Resolving Common Issues with PropertyPlaceholderConfigurer in Spring
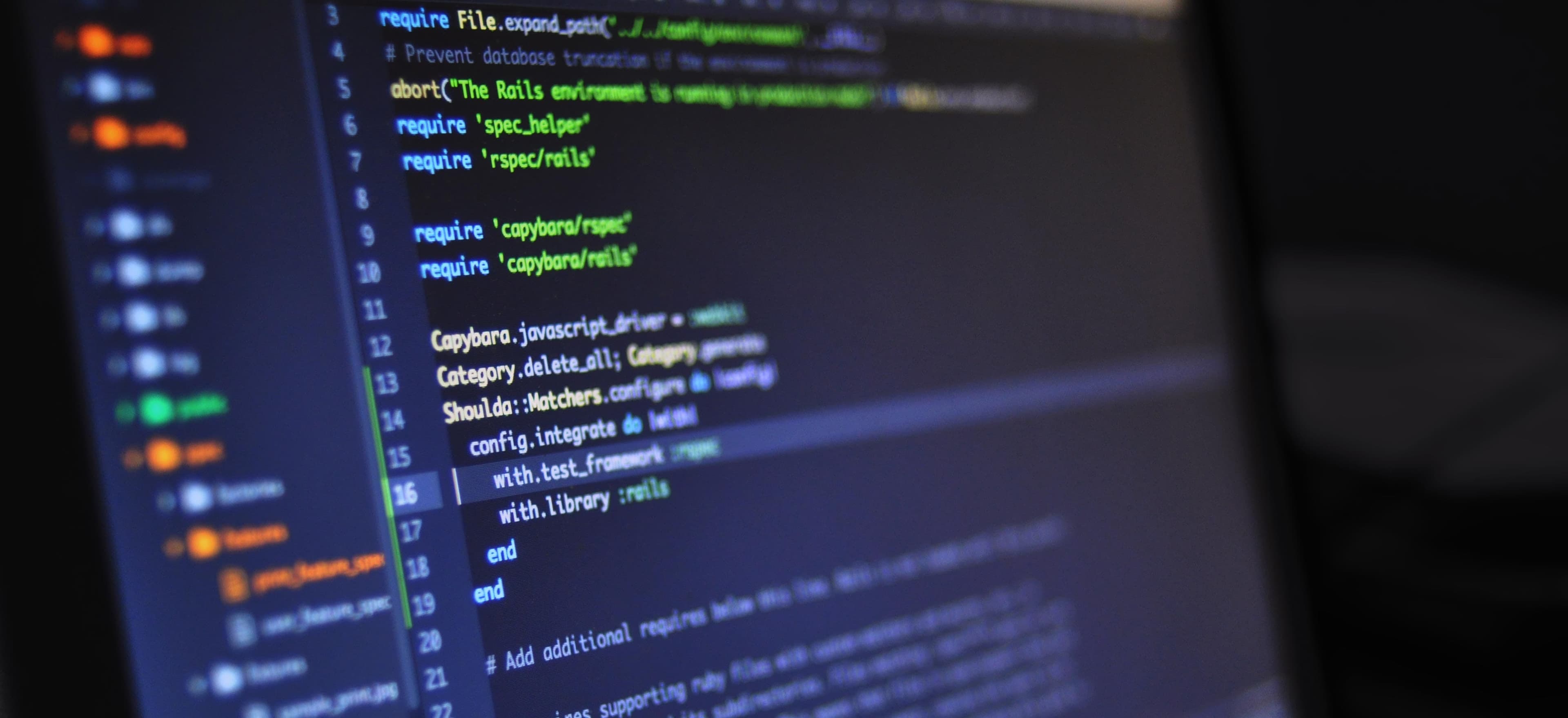
- Published on
Resolving Common Issues with PropertyPlaceholderConfigurer in Spring
Spring Framework, one of the most popular frameworks in Java, is laden with features that make managing application properties a breeze. Among these features, PropertyPlaceholderConfigurer
plays a critical role in externalizing application configuration. However, developers often encounter issues when using the PropertyPlaceholderConfigurer
. This post will explore common problems, solutions, and practical code snippets to guide you toward a seamless integration of property configurations in your Spring application.
Understanding PropertyPlaceholderConfigurer
The PropertyPlaceholderConfigurer
is part of the Spring core framework that helps in externalizing the properties of your application. It allows you to define placeholder values in your Spring configuration files, which can be conveniently populated from a properties file.
Example Properties File
Imagine you have a file named application.properties
:
db.username=root
db.password=secret
db.url=jdbc:mysql://localhost:3306/mydb
XML Configuration
To start using PropertyPlaceholderConfigurer
, configure your Spring XML context file as follows:
<bean class="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer">
<property name="location" value="classpath:application.properties" />
</bean>
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver" />
<property name="url" value="${db.url}" />
<property name="username" value="${db.username}" />
<property name="password" value="${db.password}" />
</bean>
In this configuration, ${db.url}
, ${db.username}
, and ${db.password}
are placeholders that will be replaced with the corresponding values from application.properties
at runtime.
Common Issues and Solutions
While PropertyPlaceholderConfigurer
simplifies property management, developers face several common challenges. Let's delve into these and their solutions.
1. Properties Not Found
One of the most frequent issues is when properties defined in the properties file are not found during runtime.
Solution: Check File Path
Ensure the path to your properties file is correct. Using the classpath:
prefix assumes that the file is located in the src/main/resources
directory of your project. Here’s how you can verify:
<property name="location" value="classpath:application.properties" />
2. Applications Fail to Start
When there’s a misconfiguration in your Spring XML files, your application may fail to start, throwing errors related to bean initialization.
Solution: Debugging Logs
Enable detailed logging in your application to help pin down the issue. Ensure that Spring is set to display configuration errors by adding the following to your logging configuration:
logging.level.org.springframework=DEBUG
You may find helpful messages that indicate precisely where the issue lies.
3. Using Multiple Properties Files
Sometimes, you might want to load additional properties files. Upon doing so, you might find that only one file is taken into account.
Solution: Load Multiple Files
You can separate multiple property files using commas.
<bean class="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer">
<property name="locations">
<list>
<value>classpath:application.properties</value>
<value>classpath:additional.properties</value>
</list>
</property>
</bean>
4. Placeholder Syntax Issues
Spring allows customizing the syntax of property placeholders, but incorrect configuration can lead to unresolved placeholders.
Solution: Use the Correct Syntax
Use the default ${...}
syntax for placeholders. If you've changed placeholder syntax, ensure it is appropriately set in your Spring configuration.
<bean class="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer">
<property name="placeholderPrefix" value="${" />
<property name="placeholderSuffix" value="}" />
</bean>
5. Accessibility of Property Files
If your properties file is loaded correctly but properties are still not accessible, check the file's visibility and read permissions.
Solution: Set Proper Permissions
Ensure that the properties file has the correct permissions so that the application can read it. This is particularly relevant when deploying to certain environments like Docker containers or cloud services.
6. Outdated Properties
If your application isn’t recognizing updates made to the properties files, you might suspect caching.
Solution: Refresh the Context
If you're using PropertyPlaceholderConfigurer
, when your application context is refreshed, it reloads configurations. Ensure you're invoking the context refresh appropriately or consider using @RefreshScope
if you're working with Spring Cloud.
@Configuration
@RefreshScope
public class AppConfig {
// Your beans here
}
Moving Towards Environment-Specific Configurations
With the introduction of Spring Boot, developers have leaned towards @Value
and @ConfigurationProperties
along with PropertySourcesPlaceholderConfigurer
.
Here's a brief example of using @Value
annotation:
@Configuration
public class AppConfig {
@Value("${db.url}")
private String dbUrl;
// Additional configurations
}
Closing the Chapter
Navigating the challenges of PropertyPlaceholderConfigurer
in Spring may appear daunting at first, but with the right approach, it can significantly enhance your application's manageability.
To streamline properties management, ensure correct file paths, undo unnecessary caching, and leverage more modern Spring alternatives like Spring Boot when suitable.
Embrace the power of externalized configuration through properties to produce more resilient and maintainable applications.
For further reading on Spring configuration, check out the official Spring Documentation for deeper insights.
Feel free to reach out if you encounter challenges, or share your experiences while using PropertyPlaceholderConfigurer
in your projects!