Navigating Backward Compatibility Challenges in Continuous Delivery
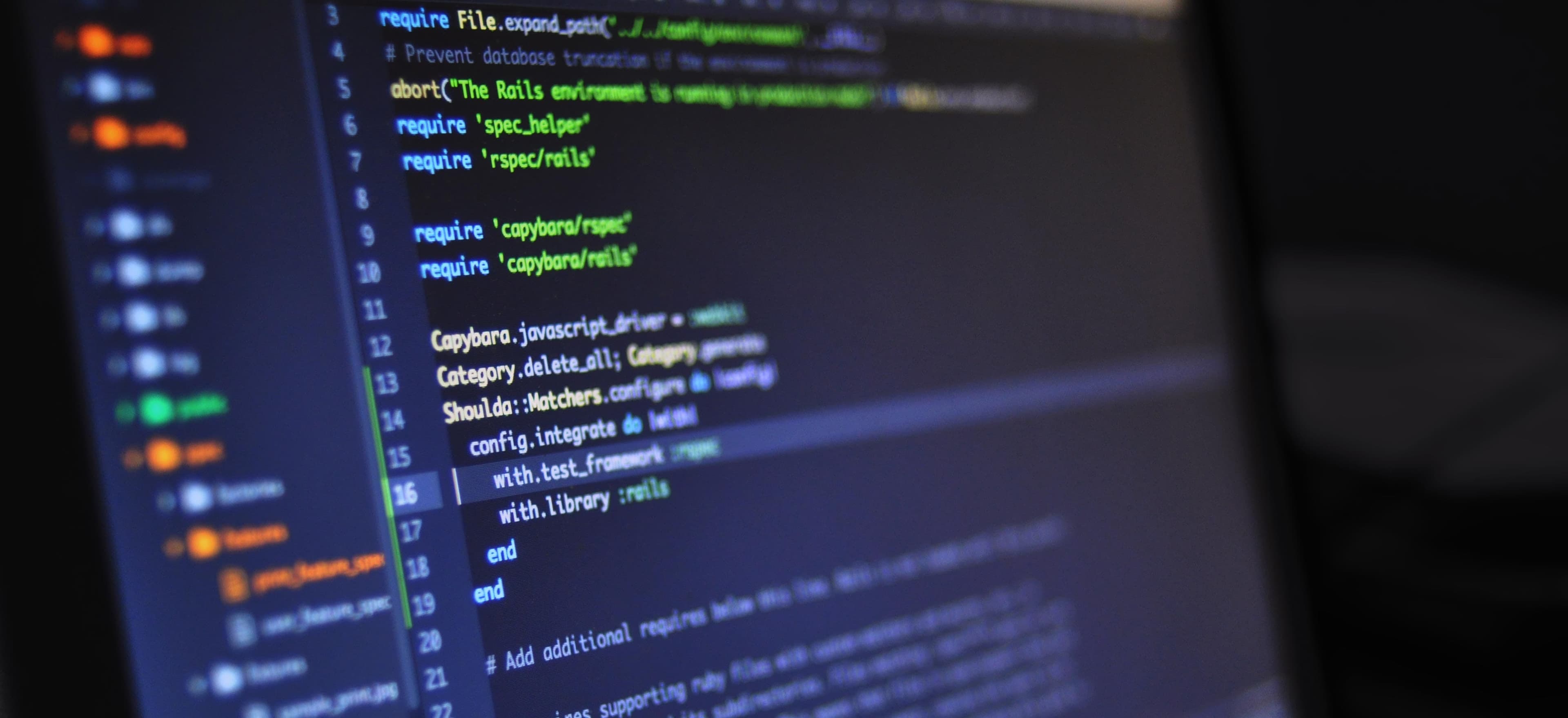
- Published on
Navigating Backward Compatibility Challenges in Continuous Delivery
In the modern software development landscape, Continuous Delivery (CD) has emerged as a pivotal practice that facilitates rapid and reliable software delivery. However, one of the often-overlooked challenges in this environment is maintaining backward compatibility. This issue, if not managed carefully, can lead to significant problems affecting users, stakeholders, and overall system integrity.
In this blog post, we will explore backward compatibility in the context of Continuous Delivery, understand its importance, identify common challenges, and review strategies to navigate these challenges effectively.
What is Backward Compatibility?
Backward compatibility, often referred to as backward compatibility, is the ability of a system to interact with older versions of itself or with other systems that have not yet been updated. This characteristic is essential in ensuring that new updates do not disrupt existing functionalities or customers' experiences.
Why is Backward Compatibility Important?
- User Satisfaction: Users rely on existing features functioning as expected even after updates. Disruptions can lead to frustration and loss of trust.
- Cost Efficiency: Fixing compatibility issues after deploying updates can be more costly than proactively considering them during development.
- Reduced Technical Debt: Addressing backward compatibility can mitigate future complexities while maintaining software.
The Challenges of Backward Compatibility in Continuous Delivery
Continuous Delivery emphasizes speed and agility, which can conflict with the meticulous attention compatibility demands. Here are some common challenges:
-
Frequent Updates: The fast pace of release cycles can lead to breaking changes, impacting users who rely on older versions of the software.
-
Diverse User Environments: Users may run different versions of software, making it challenging to ensure seamless integration across the board.
-
API Changes: Changes to application programming interfaces (APIs) can introduce breakages unless specifically managed.
-
Data Migration Issues: Changes in data structures or formats can lead to complications in accessing or interpreting legacy data.
Strategies for Ensuring Backward Compatibility
1. Semantic Versioning
Semantic versioning (semver) can be an effective way to communicate API changes clearly. Each release is assigned a version number, denoting major, minor, and patch changes. Here’s a simple breakdown:
- Major version: Incremented for incompatible changes.
- Minor version: Incremented for added functionality in a backward-compatible manner.
- Patch version: Incremented for backward-compatible bug fixes.
By following these conventions, developers and users can anticipate the implications of updates.
Example:
public class VersionHandler {
private int majorVersion;
private int minorVersion;
private int patchVersion;
public VersionHandler(int major, int minor, int patch) {
this.majorVersion = major;
this.minorVersion = minor;
this.patchVersion = patch;
}
public String getVersion() {
return majorVersion + "." + minorVersion + "." + patchVersion;
}
}
This class provides a straightforward way to manage versions, ensuring clarity and potentially mitigating confusion during upgrades.
2. Deprecation Policies
When a feature or API is no longer considered optimal, a well-thought-out deprecation policy is critical. By marking functionalities as deprecated rather than removing them entirely, software teams can warn users about upcoming changes without immediate disruption.
Example:
@Deprecated
public void oldMethod() {
// This method might be removed in future versions
System.out.println("This method is deprecated. Use newMethod() instead.");
}
public void newMethod() {
System.out.println("This is the new method to use.");
}
In this example, the oldMethod
is marked as deprecated, indicating to users that there's a new alternative without abruptly breaking existing client code.
3. Feature Toggles
Feature toggles allow developers to switch functionalities on or off without deploying new code. This creates opportunities to test new features incrementally.
Example:
public class FeatureToggle {
private boolean newFeatureEnabled;
public FeatureToggle(boolean isEnabled) {
this.newFeatureEnabled = isEnabled;
}
public void execute() {
if (newFeatureEnabled) {
newFeature();
} else {
oldFeature();
}
}
private void newFeature() {
System.out.println("Executing new feature.");
}
private void oldFeature() {
System.out.println("Executing old feature.");
}
}
This pattern allows for safe deployment while gradually introducing changes. Users can continue using the old method while new features are tested.
4. Automated Testing
A robust suite of automated tests is indispensable in Continuous Delivery. These tests can ensure that new measures do not disrupt existing functionalities.
Unit Testing Example:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class VersionHandlerTest {
@Test
public void testGetVersion() {
VersionHandler version = new VersionHandler(2, 1, 3);
assertEquals("2.1.3", version.getVersion());
}
}
In this example, unit tests assure that the code has not broken expected functionality. This is particularly useful for testing edge cases related to backward compatibility.
5. Communicating Changes Clearly
Clear communication about what to expect from updates is crucial. Update notes, customer emails, or documentation can help guide users through changes. A notification system within the software can also provide real-time updates when significant changes occur.
6. Use of Shims and Adapters
When introducing breaking changes, shims or adapters can help bridge old and new behaviors. This tactic offers a temporary solution while users transition.
Example:
public interface LegacyInterface {
void legacyMethod();
}
public class LegacyAdapter implements LegacyInterface {
public void legacyMethod() {
System.out.println("Adapting legacy method.");
}
}
public class NewSystem {
public void newMethod() {
LegacyInterface legacy = new LegacyAdapter();
legacy.legacyMethod();
}
}
This approach allows new systems to interact with outdated interfaces, providing continued functionality while newer implementations are phased in.
Closing Remarks
Navigating backward compatibility challenges in Continuous Delivery is both an art and a science. As the pace of deployment accelerates, ensuring older versions seamlessly coexist with newer functionalities becomes crucial.
By employing strategies such as semantic versioning, deprecation policies, feature toggles, automated testing, clear communication, and the use of shims, teams can create a more cohesive development environment.
Ultimately, maintaining backward compatibility fosters user trust and satisfaction, leading to a more robust and resilient software lifecycle. As developers and organizations adopt these practices, they can mitigate risks and harness the full potential of Continuous Delivery while ensuring legacy systems still operate effectively.
For further reading on Continuous Delivery concepts, you may find the following resources helpful:
- Continuous Delivery by Jez Humble and David Farley
- The Twelve-Factor App
By understanding and addressing backward compatibility, your team can advance confidently in the realm of software development. Happy coding!
Checkout our other articles