Overcoming State Pattern Pitfalls in Software Design
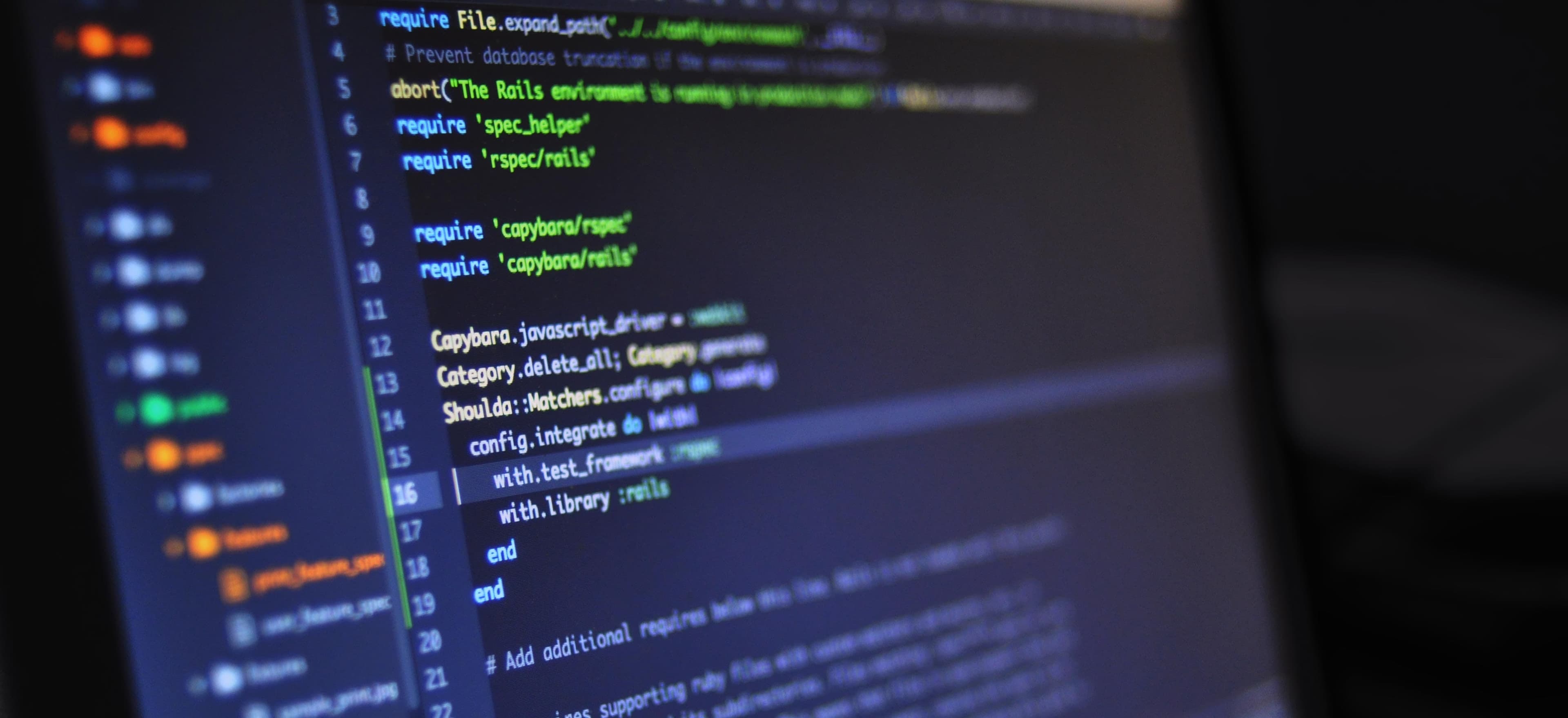
- Published on
Overcoming State Pattern Pitfalls in Software Design
The State Pattern is an integral part of object-oriented programming, often employed to manage an object’s states and behaviors throughout its lifecycle. However, like any design pattern, it comes with its own set of pitfalls. In this blog post, we will delve deep into the State Pattern, explore common challenges developers face, and discuss ways to overcome these obstacles while maximizing the strengths of this powerful design strategy.
Understanding the State Pattern
Before we dive into the pitfalls, it's essential to understand what the State Pattern is and why it is beneficial.
The State Pattern allows an object to alter its behavior when its internal state changes. Essentially, it encapsulates state-specific behaviors and delegates the requests to the current state object.
When to Use the State Pattern?
The State Pattern is particularly useful in scenarios where:
- An object has numerous states, each with distinct behaviors and requirements.
- You want to avoid giant conditional statements (like switch-case or if-else).
- The states can change dynamically at runtime.
How the State Pattern Works
Here’s a simple implementation of the State Pattern in Java.
interface State {
void handleRequest();
}
class ConcreteStateA implements State {
public void handleRequest() {
System.out.println("Handling request in State A.");
}
}
class ConcreteStateB implements State {
public void handleRequest() {
System.out.println("Handling request in State B.");
}
}
class Context {
private State state;
public void setState(State state) {
this.state = state;
}
public void request() {
state.handleRequest();
}
}
Explanation of the Code
- State Interface: This abstracts the behavior for all states.
- Concrete States:
ConcreteStateA
andConcreteStateB
implement specific behaviors. - Context Class: This class holds a reference to a State object and delegates requests to the current state.
This structure allows for clean separation of concerns. Each state can evolve independently of others, promoting easier extension and maintenance of the code.
Example Usage
To utilize our State Pattern implementation, one would use it as follows:
public class Client {
public static void main(String[] args) {
Context context = new Context();
State stateA = new ConcreteStateA();
State stateB = new ConcreteStateB();
context.setState(stateA);
context.request(); // Outputs: Handling request in State A.
context.setState(stateB);
context.request(); // Outputs: Handling request in State B.
}
}
Common Pitfalls of the State Pattern
Despite its advantages, developers often stumble upon various pitfalls when implementing the State Pattern:
1. Complex State Transitions
One of the most significant issues is managing complex state transitions. When the state machine grows, keeping track of valid transitions becomes cumbersome. It can lead to a convoluted design.
Solution: Use a State Diagram
Creating state diagrams helps visualize state transitions clearly. They serve as blueprints, preventing confusion over valid state changes and ensuring that unreachable states are eliminated. Tools like PlantUML are excellent for this purpose.
2. State Classes Explosion
More states often mean more classes, leading to what we call the "class explosion" problem. Too many states can lead to a maintenance nightmare.
Solution: Examine the Need for States
Before adding a new state, reassess if that behavior genuinely deserves a state of its own. Consider whether it could be handled via a strategy pattern or by organizing code differently, perhaps utilizing enums or configuration maps.
3. Context Burden
The context class can become overly complex as it may need to manage an extensive number of states, leading to a violation of the Single Responsibility Principle.
Solution: Delegate Responsibilities Carefully
Make sure the context's responsibilities are limited to managing the state. Any behavior that should be state-specific should remain within individual state classes. This modularity helps maintain cleaner code.
4. Debugging Challenges
Debugging state-specific issues can be tough. If a problem arises in state management, it may take time to trace through state transitions and identify the root cause.
Solution: Implement Logging
Incorporate logging mechanisms that track state transitions and actions. This practice creates a trail that simplifies debugging by providing clear insights into the state history.
5. Overcomplicating the State Management
Sometimes developers overcomplicate the State Pattern, leading to unnecessary abstractions and making the code harder to follow.
Solution: Aim for Simplicity
Implement the State Pattern with simplicity in mind. Avoid excessive abstractions and keep only the necessary functionalities. Regular code reviews can help identify and refactor overcomplicated designs.
Advanced State Pattern Techniques
Having discussed the common pitfalls, let's explore some advanced techniques to enhance the State Pattern further:
State Pattern with Singleton States
If certain states are unique across the application, we can implement them using Singletons. This ensures that there’s only one instance of the state.
class SingletonStateA implements State {
private static SingletonStateA instance = new SingletonStateA();
private SingletonStateA() {}
public static SingletonStateA getInstance() {
return instance;
}
public void handleRequest() {
System.out.println("Handling request in Singleton State A.");
}
}
Combining State and Strategy Patterns
Another advanced technique involves combining the State Pattern with the Strategy Pattern. You can encapsulate varying behaviors, selecting different strategies based on the current state.
interface Strategy {
void execute();
}
class ConcreteStrategyA implements Strategy {
public void execute() {
System.out.println("Executing Strategy A.");
}
}
class ConcreteStrategyB implements Strategy {
public void execute() {
System.out.println("Executing Strategy B.");
}
}
class StateWithStrategy implements State {
private Strategy strategy;
public void setStrategy(Strategy strategy) {
this.strategy = strategy;
}
public void handleRequest() {
if (strategy != null) {
strategy.execute();
}
}
}
Lessons Learned
The State Pattern is a powerful tool that provides scalability and maintainability for object-oriented design. However, to fully realize its benefits, developers must navigate the pitfalls that can arise during implementation.
By visualizing state transitions, managing the complexity of states, and keeping the context appropriate, your application can utilize this design pattern without getting bogged down in challenges. With discernment and best practices, the State Pattern can lead to cleaner, more effective code.
For further reading on the State Pattern and software design principles, consider checking out Refactoring Guru and Martin Fowler's Refactoring.
Implementing these strategies ensures that your usage of the State Pattern is both efficient and clean. So, the next time you design a stateful object, keep these considerations in mind, and avoid those pitfalls!