Overcoming Latency Issues in Serverless Applications
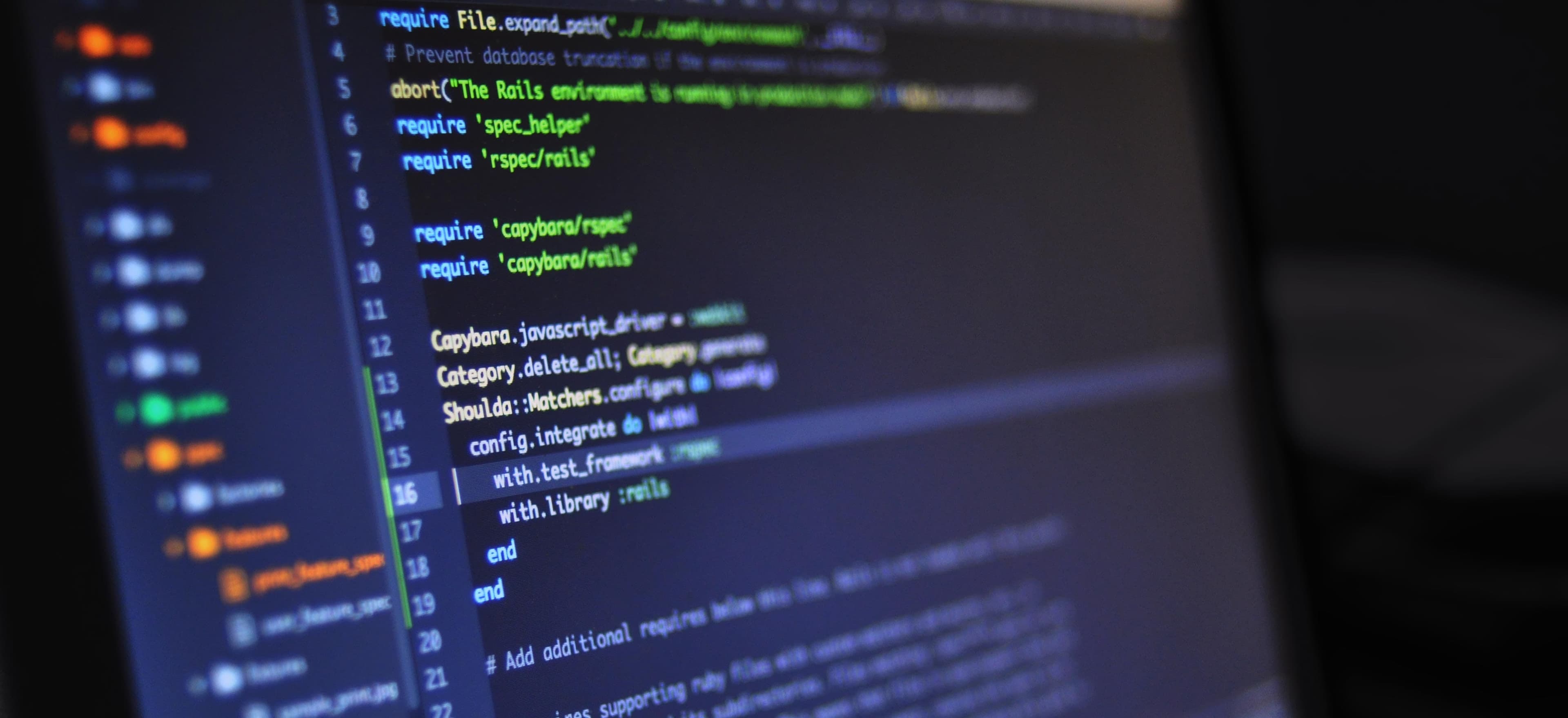
- Published on
Overcoming Latency Issues in Serverless Applications
In recent years, serverless architecture has gained immense popularity among developers due to its inherent scalability and cost-effectiveness. However, like any advanced technology, serverless applications often come with their own set of challenges, one of the most prominent being latency. In this post, we will delve into the intricacies of latency in serverless applications and offer practical strategies to mitigate them.
Understanding Latency in Serverless Architectures
Latency in serverless applications can be defined as the time taken for an application to process a request and deliver a response. In traditional server-based architectures, the underlying infrastructure is always running, so applications are primed for immediate responses. However, serverless functions are event-driven and only run when triggered. This leads to several types of latency, including:
-
Cold Starts: The time taken for a serverless function to initialize after being triggered for the first time or after a period of inactivity.
-
Network Latency: The delay caused when data travels between different servers or databases.
-
Execution Time: The processing time required to run the business logic within the serverless function.
Understanding these latency factors is crucial as they directly affect the user experience.
Cold Starts Explained
Cold starts occur when an application function hasn't been called recently, requiring the cloud provider to spin up a container. This startup time can take seconds, leading to a noticeable delay. The cause of cold starts can vary across platforms, but they generally include:
- Environment Setup: Loading the runtime environment and dependencies.
- Warming Up: Initializing the operating environment for execution.
To give you a practical perspective, let's take a look at a minimal AWS Lambda function:
public class MyLambdaFunction implements RequestHandler<String, String> {
@Override
public String handleRequest(String input, Context context) {
// Business logic goes here
return "Hello, " + input;
}
}
In a scenario where this function has not been invoked for a while, the first request would likely experience a cold start. Understanding the structure of this simple function commands insight into what causes latency.
Best Practices for Reducing Latency
Here are several strategies to mitigate latency issues:
1. Optimize Cold Start Times
Although cold starts cannot be wholly eliminated, there are techniques to minimize them:
- Keep Functions Warm: Periodically invoke the function to keep it active. This can be automated with scheduled triggers, such as AWS CloudWatch Events.
// Scheduled event to invoke the function every 5 minutes
public class ScheduleKeepAlive implements RequestHandler<Object, String> {
@Override
public String handleRequest(Object input, Context context) {
// No operation; just to keep the function alive
return "Keeping warm!";
}
}
-
Reduce Dependencies: Limit the libraries and dependencies loaded during the execution. The lighter the initialization, the faster the cold start.
-
Choose the Right Language: Some languages, like Java, might introduce higher cold starts than others like Node.js. Analyze your function's language before implementation.
2. Minimize Network Latency
Network latency can be a significant bottleneck. Here are a few strategies to optimize performance:
-
Use Edge Caching: Leverage Content Delivery Networks (CDNs) to cache responses closer to users. This decreases the time taken for requests and responses.
-
Optimize API Calls: Minimize calls to external services. Aggregate multiple calls into a single request whenever possible.
3. Enhance Execution Efficiency
The execution time needed to process the request can also be optimized:
- Efficient Code: Write efficient algorithms. For instance, if using a loop, choose an optimal data structure, such as a HashMap instead of an ArrayList, to minimize time complexity.
Map<String, Integer> userScores = new HashMap<>();
userScores.put("Alice", 90);
userScores.put("Bob", 85);
// Using HashMap allows for O(1) time complexity for retrieval
Integer aliceScore = userScores.get("Alice");
- AWS Lambda Performance Optimization: For AWS Lambda, consider adjusting the allocated memory for your functions since it comes with an increase in CPU power, which may lead to faster execution.
4. Choose the Right Region
Your serverless function's location plays a crucial role in latency. Choose a region close to your user base. For example, if your users are primarily in Europe, deploying functions in the European region can significantly reduce latency.
5. Analyze and Monitor
Utilizing monitoring tools can drastically improve your application's performance by providing critical insights into where latency issues arise. AWS CloudWatch, for example, allows monitoring your Lambda functions to identify cold starts and execution time metrics.
The Last Word
In a world where speed and efficiency matter, overcoming latency issues in serverless applications is crucial. By understanding the different types of latency and implementing optimization strategies, developers can provide better user experiences while fully leveraging the benefits of serverless architecture.
For further reading, consider visiting AWS Documentation on AWS Lambda for advanced configurations and strategies, and check out Google Cloud Functions for similar insights on how to manage serverless applications on Google Cloud.
By keeping these considerations in mind, you can ensure your serverless applications perform optimally, offering users both speed and reliability in their interactions. The future of development is serverless, and overcoming these challenges is just the beginning of maximizing its potential.
Checkout our other articles