Common Mistakes When Coding Multiplication Tables in Java
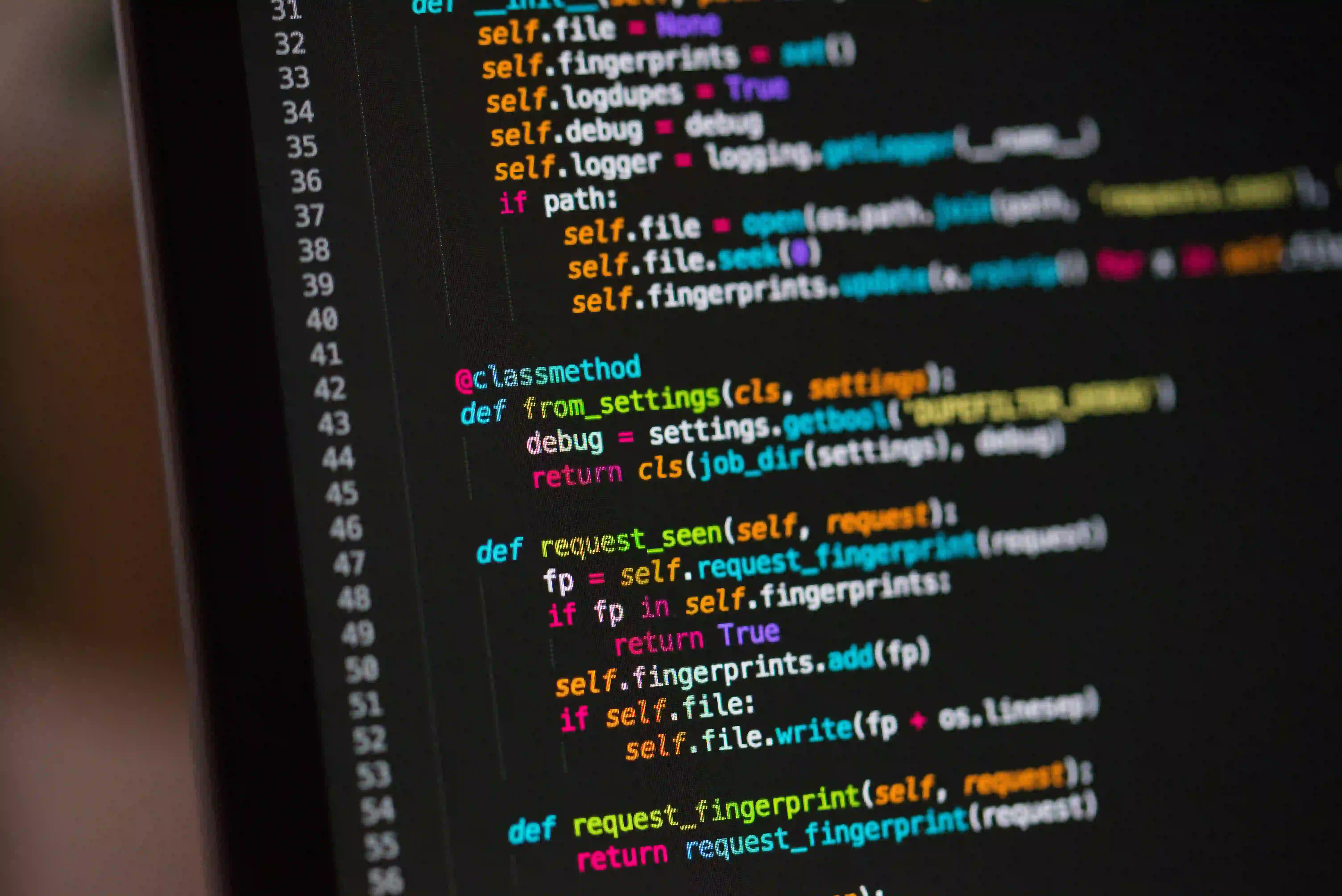
Common Mistakes When Coding Multiplication Tables in Java
Creating a multiplication table is a fundamental exercise that many beginners encounter while learning Java. It helps solidify their understanding of loops, arrays, and basic programming logic. However, like any programming task, common mistakes can occur. In this blog post, we will explore these pitfalls, provide exemplary code snippets, and clarify the reasoning behind each section of the code.
1. Incorrect Loop Structure
One of the first mistakes novice Java developers often make is using incorrect loop structures. Multiplication tables typically involve two nested loops: one for the rows (the multiplier) and another for the columns (the multiplicand).
Let's look at an example of a poorly structured multiplication table:
public class IncorrectMultiplicationTable {
public static void main(String[] args) {
// Incorrect use of loops
for (int i = 1; i <= 10; i++) {
for (int j = 1; j <= 5; j++) { // Missing the correct number of columns
System.out.print(i * j + " ");
}
System.out.println();
}
}
}
Why is this incorrect?
In the above example, we've set the outer loop to iterate from 1 to 10, and the inner loop only goes to 5. This means we are generating a 10x5 multiplication table instead of a full 10x10 table. It's crucial to match the dimensions you intend to output.
Corrected Version
public class CorrectMultiplicationTable {
public static void main(String[] args) {
// Correct loop structure to generate a 10x10 multiplication table
for (int i = 1; i <= 10; i++) {
for (int j = 1; j <= 10; j++) {
System.out.print(i * j + "\t");
}
System.out.println();
}
}
}
In this corrected version, both loops iterate from 1 to 10, producing the desired 10x10 multiplication table. The use of "\t"
(tab character) provides cleaner formatting in the output.
2. Not Using Proper Formatting
Outputting tables can sometimes look cluttered if not properly formatted. Using spaces or tabs can create alignment issues that make it hard to read.
Instead of printing the output directly, consider formatting each number with a defined width.
Example without Formatting
for (int i = 1; i <= 10; i++) {
for (int j = 1; j <= 10; j++) {
System.out.print(i * j + " "); // Poorly aligned output
}
System.out.println();
}
Example with Formatting
public class FormattedMultiplicationTable {
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
for (int j = 1; j <= 10; j++) {
System.out.printf("%4d", i * j); // Using printf for better formatting
}
System.out.println();
}
}
}
Why Use printf
?
Using System.out.printf("%4d", i * j);
allows you to define a width (4 spaces here) for each output number, ensuring that regardless of whether the product is one digit or two, the table aligns perfectly.
3. Missing Edge Cases
When implementing multiplication tables, it’s crucial to consider potential edge cases. What if you want to create a multiplication table of size zero or negative values? Not incorporating logic to handle these scenarios can lead to unexpected behaviors.
Example of Missing Edge Cases
public class EdgeCaseMultiplicationTable {
public static void main(String[] args) {
int size = 10; // Try changing the size to 0 or negative
for (int i = 1; i <= size; i++) {
for (int j = 1; j <= size; j++) {
System.out print(i * j + "\t");
}
System.out.println();
}
}
}
Improved Logic
public class SafeMultiplicationTable {
public static void main(String[] args) {
int size = 10; // Can be set to 0 or negative for testing
if (size <= 0) {
System.out.println("Size must be a positive integer.");
return;
}
for (int i = 1; i <= size; i++) {
for (int j = 1; j <= size; j++) {
System.out.printf("%4d", i * j);
}
System.out.println();
}
}
}
Why This Matters
Adding simple validation to ensure the 'size' variable is positive prevents unnecessary computations and allows for early feedback to the user.
4. Hardcoding Values
Hardcoding values might seem convenient, but it can lead to rigidity in your code. For instance, rather than using fixed numbers throughout your code, consider utilizing constants or variables for better adaptability.
Example of Hardcoded Values
public class HardcodedMultiplicationTable {
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
for (int j = 1; j <= 10; j++) {
System.out.print(i * j + "\t");
}
System.out.println();
}
}
}
Improved Version with Constants
public class AdjustableMultiplicationTable {
private static final int SIZE = 10; // Constant for table size
public static void main(String[] args) {
for (int i = 1; i <= SIZE; i++) {
for (int j = 1; j <= SIZE; j++) {
System.out.printf("%4d", i * j);
}
System.out.println();
}
}
}
Why Use Constants?
Constants enhance code maintainability. If you ever decide to change the size of the table, you can update one line, and the change will reflect throughout the program.
5. Ignoring Optimization Opportunities
While coding a basic multiplication table may not require heavily optimized code, it is essential to grasp the concept of efficiency.
For example, calculating the product i * j
can be abstracted or stored in a variable if you plan on using it multiple times in the same loop iteration.
Example Ignoring Optimization
public class NonOptimizedMultiplicationTable {
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
for (int j = 1; j <= 10; j++) {
// Unnecessarily recalculating the product
System.out.print(i * j + "\t");
// Some other operation involving i * j
}
System.out.println();
}
}
}
Optimized Version
public class OptimizedMultiplicationTable {
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
for (int j = 1; j <= 10; j++) {
int product = i * j; // Storing the product
System.out.printf("%4d", product);
// Use product for any other operation here
}
System.out.println();
}
}
}
Why is Optimization Important?
This improves readability and performance, especially in larger-scale applications where computational efficiency can significantly impact performance.
Final Thoughts
In conclusion, building a multiplication table might be a simple task, yet it serves as an excellent way to practice Java fundamentals. By avoiding common mistakes such as improper loops, poor formatting, and neglecting edge cases, you can enhance the reliability and readability of your code.
Utilizing best practices, such as using constants, proper validation, and optimization techniques, lays a strong foundation for future programming challenges.
For more insights on Java programming, check out Oracle's official documentation and broaden your knowledge base of Java fundamentals.
Embrace the journey of coding—each mistake is an opportunity to learn and grow as a developer!