Boost Your Agile Workflow: Effective Regression Testing Tips
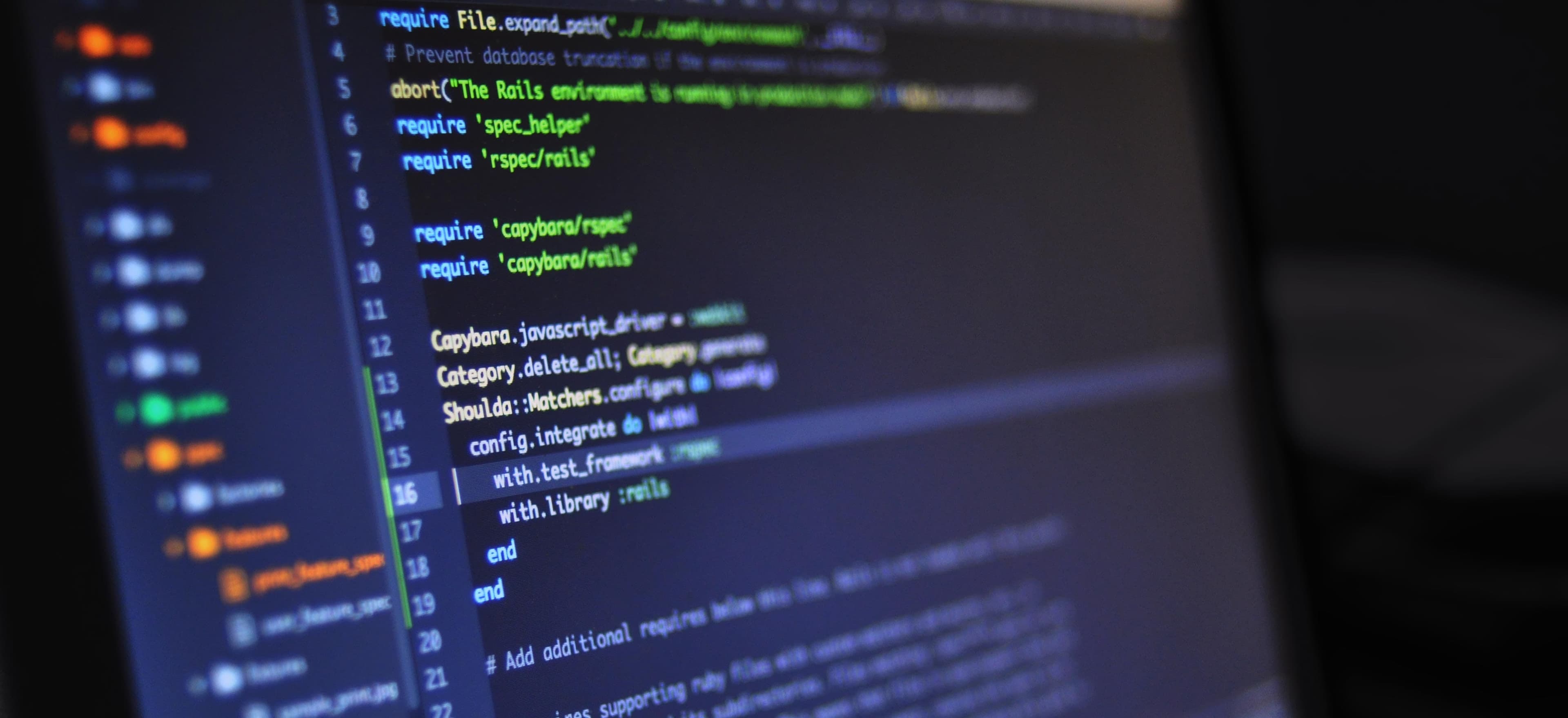
- Published on
Boost Your Agile Workflow: Effective Regression Testing Tips
In the fast-paced world of software development, maintaining agility while ensuring high-quality performance can be an overwhelming challenge. One of the key practices that help teams uphold these standards is regression testing. This blog post aims to provide you with effective tips on managing regression testing in an agile environment. We will cover essential aspects and methods to optimize your workflow, enhancing both productivity and code reliability.
Understanding Regression Testing
Regression testing is a type of software testing that ensures that previously developed and tested features still function after changes, whether those changes are enhancements, bug fixes, or other modifications. It acts as a safety net, ensuring that new code does not adversely affect existing functionalities.
Why Is Regression Testing Critical?
- Bug Prevention: As code evolves, new bugs may inadvertently emerge. Regression testing serves as a proactive measure to identify these issues before deployment.
- Savvy Development: In an Agile environment where speed is essential, regression tests allow developers to move quickly without fear of breaking existing features.
- Confidence Building: Having a robust suite of regression tests instills confidence in team members as well as stakeholders, assuring them of the application’s stability.
For more insights on the principles that support regression testing, consider checking ISTQB's official documentation.
Tips for Implementing Effective Regression Testing
1. Automate Wherever Possible
Automation is the backbone of effective regression testing. Manual testing can be tedious and prone to human error, which can compromise the quality of your tests.
Automating with Java:
Using a testing framework like JUnit can simplify the automation process in Java applications. Here's an example of how to set up a basic regression test:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
Calculator calculator = new Calculator();
@Test
public void testAddition() {
// This test checks the addition functionality
assertEquals(5, calculator.add(2, 3));
}
}
Why This Matters: The simplicity of using JUnit not only saves time but also allows for a systematic approach to managing tests. Automated tests can be rerun after changes to ensure that existing functionality is intact, thus creating a cycle of continuous improvement.
2. Keep Your Test Suite Up-to-Date
Your regression test suite should evolve alongside your codebase. Regularly review and refine tests to remove obsolete cases and include any new functionalities.
- Tip: Embrace code reviews as a way to assess the relevance of your tests. A fresh pair of eyes may identify redundant tests that can be removed, or new features that require testing.
3. Prioritize Tests Based on Risk
Not every feature holds equal weight regarding business impact. Prioritizing tests based on their criticality helps streamline the testing process.
- Test Types:
- High Priority: Interactions related to payment, user login, and product checkouts should be tested thoroughly.
- Medium Priority: Features like reporting tools or user settings can be tested less rigorously.
- Low Priority: Minor UI tweaks or non-essential features may require minimal testing.
This approach maximizes the effectiveness of your regression testing efforts while aligning them with overall business goals.
4. Establish a Continuous Integration Pipeline
Incorporating regression tests into a Continuous Integration (CI) pipeline makes them an integral part of the development lifecycle.
Here’s a simple CI configuration using Jenkins:
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
sh 'mvn clean install'
}
}
}
stage('Test') {
steps {
script {
sh 'mvn test'
}
}
}
}
}
Understanding CI in This Context: Automation within the CI pipeline ensures that regression tests are executed with every code change, allowing the team to rapidly identify issues. This immediate feedback loop encourages a more agile approach to development.
5. Facilitate Collaboration Between Teams
Regression testing is not just the responsibility of the QA team. Developers should also be involved, contributing their insights and knowledge.
Regular cross-functional meetings can foster an open dialogue between developers and testers, allowing for a shared understanding of the application's past, present, and future. Collaborative tools like Jira or Trello also can help track issues and prioritize them effectively.
6. Analyze Results With a Critical Eye
Executing tests without a deeper analysis can lead to a false sense of security. Utilize reporting tools to generate insights from the regression test results.
- Key Metrics:
- Pass Rate: Monitor how many tests pass versus fail.
- Test Coverage: Ensure coverage across critical parts of the application.
- Time to Execute: Evaluate efficiency and seek improvements.
Why Analysis Matters: Having the right metrics helps identify weak spots in your code, maintain the integrity of the application and understand the impact of new changes.
7. Incorporate Exploratory Testing
While automated tests cover defined scenarios, exploratory testing can uncover hidden bugs often missed during scripted tests.
Encourage your team to delve into the features as a user would, investigating unexpected conditions and use cases. Document any findings and ensure appropriate regression tests are added for similar scenarios in the future.
8. Document and Share Knowledge
As your testing paradigm evolves, documentation becomes crucial. Maintain a knowledge base to track changes in the codebase, test cases, and results.
Utilizing tools like Confluence or Google Docs to record lessons learned can ensure that future team members do not make the same mistakes.
Closing the Chapter
Effective regression testing is a cornerstone of an agile software development process. Through automation, prioritization, collaboration, and continuous learning, teams can significantly boost their productivity while ensuring high-quality standards.
By implementing these tips, your team will not only minimize bugs but also foster an environment of confidence and efficiency.
For further reading on enhancing your testing approach, you may want to explore resources on Agile Testing.
Embracing these strategies can transform your regression testing process, leading to a more robust, reliable, and agile software development lifecycle. Happy testing!