Speed Up Your Java Coding: Eclipse Remove Unused Imports Shortcut
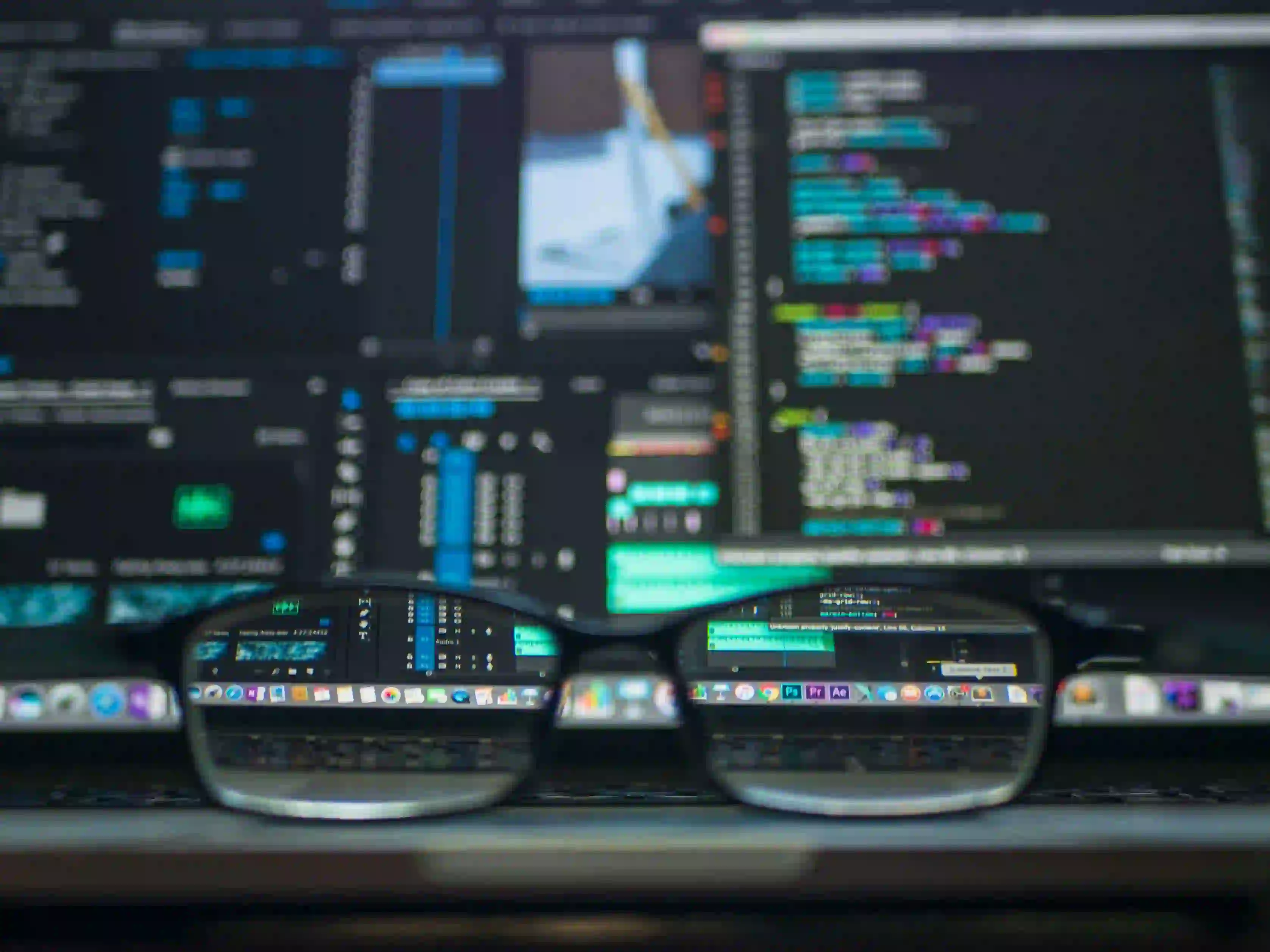
Speed Up Your Java Coding: Eclipse Remove Unused Imports Shortcut
In the world of Java development, efficiency is key. Developers seek to streamline their processes, enhance productivity, and write cleaner code. One of the simple yet effective practices is managing imports efficiently. Eclipse IDE provides a handy Remove Unused Imports feature that can significantly speed up your coding process while keeping your code clean and error-free.
In this blog post, we will explore the importance of managing imports in Java, how to remove unused imports in Eclipse, and some additional tips to enhance your Java coding experience.
Why Is Managing Imports Important?
Java is an object-oriented programming language that relies heavily on classes and libraries. This means that as a Java developer, you often need to import various classes and packages into your code. Over time, as your code evolves, some of these imports may become unnecessary. Here’s why managing imports is crucial:
1. Improved Readability
Unused imports can clutter your code, making it harder to read and understand. By removing these unnecessary statements, your code will be cleaner, allowing others (or even yourself) to understand it more easily in the future.
2. Reduced Compile Time
Although the impact might be minor for small applications, managing imports can reduce compilation time, especially in large codebases. The Java compiler doesn't need to deal with unnecessary imports, making your builds slightly faster.
3. Error Prevention
Sometimes, unused imports can lead to confusion and errors. For instance, if two classes share the same name but belong to different packages, the wrong import could be selected, leading to compilation errors.
Now that we understand the importance of managing imports, let’s delve into how you can quickly remove them in Eclipse.
How to Remove Unused Imports in Eclipse
Eclipse IDE offers an efficient shortcut to remove unused imports throughout your Java files. Here’s how to do it:
Step 1: Open Your Java File
Start by opening the Java file from which you want to remove unused imports.
Step 2: Use the Remove Unused Imports Shortcut
Eclipse provides a keyboard shortcut for this feature. Simply press Ctrl + Shift + O
(on Windows/Linux) or Cmd + Shift + O
(on macOS).
Step 3: Observe the Changes
Upon executing this shortcut, Eclipse will automatically refactor your imports, removing any that are not used in your code. You can easily spot the changes in the Imports
section at the top of your Java file.
For instance, if your file initially looks like this:
import java.util.List;
import java.util.ArrayList;
import java.awt.Color; // Unused import
public class Example {
public void createList() {
List<String> items = new ArrayList<>();
// Logic to populate the list
}
}
After applying the shortcut, it will be streamlined to:
import java.util.List;
import java.util.ArrayList;
public class Example {
public void createList() {
List<String> items = new ArrayList<>();
// Logic to populate the list
}
}
Notice how the unused import java.awt.Color;
was removed, making the code cleaner.
Customizing the Import Clean-Up Behavior
Although the default behavior works for most situations, you might want to further customize how Eclipse handles imports.
Changing the Import Preferences
You can access import preferences in Eclipse by going to:
Window -> Preferences -> Java -> Code Style -> Organize Imports
Here, you can specify rules for how Eclipse organizes imports. For example:
- Set the maximum number of imports to be statically organized.
- Define how to handle wildcard imports versus individual imports.
This level of customization allows you to tailor the IDE to your development style, enhancing your productivity even further.
Additional Tips for Efficient Java Coding
- Turn on Automatic Import Management
You can configure Eclipse to automatically manage your imports as you code. To enable this:
- Go to
Window -> Preferences -> Java -> Editor -> Save Actions
. - Check the boxes for "Perform the selected actions on save" and choose to organize imports.
Now, every time you save your Java file, Eclipse will ensure that all unused imports are removed automatically.
- Utilize Code Formatting Tools
Eclipse also has built-in tools for formatting your code. Press Ctrl + Shift + F
to format your code according to the specified coding standards, which often includes organizing imports.
- Use Java Annotations Wisely
Annotations such as @SuppressWarnings("unused")
can help you manage potential warnings for unused variables or methods. However, use them judiciously; they can sometimes mask deeper issues in your code.
Example Code Snippet Using Annotations
public class Example {
@SuppressWarnings("unused")
private String unusedString;
public void displayMessage() {
System.out.println("Hello World");
}
}
In this example, the unusedString
variable is marked with the annotation, which prevents the compiler from throwing a warning about it being unused.
- Regular Code Reviews
Engaging in regular peer code reviews can help identify areas for improvement, including management of imports. Fresh eyes may catch unused imports that the original developer overlooked.
Lessons Learned
Efficient code management is fundamental in Java development, and managing imports plays a significant role in maintaining clean, readable, and error-free code. The Eclipse IDE's capability to remove unused imports with a simple shortcut not only saves time but also enhances your programming experience.
After implementing these tips and shortcuts, you'll likely notice significant improvements in your workflow. Regularly maintaining your imports will lead to cleaner code and a more efficient coding environment.
For more insights on Java coding or to optimize your programming skills with tools like Eclipse, keep following our blog. Happy coding!
By engaging with the aspects discussed in this article, you can significantly enhance your Java coding efficiency using Eclipse. For further reading on Java practices, check out Oracle's Java Documentation or Baeldung's Java Tutorials.