Mastering the Producer-Consumer Problem with Efficient Solutions
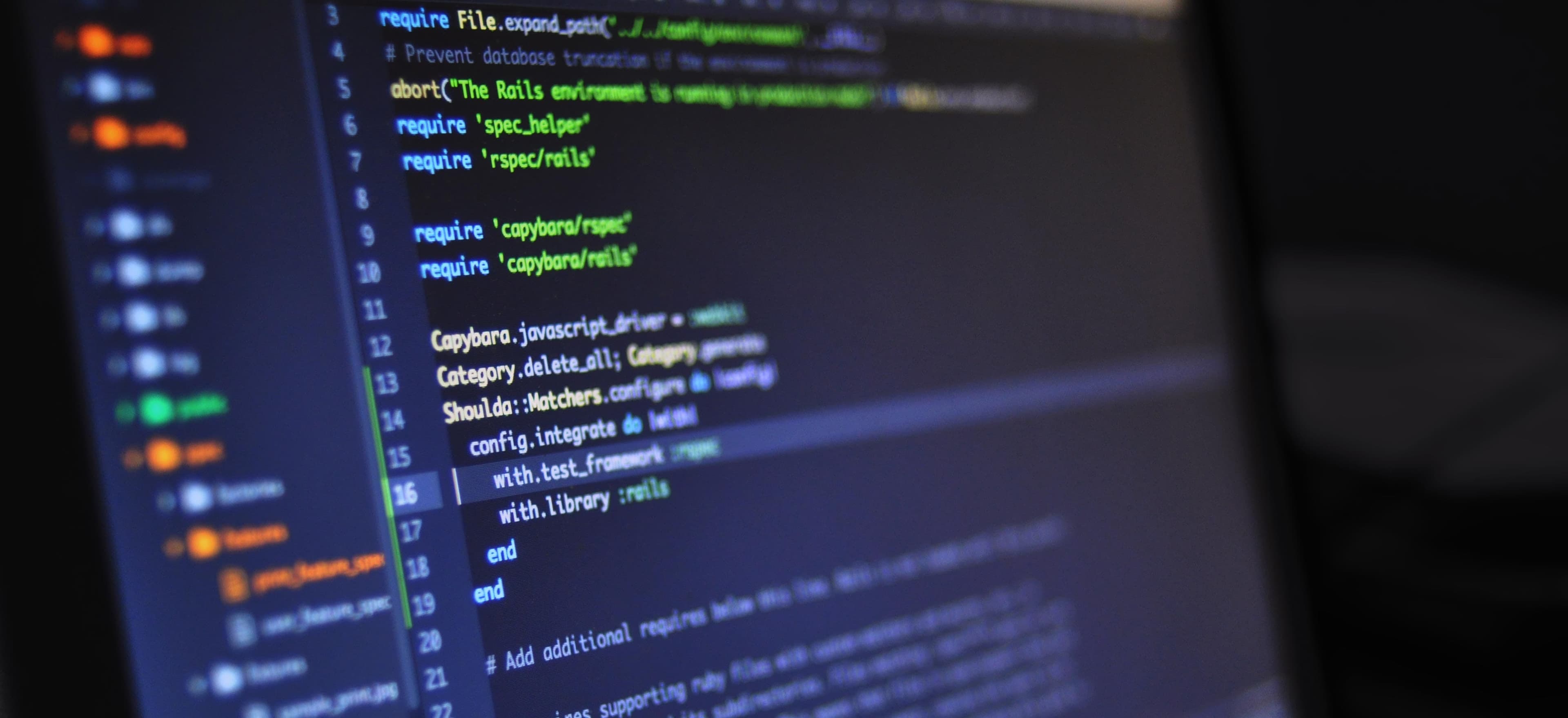
- Published on
Mastering the Producer-Consumer Problem with Efficient Solutions
The Producer-Consumer problem is a classic scenario in concurrent programming. It describes the situation where two processes—producers and consumers—share a common, finite-size buffer. Producers generate data, put it into the buffer, and consumers take data out of the buffer. This model has real-world applications in multiple domains, including operating systems, database management, and network communication.
Understanding and efficiently solving the Producer-Consumer problem is essential for any developer working in concurrent environments. In this blog post, we will delve into the Producer-Consumer problem, explore its intricacies, and provide you with effective solutions in Java.
Understanding the Producer-Consumer Problem
Why is it Important?
In parallel processing, the Producer-Consumer problem highlights the need for synchronization. If a producer tries to add to a full buffer, or if a consumer attempts to remove from an empty buffer, it can lead to data inconsistency or even cause a crash. Hence, implementing synchronization mechanisms is crucial for stability and reliability.
Fundamental Concepts
- Producers: These processes produce data at a rate that may not necessarily match the consumers. They place items in a shared buffer.
- Consumers: These processes consume data from the buffer and do so at a rate that may differ from the producers.
- Buffer: A finite-size data structure that holds the items produced by the producers until they are consumed.
Implementing the Producer-Consumer Problem in Java
In Java, there are several methods for implementing the Producer-Consumer pattern. We will look at two widely used techniques: using wait()
, notify()
, and using Java's built-in concurrency library featuring BlockingQueue
.
Method 1: Using wait() and notify()
The first method utilizes the Object class's wait()
and notify()
methods for inter-thread communication. Below is a simplified example.
import java.util.LinkedList;
class Buffer {
private final LinkedList<Integer> queue = new LinkedList<>();
private final int limit;
public Buffer(int limit) {
this.limit = limit;
}
public synchronized void produce(int value) throws InterruptedException {
while (queue.size() == limit) {
wait(); // Wait until space is available
}
queue.add(value);
notifyAll(); // Notify consumers
}
public synchronized int consume() throws InterruptedException {
while (queue.isEmpty()) {
wait(); // Wait until an item is available
}
int value = queue.removeFirst();
notifyAll(); // Notify producers
return value;
}
}
Commentary
- Synchronize: The
synchronized
keyword ensures that only one thread can execute the methods at a time. - wait() and notifyAll(): Both methods enable thread communication. If the buffer is full, producers wait until consumers consume an item. Conversely, if the buffer is empty, consumers wait until producers produce an item.
Method 2: Using BlockingQueue
Java provides built-in concurrency utilities, with BlockingQueue
being an excellent option for implementing the Producer-Consumer problem. The BlockingQueue
interface allows for better handling of thread communication without explicitly using wait and notify.
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.BlockingQueue;
class ProducerConsumer {
private final BlockingQueue<Integer> queue;
public ProducerConsumer(int limit) {
this.queue = new ArrayBlockingQueue<>(limit);
}
public void produce(int value) throws InterruptedException {
queue.put(value); // Automatically waits if the queue is full
}
public int consume() throws InterruptedException {
return queue.take(); // Automatically waits if the queue is empty
}
public static void main(String[] args) {
ProducerConsumer pc = new ProducerConsumer(5);
// Producer thread
Thread producerThread = new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
pc.produce(i);
System.out.println("Produced: " + i);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
});
// Consumer thread
Thread consumerThread = new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
int value = pc.consume();
System.out.println("Consumed: " + value);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
});
producerThread.start();
consumerThread.start();
}
}
Commentary
- BlockingQueue Implementation: Here,
ArrayBlockingQueue
is used. It implements theBlockingQueue
interface and provides a thread-safe way to handle producer-consumer workflows. - put() and take(): These methods block the calling thread until space becomes available or until an item can be retrieved, respectively. There is no need for explicit
wait()
andnotify()
.
Performance Considerations
While both methods allow for the correct implementation of the Producer-Consumer problem, the choice between them depends on the application requirements:
-
Manual Synchronization (
wait()
andnotify()
):- Provides total flexibility but requires careful design to avoid issues like deadlocks.
- More lines of code to maintain.
-
Java’s
BlockingQueue
:- Cleaner and easier to maintain.
- Automatically handles waiting and notifying, allowing developers to focus on the application logic rather than thread management.
For more in-depth information on concurrency in Java, refer to the Java Concurrency Tutorial.
The Last Word
Mastering the Producer-Consumer problem is fundamental in concurrent programming. By using Java's concurrency features, such as wait()
and notify()
, or the more convenient BlockingQueue
, developers can create robust applications that efficiently manage resources without running into common pitfalls.
We hope this article has provided you with a clear understanding of the Producer-Consumer problem and how to implement it in Java. Whether you're a seasoned developer or a newcomer, mastering this concept will undoubtedly enhance your programming skills in multi-threaded environments.
For further reading, check out these resources on concurrency patterns and practices:
- Java Concurrency in Practice, a must-read book for anyone interested in deepening their understanding of Java's concurrency model.
- Effective Java focuses on best practices and patterns that you should adhere to for excellent Java programming.
Happy coding!
Checkout our other articles