Common Pitfalls in Cucumber's Pass List Parameter Technique
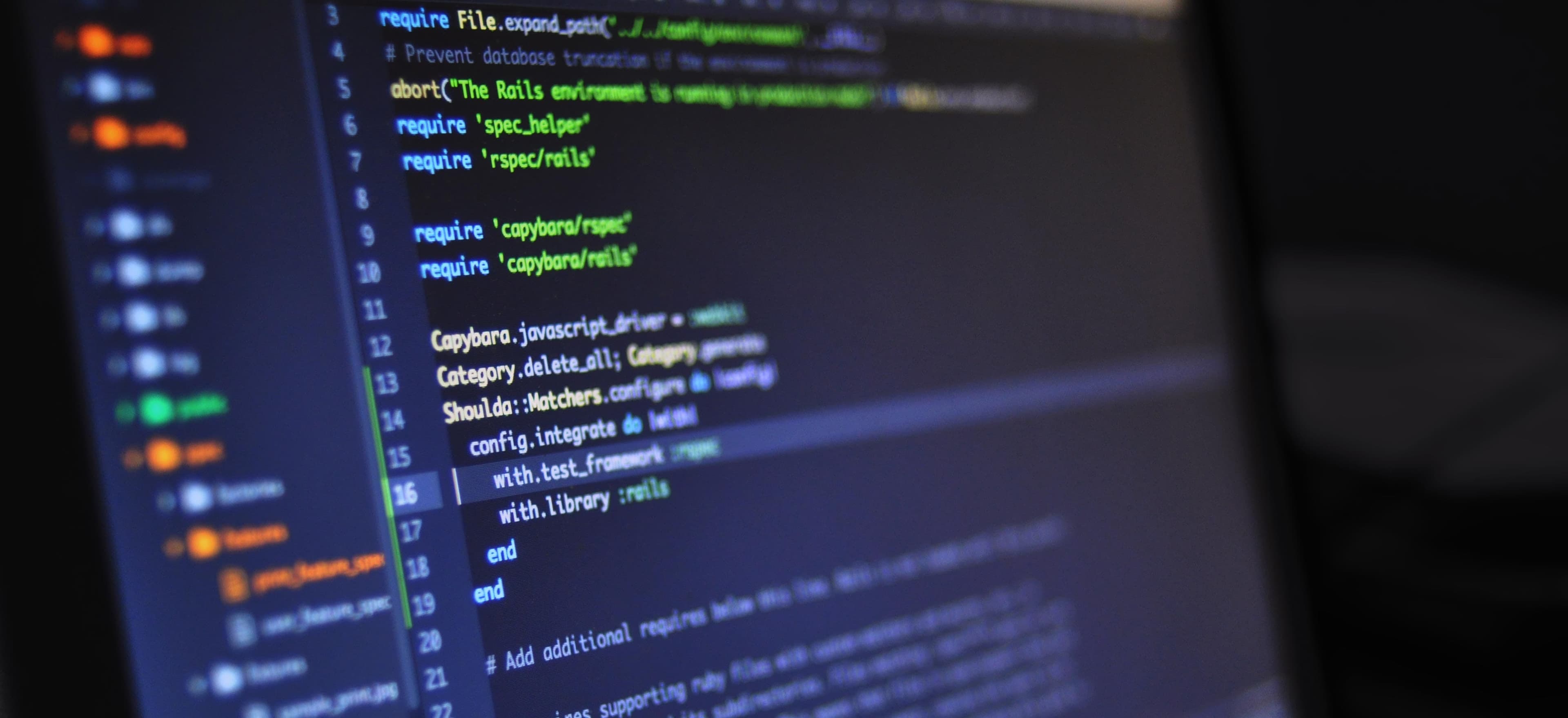
- Published on
Common Pitfalls in Cucumber's Pass List Parameter Technique
Cucumber is widely recognized as one of the most effective tools for behavior-driven development (BDD). It allows testers, developers, and business stakeholders to collaborate and ensure that user stories are converted into conducted tests. One of its powerful features is the ability to pass list parameters in step definitions. However, this capability can lead to various pitfalls if not understood and utilized properly. In this post, we will explore these common pitfalls, and through code snippets, we will demonstrate best practices to avoid them.
Table of Contents
- Introduction to Cucumber
- Understanding List Parameters
- Common Pitfalls
- Incorrect Data Structure
- Misinterpretation of Data Types
- Lack of Clear Documentation
- Inconsistent Naming Conventions
- Best Practices
- Conclusion
Let us Begin to Cucumber
Cucumber allows users to write test scenarios in a human-readable format through Gherkin syntax. This format facilitates communication among team members and promotes a shared understanding of system behavior. For more information on Gherkin syntax, you can visit the Cucumber Documentation.
Let’s begin our exploration by understanding the concept of list parameters in Cucumber.
Understanding List Parameters
In Cucumber, you can pass multiple parameters to a step definition as a list. This is particularly useful for scenarios where you want to input several values at once. For example, if you are testing a shopping cart feature and want to add multiple items at once, you might use the following Gherkin scenario:
Given the following items exist in the cart:
| item name | quantity |
| Apple | 2 |
| Banana | 5 |
| Orange | 3 |
In a step definition, you would capture this data as a list of maps or lists, enabling easier manipulation and assertions in your test code.
Common Pitfalls
1. Incorrect Data Structure
One of the most common mistakes when passing list parameters is using the wrong data structure. While Cucumber's Gherkin can represent tabular data using the pipe (|
) symbol, how you handle this data in your step definitions can drastically affect performance and outcome.
For example:
@Given("the following items exist in the cart:")
public void addItemsToCart(List<List<String>> items) {
for (List<String> item : items) {
String name = item.get(0);
int quantity = Integer.parseInt(item.get(1));
// Add the item to the cart logic here
}
}
The Pitfall: Using List<List<String>>
instead of List<Map<String, String>>
makes the code harder to read and manage. It is challenging to associate item names with their quantities.
Best Practice: Use a map to represent named sets of data.
@Given("the following items exist in the cart:")
public void addItemsToCart(List<Map<String, String>> items) {
for (Map<String, String> item : items) {
String name = item.get("item name");
int quantity = Integer.parseInt(item.get("quantity"));
// Add the item to the cart here
}
}
2. Misinterpretation of Data Types
When passing parameters, it’s easy to overlook the types of data being handled. Misinterpreting the data type can lead to runtime errors that could have been avoided.
In code like this:
@Given("I have {int} items in the cart")
public void iHaveItemsInCart(int quantity) {
// Logic to check items in the cart
}
The Pitfall: You expect quantity
to be an integer, but if the scenario mistakenly passes a string value, it will result in a NumberFormatException
.
Best Practice: Always validate the data format at the point of reading the inputs.
@Given("the following items exist in the cart:")
public void addItemsToCart(List<Map<String, String>> items) {
for (Map<String, String> item : items) {
String quantityStr = item.get("quantity");
int quantity;
try {
quantity = Integer.parseInt(quantityStr);
} catch (NumberFormatException e) {
throw new IllegalArgumentException("Invalid quantity: " + quantityStr);
}
// Add item to the cart logic here
}
}
3. Lack of Clear Documentation
One of the strengths of Cucumber is how it promotes collaboration and communication between team members. However, not having proper documentation for your step definitions can lead to misunderstandings.
The Pitfall: A developer working on the project may not know the expected formats for the list parameters.
Best Practice: Provide clear comments on what parameters are expected in each step definition.
/**
* Adds items to the cart based on the provided data.
*
* @param items A list of maps where each map contains:
* - item name: the name of the item (String)
* - quantity: the number of items to add (String, must be convertible to int)
*/
@Given("the following items exist in the cart:")
public void addItemsToCart(List<Map<String, String>> items) {
// Method implementation
}
4. Inconsistent Naming Conventions
Consistency in naming conventions is crucial for maintainable code. If your naming conventions differ between the Gherkin scenarios and your step definitions, this inconsistency can lead to confusion.
The Pitfall: If the names in the Gherkin do not match those expected by the step definition, your tests will fail.
Best Practice: Maintain consistent naming conventions for parameters. For example, ensure the columns in your Gherkin scenario unify with the keys used in your code.
Given the following items exist in the cart:
| item_name | quantity |
| Apple | 2 |
| Banana | 5 |
@Given("the following items exist in the cart:")
public void addItemsToCart(List<Map<String, String>> items) {
for (Map<String, String> item : items) {
String name = item.get("item_name");
int quantity = Integer.parseInt(item.get("quantity"));
// Ensure we add items correctly
}
}
Best Practices
To ensure you avoid these pitfalls, here are some best practices to consider:
-
Use Meaningful Data Structures: Use
List<Map<String, String>>
for clarity when passing lists of parameters. -
Validate Input Types: Implement checks that ensure input integrity, throwing meaningful errors wherever necessary.
-
Document Everything: Comments that explain the expected structures for inputs promote collaboration.
-
Maintain Consistency: Keep naming conventions consistent across Gherkin and step definitions.
-
Keep it Simple: When in doubt, simplify your approach. A complex setup can lead to more misunderstandings.
Final Thoughts
While passing list parameters in Cucumber is a powerful feature, it comes with its own set of challenges. By being aware of common pitfalls and implementing best practices, you can ensure your tests remain clear, maintainable, and effective. Robust testing leads to better software quality, which is ultimately what every development team strives for.
If you’re interested in taking a deeper dive into Cucumber practices, be sure to check out the official Cucumber Documentation. Happy testing!
Checkout our other articles