Unlocking Java 8: Common Pitfalls to Avoid When Adapting
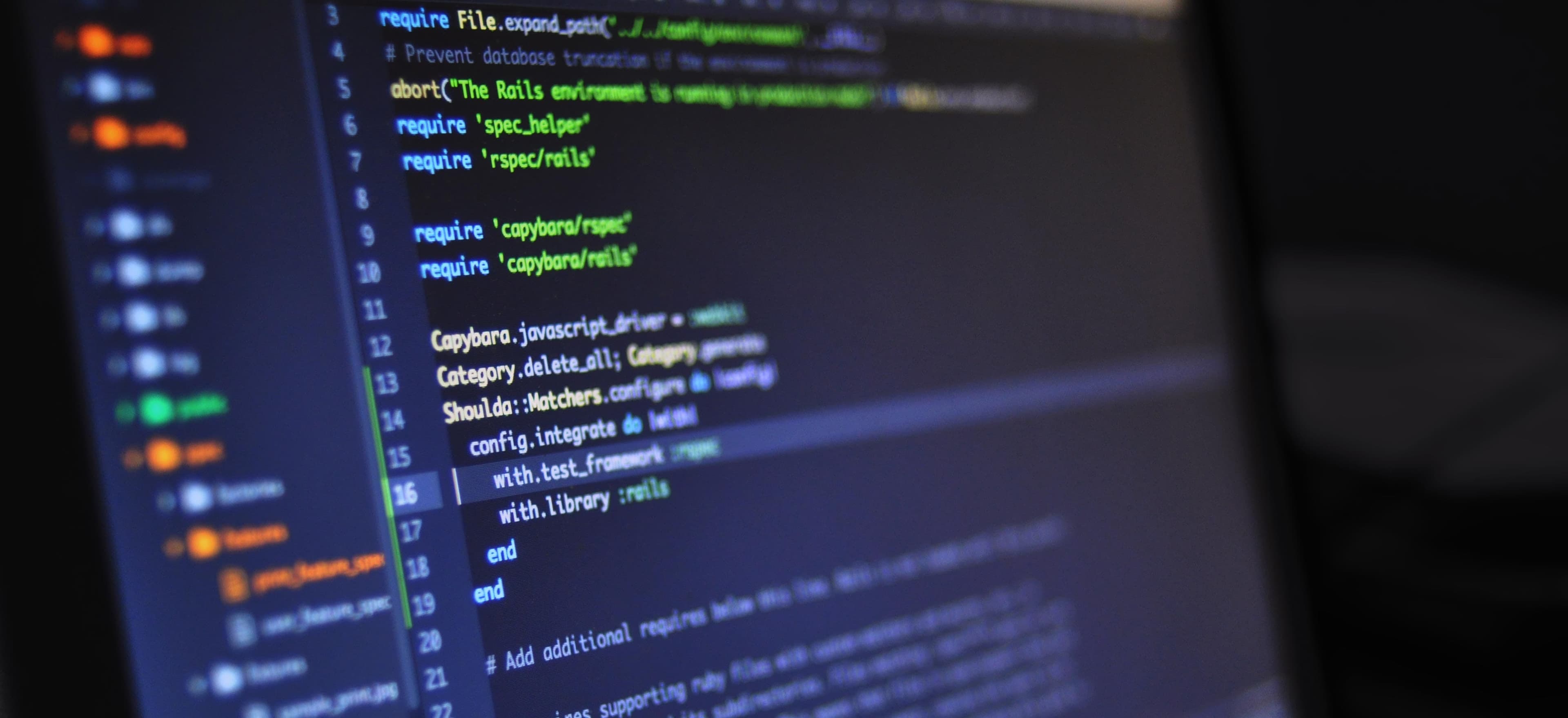
- Published on
Unlocking Java 8: Common Pitfalls to Avoid When Adapting
Java 8 has made monumental shifts in the way we write Java code. With the advent of lambda expressions, the Stream API, and other modern features, developers are encouraged to adopt a more functional programming style. However, transitioning to these new paradigms can pose challenges. In this blog post, we will explore common pitfalls developers face when adapting to Java 8 and provide actionable tips to overcome them.
Understanding Functional Programming
Before delving into specific pitfalls, it is vital to comprehend the foundation of Java 8's new features. Functional programming emphasizes immutability, higher-order functions, and a declarative style of coding. Java 8 attempts to bring some of these principles to the language by introducing:
- Lambda Expressions: Allowing you to express instances of single-method interfaces (functional interfaces) in a concise way.
- Stream API: Enabling manipulation of a sequence of elements in a functional style, which can lead to more readable and efficient code.
Understanding the philosophy behind these features will help you avoid potential pitfalls.
Pitfall 1: Misusing Lambda Expressions
Issue
Lambda expressions can make code more concise and expressive. However, misusing or overusing them can lead to code that is difficult to read and maintain. Developers might be tempted to replace every anonymous class with a lambda, even when an anonymous class might still be the better choice for clarity.
Solution
Keep lambda expressions to simple tasks or operations where their benefits are apparent. For more complex logic or situations needing stateful behavior, consider sticking with traditional anonymous classes.
Example
// Using lambda expression for simplicity
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println(name));
In cases where we need more than just printing, an anonymous class might be a better choice:
// Prefer anonymous class for more complexity
names.forEach(new Consumer<String>() {
@Override
public void accept(String name) {
if (name.startsWith("A")) {
System.out.println(name);
}
}
});
The anonymous class retains more context and clarity when conditions become more nuanced.
Pitfall 2: Neglecting Performance Implications of Streams
Issue
The Stream API excels at processing collections efficiently, but developers sometimes overlook potential performance issues. Streaming operations can sometimes yield worse performance compared to traditional loops, especially for smaller data sets.
Solution
Use the Stream API judiciously. For small collections, traditional looping can be more efficient. Always profile your code to ensure that you're not sacrificing performance for stylistic choices.
Example
// Stream API for demonstration
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream().mapToInt(Integer::intValue).sum();
System.out.println("The sum is: " + sum);
For larger datasets or when working with parallelism, the Stream API demonstrates clear performance advantages. However, for small collections, it may be worth sticking with a for-loop:
// Traditional loop for comparison
int total = 0;
for (int num : numbers) {
total += num;
}
System.out.println("The total is: " + total);
Pitfall 3: Misinterpreting Optional
Issue
The Optional
class was introduced to represent a value that may or may not be present, aiming to reduce NullPointerExceptions
. However, it can be misused by overloading it inappropriately, or using it as a replacement for every nullable type.
Solution
Use Optional
judiciously. It should mainly be used as a return type for methods to denote absence rather than as a field type. Optional
aims to enhance code clarity and safety, so leveraging its method capabilities is beneficial.
Example
// Proper use of Optional in a return type
public Optional<String> findNameById(int id) {
// Assume 'findName' returns a String or null
String name = findName(id);
return Optional.ofNullable(name);
}
Using it as a field type can lead to confusing code:
// Avoid using Optional as a field type
public class User {
private Optional<String> nickname;
// Better to use String or other valid types
}
Pitfall 4: Ignoring Type Inference
Issue
Java’s type inference can reduce verbosity in code involving generics, but misinterpretation can lead to errors or unexpected behaviors. Developers may become too reliant on inferred types without fully understanding what types are being inferred.
Solution
Clarify type declarations whenever possible. Explicitly specifying generic types can safeguard against misinterpretation by the compiler. This helps maintain clarity for yourself and other developers.
Example
// Type inference with lambda
List<String> items = Arrays.asList("A", "B", "C");
items.forEach(item -> System.out.println(item));
To improve clarity, specify the types explicitly:
// Specifying types
items.forEach((String item) -> System.out.println(item));
Pitfall 5: Overlooking the New Date and Time API
Issue
Java 8 introduced a new Date and Time API (java.time
) to avoid the pitfalls of the older Date
and Calendar
classes. However, many developers still resort to using the older classes, either out of habit or lack of understanding.
Solution
Familiarize yourself with the new API and embrace its use. It provides immutability, better handling of time zones, and a more intuitive interface.
Example
// Using the old Date class (Not recommended)
Date today = new Date();
System.out.println("Old Date: " + today);
Instead, use the new API for clarity:
// Using the new Date and Time API
LocalDate today = LocalDate.now();
System.out.println("New Date: " + today);
Lessons Learned
Java 8 has profoundly changed the way we approach software development in Java, introducing paradigms that enable cleaner and more maintainable code. However, with these changes come potential pitfalls. By being aware of these common issues—such as misusing lambda expressions, neglecting performance implications, misinterpreting Optional
, ignoring type inference, and overlooking the new Date and Time API—you can take full advantage of Java 8’s capabilities.
For further reading on Java 8's features and best practices, visit Oracle's official documentation and explore the Java Magazine for in-depth articles and tips.
By incorporating these mindful strategies, you will not only enhance your own programming proficiency but also contribute to an overall improvement in code quality within your team and projects. Happy coding in Java 8!
Checkout our other articles