Common WebSocket Pitfalls in Spring and Vue.js Integration
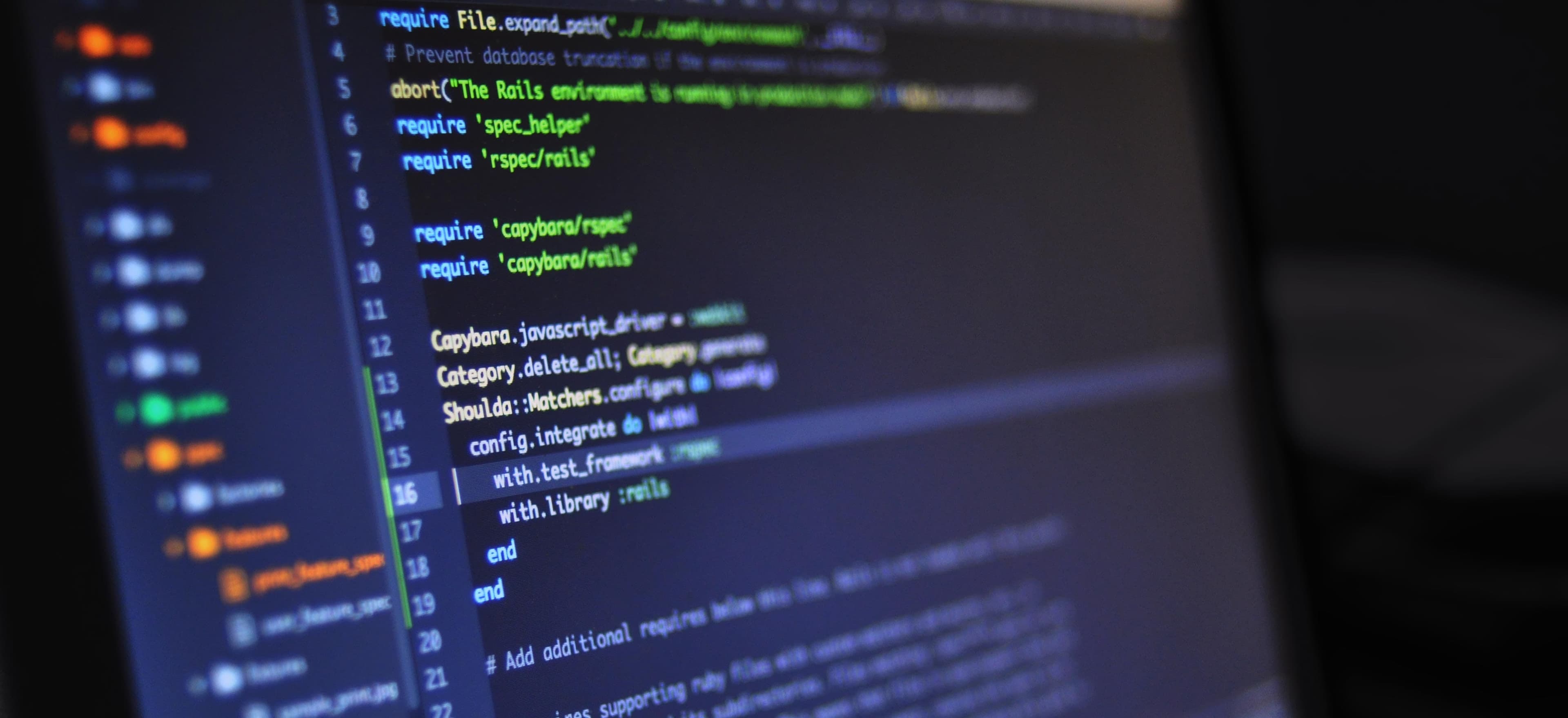
- Published on
Common WebSocket Pitfalls in Spring and Vue.js Integration
As real-time applications continue to rise in popularity, WebSockets have become a cornerstone for enabling efficient, bidirectional communication between clients and servers. Integrating WebSockets in a Spring and Vue.js environment can accelerate application responsiveness, but it isn't without its challenges. In this post, we will explore common pitfalls when integrating WebSockets between Spring on the back end and Vue.js on the front end. By the end of this article, you should have a comprehensive understanding of these issues and how to overcome them.
Table of Contents
- Understanding WebSockets
- The Importance of Spring WebSocket
- Vue.js: A Reactive Frontend Framework
- Common Pitfalls
- Pitfall 1: Connection Handling
- Pitfall 2: Message Format and Serialization
- Pitfall 3: Error Handling
- Pitfall 4: Authentication and Security
- Pitfall 5: Cleanup and Resource Management
- Best Practices for WebSocket Integration
- Conclusion
Understanding WebSockets
WebSockets are a protocol for full-duplex communication channels over a single TCP connection. This allows for ongoing communication between a client and a server, making them ideal for applications that require real-time updates like chat applications, live notifications, or collaborative editing.
The Importance of Spring WebSocket
Spring Framework offers first-class support for WebSockets through its Spring WebSocket module. This simplifies the server-side implementation by providing abstractions for WebSocket sessions, message handling, and more. It is particularly useful for Java developers who wish to build scalable applications that leverage real-time features.
Documentation Reference: For detailed guidance, you can check out the official Spring WebSocket Documentation.
Vue.js: A Reactive Frontend Framework
Vue.js is a progressive JavaScript framework for building user interfaces. It is particularly effective for SPAs (Single Page Applications) because of its reactive data binding capabilities. Vue's ability to seamlessly update the UI based on underlying data changes makes it an excellent companion for real-time features delivered via WebSockets.
Common Pitfalls
Pitfall 1: Connection Handling
Problem: Establishing and maintaining WebSocket connections can be tricky, especially when it comes to handling connection retries.
Solution: Always implement robust retries in your connection logic. Here's an exemplary way to handle WebSocket connections in Vue.js:
let websocket;
function connect() {
websocket = new WebSocket("ws://yourserver.com/socket-endpoint");
websocket.onopen = () => {
console.log('WebSocket connection established');
};
websocket.onmessage = (event) => {
console.log('Message from server: ', event.data);
// Process incoming message
};
websocket.onerror = (error) => {
console.error('WebSocket error: ', error);
};
websocket.onclose = (event) => {
console.log('WebSocket connection closed: ', event);
// Reconnect after a few seconds
setTimeout(connect, 5000);
};
}
connect();
This code connects to the WebSocket server and incorporates a retry mechanism that re-establishes the connection if it gets closed unexpectedly.
Pitfall 2: Message Format and Serialization
Problem: Different systems may expect different message formats (e.g. JSON, XML), and incorrectly configured serialization can lead to application errors.
Solution: Choose a common format like JSON for both sending and receiving messages. Here's an example of structuring messages on the Spring side:
@MessageMapping("/chat")
@SendTo("/topic/messages")
public ChatMessage sendMessage(ChatMessage message) {
message.setTimestamp(LocalDateTime.now());
return message; // This object is serialized to JSON
}
In this case, the ChatMessage
class should be properly configured to serialize to JSON. Make sure your front end understands the structure:
// Frontend send message
const message = {
username: "user1",
content: "Hello, World!"
};
websocket.send(JSON.stringify(message));
By keeping the message format consistent across both ends, you minimize unnecessary errors.
Pitfall 3: Error Handling
Problem: Neglecting to implement comprehensive error handling can lead to silent failures.
Solution: Always include error handling in both your Vue and Spring implementations. Here is an example of how to handle errors within Vue.js:
websocket.onerror = (error) => {
console.error('WebSocket error: ', error);
alert('An error occurred with the WebSocket connection.');
};
On the Spring side, you can handle exceptions in message controllers by using @ExceptionHandler
:
@MessagingExceptionHandler
public void handleError(MessagingException e) {
// Log the error or notify the client
}
Pitfall 4: Authentication and Security
Problem: By default, WebSocket connections are vulnerable unless you implement proper authentication and authorization measures.
Solution: Use token-based authentication (like JWT) to secure your WebSocket connections. Verify tokens upon connection:
@EventListener
public void handleWebSocketConnect(SessionConnectedEvent event) {
String token = event.getUser().getName(); // Extract token from session user
if (!isValidToken(token)) {
throw new AuthenticationException("Invalid Token");
}
}
Integrating this in your Vue.js frontend looks like this:
function authenticateWebSocket() {
const token = localStorage.getItem('token');
websocket = new WebSocket("ws://yourserver.com/socket-endpoint?token=" + token);
}
Pitfall 5: Cleanup and Resource Management
Problem: Failing to properly close WebSocket connections or clean up listeners can lead to memory leaks.
Solution: Ensure that you close the connection when your Vue component is destroyed. Here's an example:
beforeDestroy() {
websocket.close();
}
In Spring, also ensure you clean up WebSocket sessions. By using @PreDestroy
, manage session finalization accordingly.
@PreDestroy
public void closeWebSocketSession() {
// Cleanup logic
}
Best Practices for WebSocket Integration
- Use Fallbacks: Implement fallbacks for browsers that do not support WebSockets (like using AJAX long polling).
- Monitor Performance: Keep an eye on performance metrics for connections, message sizes, and load times to ensure optimal user experience.
- Implement Backoff Strategies: For better stability, implement exponential backoff when reconnecting failed connections.
- Deploy in Production Carefully: Configure your WebSocket servers adequately for handling concurrent connections, especially in cluster environments.
The Bottom Line
Integrating WebSockets in a Spring and Vue.js environment can enhance the interactivity of your applications significantly. However, it's crucial to be aware of common pitfalls, such as connection handling, message formats, error management, security concerns, and resource cleanup. By proactively addressing these challenges and adhering to best practices, you can build robust, real-time applications.
For more information on WebSockets in Spring, consult the Spring Documentation.
Whether you're building a chat application, live notifications, or any real-time feature, understanding these pitfalls will better serve you in creating effective and efficient systems. Happy coding!
Checkout our other articles