Bridging the Gap: Java Objects Communicating with iOS
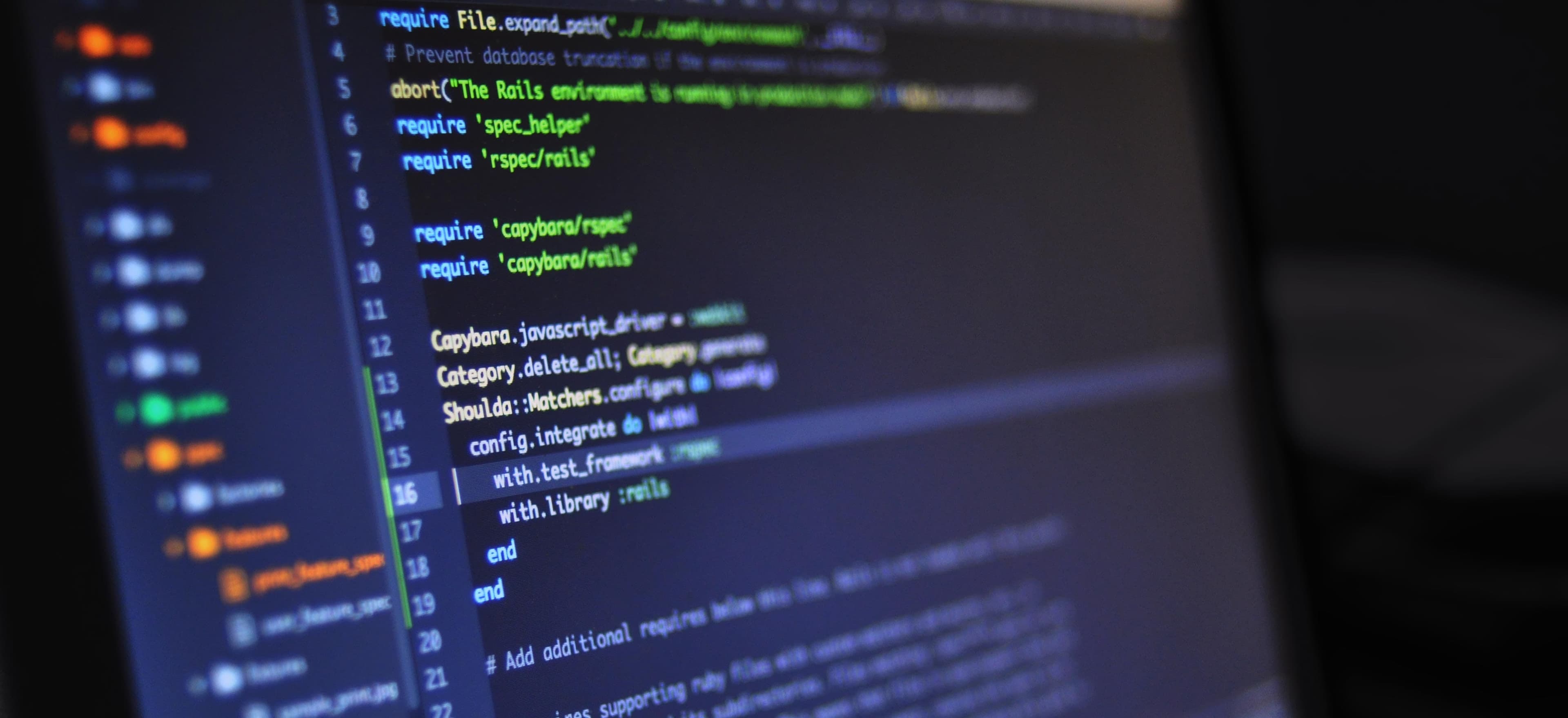
- Published on
Bridging the Gap: Java Objects Communicating with iOS
In today's world, where software solutions often rely on cross-platform interoperability, understanding how different programming environments can communicate is critical. Java and iOS are two prevalent platforms, serving diverse application needs. Bridging the gap between Java objects and iOS is a challenge that comes with immense opportunities. In this blog post, we’ll explore how to enable communication between Java and iOS, focusing on practical implementation, relevant code snippets, and best practices.
Understanding the Need for Communication
The necessity of enabling communication between Java and iOS stems from various scenarios. Java is often used for server-side applications, and iOS for client-side mobile applications. Suppose your Java application serves data via a RESTful API. In that case, you might want to access this data on an iOS app. This bridging allows developers to leverage the strengths of both platforms while providing a seamless user experience.
Key Concepts to Know
Before we dive into implementation, let’s establish some concepts that will help along the way:
-
RESTful APIs: APIs are crucial for interoperability. A RESTful API uses HTTP requests to access and manipulate data. This means you can easily send and receive data between a Java server and an iOS client.
-
JSON: JavaScript Object Notation (JSON) is the primary format used to exchange data between a Java backend and an iOS frontend. JSON is lightweight and easy to parse, making it ideal for mobile applications.
-
Java Virtual Machine (JVM): Java runs on the JVM, which can interact with different environments. Java, being platform-independent, helps in keeping your server logic separate from client-side applications.
Establishing a RESTful API in Java
Let's create a simple RESTful API in Java using Spring Boot, a popular framework. To set up a Spring Boot application:
-
Create a new project: You can use Spring Initializr to generate your project by selecting the required dependencies like "Spring Web" and "Spring Boot DevTools".
-
Define a model: Create a simple Java model that we want to expose via the API.
// Model class public class User { private Long id; private String name; // Constructors, getters, and setters public User(Long id, String name) { this.id = id; this.name = name; } public Long getId() { return id; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
Here, we’ve created a
User
class with anid
andname
. Each field is declared along with its respective getter and setter methods. This class will represent our data model. -
Create a REST Controller: Now, let’s create a controller that manages our API endpoints.
import org.springframework.web.bind.annotation.*; import java.util.ArrayList; import java.util.List; @RestController @RequestMapping("/api/users") public class UserController { private List<User> users = new ArrayList<>(); @PostMapping public User createUser(@RequestBody User user) { users.add(user); return user; } @GetMapping public List<User> getUsers() { return users; } }
Explanation of the Code:
- The
@RestController
annotation indicates that this class will handle HTTP requests. @RequestMapping("/api/users")
specifies that any request to/api/users
will be handled by this controller.- The
createUser
method handles POST requests to create a new user, whilegetUsers
handles GET requests to retrieve the list of users.
- The
Testing the REST API
To test our REST API, you can use tools like Postman or cURL. Here's a quick command using cURL:
curl -X POST -H "Content-Type: application/json" -d '{"id":1, "name":"John Doe"}' http://localhost:8080/api/users
Setting Up Swift for iOS
Now that we have a RESTful API ready, let's set up an iOS application to communicate with this API using Swift, Apple's programming language.
-
Create a new iOS project in Xcode and select the Single View App template.
-
Make an API Call: You can utilize
URLSession
to make network requests in Swift.import UIKit struct User: Codable { let id: Int let name: String } class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() fetchUsers() } func fetchUsers() { guard let url = URL(string: "http://localhost:8080/api/users") else { return } let task = URLSession.shared.dataTask(with: url) { (data, response, error) in guard let data = data, error == nil else { return } do { let users = try JSONDecoder().decode([User].self, from: data) print(users) } catch { print(error.localizedDescription) } } task.resume() } }
Explanation of the Code:
- The
User
struct conforms to theCodable
protocol, which lets us easily serialize and deserialize JSON data. - The
fetchUsers
method creates a data task that retrieves user data from our Java API, decodes it, and prints it to the console.
- The
Handling Cross-Origin Resource Sharing (CORS)
When interacting with the REST API from a different domain, you may encounter CORS issues. To resolve this in Spring Boot, you can add a CORS configuration:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**").allowedOrigins("http://localhost:3000");
}
}
In this example, we've allowed requests from http://localhost:3000
, which is where your iOS simulator or device would make requests when running the app.
Final Thoughts
The integration between Java objects and iOS applications opens avenues for building robust applications that leverage the strengths of both environments. By following the steps outlined in this article, you can set up a seamless communication channel between your Java backend and your iOS client app.
For more detailed insights into RESTful services with Java, you may find this definitive guide on REST APIs helpful.
As you venture into cross-platform development, remember that mastering APIs is crucial in bridging the gap between diverse systems. Happy coding!
Checkout our other articles