Common JGit Setup Issues and How to Fix Them
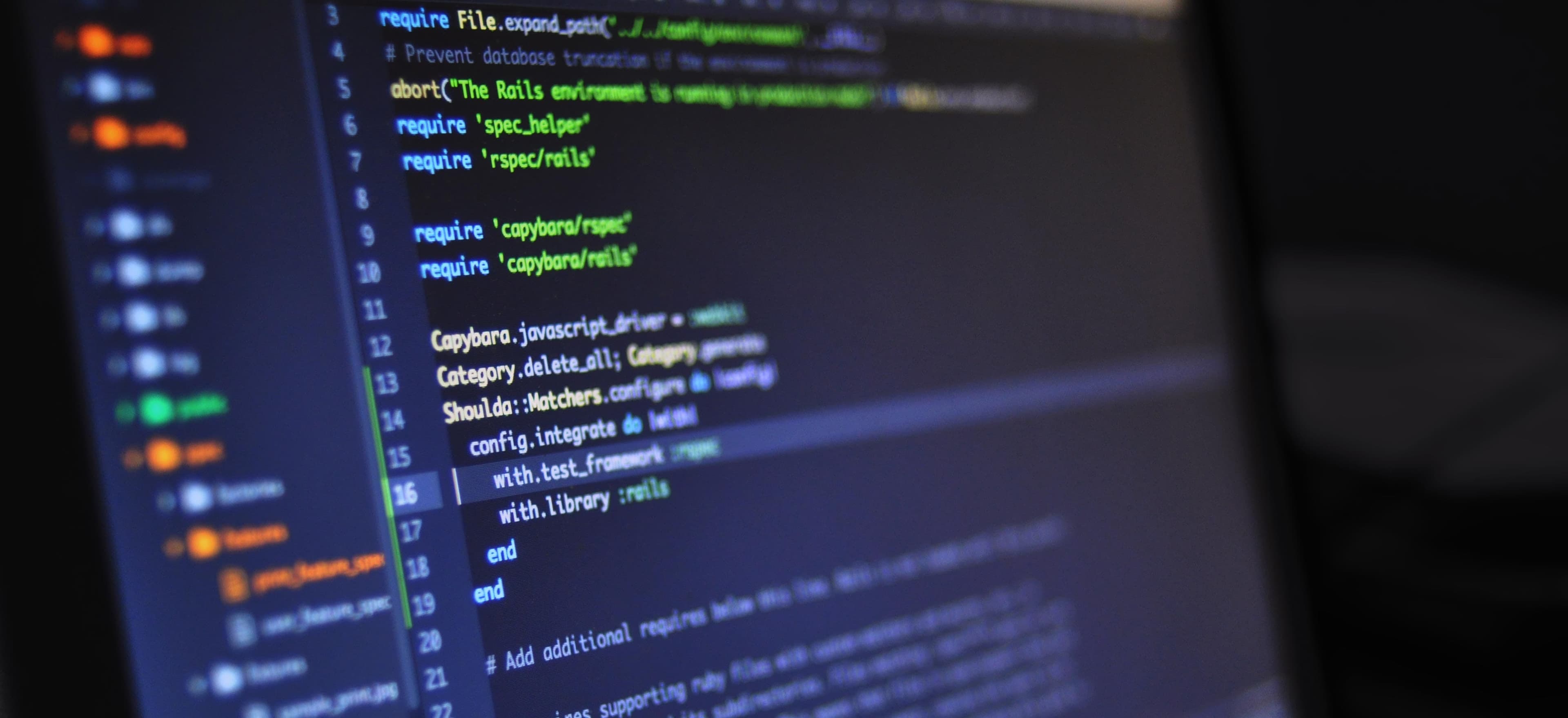
- Published on
Common JGit Setup Issues and How to Fix Them
JGit is a pure Java implementation of the Git version control system, designed to allow developers to interact with Git repositories programmatically. Although JGit offers immense flexibility and power, it’s not without its share of setup challenges. This blog post will walk you through common issues that arise during JGit setup and how to address them effectively.
Table of Contents
- Introduction to JGit
- Common Setup Issues
- Fixing JGit Configuration Problems
- Working with JGit
- Conclusion
Essentials at a Glance to JGit
JGit provides a robust API for managing Git repositories without needing the Git command-line tools. Its primary use cases include integrating Git functionalities into Java applications and automating Git workflows. Here’s how you can get started with a simple Maven dependency for JGit:
<dependency>
<groupId>org.eclipse.jgit</groupId>
<artifactId>org.eclipse.jgit</artifactId>
<version>5.13.1.202308271419-r</version>
</dependency>
Including the above in your pom.xml
will allow Maven to download JGit and make it available to your Java application.
Common Setup Issues
While setting up JGit, developers often encounter several issues that can hinder their workflow. Below are some of the most common problems, along with their solutions.
1. Incorrect Maven dependency version
One of the primary challenges arises from using the wrong version of the JGit library. If your project isn't referencing the correct version, you may face runtime errors or missing classes.
Solution
Always check the JGit Maven Repository to ensure you have the latest stable version available. It's a good practice to specify your dependencies explicitly and avoid using the latest
version tag.
2. Java Version Compatibility
Another common issue is the compatibility between the JGit library and the Java version you are using. JGit
has moved to Java 8 and later versions, and using an outdated Java version can lead to compilation errors.
Solution
Make sure that you are using a compatible Java version for your application. You can check your current Java version with this command:
java -version
To specify the Java version in your pom.xml
, use the following:
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
3. Missing Git Command-Line Tools
JGit aims to provide a Java-centric experience, but some functionalities may still require access to Git command-line tools. If you try to execute certain operations without having Git installed, you’ll encounter Command failed
messages.
Solution
Make sure to install Git on your machine. You can download it from the official Git website. After installation, verify it by running:
git --version
Fixing JGit Configuration Problems
Configuration is crucial when setting up any library, including JGit. Below are some configuration challenges and their respective fixes.
1. Invalid Repository Path
One of the most frequent issues is providing an incorrect path to the Git repository. If the repository doesn’t exist at the specified location, JGit will throw a “repository not found” error.
Solution
To create and open a JGit repository, follow this example:
import org.eclipse.jgit.api.Git;
import org.eclipse.jgit.api.errors.GitCommandException;
public class JGitExample {
public static void main(String[] args) {
try (Git git = Git.init().setDirectory(new File("path/to/repo")).call()) {
System.out.println("Created a new repository at " + git.getRepository().getDirectory());
} catch (GitCommandException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
2. Authentication Issues with Remote Repositories
When working with remote repositories, you might run into authentication problems, especially if you’re cloning a private repo.
Solution
To resolve this, you’ll need to provide valid credentials. JGit supports SSH keys and HTTPS authentication. Below is an example of cloning a repository using HTTPS:
import org.eclipse.jgit.api.Git;
import org.eclipse.jgit.api.errors.GitAPIException;
import org.eclipse.jgit.transport.UsernamePasswordCredentialsProvider;
public class CloneRepo {
public static void main(String[] args) {
String repoUrl = "https://github.com/your-repo.git";
String username = "your-username";
String password = "your-password";
try {
Git git = Git.cloneRepository()
.setURI(repoUrl)
.setCredentialsProvider(new UsernamePasswordCredentialsProvider(username, password))
.setDirectory(new File("local-repo"))
.call();
System.out.println("Cloned repository at " + git.getRepository().getDirectory());
} catch (GitAPIException e) {
e.printStackTrace();
}
}
}
3. Performance Problems with Large Repositories
When working with large repositories, JGit might exhibit performance issues. Loading large files or many commits can be time-consuming.
Solution
Consider optimizing your repository management. Limit the number of files being tracked or use a shallow clone if only recent history is required. You can create a shallow clone like this:
Git.cloneRepository()
.setURI(repoUrl)
.setDepth(1) // Only clone the latest commit
.setDirectory(new File("shallow-repo"))
.call();
Working with JGit
Once the setup issues are resolved, you can start working with JGit. Below, you will find a simple example of adding, committing, and pushing changes to a repository.
import org.eclipse.jgit.api.Git;
import org.eclipse.jgit.api.errors.GitAPIException;
import org.eclipse.jgit.api.errors.InvalidRemoteException;
import java.io.File;
public class CommitAndPush {
public static void main(String[] args) {
File repoDir = new File("path/to/repo");
try (Git git = Git.open(repoDir)) {
// Add files
git.add().addFilepattern(".").call();
// Commit changes
git.commit().setMessage("Your commit message").call();
// Push changes
git.push().call();
System.out.println("Changes pushed to remote repository.");
} catch (IOException | GitAPIException e) {
e.printStackTrace();
}
}
}
In this code snippet:
- We open the existing repository.
- We add all changes and commit them with a message.
- Finally, we push the changes to the remote repository.
Closing the Chapter
Setting up JGit can indeed be filled with challenges, but with the right knowledge and guidance, it's a seamless experience. Remember to check for compatibility, ensure you've installed the necessary tools, and proactively address configuration issues. Mastering JGit can empower you to leverage Git capabilities programmatically within your Java applications.
For deeper insights into JGit and its capabilities, consider exploring the official JGit documentation for more advanced features and use cases.
Checkout our other articles