Common UI Testing Pitfalls: How to Avoid Them
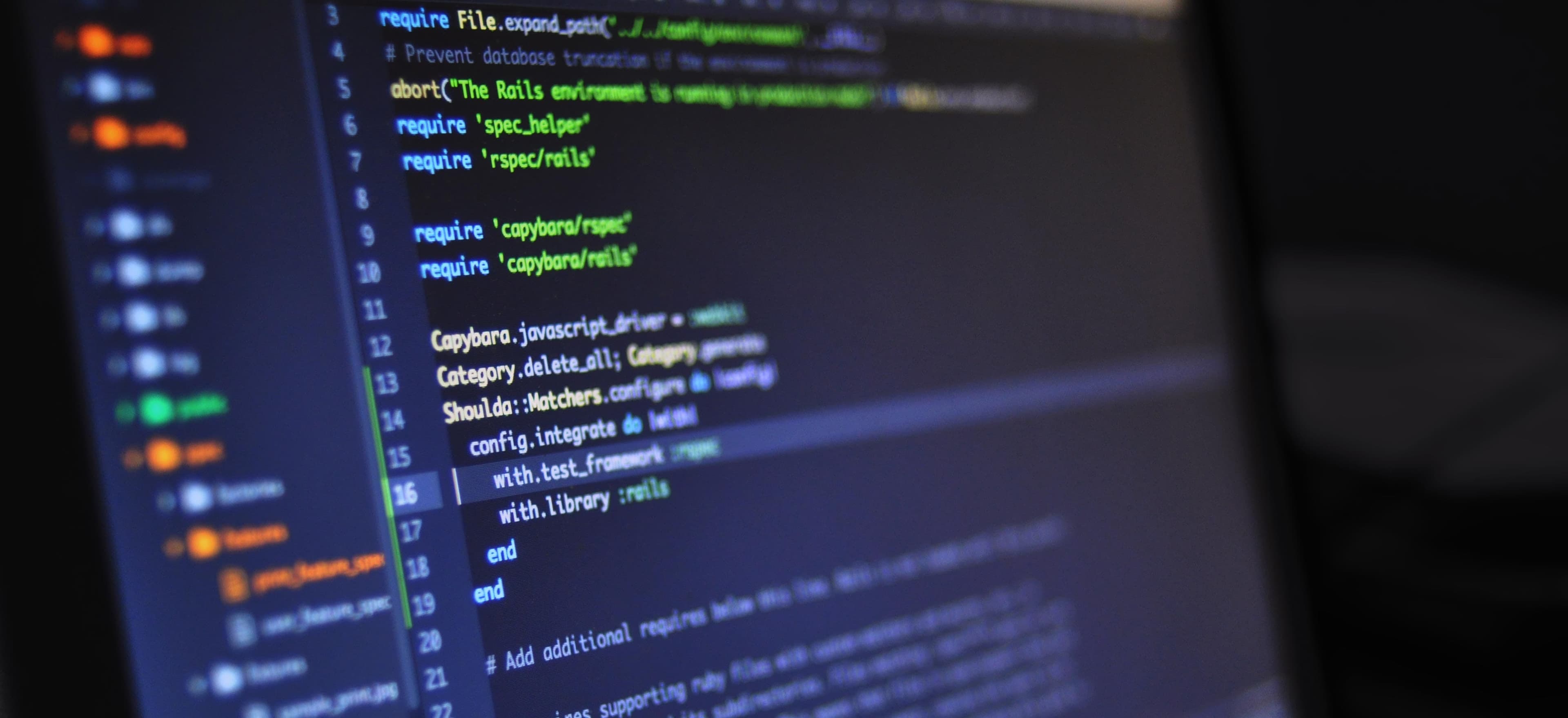
- Published on
Common UI Testing Pitfalls: How to Avoid Them
User Interface (UI) testing is a critical part of maintaining the quality of web applications. It ensures that users have a seamless experience while interacting with your application. However, even seasoned developers often encounter pitfalls that can compromise the efficiency and effectiveness of their UI tests. In this post, we'll explore common mistakes in UI testing and how to avoid them to enhance the quality of your application while saving time and resources.
Table of Contents
- Understanding UI Testing
- Common Pitfalls
- Overlapping UI Tests
- Ignoring Accessibility
- Not Using Automation Wisely
- Insufficient Test Coverage
- Lack of Maintenance
- Best Practices to Avoid Pitfalls
- Conclusion
Understanding UI Testing
UI testing involves testing the graphical user interface of an application to ensure it meets specified standards and provides a good user experience. This form of testing can be manual or automated. Automated UI testing frameworks, such as Selenium or Cypress, allow developers to write scripts that simulate user interactions.
The main goals are:
- Verifying user interfaces behave as expected.
- Ensuring the application meets design specifications.
- Detecting issues that users might encounter.
For a detailed overview of UI testing methodologies, check this guide.
Common Pitfalls
1. Overlapping UI Tests
Description: Overlapping tests can lead to redundancy and longer build times. If multiple tests are validating the same assertion, it wastes resources and can create confusion.
Solution: Maintain a clear test suite strategy. Organize tests so that each one has a distinct purpose. Here's an example of how to structure your test files effectively using Selenium:
public class LoginTest {
@Test
public void testLoginValidCredentials() {
// code for testing valid login
}
}
public class RegistrationTest {
@Test
public void testUserRegistration() {
// code for testing user registration
}
}
Why: By separating concern areas in test classes, you avoid redundancy and make maintenance easier.
2. Ignoring Accessibility
Description: Accessibility is not just a legal requirement; it's crucial for usability. Ignoring it can alienate a significant portion of your audience.
Solution: Always incorporate accessibility checks in your UI tests using tools like Axe or aXe-core. Here’s an example using JavaScript's aXe:
axe.run(document, {}, (err, results) => {
if (err) throw err;
console.log(results.violations);
});
Why: This code runs accessibility checks on the current document and logs any violations found. By addressing these issues, you ensure a more inclusive user experience.
3. Not Using Automation Wisely
Description: Automation can be powerful, but misusing it can lead to fragile tests. Automated tests should not attempt to cover every scenario especially when UI changes are frequent.
Solution: Use automation for repetitive tasks and focus on high-value scenarios. For example, don’t automate every button click; automate critical pathways instead.
// High-value path automated test
@Test
public void testCheckoutProcess() {
driver.get("http://example.com/shop");
driver.findElement(By.id("buy-button")).click();
// Additional checkout steps
}
Why: Automating only the most important paths reduces maintenance overhead and increases reliability in your tests.
4. Insufficient Test Coverage
Description: Not covering edge cases can lead to unexpected errors in production. If your tests only cover common usage scenarios, you run the risk of encountering critical failures in less frequented areas.
Solution: Develop a comprehensive testing strategy that considers normal scenarios, edge cases, and negative tests.
public void testLoginInvalidPassword() {
driver.get("http://example.com/login");
driver.findElement(By.id("username")).sendKeys("user");
driver.findElement(By.id("password")).sendKeys("wrongpassword");
driver.findElement(By.id("login-button")).click();
assertTrue(driver.findElement(By.id("error-message")).isDisplayed());
}
Why: This assert ensures an error message is displayed when invalid credentials are used, simulating real-world usage scenarios that could lead to user frustration.
5. Lack of Maintenance
Description: UI tests can become obsolete quickly as user interfaces evolve. Without regular maintenance, tests can become unreliable and lead to failed deployments.
Solution: Implement a robust test maintenance schedule. Review and update tests regularly—ideally after each release or major UI change.
public void updateLoginTest() {
// Review test case as per new UI updates
}
Why: Regularly updating test cases helps ensure they remain relevant and functional with the latest application changes.
Best Practices to Avoid Pitfalls
- Create a Test Plan: Craft a detailed test plan that outlines your testing objectives, resources needed, and timeline.
- Prioritize Tests: Identify which tests add the most value and prioritize their execution.
- Use Page Object Models: Implement the Page Object Model (POM) design pattern. This helps manage UI changes with less impact on your test logic.
public class LoginPage {
private WebDriver driver;
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void enterUsername(String username) {
driver.findElement(By.id("username")).sendKeys(username);
}
public void enterPassword(String password) {
driver.findElement(By.id("password")).sendKeys(password);
}
// Other page-specific methods
}
Why: POM abstracts page details, making tests easier to read and maintain, especially when the UI changes frequently.
- Use Continuous Integration: Automate your testing by integrating with CI/CD pipelines. This helps catch issues early, reducing the pain of fixing problems later in development.
Wrapping Up
Addressing these common UI testing pitfalls will not only enhance the quality of your application but also improve the efficiency of your development cycles. By being mindful of overlapping tests, accessibility, automation practices, coverage, and maintenance, you can create a robust testing environment that yields reliable results.
Remember, the objective of UI testing is not just to validate the application’s visual appearance but to optimize the entire user experience. For an in-depth look at UI testing tools and methodologies, visit Sauce Labs.
Implement these best practices and watch as your testing strategy turns into an invaluable asset for maintaining your application’s quality and reliability. Happy testing!
Checkout our other articles