How to Resolve Build Dependency Issues in TeamCity
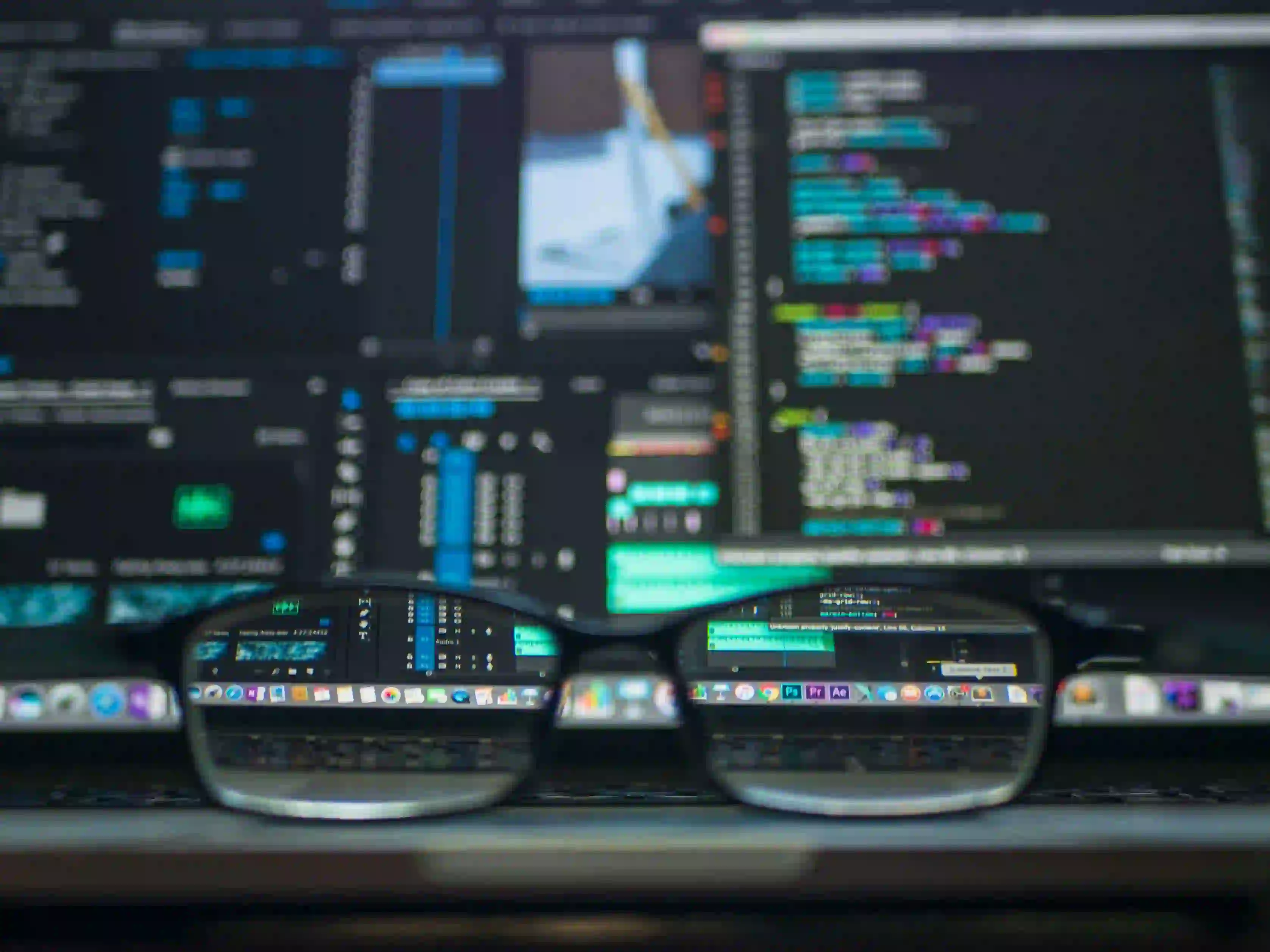
How to Resolve Build Dependency Issues in TeamCity
TeamCity is a powerful continuous integration (CI) and continuous deployment (CD) server used by many teams to keep their software projects on track. However, like any robust CI tool, users may encounter build dependency issues that can be challenging to resolve. In this blog post, we will explore common build dependency issues within TeamCity and provide actionable solutions to tackle them.
Understanding Build Dependencies
Before diving into solutions, let's clarify what build dependencies are. In the context of CI/CD, dependencies are components or libraries your code relies upon to build and run successfully. When deploying applications across multiple stages, it’s critical to manage these dependencies effectively. Common issues arise when dependencies are not resolved properly, leading to build failures or inconsistencies between builds.
Common Dependency Issues in TeamCity
- Unresolved Artifacts: Artifacts generated from previous builds may not be accessible for downstream builds.
- Version Conflicts: Different build configurations may utilize different versions of the same dependency, leading to unexpected behaviors.
- Broken Build Chains: If one build fails, it can break the chain and affect other builds that depend on its output.
Let’s discuss how to diagnose and resolve these issues effectively.
1. Diagnosing Dependency Issues
Before resolving issues, it's important to diagnose their root causes. TeamCity provides extensive logging and build history features. Follow these steps:
- Check the Build Log: Look for error messages related to dependency resolution.
- Inspect Artifact Dependencies: Ensure that all necessary artifacts are produced by upstream builds.
- Version Control: Review the
pom.xml
(for Maven projects) orbuild.gradle
(for Gradle projects) files, especially if version conflicts are suspected.
2. Resolving Unresolved Artifacts
Artifacts can go missing due to several reasons. For example, if a build configuration fails before producing its expected artifacts, downstream builds will fail due to missing dependencies.
Solution
Ensure that the upstream build is complete and produces the required artifacts. You can do this by setting proper triggers and dependencies. Let's look at configuring artifact dependencies in a build:
# Example of setting artifact dependencies in TeamCity
buildType {
id("Build_Configuration")
artifacts {
artifactRules = "output/* => output"
}
}
Here, artifactRules
specifies that all files in the output
folder of the build will be collected as artifacts. Be sure to correctly set this up in your TeamCity build configuration to avoid missing artifacts.
Tip
To ensure artifacts are available, review the artifact paths and make sure they match between builds.
3. Handling Version Conflicts
Version conflicts often occur when your project has dependencies that rely on different versions of the same library or framework.
Solution
- Dependency Management: Use dependency management tools integrated into your build systems, like Maven and Gradle, to enforce versioning. Here’s how to exclude a transitive dependency in Maven:
<dependency>
<groupId>com.example</groupId>
<artifactId>example-library</artifactId>
<version>1.0.0</version>
<exclusions>
<exclusion>
<groupId>org.conflict</groupId>
<artifactId>conflicting-library</artifactId>
</exclusion>
</exclusions>
</dependency>
By excluding the conflicting library, you can avoid build errors and ensure compatibility.
- Consistent Versioning: Adopt a versioning strategy (e.g., semantic versioning) to avoid conflicts. Only update dependencies when necessary, and monitor your build configurations for any discrepancies.
4. Fixing Broken Build Chains
In CI/CD, build chains represent dependencies between multiple builds. A failure in one build can cascade through dependent builds.
Solution
- Fail Fast: Use “fail fast” policies to avoid unnecessary resource consumption. For instance, configure build triggers to only run if their upstream dependencies are successfully built.
# Example of fail fast in TeamCity
buildTrigger {
id("Trigger_Configuration")
triggerRules = "+:default:/:+:test:/:+:build:/:"
}
This configuration will prevent builds from running if their dependent builds fail.
- Build Retry: Implement retry logic for intermittent failures. Here’s how to configure a build retry policy:
failures {
retry {
maxRetries = 3
}
}
This configuration will automatically retry the build up to three times before failing completely.
5. Utilizing TeamCity Features
TeamCity comes with numerous built-in features to manage dependencies:
- Build Chains: Create build chains to visualize dependency sequences. This helps in understanding which builds rely on one another.
- Snapshot Dependencies: Use snapshot dependencies to build a particular version of an artifact. This ensures that the correct version is used in subsequent builds.
addSnapshotDependency("Build_Configuration")
This command would ensure that the latest successful build of "Build_Configuration" is used.
- Artifact Dependencies: Make use of artifact dependencies for sharing output between builds in the same build configuration.
6. Tips for Best Practices
- Environment Consistency: Ensure that your builds run in a consistent environment, ideally using docker containers or virtual environments.
- Regular Cleanup: Periodically clean up unused or outdated build artifacts and dependencies to keep your builds lean and efficient.
- Documentation: Always document your dependency management strategy, including how to add, update, or remove dependencies.
In Conclusion, Here is What Matters
Resolving build dependency issues in TeamCity is critical for maintaining a smooth development cycle. By understanding the root causes and employing effective practices, you can enhance your build reliability.
For additional information on setting up build configurations, check out the TeamCity Documentation.
By adopting best practices and leveraging TeamCity’s features, you will minimize dependency-related disruptions and maintain a stable CI/CD pipeline.
Happy building!