Balancing Autonomy and Authority in Event-Driven Microservices
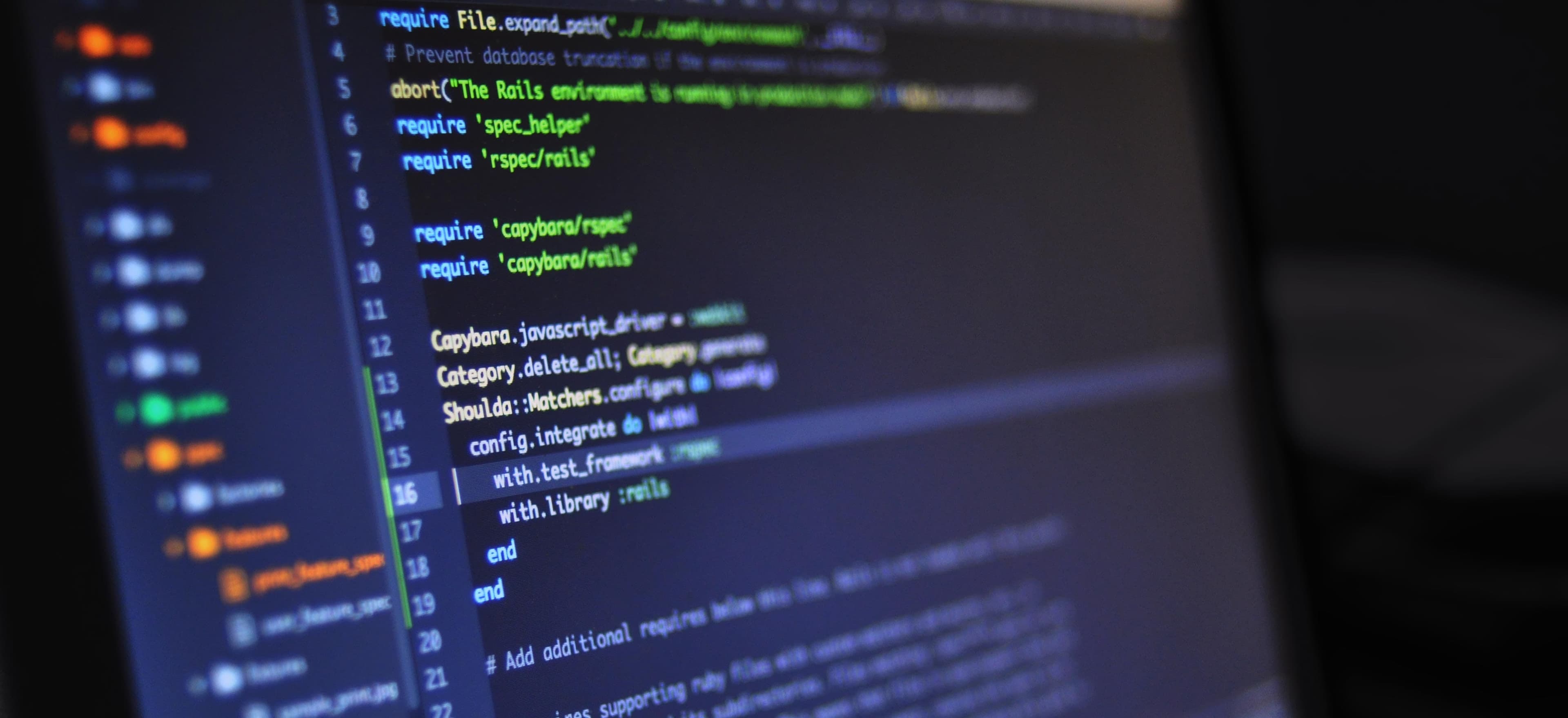
- Published on
Balancing Autonomy and Authority in Event-Driven Microservices
In today's software architecture landscape, microservices have become synonymous with agility, scalability, and maintainability. However, with the promise of better separation of concerns comes the challenge of balancing autonomy and authority. This balance is particularly crucial in event-driven microservices where services communicate asynchronously through events.
In this blog post, we will explore the concepts of autonomy and authority within event-driven microservices and offer practical code snippets to illustrate key points. Our goal is to equip you with an understanding of how to manage these aspects effectively to create robust systems.
Understanding Autonomy and Authority
Autonomy
Autonomy in microservices refers to the ability of each service to operate independently. With autonomy, a service can be developed, deployed, and scaled without needing to synchronize with other services. This independence encourages faster development cycles and reduces the risk of cascading failures in a monolithic architecture.
Authority
Authority, on the other hand, deals with decision-making power. It defines which service is the source of truth for a particular aspect of the application's functionality. When it comes to event-driven architectures, having clearly defined authority is essential to prevent data inconsistencies, particularly when multiple services listen to the same events.
The Challenge
The challenge lies in finding a middle ground. If a microservice is too autonomous, it might lead to data inconsistency and logic fragmentation. Excessive authority, however, can stifle the benefits of microservices by creating bottlenecks and eliminating flexibility.
To illustrate, let’s consider a simple scenario involving Order
and Inventory
microservices in an online shopping platform.
An Example Scenario
In our example, the Order
service handles order placement, while the Inventory
service tracks product stock levels. When an order is placed, the Order
service must inform the Inventory
service to update the stock.
Event-Driven Communication
The communication takes place through events. When an order is created, the Order
service publishes an event:
// OrderService.java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.kafka.core.KafkaTemplate;
import org.springframework.stereotype.Service;
@Service
public class OrderService {
@Autowired
private KafkaTemplate<String, OrderEvent> kafkaTemplate;
public void createOrder(Order order) {
// Logic to save the order
OrderEvent orderEvent = new OrderEvent(order.getId(), order.getProductId(), order.getQuantity());
kafkaTemplate.send("order-topic", orderEvent);
}
}
Why This Works:
In this snippet, the createOrder
method saves the order and then publishes an OrderEvent
to a Kafka topic. This decouples the Order
and Inventory
services, allowing them to evolve independently.
Listening to Events
The Inventory
service listens for these events to adjust stock levels:
// InventoryService.java
import org.springframework.kafka.annotation.KafkaListener;
import org.springframework.stereotype.Service;
@Service
public class InventoryService {
@KafkaListener(topics = "order-topic", groupId = "inventory")
public void consumeOrderEvent(OrderEvent orderEvent) {
// Logic to update inventory based on orderEvent
updateInventory(orderEvent);
}
public void updateInventory(OrderEvent orderEvent) {
// Update inventory logic here
System.out.println("Updating inventory for product ID: " + orderEvent.getProductId());
}
}
Why This Works:
Here, the InventoryService
listens to the order-topic
. When a message arrives, the service executes updateInventory
, effectively maintaining an up-to-date stock count without any direct dependency on the Order
service.
Balancing Autonomy and Authority
To achieve the right balance between autonomy and authority:
-
Define Clear Ownership: Designate which service owns which domain and ensure that it is the single source of truth for that domain. For orders, it should be the
Order
service; for inventory, theInventory
service. -
Decouple Services: Use events to decouple services. This promotes autonomy, allowing services to become independent and resilient against failures in other services.
-
Establish Consistency: Manage eventual consistency through events. Services can still operate independently but must implement strategies to handle data synchronizations, such as listening to relevant events.
-
Versioning Events: As your services evolve, the structures of events may change. Implement versioning for your events to manage breaking changes effectively without disrupting dependent services.
-
Monitoring and Observability: Use monitoring tools to track service interactions and identify potential issues in real-time. This aids in understanding the authority each service holds over its domain.
Event Schema Evolution
Schema evolution can be a challenge when maintaining the authority of events. Let's take a look at how we can manage this using versioning:
// OrderEvent.java
public class OrderEvent {
private String orderId;
private String productId;
private int quantity;
private String version; // New field for versioning
// Getters and setters
}
Why This Matters:
By incorporating a version field, you can handle multiple versions of the OrderEvent
. This allows services to gracefully transition while minimizing disruptions to ongoing operations.
To Wrap Things Up
Balancing autonomy and authority in event-driven microservices is an essential aspect of modern software architecture. By defining clear ownership, decoupling services, establishing consistency, utilizing event versioning, and ensuring observability, we can create a resilient microservices ecosystem.
Additional Resources
- For more insights on microservices architecture, check out Microservices.io.
- Learn more about event-driven systems with Kafka's documentation.
Balancing these two elements will not only enhance the robustness of your system but also align teams around shared goals, giving you the agility and control necessary in today's competitive environment. By leveraging these strategies, you can build scalable and maintainable microservices that satisfy both business and technical requirements.
Checkout our other articles