Choosing Between Test Data Builders and Object Mother
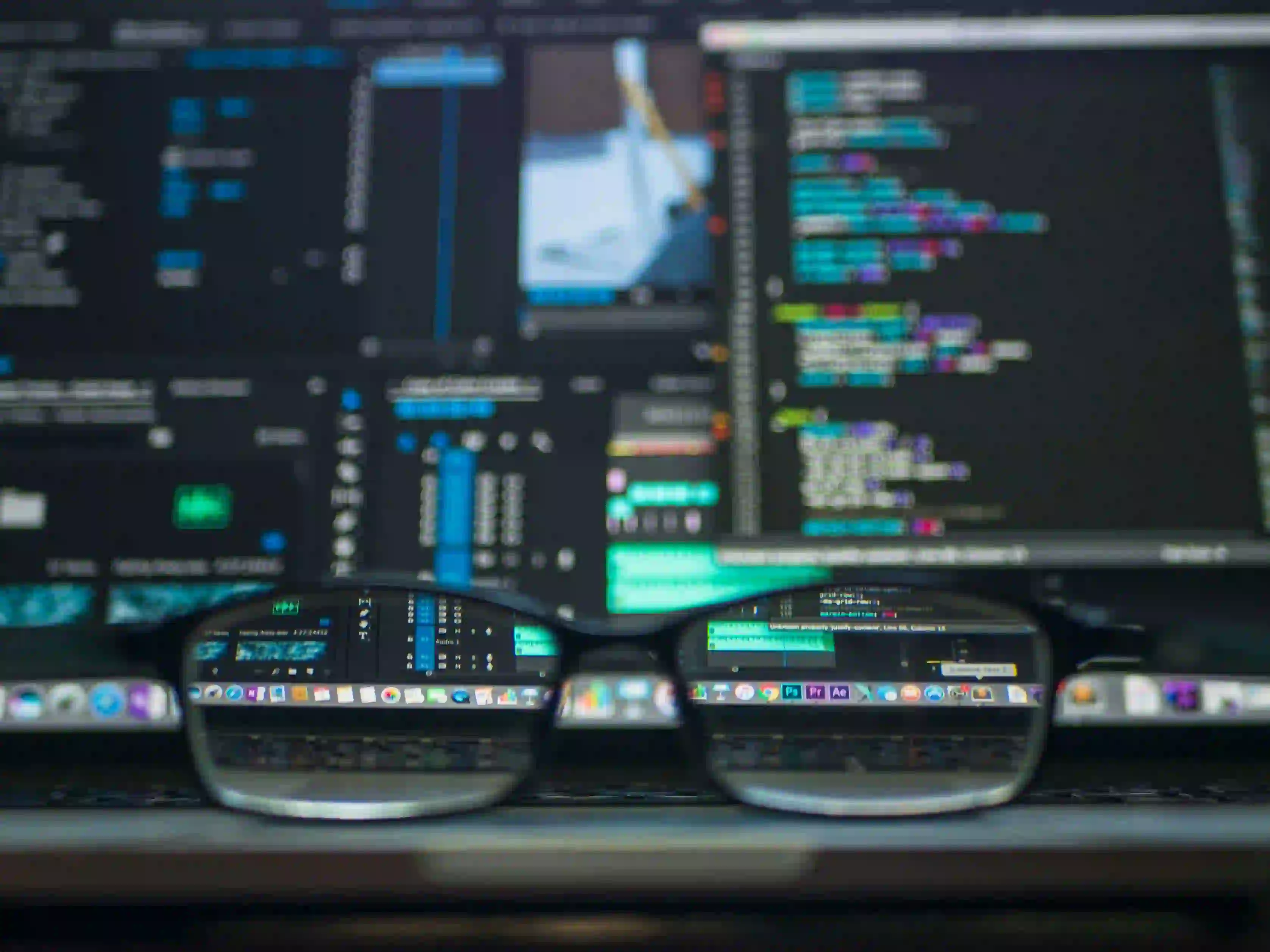
Choosing Between Test Data Builders and Object Mother
When it comes to unit testing in Java, managing the complexity of test data can often become a significant challenge. This is where patterns like Test Data Builder and Object Mother come into play. Both aim to simplify test data creation, but they approach the problem differently and are ideal for different situations. In this post, we'll dive into what each of these patterns entails, their advantages and disadvantages, and when to use each one.
Understanding Object Mother
Object Mother is a design pattern that provides a way to create and reuse test data. It acts like a factory, creating fully initialized objects that can be used in tests. This is especially useful for creating complex objects that require multiple parameters for their construction.
Example of Object Mother
Let's say we have a User
class, which requires both a name
and an age
for instantiation:
public class User {
private String name;
private int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
// Getters and setters omitted for brevity
}
Our Object Mother would look something like this:
public class UserMother {
public static User createDefaultUser() {
return new User("Default User", 30);
}
public static User createUserWithName(String name) {
return new User(name, 30);
}
public static User createUserWithAge(int age) {
return new User("Default User", age);
}
public static User createUser(String name, int age) {
return new User(name, age);
}
}
Advantages of Object Mother
- Reusability: You can create multiple variations of objects without repeating code, thereby promoting DRY (Don't Repeat Yourself).
- Readability: You can provide meaningful names to the methods, making the test code clearer.
- Abstraction: It abstracts the complexity of initializing objects, so tests can focus on logic rather than setup.
Disadvantages of Object Mother
- Fixed variations: If more test cases require different combinations of parameters, you’ll eventually find yourself updating the Object Mother, leading to potential bloat.
- Complexity in Large Classes: If a class has many fields, the Object Mother can become cumbersome.
Delving Into Test Data Builder
On the other hand, Test Data Builders offer a more flexible alternative. The builder pattern allows you to construct objects step-by-step, providing defaults while letting you override as needed. This is beneficial when objects have multiple optional fields.
Example of Test Data Builder
Let's revisit our User
class. Here's an illustrative implementation of a Test Data Builder:
public class UserBuilder {
private String name = "Default User";
private int age = 30;
public UserBuilder withName(String name) {
this.name = name;
return this;
}
public UserBuilder withAge(int age) {
this.age = age;
return this;
}
public User build() {
return new User(name, age);
}
}
Usage of Test Data Builder
Creating a user with the builder would look like this:
User user1 = new UserBuilder().build(); // Default values
User user2 = new UserBuilder().withName("John Doe").withAge(25).build(); // Custom values
Advantages of Test Data Builders
- Flexibility: You can easily create many variations of an instance without modifying existing methods.
- Clear Intent: Method names like
withName
andwithAge
clarify which settings are being configured. - Fluid Interface: Building an object can be chained together, keeping the code compact and readable.
Disadvantages of Test Data Builders
- Boilerplate Code: For very simple objects, a builder can introduce unnecessary complexity.
- Overhead: The creation and use of builders may slightly slow down the initialization process, though this is often negligible.
When to Use Object Mother vs. Test Data Builder
Choosing between these two designs depends largely on the complexity and requirements of your tests.
Use Object Mother when:
- Your objects are relatively simple.
- You want straightforward reusability of common setups across multiple tests.
- You have a limited number of variations that can efficiently be encapsulated in method calls.
Use Test Data Builder when:
- Your objects are more complex with many optional fields.
- You need the flexibility to customize object creation for different test scenarios without cluttering your test code.
- You prefer a more expressive and maintainable way to configure your object properties.
A Final Look
Both Object Mother and Test Data Builder patterns have their places in unit testing. The key is recognizing the nature of the objects you are working with and the requirements of your tests. If your tests often create a wide variety of complex objects, the builder pattern is likely your best bet. Conversely, if you frequently need to create a standard configuration of a simple object, Object Mother will work just fine.
By choosing the right pattern for the job, you can increase the maintainability and readability of your test suite, which ultimately leads to higher quality code.
For deeper understanding, you may also want to explore more about design patterns in Java or find best practices on testing in Java that can complement these patterns effectively.
Happy testing!