Mastering Secure Data Retrieval: Overcoming Encryption Hurdles
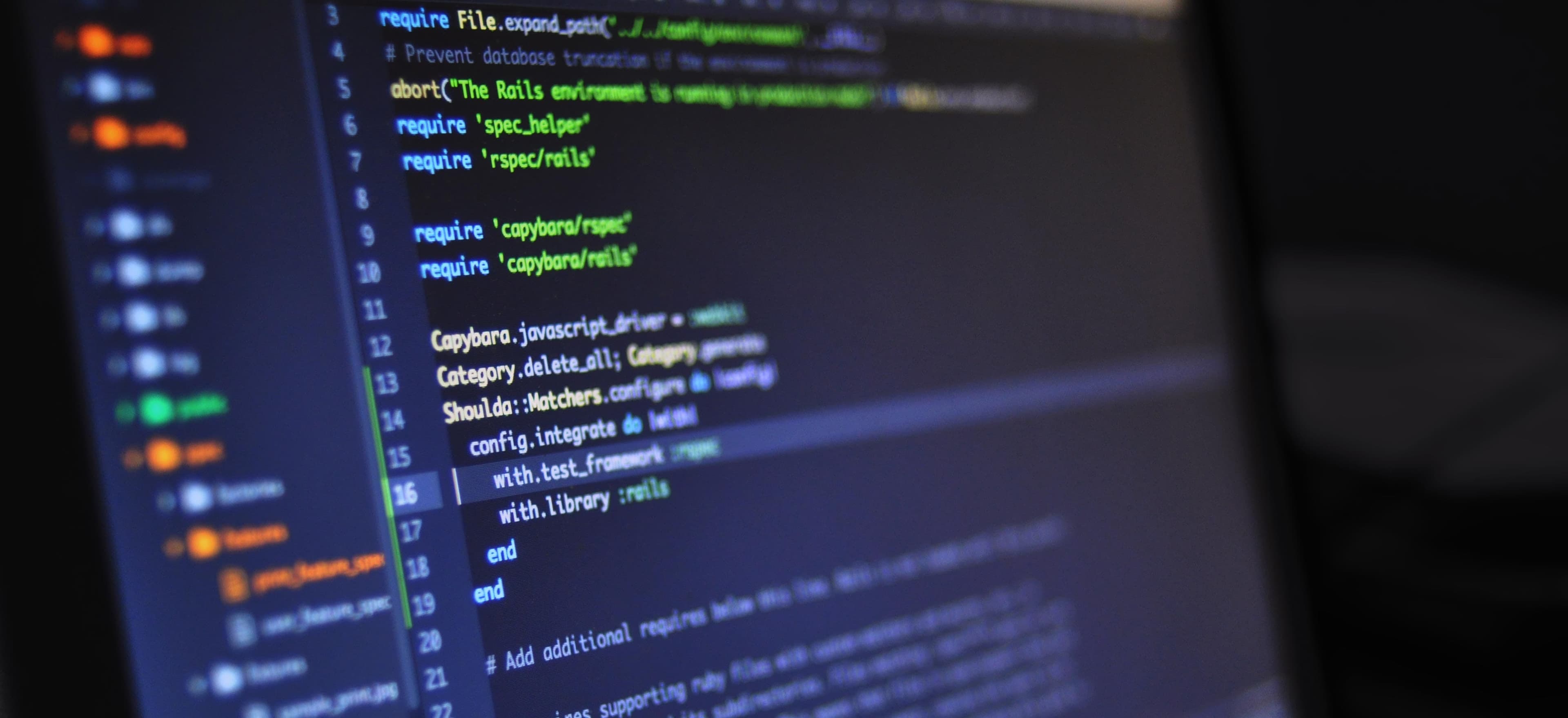
- Published on
Mastering Secure Data Retrieval: Overcoming Encryption Hurdles
In today's digital landscape, ensuring the privacy and security of data is paramount. With the increase in data breaches and privacy concerns, encryption has become a frontline defense against unauthorized access. However, while encryption provides robust security, it can also pose challenges in data retrieval. In this blog post, we will explore these challenges and provide insights on how to overcome them through effective design and implementation strategies.
Understanding Encryption Basics
Before we dive deep into securing data retrieval, let’s briefly cover what encryption is. Encryption is the process of converting readable data into an encoded format that can only be decoded by someone with the correct decryption key. This transforms sensitive information into a format that is unreadable to unauthorized users.
Common Encryption Techniques
-
Symmetric Encryption: This method uses a single key for both encryption and decryption. It is efficient for large data sets but poses risks if the key is compromised. An example of symmetric encryption is the Advanced Encryption Standard (AES).
-
Asymmetric Encryption: This employs a pair of keys – a public key for encryption and a private key for decryption. RSA (Rivest-Shamir-Adleman) is a widely used asymmetric encryption technique. The challenge here is that it tends to be slower and is typically used to encrypt small amounts of data, like session keys.
Why Is Secure Data Retrieval Crucial?
The primary goal of secure data retrieval is to ensure that only authorized users can access sensitive information. Particularly in industries like finance, healthcare, and government services, secure access is not just a best practice; it is often a regulatory requirement. This necessity leads to three key aspects:
-
Protection Against Unauthorized Access: Sensitive data, if left unprotected, can lead to identity theft and financial loss.
-
Maintaining Data Integrity: Being able to access unaltered data is crucial for decision-making. Encryption helps maintain the integrity of data through hashing.
-
Regulatory Compliance: Many regulations, such as GDPR and HIPAA, mandate strict protocols for data protection, including encryption.
Challenges in Encrypted Data Retrieval
While encryption is effective in securing data, it creates hurdles in retrieval. Let’s explore some common challenges and potential solutions:
1. Key Management
Challenge: Managing cryptographic keys securely is no small feat. If someone unauthorized gains access to the keys, the entire system is compromised.
Solution: Use a dedicated key management solution (KMS). A KMS enables organizations to create, manage, and revoke keys securely. Using cloud providers like AWS KMS can allow for better scalability and control.
Here’s a simple code snippet demonstrating how to use AWS KMS with Java:
import com.amazonaws.services.kms.AWSKMS;
import com.amazonaws.services.kms.AWSKMSClientBuilder;
import com.amazonaws.services.kms.model.DecryptRequest;
import com.amazonaws.services.kms.model.DecryptResult;
import java.nio.ByteBuffer;
public class KMSDecrypt {
public static byte[] decryptData(String keyId, ByteBuffer cipherText) {
AWSKMS kmsClient = AWSKMSClientBuilder.defaultClient();
// Decrypt the data
DecryptRequest decryptRequest = new DecryptRequest()
.withCiphertextBlob(cipherText);
DecryptResult decryptResult = kmsClient.decrypt(decryptRequest);
return decryptResult.getPlaintext().array(); // Return the decrypted data
}
}
Commentary: This snippet demonstrates how to utilize AWS KMS to decrypt encrypted data securely. By encapsulating the decryption logic here, we ensure that the key management is handled by a specialized service, minimizing risks.
2. Performance Overhead
Challenge: Encryption can slow down data retrieval processes, especially for large datasets or real-time applications.
Solution: Use appropriate algorithms and optimize for performance. In scenarios where performance is critical, consider using encryption-at-rest along with encryption-in-transit selectively based on data sensitivity.
3. Access Control
Challenge: Implementing effective access control is necessary to ensure only authorized users can decrypt the data.
Solution: Adopt a role-based access control (RBAC) model. This ensures that permissions are granted based on user roles rather than individual user accounts, simplifying management.
This model can be implemented easily in Java using the Spring Security framework:
import org.springframework.security.access.prepost.PreAuthorize;
public class DocumentService {
@PreAuthorize("hasRole('ADMIN')")
public Document getSensitiveDocument(Long documentId) {
// Retrieve and return the sensitive document
return findDocumentById(documentId);
}
}
Commentary: The @PreAuthorize
annotation ensures that only users with the 'ADMIN' role can access sensitive documents, effectively securing the data retrieval process.
4. Data Loss or Corruption
Challenge: If data gets corrupted or lost while encrypted, the ability to retrieve that information becomes critical.
Solution: Implement regular backup processes and consider using redundancy in encryption keys. Use hashing algorithms like SHA-256 to verify data integrity when retrieving the data.
Here’s an example of how you might implement a hash check in Java:
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class HashUtil {
public static String generateHash(String data) throws NoSuchAlgorithmException {
MessageDigest digest = MessageDigest.getInstance("SHA-256");
byte[] hash = digest.digest(data.getBytes());
StringBuilder hexString = new StringBuilder();
for (byte b : hash) {
String hex = Integer.toHexString(0xff & b);
if(hex.length() == 1) hexString.append('0');
hexString.append(hex);
}
return hexString.toString();
}
}
Commentary: This code snippet provides a way to generate a hash for your data, ensuring that you can verify the integrity of that data during retrieval.
5. User Experience
Challenge: Secure measures often complicate the user experience, leading to frustration and, in some cases, improper workarounds.
Solution: Strive for a balance between security and usability. Implement single sign-on (SSO) solutions to simplify access without compromising security.
To Wrap Things Up
Secure data retrieval requires careful consideration of encryption strategies and methodologies. By understanding common challenges, you can implement robust solutions that not only protect sensitive information but also enhance user experience.
Effective key management, performance optimization, strict access controls, data integrity checks, and a focus on usability will lead to a secure and efficient data retrieval process in your applications.
For further reading on encryption and data security, consider exploring AWS's whitepapers on security best practices or attain knowledge from the OWASP guidelines on securing application environments.
Mastering secure data retrieval is not just about encryption itself; it is about building a secure architecture that supports business goals while ensuring comprehensive safety for sensitive data.
By following these principles and utilizing the provided code examples, you can navigate the intricate landscape of secure data retrieval while overcoming common encryption hurdles effectively.
Checkout our other articles