Understanding JVM Pressure: Context Switching Pitfalls
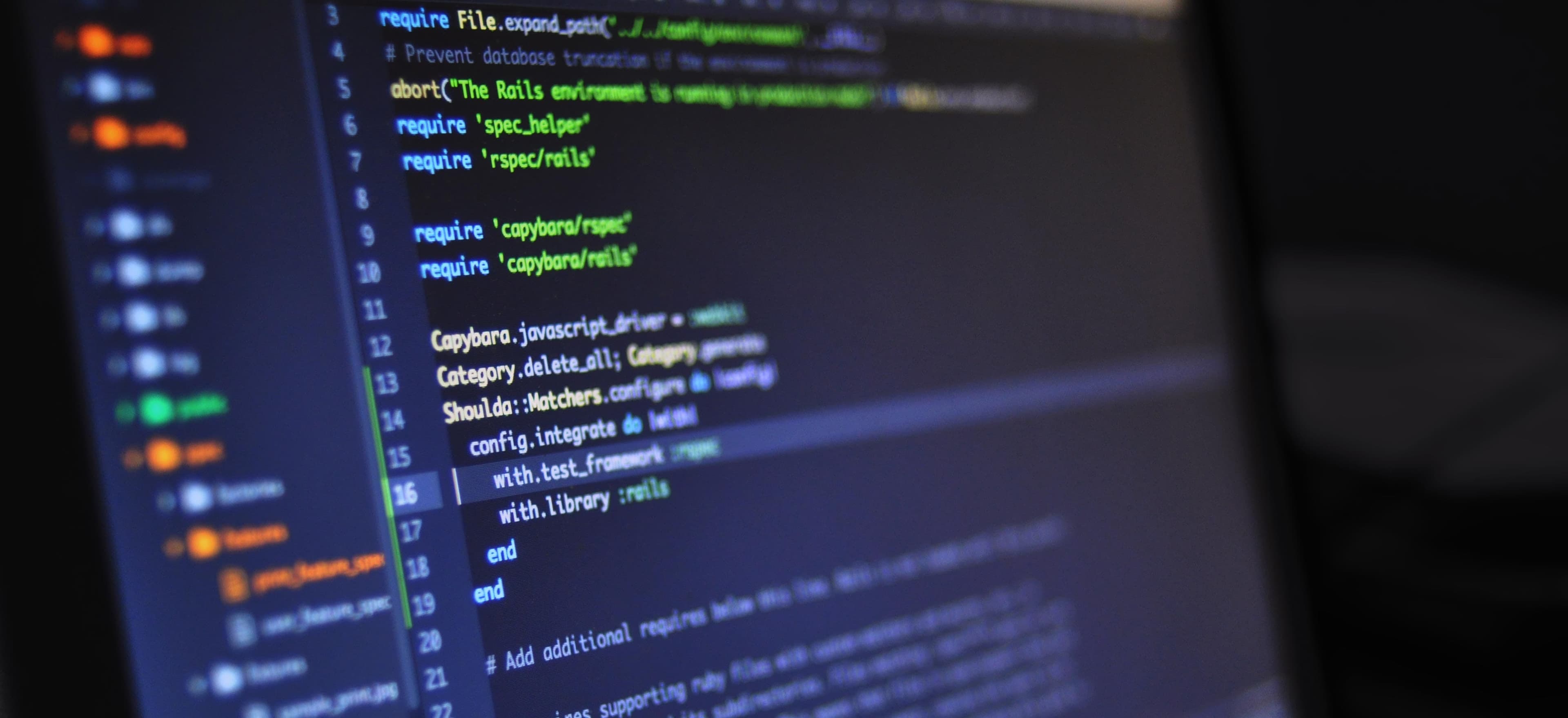
- Published on
Understanding JVM Pressure: Context Switching Pitfalls
In the world of Java programming, performance is often dictated by how well the Java Virtual Machine (JVM) manages resources. One of the critical concepts that developers must understand is the nature of JVM pressure, particularly when it comes to context switching. In this blog post, we'll explore what context switching is, how it impacts the JVM's performance, specific pitfalls to avoid, and practical code examples to illustrate these concepts.
What is Context Switching?
Context switching is the process by which a CPU (or a thread) switches from one task to another. This can occur when multiple threads are competing for CPU time. Each time a context switch occurs, the state of the current thread must be saved, and the state of the new thread must be loaded. This process consumes CPU cycles and can significantly hinder performance—especially in a multithreaded environment like the JVM.
The Cost of Context Switching
Context switches are not free. When the CPU switches between threads, it incurs several costs:
-
CPU Cycle Overhead: Each context switch requires saving the current state and loading the new state, which consumes CPU time.
-
Cache Performance: CPU caches may not be optimized for the newly scheduled thread, which can lead to poorer performance due to cache misses.
-
Resource Contention: With many threads, resource locks can become a bottleneck, leading to increased contention and reduced throughput.
JVM Architecture
Before diving deeper into the impact of context switching, it's essential to understand how the JVM operates. The JVM manages memory, threads, and garbage collection, and it operates on top of the host OS. When multiple Java threads are in play, the JVM must efficiently schedule these threads, often leading to context switching.
Understanding JVM Pressure
JVM pressure occurs when the JVM is stressed due to resource contention, memory allocation issues, or excessive context switches. This pressure can lead to poor performance and increased garbage collection times.
Signs of JVM Pressure
-
High CPU Usage: An increase in context switches typically leads to high CPU consumption.
-
Garbage Collection (GC) Pauses: If the JVM is under pressure, GC may take longer or become more frequent.
-
Increased Latency: Users may experience delays when interacting with applications.
The Hidden Costs of Context Switching
Let's delve deeper into the specific pitfalls that arise from excessive context switching:
1. Thread Exhaustion
When too many threads are created, the OS has to work hard to switch between them. This isn't just a performance drop; it can lead to thread exhaustion where new tasks can't be scheduled until existing ones complete.
public class ThreadExhaustionExample {
public static void main(String[] args) {
for (int i = 0; i < 10000; i++) {
new Thread(() -> {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}).start();
}
}
}
In this example, creating too many threads leads to high overhead due to constant context switching, degrading performance significantly.
2. Unoptimized Resource Allocation
When the JVM tries to manage memory and resources under pressure, it can lead to inefficient allocation and fragmentation.
public class ResourceAllocationExample {
public static void main(String[] args) {
for (int i = 0; i < 100; i++) {
// Simulate heavy resource allocation
byte[] bytes = new byte[1024 * 1024]; // Allocate 1MB
}
}
}
Here, rapid memory allocation can trigger frequent GC cycles, which further adds to latency.
Optimizing Context Switching in Java
Understanding how to optimize your JVM for context switching can lead to better performance. Here are some strategies:
1. Use Thread Pools
Instead of creating a new thread for each task, consider using a thread pool. Java's ExecutorService
framework provides a convenient method to manage a pool of threads effectively.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(10);
for (int i = 0; i < 100; i++) {
executor.submit(() -> {
// Simulated task
System.out.println("Task executed by: " + Thread.currentThread().getName());
});
}
executor.shutdown();
}
}
Using thread pools reduces the overhead associated with creating and destroying threads, minimizing context switching.
2. Optimize Locking
Avoiding unnecessary locking can help reduce contention among threads. Use read-write locks, and ensure critical sections are as short as possible.
import java.util.concurrent.locks.ReentrantReadWriteLock;
public class LockingExample {
private final ReentrantReadWriteLock lock = new ReentrantReadWriteLock();
public void readData() {
lock.readLock().lock();
try {
// Read operation here
} finally {
lock.readLock().unlock();
}
}
public void writeData() {
lock.writeLock().lock();
try {
// Write operation here
} finally {
lock.writeLock().unlock();
}
}
}
Using read-write locks allows multiple threads to read simultaneously, which can reduce the likelihood of context switching.
3. Monitor and Tune the JVM
JVM performance monitoring tools, such as JVisualVM or JConsole, allow you to analyze threads, memory, and CPU consumption.
You can find additional insights about tuning the JVM for performance at the official Oracle documentation.
The Last Word
Understanding JVM pressure and its relationship with context switching is essential for any Java developer. By utilizing strategies such as thread pooling, optimizing resource allocation, and monitoring JVM performance, we can significantly enhance the efficiency of our applications. Emphasizing these best practices will lead to a more responsive and robust Java application capable of handling the demands of modern software.
For further reading, you might explore The Java Concurrency in Practice to understand thread management and concurrency aspects in depth.
Embrace these best practices and let your applications run smoothly, efficiently, and effectively within the constraints of the JVM!
Checkout our other articles