Common Pitfalls in Android Push Notifications with Parse
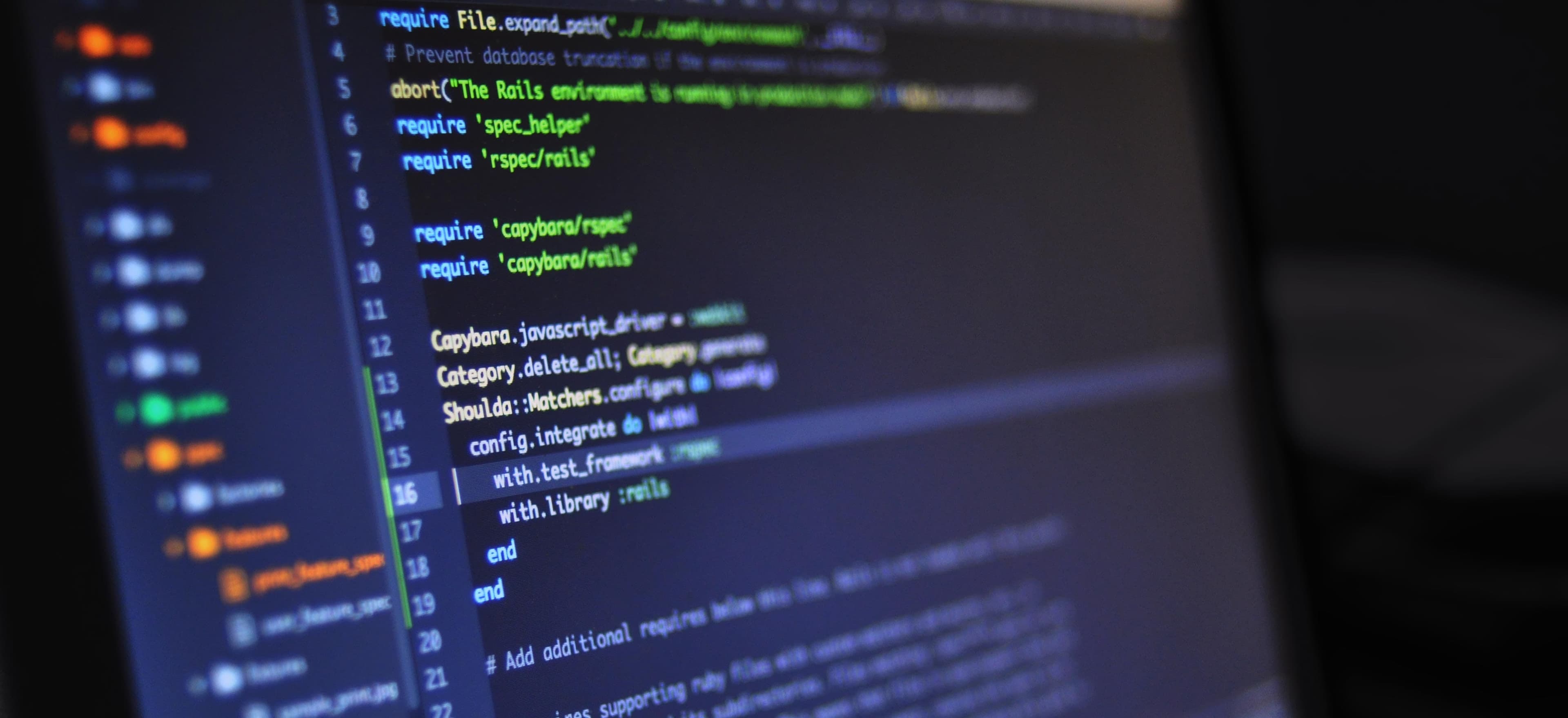
- Published on
Common Pitfalls in Android Push Notifications with Parse
Push notifications are an essential feature in modern mobile applications, keeping users informed and engaged with real-time updates. For Android developers, setting up push notifications using Parse can be both a blessing and a curse. While Parse provides an excellent framework for simplifying backend tasks, it’s easy to stumble into common pitfalls that can affect your app's user experience and functionality. In this blog post, we’ll discuss these pitfalls, how to avoid them, and best practices to ensure smooth implementation.
Understanding Push Notifications
Before delving into potential pitfalls, it's vital to clarify what push notifications are. Push notifications are messages sent from a server to a user's device, alerting them to new content, updates, or reminders. They work by leveraging services such as Firebase Cloud Messaging (FCM) and Parse.
When implementing push notifications, especially via Parse, you may encounter various issues that can lead to suboptimal user experiences. Below are some common pitfalls developers face.
1. Not Registering Device Tokens Properly
Problem:
Failing to register device tokens can prevent your app from receiving notifications.
Solution:
Ensure that you have the following code in your Application
class to register for push notifications:
ParseInstallation installation = ParseInstallation.getCurrentInstallation();
installation.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e == null) {
// Successfully saved installation
} else {
// Handle error
}
}
});
Why:
This code snippet fetches the current installation token and saves it, which is crucial for addressing push notifications to the correct device.
2. Neglecting User Permissions
Problem:
Push notifications will not function if users have not granted the necessary permissions.
Solution:
Before sending notifications, ensure your application checks whether users have enabled notifications. Use the following code:
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
NotificationManager notificationManager = (NotificationManager) getSystemService(NOTIFICATION_SERVICE);
if (!notificationManager.areNotificationsEnabled()) {
// Prompt user to enable notifications
}
}
Why:
This check is vital because starting from Android Oreo, users can customize notification settings, and you need to ensure they aren't disabled.
3. Sending Irrelevant Notifications
Problem:
Sending irrelevant or too many notifications can lead to user disengagement and even app uninstallations.
Solution:
Consider implementing user segmentation based on interests and activity. This allows you to send tailored notifications to relevant users. For example:
ParseQuery<ParseInstallation> query = ParseInstallation.getQuery();
query.whereEqualTo("user_id", userId);
Why:
Targeted notifications lead to higher user satisfaction and retention rates. Using demographic or behavioral data can improve engagement significantly.
4. Ignoring Notification Channels
Problem:
With Android Oreo and later versions, ignoring notification channels can lead to low engagement rates.
Solution:
Set up notification channels to provide users with control over the types of notifications they receive:
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
NotificationChannel channel = new NotificationChannel("YOUR_CHANNEL_ID", "Channel Name", NotificationManager.IMPORTANCE_DEFAULT);
NotificationManager notificationManager = getSystemService(NotificationManager.class);
notificationManager.createNotificationChannel(channel);
}
Why:
Notification channels allow users to manage notifications more effectively, boosting the chances of your messages being viewed.
5. Misconfigured Parse Server Settings
Problem:
Mistakes in the server settings can result in failed push notifications.
Solution:
Verify that your Parse Server is correctly configured for sending push notifications, including the correct FCM credentials.
Make sure you have the following in your ParseServer
configuration:
{
"push": {
"android": {
"fcmServerKey": "YOUR_FCM_SERVER_KEY"
}
}
}
Why:
Without the right server configuration, your push messages won’t be transmitted effectively, leading to a frustrating experience for both users and developers.
6. Not Handling Notification Actions
Problem:
Failing to manage user interactions with the notification.
Solution:
Implement a broadcast receiver to handle the actions when a user taps on the notification:
public class PushReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
Intent notificationIntent = new Intent(context, MainActivity.class);
notificationIntent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
context.startActivity(notificationIntent);
}
}
Why:
Understanding what happens when a user interacts with a notification enhances the user experience. Making it easy to navigate back to the app upon interaction encourages user engagement.
7. Not Testing Across Devices
Problem:
Different devices and versions of Android may handle push notifications differently.
Solution:
Thoroughly test your notifications across various devices and Android versions. Use both emulators and real devices to cover a wide range of scenarios.
Why:
Things that work on one device may not work on another due to manufacturer customizations or OS limitations. Testing helps ensure a consistent user experience.
8. Overlooking Analytics
Problem:
Ignoring analytics on push notifications can lead to missed improvement opportunities.
Solution:
Leverage analytics for evaluating the performance of your push notifications. Tools like Google Analytics or Firebase can help you determine open rates, user engagement, and more.
Why:
Data-driven decisions can greatly enhance your app's push notification strategy. Understanding user behavior can guide you to make informed tweaks and improvements.
Closing Remarks
Implementing push notifications via Parse can greatly enhance user engagement and retention if done correctly. However, it is crucial to be mindful of common pitfalls along the way. By following the best practices outlined in this post—such as registering device tokens, handling user permissions, and managing notification settings—you can ensure that your notifications serve their intended purpose.
Push notifications can be a powerful tool if executed thoughtfully. Make your notifications meaningful, relevant, and engaging to deliver the best experience for your users. If you have questions or share your experience with push notifications, feel free to add your thoughts in the comments below!
For additional details, consider checking the Parse Documentation. Happy coding!
Checkout our other articles