Troubleshooting WebSocket Connections on WildFly and OpenShift
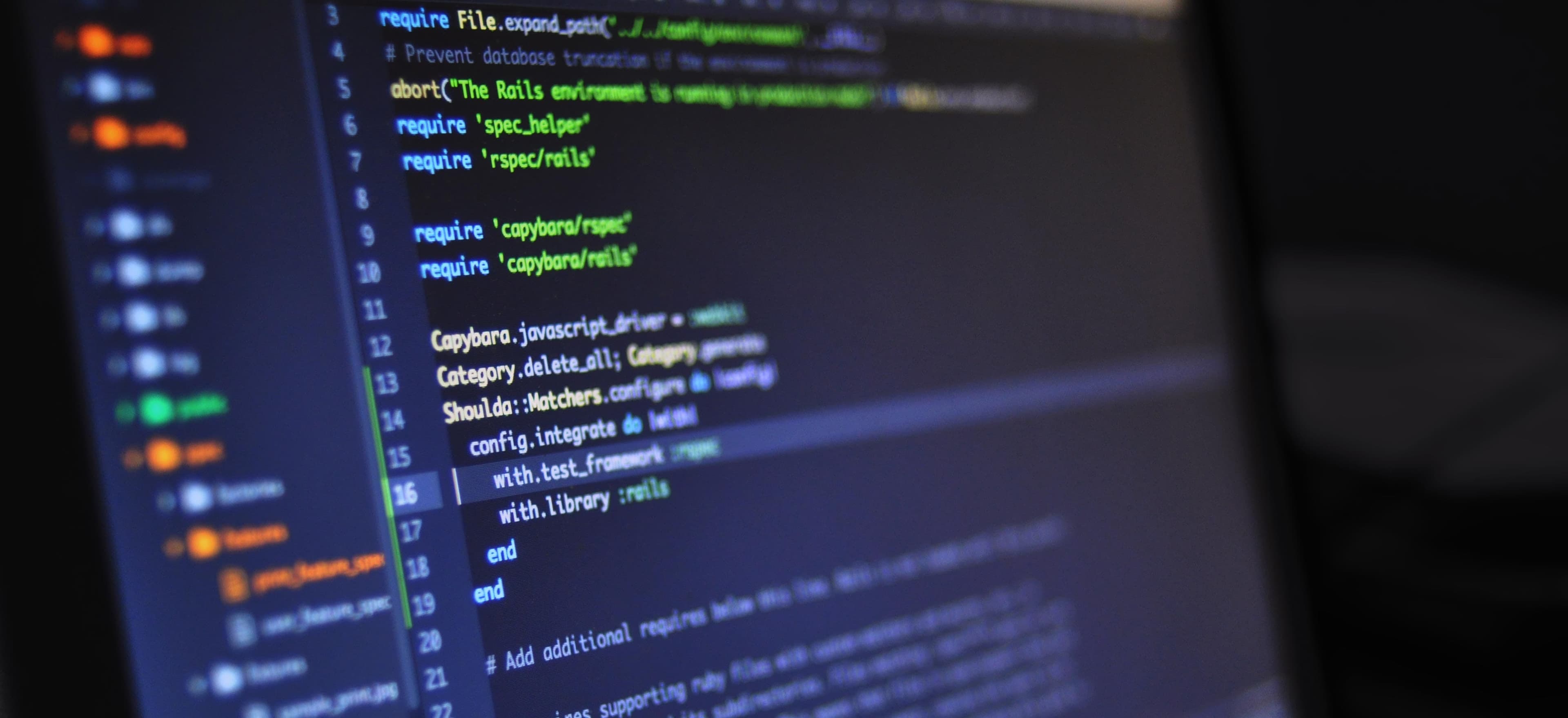
- Published on
Troubleshooting WebSocket Connections on WildFly and OpenShift
WebSockets facilitate full-duplex communication channels over a single TCP connection, making them crucial for real-time applications. In a microservices architecture, like one deployed on OpenShift, debugging WebSocket connections can sometimes be challenging. This blog post delves into troubleshooting WebSocket connections specifically within WildFly, a powerful Jakarta EE application server, running on an OpenShift platform.
Understanding WebSocket Basics
Before delving into troubleshooting, let’s briefly revisit what WebSockets are. They were designed to enable interactive communication between a client and server. This is particularly vital for applications that require real-time data, such as chats, notifications, or live updates.
Here's a basic WebSocket server endpoint implemented in Java:
import javax.websocket.OnMessage;
import javax.websocket.OnOpen;
import javax.websocket.Session;
import javax.websocket.server.ServerEndpoint;
@ServerEndpoint("/websocket")
public class MyWebSocketServer {
@OnOpen
public void onOpen(Session session) {
System.out.println("New connection: " + session.getId());
}
@OnMessage
public void onMessage(String message, Session session) {
System.out.println("Received message from " + session.getId() + ": " + message);
}
}
Why This Code is Important
This example illustrates the simplest form of a WebSocket server. It opens a connection and listens for incoming messages. Understanding this basic endpoint structure is crucial as it forms the foundation for identifying where potential issues may arise.
Common Issues When Deploying on OpenShift
When deploying WebSocket applications on OpenShift with WildFly, developers can encounter several common issues:
- Network Policies: OpenShift's security configurations may block WebSocket connections.
- Service Configuration: The way services are defined can affect the ability to proxy WebSocket connections.
- Application Logs: Logs, or the lack thereof, can make debugging difficult.
Inspecting Network Policies
Access vs. Security policies are often the root cause of WebSocket connectivity problems. Ensure your pods can accept traffic on the required port. Use the command:
oc get networkpolicies
If your WebSocket connection is blocked, you might need to modify the network policy. A typical Network Policy might look like this:
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: allow-websocket
spec:
podSelector:
matchLabels:
app: your-websocket-app
policyTypes:
- Ingress
ingress:
- from:
- podSelector:
matchLabels:
app: your-client-app
ports:
- protocol: TCP
port: 8080 # Ensure this matches your WebSocket port
This configuration allows traffic from specified pods to the WebSocket app. Always verify that the ports match your WebSocket configuration.
Reviewing Service Configuration
In OpenShift, services are defined to route network traffic to pods. Incorrect service configurations can hinder WebSocket connections. For WebSockets, you generally need to define a service that requires a Type
of NodePort
or LoadBalancer
.
Here’s a sample service definition:
apiVersion: v1
kind: Service
metadata:
name: websocket-service
spec:
selector:
app: your-websocket-app
ports:
- protocol: TCP
port: 8080
targetPort: 8080
type: LoadBalancer
Why This is Essential
The LoadBalancer
type is critical for public access. Ensure you expose the correct port for WebSocket connections (often 80 for HTTP and 443 for secure WebSocket connections).
Examining Application Logs on WildFly
Logs are crucial in understanding what goes wrong. In WildFly, you can tweak your logging configuration to gain more insight into WebSocket activity. Adjust the standalone.xml
configuration for better logging of WebSocket events:
<logger category="org.jboss.ws">
<level name="DEBUG"/>
</logger>
Restart your WildFly server after changing the configuration. You can view logs using:
kubectl logs <pod-name>
Look specifically for any errors related to WebSocket connections or authentication issues.
Testing WebSocket Connections
Once your configuration is finalized, it's beneficial to test the WebSocket connection thoroughly. Using tools like wscat allows for manual testing:
npx wscat -c ws://your-openshift-url/websocket
If the connection is successful, you should see a prompt where you can send messages. Sending a string should trigger the corresponding onMessage
callback in your server.
Handling Common Errors
1. Connecting to the Wrong Port: Ensure you're using the correct port and WebSocket endpoint URL.
2. CORS Issues: If you’re connecting from a different domain, ensure your server allows cross-origin requests.
3. Firewall Rules: Check firewalls on your local machine, server, and cloud provider to ensure they permit WebSocket traffic.
Closing Remarks
Troubleshooting WebSocket connections on WildFly deployed in OpenShift requires a systematic approach to diagnosing potential issues ranging from network configurations to application logs. By ensuring proper network policies, configuring services correctly, and utilizing logging effectively, you can significantly improve your chances of resolving WebSocket connectivity problems.
For further reading, consider these resources:
- WebSocket API Documentation
- OpenShift Networking
- WildFly Documentation
Remember, successful WebSocket communication is a combination of clear configurations, understanding underlying concepts, and diligent testing. Happy coding!
Checkout our other articles