Overcoming Challenges in OpenAPI Custom Code Generation
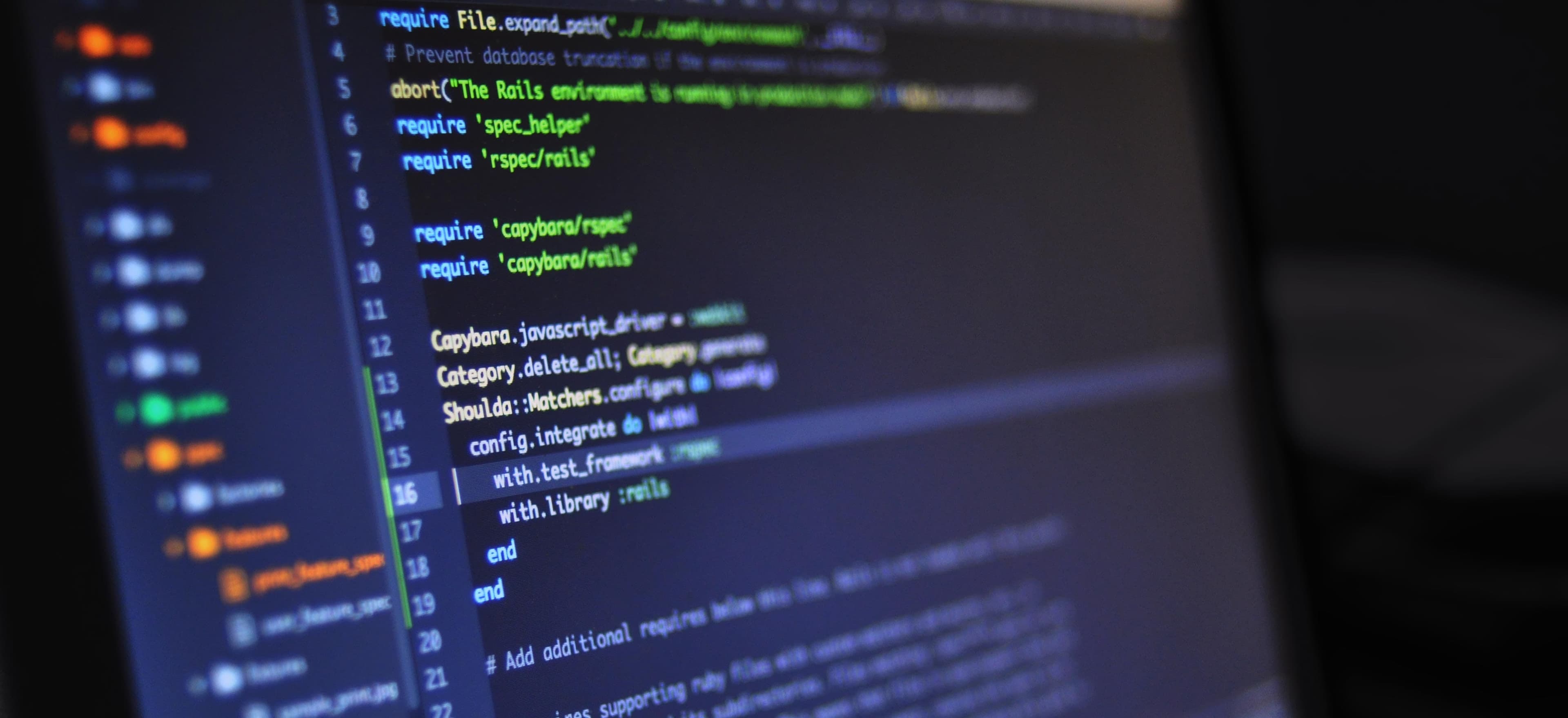
- Published on
Overcoming Challenges in OpenAPI Custom Code Generation
In today's software development landscape, APIs have become the cornerstone of creating scalable and maintainable applications. OpenAPI, formerly known as Swagger, is a specification for documenting RESTful APIs, offering a comprehensive way to define everything from endpoints to input-output formats. However, as organizations leverage OpenAPI for code generation, they often encounter several challenges. In this article, we will explore these challenges and provide actionable solutions to overcome them.
Understanding OpenAPI and Code Generation
What is OpenAPI?
OpenAPI is a specification that provides a standard way to describe RESTful APIs. The beauty of OpenAPI lies in its ability to facilitate code generation — both server and client-side code can be auto-generated from an OpenAPI definition file. This can significantly reduce human error and expedite the development workflow.
Why Code Generation?
Code generation from an OpenAPI specification offers several advantages, including:
- Consistency: It standardizes how APIs are constructed, making it easier for teams to work together.
- Speed: By auto-generating boilerplate code, developers can focus on implementing business logic.
- Documentation: Code generated from OpenAPI comes with built-in documentation, making APIs easier to consume.
Common Challenges in OpenAPI Custom Code Generation
Despite the benefits, numerous obstacles can arise during custom code generation from OpenAPI specifications. Below, we discuss some of the key challenges and their solutions.
1. Incomplete or Inaccurate Specifications
One of the biggest challenges developers face is working with incomplete or inaccurate OpenAPI specifications.
Solution
Ensure that the OpenAPI specification is thoroughly reviewed and validated before generation. Tools such as Swagger Editor allow you to edit and validate your specification in real-time. Additionally, using tools like Speccy can help in linting OpenAPI files, ensuring they adhere to best practices.
2. Complexity in Customization
Out-of-the-box code generators may not cover all custom use cases or specific architectural requirements.
Solution
Consider creating a custom generator. For example, if you are using Java, you can employ the OpenAPI Generator to create a tailored implementation that suits your needs. Below is a simple snippet demonstrating how to generate a Java client using OpenAPI Generator:
openapi-generator generate -i your-api-spec.yaml -g java -o /path/to/output/directory
The command above specifies an OpenAPI file (your-api-spec.yaml
), the target language (java
), and the output directory. You can customize the generator by creating your own templates based on the default ones. This flexibility allows for adaptations to frameworks like Spring or Jakarta EE.
3. Versioning Issues
As APIs evolve, versioning can become a significant headache, especially if the OpenAPI specification is not versioned correctly.
Solution
Adopt semantic versioning for your OpenAPI specifications and maintain multiple versions as necessary. Ensure your CI/CD pipeline incorporates these specifications in a structured manner. Here, tagging versions in your git repository can be highly beneficial:
git tag -a v1.0 -m "Initial version of OpenAPI specification"
This allows teams to reference specific versions without breaking existing consumer dependencies.
4. Dependency Management
Generated code often relies on third-party libraries that may not align with your existing ecosystem.
Solution
Carefully evaluate and manage dependencies. Use tools such as Maven or Gradle to handle library versions. Below is an example of how to specify dependencies in a Maven pom.xml
:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.6.0</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.12.5</version>
</dependency>
</dependencies>
Make sure to periodically audit these dependencies as part of your development process.
5. Testing and Validation
Generated code does not guarantee that APIs will behave as intended. An absence of thorough testing can lead to production issues.
Solution
Implement a robust testing strategy. Use tools like JUnit for unit tests and RestAssured for API testing. Here’s how you might write a simple test case using JUnit and RestAssured for an OpenAPI-generated endpoint:
import io.restassured.RestAssured;
import org.junit.jupiter.api.Test;
import static io.restassured.RestAssured.*;
import static org.hamcrest.Matchers.*;
public class ApiTest {
@Test
public void testGetUser() {
RestAssured.baseURI = "http://localhost:8080/api";
given().
when().
get("/users/1").
then().
statusCode(200).
body("username", equalTo("john_doe"));
}
}
This test ensures that the API behaves correctly and returns the expected data.
Summary
Navigating the complexities of OpenAPI custom code generation doesn't have to be an overwhelming task. By focusing on creating accurate specifications, employing custom generators, managing versions and dependencies, and instituting thorough testing, developers can effectively overcome these challenges.
For further reading on the OpenAPI specification and its applications, check out these resources:
By proactively addressing these challenges, you can maximize the efficiency of your development workflow and build robust applications that stand the test of time. Whether you're a seasoned developer or just starting, understanding these intricacies will help you harness the full power of OpenAPI and streamline your API development cycle.