Unlock the Hidden Gems: Must-Have GitHub Projects
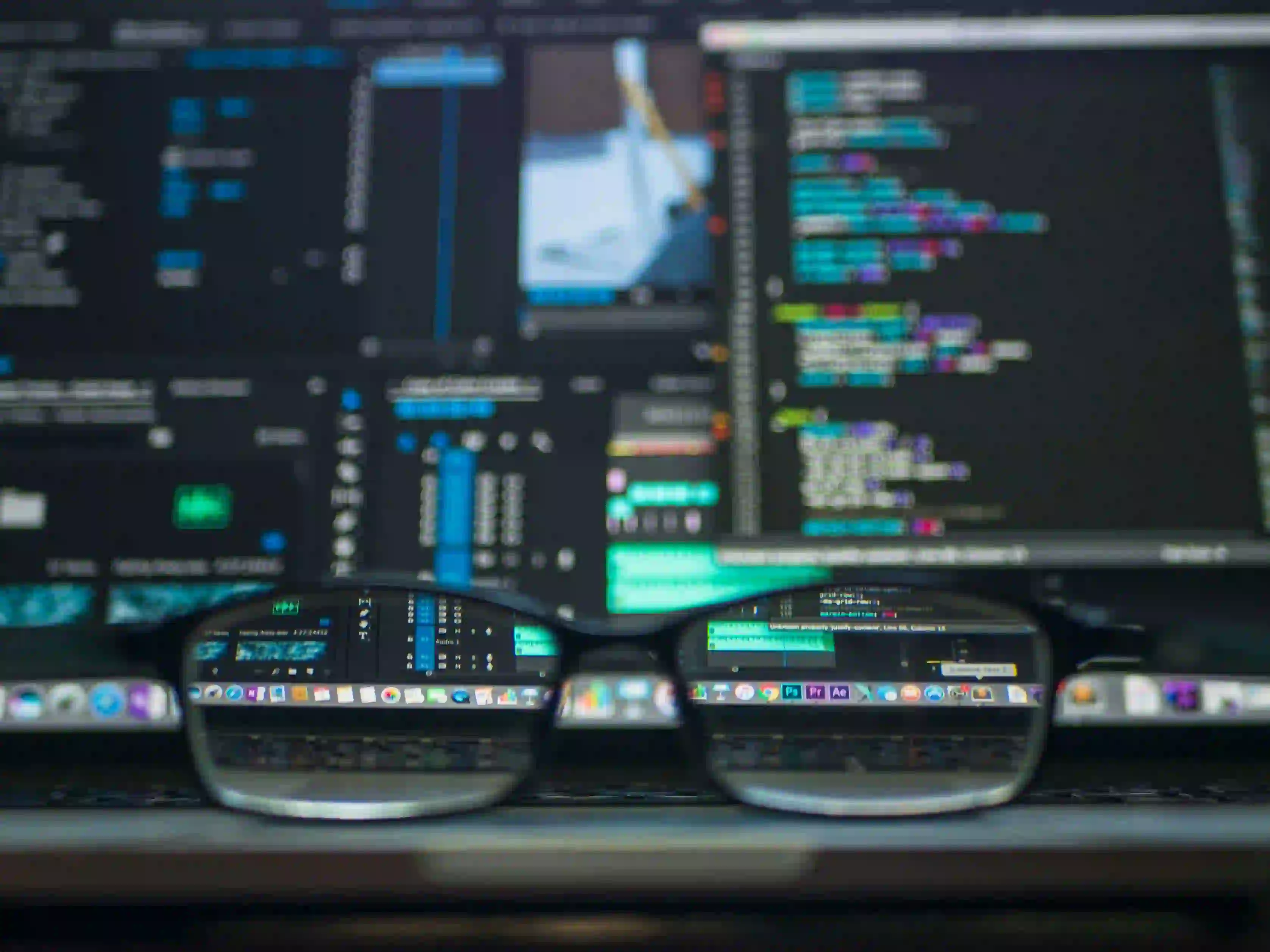
Unlock the Hidden Gems: Must-Have GitHub Projects
GitHub has become a treasure trove for developers and tech enthusiasts alike. With millions of repositories available, it can sometimes feel overwhelming to find the truly innovative projects that stand out among the voluminous list. In this blog post, we'll uncover some hidden gems of GitHub projects that you might not have heard about yet, giving you insights into why they're worth your attention.
What Makes a GitHub Project a Hidden Gem?
When we talk about hidden gems on GitHub, we're referring to projects that:
- Offer Unique Functionality: They solve specific problems that mainstream tools do not.
- Have Active Communities: They are maintained and improved by a community of developers.
- Provide Clear Documentation: They are easily understandable, making it easy for new users and contributors to engage.
- Showcase Innovative Technologies: They utilize or introduce cutting-edge technologies or methodologies.
Let’s dive into the specifics, featuring some noteworthy examples that exemplify these qualities!
1. Deno
What Is Deno?
Developed by Ryan Dahl, the creator of Node.js, Deno is a modern runtime for JavaScript and TypeScript that focuses on security and simplicity. It comes with built-in TypeScript support and an improved module system.
Why Consider Deno?
Deno isn't just another Node.js; it's a response to the shortcomings of Node. Some of its standout features include:
- Secure by Default: Deno restricts file, network, and environment access unless explicitly allowed.
- Native TypeScript Support: Allows for seamless integration and development in TypeScript without requiring any additional configurations.
- Deno CLI: It comes with a powerful command-line interface that simplifies package management and testing.
Code Snippet
Here's a simple HTTP server using Deno:
import { serve } from "https://deno.land/std/http/server.ts";
const server = serve({ port: 8000 });
console.log("http://localhost:8000/");
for await (const request of server) {
request.respond({ body: "Hello World\n" });
}
Why This Code?
This snippet is a superb starting point for understanding Deno's simplicity. You can see how quickly you can spin up an HTTP server while using an official module hosted on Deno's land. This is a great showcase of the community module system and easy-to-read syntax that TypeScript provides.
2. TensorFlow.js
What Is TensorFlow.js?
TensorFlow.js is an open-source library that allows high-performance machine learning in the browser and on Node.js. It allows developers to train and deploy models and get predictions using client-side JavaScript.
Why Use TensorFlow.js?
This project enables developers to integrate machine learning into applications seamlessly. Some benefits include:
- Run ML Models in the Browser: No need to manage back-end servers for ML tasks.
- Hardware Acceleration: Utilizes WebGL for faster computation on devices.
- Interactive Applications: Directly manipulate data and visualize results immediately in the browser.
Code Snippet
Let’s demonstrate how to implement a simple predictive model with TensorFlow.js:
// Import TensorFlow.js
import * as tf from '@tensorflow/tfjs';
// Prepare your data
const xs = tf.tensor2d([1, 2, 3, 4], [4, 1]);
const ys = tf.tensor2d([1, 3, 5, 7], [4, 1]);
// Create a simple linear model
const model = tf.sequential();
model.add(tf.layers.dense({ units: 1, inputShape: [1] }));
// Compile the model
model.compile({ loss: 'meanSquaredError', optimizer: 'sgd' });
// Train the model
await model.fit(xs, ys);
// Make predictions
const output = model.predict(tf.tensor2d([5], [1, 1]));
output.print();
Why This Code?
This JavaScript snippet exemplifies how accessible machine learning can be with TensorFlow.js. You can visualize data, train models, and predict all in the browser, which opens unbounded possibilities for real-time applications.
3. Flutter
What Is Flutter?
Flutter is Google's portable UI toolkit for building beautiful, natively compiled applications for mobile, web, and desktop from a single codebase. It's built on the Dart language and has gained immense popularity among developers.
Why Use Flutter?
The main advantages are:
- Faster Development: Hot Reload allows developers to see changes instantly.
- Unified Codebase: Write once, deploy anywhere.
- Highly Customizable: Offers a rich set of widgets and customizable features.
Code Snippet
Here’s a simple Flutter app that displays Hello World
:
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Hello World App'),
),
body: Center(
child: Text('Hello, World!'),
),
),
);
}
}
Why This Code?
This snippet demonstrates Flutter's straightforward approach to creating cross-platform applications. You can build visually appealing user interfaces with minimal code, showcasing the power of widgets in Flutter.
4. K6
What Is K6?
K6 is an open-source load testing tool. It’s a developer-centric way to test the performance of your system before deploying it, making it invaluable for DevOps teams.
Why Use K6?
Load testing cannot be overlooked. K6 provides:
- Easy to Use: Scripts can be written in JavaScript and are simple to comprehend.
- Automation Capabilities: Integrate easily with CI/CD pipelines.
- Cloud and Local Execution: You can run tests in clusters for scalability.
Code Snippet
Below is an example of a simple K6 test:
import http from 'k6/http';
import { check, sleep } from 'k6';
export default function () {
let res = http.get('https://test.k6.io');
check(res, {
'status is 200': (r) => r.status === 200,
});
sleep(1);
}
Why This Code?
This simple script fetches a webpage and checks if the response status is 200. K6 is excellent for both performance testing and scripting because it allows developers to articulate their tests in a familiar JavaScript environment.
Final Thoughts
Exploring GitHub can help uncover various hidden gems that could enhance your development workflow significantly. Deno, TensorFlow.js, Flutter, and K6 represent only a fraction of the innovative work being done in the developer community.
For further insights, consider browsing through GitHub Trending to see what projects are gaining momentum. Engaging with these projects can widen your skill set and enrich your programming journey.
As you explore these technologies, don’t forget to dive into the documentation and community discussions. There’s always something new to learn, and after all, you never know what hidden gem you might stumble upon next. Happy coding!