Mastering Simplified Array Ops in Jackson's JsonNode
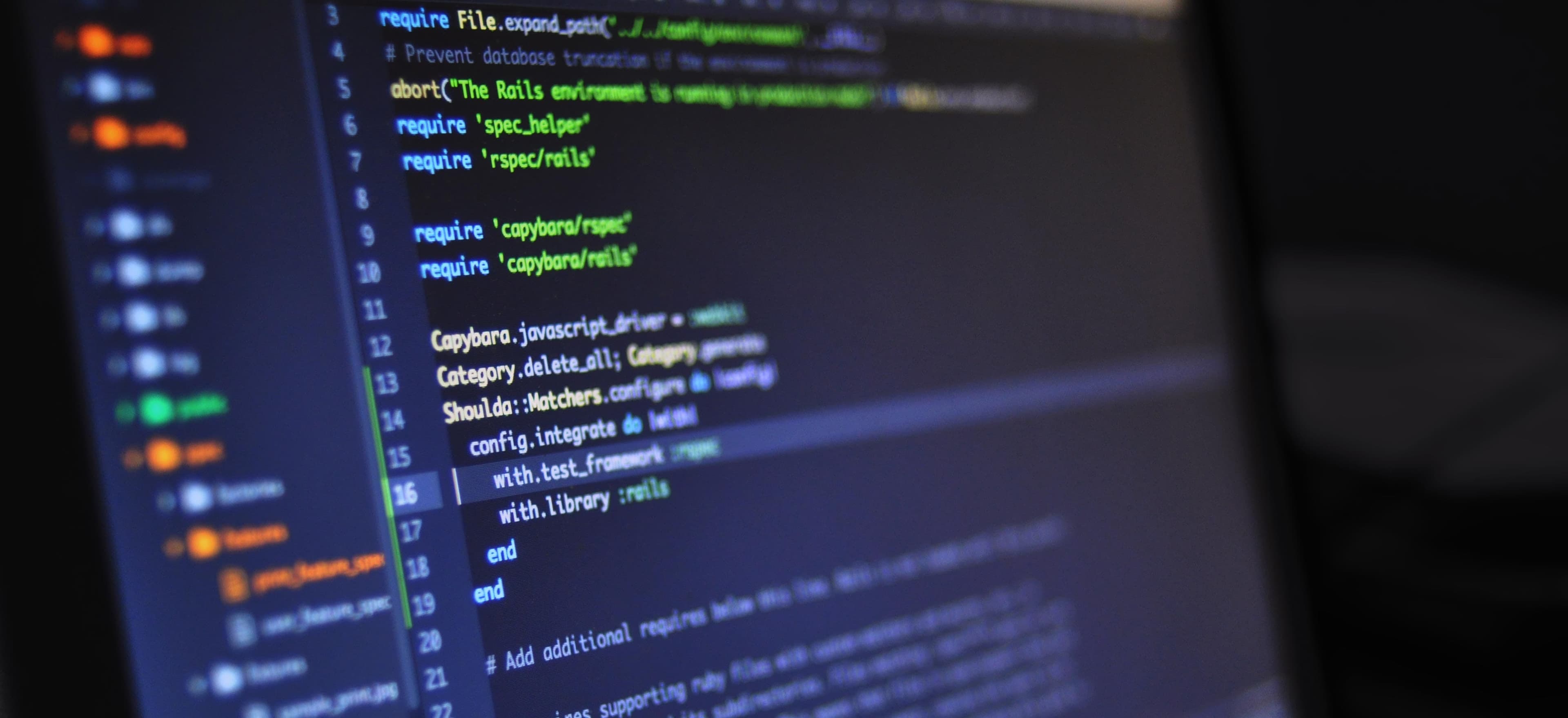
- Published on
Mastering Simplified Array Operations in Jackson's JsonNode
JSON (JavaScript Object Notation) is a widely used data format for data interchange. With the rise of web applications, the ability to handle JSON data efficiently has become increasingly important. One of the most robust libraries for processing JSON in Java is Jackson. In this blog post, we will dive deep into JsonNode
, a fundamental component of the Jackson library, focusing on simplified array operations.
Understanding Jackson's JsonNode
JsonNode
is a part of the Jackson library that represents a JSON object or an array. Jackson treats JSON structures as a tree of JsonNode
instances, enabling easy traversal and manipulation of JSON data.
Here are some key functionalities of JsonNode
:
- Accessing elements.
- Modifying values.
- Iterating over arrays and objects.
- Converting from and to Java objects.
Setting Up Jackson
Before we dive into the operations with JsonNode
, you need to include the Jackson dependencies in your Maven pom.xml
file.
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.13.0</version> <!-- Use the latest version available -->
</dependency>
Creating JsonNode Objects
You can create a JsonNode
instance through a simple conversion of a JSON string with ObjectMapper
. Below is a sample code snippet demonstrating this.
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonNodeExample {
public static void main(String[] args) {
String jsonString = "{ \"employees\": [ { \"name\": \"John\", \"age\": 30 }, { \"name\": \"Doe\", \"age\": 25 } ] }";
ObjectMapper objectMapper = new ObjectMapper();
try {
// Converting the JSON string to JsonNode
JsonNode jsonNode = objectMapper.readTree(jsonString);
System.out.println(jsonNode.toPrettyString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary
The ObjectMapper
is the heart of Jackson's functionality. In the code above, we leverage the readTree
method to read and convert a JSON string into a JsonNode
object. It allows for easy manipulation and retrieval of JSON data.
Accessing Array Elements in JsonNode
Let’s explore how to access elements of a JSON array via JsonNode
. Consider the following JSON structure:
{
"employees": [
{ "name": "John", "age": 30 },
{ "name": "Doe", "age": 25 }
]
}
Accessing Elements
We can access employees array elements as follows:
public class AccessingArrayElements {
public static void main(String[] args) {
String jsonString = "{ \"employees\": [ { \"name\": \"John\", \"age\": 30 }, { \"name\": \"Doe\", \"age\": 25 } ] }";
ObjectMapper objectMapper = new ObjectMapper();
try {
JsonNode jsonNode = objectMapper.readTree(jsonString);
JsonNode employees = jsonNode.get("employees");
for (JsonNode employee : employees) {
String name = employee.get("name").asText();
int age = employee.get("age").asInt();
System.out.println("Employee Name: " + name + ", Age: " + age);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary
Here, we retrieve the employees
node and iterate through each employee
node within the array. asText()
and asInt()
methods allow us to extract values in the desired format.
Modifying Array Elements in JsonNode
Modifying JSON data using JsonNode
is straightforward. Let's say we want to update the age of "John" in our JSON array.
Updating Values
public class ModifyingArrayElements {
public static void main(String[] args) {
String jsonString = "{ \"employees\": [ { \"name\": \"John\", \"age\": 30 }, { \"name\": \"Doe\", \"age\": 25 } ] }";
ObjectMapper objectMapper = new ObjectMapper();
try {
JsonNode jsonNode = objectMapper.readTree(jsonString);
JsonNode employees = jsonNode.get("employees");
for (JsonNode employee : employees) {
if (employee.get("name").asText().equals("John")) {
// Update age
((ObjectNode) employee).put("age", 35);
}
}
System.out.println("Updated JSON: " + jsonNode.toPrettyString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary
In this example, we cast the JsonNode
to ObjectNode
to make modifications. This allows us to set the value of a JsonNode
representing a JSON object, demonstrating how to manipulate nested data structures.
Adding Elements to an Array in JsonNode
Adding a new employee to the array is another critical operation. We can do this easily with JsonNode
.
public class AddingArrayElements {
public static void main(String[] args) {
String jsonString = "{ \"employees\": [ { \"name\": \"John\", \"age\": 30 }, { \"name\": \"Doe\", \"age\": 25 } ] }";
ObjectMapper objectMapper = new ObjectMapper();
try {
JsonNode jsonNode = objectMapper.readTree(jsonString);
ObjectNode employeesNode = (ObjectNode) jsonNode;
ArrayNode employees = (ArrayNode) employeesNode.get("employees");
ObjectNode newEmployee = objectMapper.createObjectNode();
newEmployee.put("name", "Alice");
newEmployee.put("age", 28);
employees.add(newEmployee);
System.out.println("JSON after adding new employee: " + jsonNode.toPrettyString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary
By creating a new ObjectNode
, we can add a complete JSON object to our existing array. The ArrayNode
interface provides methods like add
for easy manipulation of array structures.
Bringing It All Together
In this post, we've explored the functionalities of the Jackson library's JsonNode
for simplified array operations. We walked through creating JsonNode objects, accessing elements, modifying values, and adding new elements to JSON arrays.
Understanding these operations can streamline your process when dealing with APIs or data storage solutions that communicate using JSON.
If you want to learn more about the Jackson library, check out the official Jackson Documentation and deepen your knowledge about manipulating JSON data efficiently.
These concepts are integral to working with Java and JSON, enhancing code maintainability and robustness. Should you have any follow-up questions or require implementation assistance, feel free to leave a comment below!
Checkout our other articles