Navigating the Changes in Effective Java's Third Edition
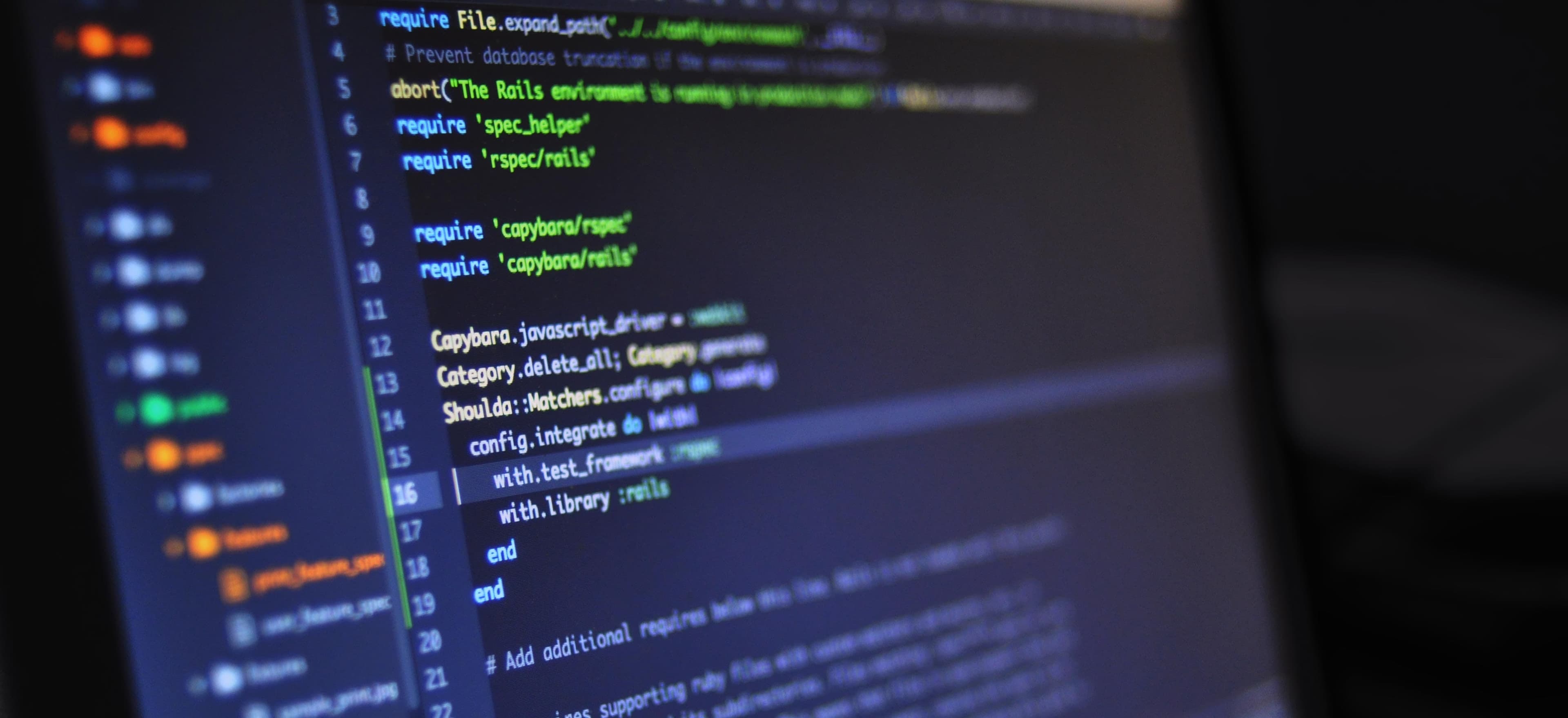
- Published on
Navigating the Changes in Effective Java's Third Edition
Effective Java, written by Joshua Bloch, has been a staple for Java developers since its first edition was released in 2001. The third edition, released in 2018, introduced a plethora of updates to reflect modern Java best practices. This blog post will delve into the significant changes in the third edition and provide insights into how these principles can enhance your Java programming skills.
Why Read Effective Java?
Before we dive into the changes, let’s discuss why Effective Java is a must-read for Java developers. This book contains 90 distinct items grouped into best practices and design principles that are crucial for writing robust, maintainable, and efficient Java code. Bloch's experience at Sun Microsystems and Google lends credibility to his recommendations, making this guide invaluable.
Structure of Effective Java
The book is sorted into several chapters:
- Creating and Destroying Objects
- Methods Common to All Objects
- Classes and Interfaces
- Generics
- Enums and Annotations
- Lambdas and Streams
- Methods
- Concurrency
- Serialization
With the third edition, Bloch updated many existing items and added new ones, ensuring the content is fresh and relevant to the evolving Java landscape.
Key Changes in the Third Edition
Let’s explore some of the most impactful changes in the third edition with focused discussions, code snippets, and practical examples.
1. Improved Items in Object Creation
One of the critical areas addressed is object creation and destruction. The third edition emphasizes using factory methods instead of constructors for more controlled object creation.
Code Example:
public class NutritionFacts {
private final int servings;
private final int calories;
private final int fat;
private final int sodium;
private final int carbohydrate;
public static class Builder {
// Required parameters
private final int servings;
// Optional parameters - initialized to default values
private int calories = 0;
private int fat = 0;
private int sodium = 0;
private int carbohydrate = 0;
public Builder(int servings) {
this.servings = servings;
}
public Builder calories(int val) {
calories = val;
return this;
}
public Builder fat(int val) {
fat = val;
return this;
}
public Builder sodium(int val) {
sodium = val;
return this;
}
public Builder carbohydrate(int val) {
carbohydrate = val;
return this;
}
public NutritionFacts build() {
return new NutritionFacts(this);
}
}
private NutritionFacts(Builder builder) {
servings = builder.servings;
calories = builder.calories;
fat = builder.fat;
sodium = builder.sodium;
carbohydrate = builder.carbohydrate;
}
}
Explanation:
- The builder pattern allows for a more readable and maintainable code structure.
- It provides a flexible mechanism for object creation, especially when dealing with classes with many optional parameters. This pattern also eliminates constructor overloading, which can be confusing to users.
2. Enhanced Use of Annotations
In the third edition, Bloch emphasizes the importance of annotations, particularly with the introduction of new annotations like @NonNull
and @ThreadSafe
. This leads to clearer code and improves maintainability.
Code Example:
import javax.annotation.Nonnull;
public class Account {
private double balance;
public Account(double initialBalance) {
balance = initialBalance;
}
@Nonnull
public Double getBalance() {
return balance;
}
public void deposit(double amount) {
if (amount > 0) {
balance += amount;
}
}
}
Explanation:
- Annotations help denote contract significance in your code.
- The
@Nonnull
annotation clearly communicates that thegetBalance
method will never return anull
value, thus reducing potential issues related toNullPointerExceptions
.
3. Embracing Functional Programming
The shift towards functional programming in Java, especially with the addition of lambdas and streams in Java 8, is significant. Effective Java’s third edition integrates these concepts extensively.
Code Example:
import java.util.Arrays;
import java.util.List;
public class FunctionalProgrammingExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Java", "JavaScript", "Python", "C#", "Ruby");
names.stream()
.filter(name -> name.startsWith("J"))
.map(String::toUpperCase)
.forEach(System.out::println);
}
}
Explanation:
- The use of streams makes it easy to process collections of data in a declarative way.
- Such constructs are cleaner and easier to understand, making codebases easier to maintain.
4. Generics for Type Safety
Generics have always been part of Java, but the third edition provides deeper insights into how to utilize them effectively.
Code Example:
import java.util.ArrayList;
import java.util.List;
public class GenericMethodExample {
public static <T> void addToList(List<T> list, T item) {
list.add(item);
}
public static void main(String[] args) {
List<String> stringList = new ArrayList<>();
addToList(stringList, "Hello");
List<Integer> intList = new ArrayList<>();
addToList(intList, 123);
}
}
Explanation:
- Generics allow for type safety across collections. In this example, the
addToList
method can accept any type, which prevents runtime errors and enhances code reusability. - Such practices promote better design and reduce the likelihood of class cast exceptions.
5. Best Practice for Enum Usage
Enums are a powerful feature in Java, and the third edition emphasizes their use for representing specific, immutable types.
Code Example:
public enum Operation {
PLUS, MINUS, TIMES, DIVIDE;
public double apply(double x, double y) {
switch (this) {
case PLUS: return x + y;
case MINUS: return x - y;
case TIMES: return x * y;
case DIVIDE: return x / y;
default: throw new UnsupportedOperationException("Unknown operation: " + this);
}
}
}
Explanation:
- Using enums in this way promotes type safety and clarity in operations.
- Each enum constant can be extended with behaviors, allowing for clear and maintainable code structures.
Closing the Chapter
Effective Java's third edition presents an upgraded approach to Java programming. Incorporating modern practices, principles, and design patterns, it helps developers write cleaner and more efficient code. By comprehensively understanding the changes and new best practices, you'll be able to elevate your Java prowess.
If you're looking to deepen your understanding of Java, pick up a copy of Effective Java and start integrating these practices into your coding toolbox.
Additional Reading
For those looking to expand their knowledge further, consider checking out:
- Java Concurrency in Practice by Brian Goetz
- Clean Code: A Handbook of Agile Software Craftsmanship by Robert C. Martin
Embrace the changes and let Effective Java guide you to becoming a more proficient Java developer. Happy coding!
Checkout our other articles