Speed Up Your Software Project: Key Steps to Start Fast
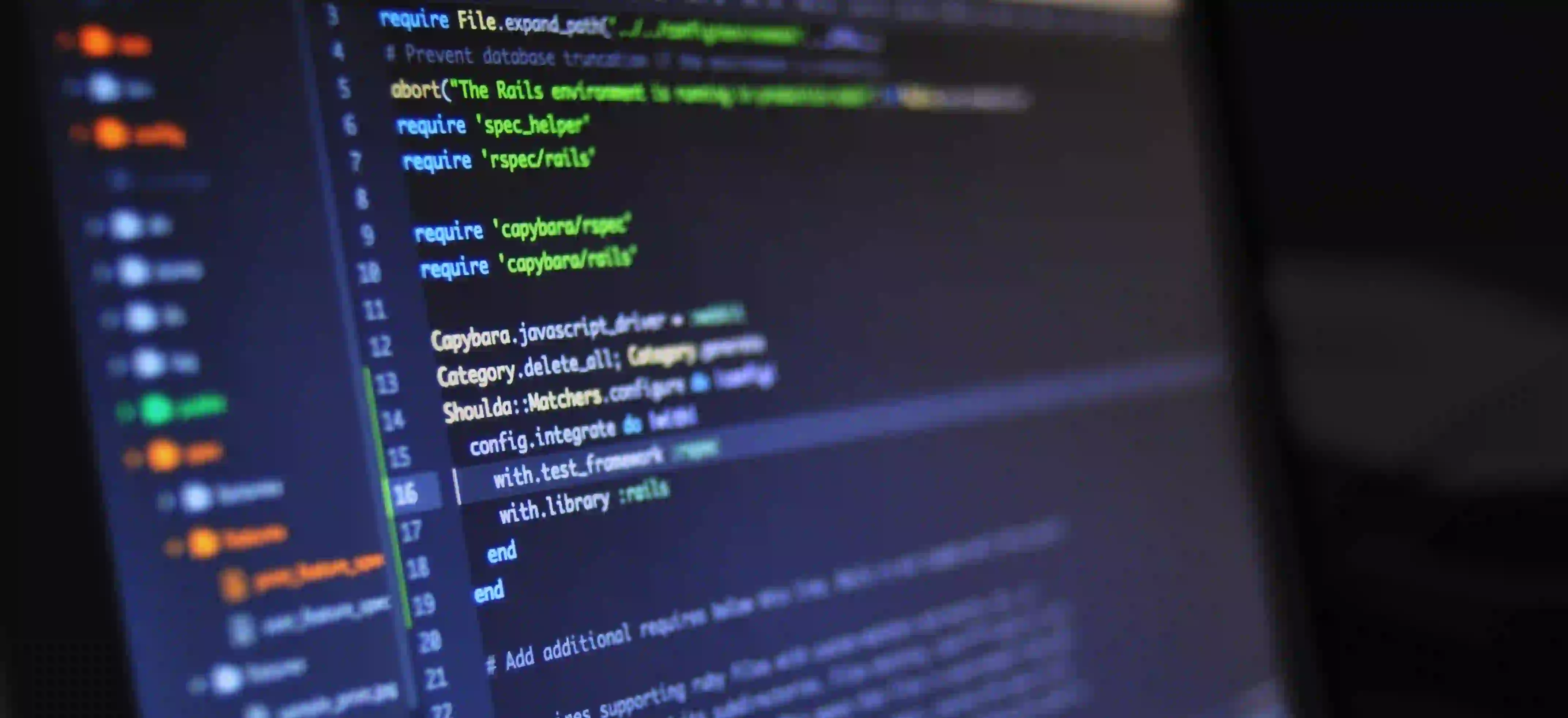
Speed Up Your Software Project: Key Steps to Start Fast
In today's fast-paced tech environment, timely delivery of software products is crucial to staying ahead of the competition. Many software projects fail not due to a lack of skills or resources, but because they lack proper planning and execution strategies. Let’s explore some key steps you can take to ramp up your software projects quickly and efficiently.
1. Define Clear Objectives
Before diving into coding, it's essential to have a clear understanding of the project's goals. What problem does your software aim to solve? Who is your target audience? These questions establish a foundation for all future decisions.
Example:
If you are developing a task management app, your core objectives might include:
- Simplifying task creation for users.
- Integrating calendar functionalities.
- Providing a mobile-friendly interface for on-the-go usability.
The clearer your objectives, the easier it becomes to prioritize features and allocate resources.
2. Create a Minimum Viable Product (MVP)
An MVP is a product with just enough features to be usable by early adopters and provide feedback for future development. This helps you validate your concept before extensive investing time and resources.
Code Example:
The following Java code snippet demonstrates the concept of a simple task entity that could be part of your MVP:
public class Task {
private String title;
private boolean completed;
public Task(String title) {
this.title = title;
this.completed = false;
}
public String getTitle() {
return title;
}
public boolean isCompleted() {
return completed;
}
public void complete() {
this.completed = true;
}
}
Why this code?
The Task
class is a simple data structure that captures the essence of a task in the app. It has essential properties, which keeps the code minimal, allowing rapid development and iterative updates based on user feedback.
3. Adopt Agile Methodologies
Agile is a project management framework that encourages continuous iteration of development and testing throughout the project lifecycle. Using Agile allows teams to be flexible and make adjustments based on user feedback and changing requirements.
Benefits of Agile:
- Adaptive Planning: Steer your project based on current needs.
- Increased Product Quality: Short cycles mean regular testing.
- Customer Collaboration: Enhanced communication with stakeholders leads to a product more aligned with user expectations.
For more on Agile methodologies, visit Agile Alliance.
4. Prioritize Features
In a software project, not all features carry the same weight. Use frameworks like MoSCoW (Must have, Should have, Could have, Won't have) to differentiate essential features from the nice-to-have ones.
Example of Prioritization:
Suppose your task management app includes features like:
- User authentication (Must have)
- Real-time notifications (Should have)
- Custom themes (Could have)
By prioritizing, your team can focus on implementing crucial features first, speeding up the time to market.
5. Use Collaboration Tools
Today’s software development process heavily relies on collaboration tools. Tools like JIRA, Trello, or Asana help keep track of progress, manage tasks, and enable communication among team members.
Advantages of Collaboration Tools:
- Transparency: Team members can see the project status at a glance.
- Simplified Communication: Chat functions promote quick discussions.
- Task Management: Proper organization helps avoid project delays.
6. Implement Continuous Integration/Continuous Deployment (CI/CD)
CI/CD practices enable developers to frequently merge code changes into a central repository, followed by automated builds and tests. This ensures that your code is always in a deployable state and can considerably speed up your release cycles.
CI/CD Example in Java:
Here’s a simple example using Maven for a CI/CD pipeline:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example.taskapp</groupId>
<artifactId>task-app</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.2</version>
</plugin>
</plugins>
</build>
</project>
Why this code?
The Maven build configuration enables automated testing and packaging of your application. That results in faster builds and optimized deployment processes.
7. Conduct Regular Retrospectives
At the end of every sprint or development phase, conduct retrospective meetings. These discussions provide insight into what went well, what didn’t, and how you can improve.
Benefits of Retrospectives:
- Team Reflection: Encourages open dialogue about issues.
- Process Improvement: Identify bottlenecks and enhance workflow.
- Employee Engagement: Involves team members in the decision-making process.
8. Stay Updated with Technology
Lastly, technology evolves rapidly. Continuous learning is crucial for any software team. Whether it’s exploring new frameworks, libraries, or best practices, investing in skills development ensures your team remains competitive.
Resources to Consider:
- Documentation: Always refer to official Java documentation and tutorials.
- Online Courses: Websites like Coursera or Udemy offer courses tailored for developers.
- Meetups: Join local or virtual meetups to learn and network with peers.
The Last Word
Speeding up your software project requires a strategic approach that encompasses thorough planning, adopting the right tools, and staying flexible. By defining clear objectives, iterating with an MVP, leveraging Agile methodologies, and committing to continuous integration, you can streamline your development process.
Remember, efficiency does not mean cutting corners. Strive for quality alongside speed. Implement these strategies and watch your project transition from a concept to a full-fledged software application efficiently!
For more information on software development methodologies, check out Scrum.org. This site offers insightful resources for teams wanting to implement Scrum practices.
Happy coding!