Overcoming Complexity: The Challenges of Building Type Systems
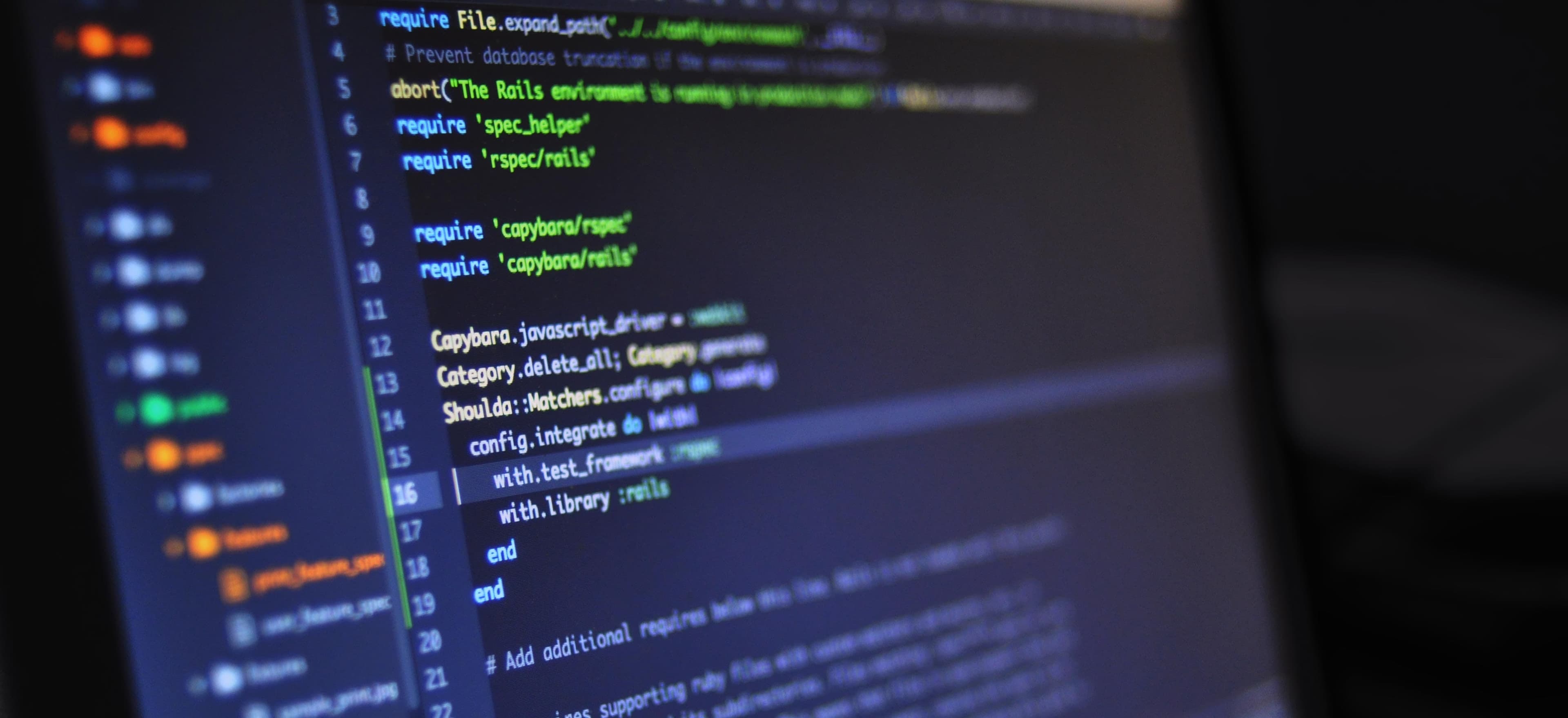
- Published on
Overcoming Complexity: The Challenges of Building Type Systems in Java
In the realm of programming languages, type systems play a pivotal role. They ensure that developers can write robust, maintainable, and error-free code. Java, a language popular for its strong type system, is particularly notable for how it influences both beginner and expert developers alike. However, building efficient and effective type systems can present several challenges.
What is a Type System?
A type system is a set of rules that assigns a property called "type" to data structures so that there can be an understanding of how different data types interact. In the context of programming, a type dictates what operations can be performed on a variable, how that variable can be used, and how it's stored in memory.
For instance, in Java, if you declare a variable as an integer, you can only perform operations applicable to integers unless explicitly converted to another type. Here’s a simple example demonstrating type declaration:
int number = 5; // This declares a variable of type 'int' and assigns it a value.
In the above code, 'int' is the type, which fundamentally controls what operations are permissible on the number
variable.
The Objectives of a Type System
The main goals of a type system in any language, including Java, are:
- Safety: Ensure that operations on data are type-safe.
- Expressiveness: Allow developers to define their own types for better abstraction.
- Efficiency: Enable the compiler to optimize the code by understanding data types.
- Readability: Improve the clarity of code, aiding both the writer and future maintainers.
Challenges in Building Type Systems
While building type systems, there are various complexities developers may encounter. Let’s discuss some of these key challenges that Java developers often face.
1. Type Inference
Type inference is the ability of a compiler to automatically deduce the type of a variable from its context. While Java has made strides in this area, especially with the introduction of features like the diamond operator (<>
) in Java 7 and the var keyword in Java 10, it can still be a complicated process.
Why is this challenging?
A robust type inference system needs to be both precise and efficient. It must minimize ambiguity while ensuring that the program remains flexible and readable. Errors in type inference can lead to runtime exceptions, undermining the reliability that strong type systems aim to provide.
var list = new ArrayList<String>(); // Automatic type inference with var
This allows developers to avoid redundant type declarations, but can sometimes lead to unexpected outcomes if not used carefully, as highlighted in this article.
2. Type Compatibility
Type compatibility dictates how similar types are in the system. Java uses strict rules and relationships, for instance, primitive types and their wrapper classes (int vs. Integer) are entirely distinct.
int primitiveVal = 10;
Integer wrapperVal = Integer.valueOf(primitiveVal); // explicit conversion
Why is this important?
A developer must keep these distinctions in mind, as this can lead to increased boilerplate code. Developers may need to perform additional conversions, impacting code clarity and maintainability.
3. Generics
Generics allow for type ambiguity and enable developers to create classes, interfaces, and methods with type parameters. However, the introduction of generics increased the complexity of the type system.
The challenge lies in the implementation.
One must ensure that type parameters are resolved correctly during both compilation and runtime. For instance, consider a simple generic class:
public class Box<T> {
private T item;
public Box(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
In this case, Box
can handle any type, which provides flexibility. However, understanding these generics requires developers to grasp concepts like bounds and wildcards, as outlined in Oracle's documentation on generics.
4. Nullability and Type Safety
One of the greatest challenges in Java is handling null references. While Java does not have a built-in null safety mechanism, the introduction of nullable types in Java 8 via Optional can help mitigate null-related errors but adds a layer of complexity.
Developers must decide when to use Optional and when it makes sense to use null:
Optional<String> optionalValue = Optional.of("Hello");
optionalValue.ifPresent(System.out::println);
Why does this matter?
By integrating Optional
, developers can make APIs more robust against null-related issues, but doing so requires an understanding of how and when to use it effectively.
5. Variance
Variance in type systems refers to the ability to substitute different types in generics. In Java, there are three main forms of variance: covariance, contravariance, and invariance. Understanding the relationship between these forms can be non-trivial.
For example, consider the following code:
List<? extends Number> numbers = new ArrayList<Integer>(); // Covariant
Challenges in achieving Variance:
Successfully implementing variance can often mean the difference between type safety and runtime errors. Minor oversights can lead to code that compiles but fails at runtime, leading to potential liability issues.
Best Practices for Overcoming Type System Challenges
To navigate these challenges successfully, Java developers can adopt certain best practices:
- Foster understanding through documentation. Ensure that your code is well-documented, highlighting the types and potential pitfalls.
- Encourage the use of frameworks that facilitate type safety. Libraries like Lombok reduce boilerplate code associated with type declarations.
- Leverage IDE capabilities. Use IDEs (like IntelliJ IDEA or Eclipse) that provide real-time feedback and warnings regarding type issues.
- Write comprehensive unit tests. Safeguard against potential type errors through thorough testing.
Closing Remarks
Building a type system can indeed be challenging, particularly in a versatile language like Java. Nonetheless, by understanding the intricacies of type inference, compatibility, generics, nullability, and variance, developers can overcome these hurdles.
Embracing best practices, leveraging tools, and continuously being aware of the evolving language features will create a more enjoyable coding experience. In the land of type systems, knowledge is power, and every Java developer can benefit from mastering these complexities.
For further reading, you can explore resources like Java Documentation or delve into Java Generics. These invaluable references can significantly enhance your understanding and application of type systems in Java programming.
This blog post serves as an overview of the challenges of building type systems in Java, highlighting critical concepts while providing code examples and recommendations. The intricacies of type systems are both challenging and rewarding, representing a key component of a developer's toolkit in creating robust applications.
Checkout our other articles