simple Popup Editor
Now that we have discussed the common challenges and their solutions, let's look at an example of a simple popup editor that integrates all of these concepts.
Full Example Code
Here’s a complete sample demonstrating a simple popup for editing a user’s name.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextField;
import javafx.scene.layout.VBox;
import javafx.stage.Popup;
import javafx.stage.Stage;
public class PopupEditorExample extends Application {
@Override
public void start(Stage primaryStage) {
Button showPopupButton = new Button("Edit Name");
TextField nameDisplay = new TextField("User Name");
VBox vBox = new VBox(nameDisplay, showPopupButton);
Popup popup = new Popup();
TextField textField = new TextField();
textField.setPromptText("Enter new name");
Button submitButton = new Button("Submit");
submitButton.setOnAction(e -> {
String newName = textField.getText();
nameDisplay.setText(newName);
popup.hide();
});
popup.getContent().addAll(textField, submitButton);
showPopupButton.setOnAction(e -> {
popup.setAutoHide(true);
popup.show(primaryStage, 200, 200);
textField.requestFocus();
});
Scene scene = new Scene(vBox, 300, 200);
primaryStage.setScene(scene);
primaryStage.setTitle("Popup Editor Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
How It Works
- Creating the Popup: The button labeled Edit Name opens a popup that allows the user to enter a new name.
- Text Input: The user can type in the new name, and upon hitting Submit, the name displayed in the primary window is updated.
- Focus Management: The requestFocus call ensures that the text field is ready for user input as soon as the popup appears.
Closing Remarks
Popups are powerful tools in JavaFX that enhance user experience by providing seamless interaction interfaces. By addressing challenges like focus management, user input handling, and styling, developers can create effective popup editors.
For further reading on JavaFX popups, consider checking out the JavaFX Documentation and exploring resources provided by Oracle.
Incorporating these practices can help you build a more user-friendly application, minimizing frustration while maximizing efficiency.
References
- JavaFX Documentation
- JavaFX Tutorial by Oracle
- Creating Popups in JavaFX
Happy coding!