Troubleshooting Spring Data Read Replica Configuration
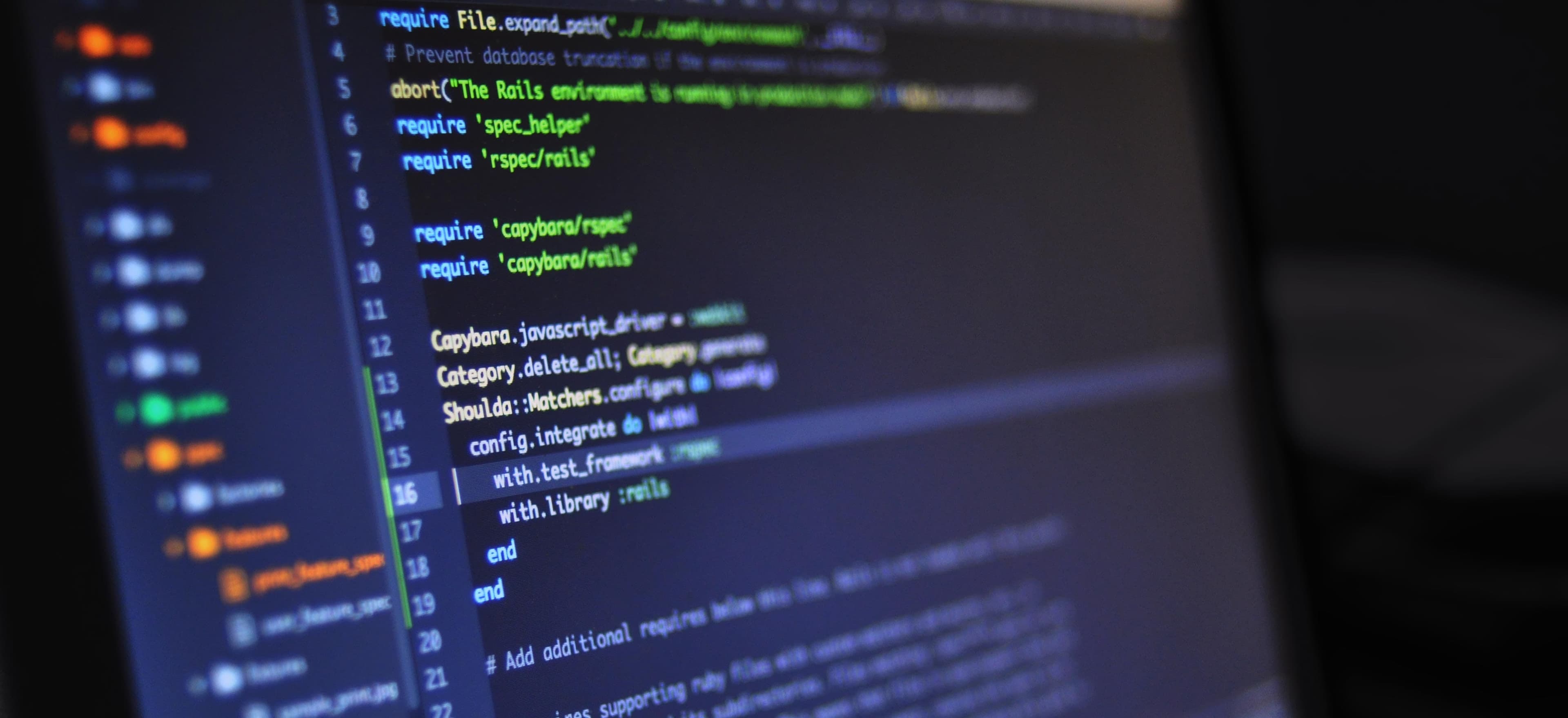
- Published on
Troubleshooting Spring Data Read Replica Configuration
In modern web applications, ensuring high availability and load balancing is paramount. As part of that architecture, using read replicas can facilitate better read performance, increased reliability, and overall scalability of the database layer. In this blog post, we will dive deep into how to configure read replicas with Spring Data, while also addressing common troubleshooting challenges that you may encounter.
Understanding Read Replicas
Before we delve into the configuration nuances, let's clarify what read replicas are. A read replica is a copy of the primary database that allows read operations without putting undue stress on the primary instance. Typically, read replicas are used to handle read-heavy workloads, allowing for efficient load distribution and enhanced performance.
Benefits of Read Replicas
- Scalability: Distributing reads across multiple replicas helps manage increased load effectively.
- Reliability: In case of a primary database failure, you can switch over to a read replica.
- Performance: By offloading read operations, the primary database can focus on write operations, improving overall application performance.
Setting Up Spring Data with Read Replicas
Spring Data provides a straightforward way to manage data repositories and connect to different databases. However, the configuration for read replicas comes with its own hitches. We'll start with an example of a basic setup involving Spring Data JPA.
Step 1: Dependency Management
Make sure you include Spring Data JPA and the relevant database connector in your pom.xml
if you are using Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
For Gradle, the corresponding configuration would look like this:
implementation 'org.springframework.boot:spring-boot-starter-data-jpa'
runtimeOnly 'com.h2database:h2'
Step 2: Configure Data Sources
To connect to read replicas, you need to define multiple data sources in your application configuration (e.g., application.yml
). A simplified setup might look like this:
spring:
datasource:
primary:
url: jdbc:mysql://primary-db-url:3306/mydb
username: user
password: password
replicas:
- url: jdbc:mysql://replica1-db-url:3306/mydb
username: user
password: password
- url: jdbc:mysql://replica2-db-url:3306/mydb
username: user
password: password
Step 3: Create Read/Write Routing
To effectively route read and write operations to the corresponding data sources, you can use a routing data source. Here’s a simple implementation:
import org.springframework.jdbc.datasource.lookup.AbstractRoutingDataSource;
public class ReadWriteRoutingDataSource extends AbstractRoutingDataSource {
@Override
protected Object determineCurrentLookupKey() {
// Logic to determine current context
return DataSourceContext.getDataSourceType();
}
}
This class overrides the determineCurrentLookupKey
method, which checks the context to route read or write operations appropriately.
Step 4: Set Up the Context
You must establish a context to hold the current data source type:
public class DataSourceContext {
private static final ThreadLocal<String> contextHolder = new ThreadLocal<>();
public static void setDataSourceType(String dataSourceType) {
contextHolder.set(dataSourceType);
}
public static String getDataSourceType() {
return contextHolder.get();
}
public static void clear() {
contextHolder.remove();
}
}
Step 5: Using Annotations for Read/Write Operations
To further streamline the data source usage, you might want to control which operations go to read replicas or the primary database. Use annotations to do this effectively:
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Pointcut;
import org.aspectj.lang.annotation.Before;
@Aspect
public class DataSourceAspect {
@Pointcut("@annotation(Write)")
public void writeOperation() {}
@Pointcut("@annotation(Read)")
public void readOperation() {}
@Before("writeOperation()")
public void setWriteDataSource() {
DataSourceContext.setDataSourceType("PRIMARY");
}
@Before("readOperation()")
public void setReadDataSource() {
DataSourceContext.setDataSourceType("REPLICA");
}
}
With this setup, you can annotate your repository methods and effortlessly switch between read and write operations.
Common Troubleshooting Steps
Configuring read replicas can sometimes lead to issues. Below are common problems and how to fix them.
1. Connections Not Routing Properly
Issue: Your application may not direct requests correctly to the primary or replica databases.
Solution: Ensure your routing key logic in determineCurrentLookupKey()
is functioning as intended. Add logging for better visibility.
@Override
protected Object determineCurrentLookupKey() {
String dataSourceKey = DataSourceContext.getDataSourceType();
System.out.println("Current DataSource Key: " + dataSourceKey); // Debugging
return dataSourceKey;
}
2. Failed Connections to Read Replicas
Issue: The application throws exceptions when trying to connect to read replicas.
Solution: Double-check your database URLs and credentials. You can also implement connection pooling to enhance robustness.
3. Data Consistency Issues
Issue: Users experience stale data because replicas lag behind.
Solution: Monitor your replication latency. Depending on your use case, you can use different read strategies or make your application more resilient by writing data to the primary before reading.
4. Read Operations Attempting Writes
Issue: A read operation tries to perform a write, leading to failures.
Solution: Review your annotations and ensure that they are appropriately applied.
5. Load Balancing Read Requests
Issue: Read requests are not distributed among read replicas efficiently.
Solution: Implement a custom load balancing strategy, such as round-robin selection of replicas for read requests to evenly distribute the load.
Closing the Chapter
Configuring and troubleshooting Spring Data read replica setups can significantly enhance your application's performance and reliability. This guide provided a robust framework for achieving this while addressing common issues.
The beauty of Spring Data lies in its powerful abstractions, allowing developers to focus more on building rather than wrestling with database connectivity. Happy coding!
For further reading, consider exploring the official Spring Data Documentation to dive deeper into repository interfaces, query creation, and more.
This guide is your roadmap to successfully implementing and troubleshooting Spring Data read replicas. Follow the practices outlined, and you will ensure a scalable, performant application architecture.
Checkout our other articles