Mastering RESTful Error Handling in Spring Applications
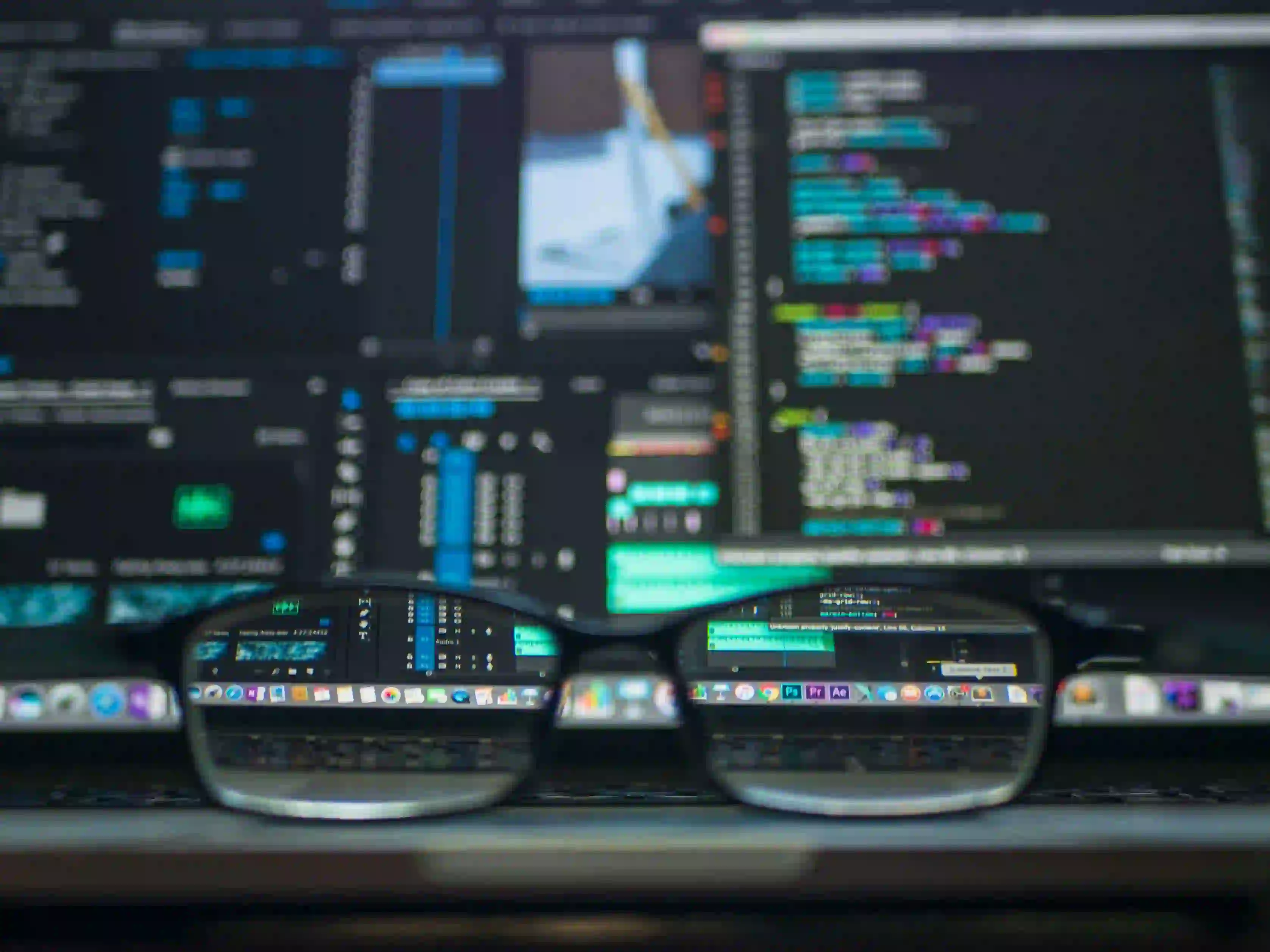
Mastering RESTful Error Handling in Spring Applications
Building robust RESTful APIs in Java with Spring is essential for creating high-quality applications that provide a seamless user experience. One key aspect of any API design is efficient error handling. In this blog post, we'll explore different strategies for error handling in Spring applications, emphasizing best practices and providing you with exemplary code snippets.
Why Error Handling Matters
Error handling is crucial for several reasons:
- User Experience: A well-handled error response informs users of what went wrong.
- Debugging: Clear error messages help developers understand issues quickly.
- API Integrity: Consistent error responses help clients manage errors effectively.
Basic Error Handling in Spring
Spring Boot automatically provides a simple error response for unhandled exceptions, but it is important to customize this behavior for better clarity.
Example of Default Error Handling
When a request fails, the default error handling looks something like this:
{
"timestamp": "2023-10-01T12:00:00",
"status": 404,
"error": "Not Found",
"message": "No message available",
"path": "/api/resource"
}
While useful, this format offers minimal information. Customizing this format can significantly improve the user's experience.
Custom Error Classes
To enhance your API's error handling, start by creating custom error classes. These classes should model the error response and include necessary fields.
Example Custom Error Class
public class ApiError {
private LocalDateTime timestamp;
private int status;
private String error;
private String message;
private String path;
public ApiError(int status, String error, String message, String path) {
this.timestamp = LocalDateTime.now();
this.status = status;
this.error = error;
this.message = message;
this.path = path;
}
// Getters and Setters
}
Why Use a Custom Error Class?
- Flexibility: Customize fields based on specific requirements.
- Uniformity: Provide a consistent error structure across the API.
Global Exception Handling with @ControllerAdvice
Using @ControllerAdvice
allows you to handle exceptions globally across all controllers. This way, you can centralize error handling logic, promoting clean code and maintainability.
Example of Global Exception Handler
@RestControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<ApiError> handleResourceNotFound(ResourceNotFoundException ex, WebRequest request) {
ApiError apiError = new ApiError(HttpStatus.NOT_FOUND.value(), "Not Found", ex.getMessage(), request.getDescription(false));
return new ResponseEntity<>(apiError, HttpStatus.NOT_FOUND);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<ApiError> handleGlobalException(Exception ex, WebRequest request) {
ApiError apiError = new ApiError(HttpStatus.INTERNAL_SERVER_ERROR.value(), "Error", ex.getMessage(), request.getDescription(false));
return new ResponseEntity<>(apiError, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Explanation of the Code
- @RestControllerAdvice: This annotation facilitates global exception handling across multiple controllers.
- @ExceptionHandler: Used to define the exception type you're handling.
- ResponseEntity: Returns a customizable HTTP response, allowing for consistent error structure.
Handling Validation Errors
Validating incoming request data is another critical aspect of error handling. When invalid data is sent to your API, you should be able to return meaningful feedback.
Example of Handling Validation Errors
@ExceptionHandler(MethodArgumentNotValidException.class)
public ResponseEntity<ApiError> handleValidationExceptions(MethodArgumentNotValidException ex, WebRequest request) {
ApiError apiError = new ApiError(HttpStatus.BAD_REQUEST.value(), "Validation Error",
ex.getBindingResult().getFieldErrors().stream()
.map(fieldError -> fieldError.getField() + ": " + fieldError.getDefaultMessage())
.collect(Collectors.joining(", ")), request.getDescription(false));
return new ResponseEntity<>(apiError, HttpStatus.BAD_REQUEST);
}
Why Handle Validation Errors?
Handling validation exceptions allows you to give tailored feedback to users, thereby preventing them from making unnecessary requests.
Creating Your Own Exception
Sometimes you need to throw application-specific exceptions. In this case, defining a custom exception class can be very useful.
Example of Custom Exception
public class ResourceNotFoundException extends RuntimeException {
public ResourceNotFoundException(String message) {
super(message);
}
}
When to Use Custom Exceptions
- Clarity: Differentiate between various error scenarios.
- Custom Handling: Tailor responses specifically for those exceptions.
Logging Errors
Logging is a fundamental aspect of monitoring and debugging your applications. Ensure that all exceptions are logged adequately to track issues.
Example of Logging Errors
@ExceptionHandler(Exception.class)
public ResponseEntity<ApiError> handleGlobalException(Exception ex, WebRequest request) {
logger.error("Unexpected error occurred: ", ex);
ApiError apiError = new ApiError(HttpStatus.INTERNAL_SERVER_ERROR.value(), "Error", ex.getMessage(), request.getDescription(false));
return new ResponseEntity<>(apiError, HttpStatus.INTERNAL_SERVER_ERROR);
}
Benefits of Logging
- Track Issues: Logs help identify and analyze the root causes of errors.
- Improve APIs: Insights from log data can refine your API's reliability.
The Last Word
Error handling in Spring applications is a critical component of developing robust RESTful APIs. Offering meaningful error responses improves the user experience, aids debugging efforts, and contributes to overall API integrity.
In this post, we've covered:
- The importance of error handling.
- Custom error classes to create meaningful responses.
- The role of
@ControllerAdvice
for central management of errors. - Strategies for validation and application-specific exceptions.
- Effective logging practices.
Mastering these techniques will help you design APIs that not only function well but are also resilient and user-friendly.
For further reading on robust error handling practices, check out the following resources:
- Spring Documentation on Error Handling
- Best Practices for REST API Error Handling
Happy coding!