Mastering Java Spoon: Overcoming Code Analysis Challenges
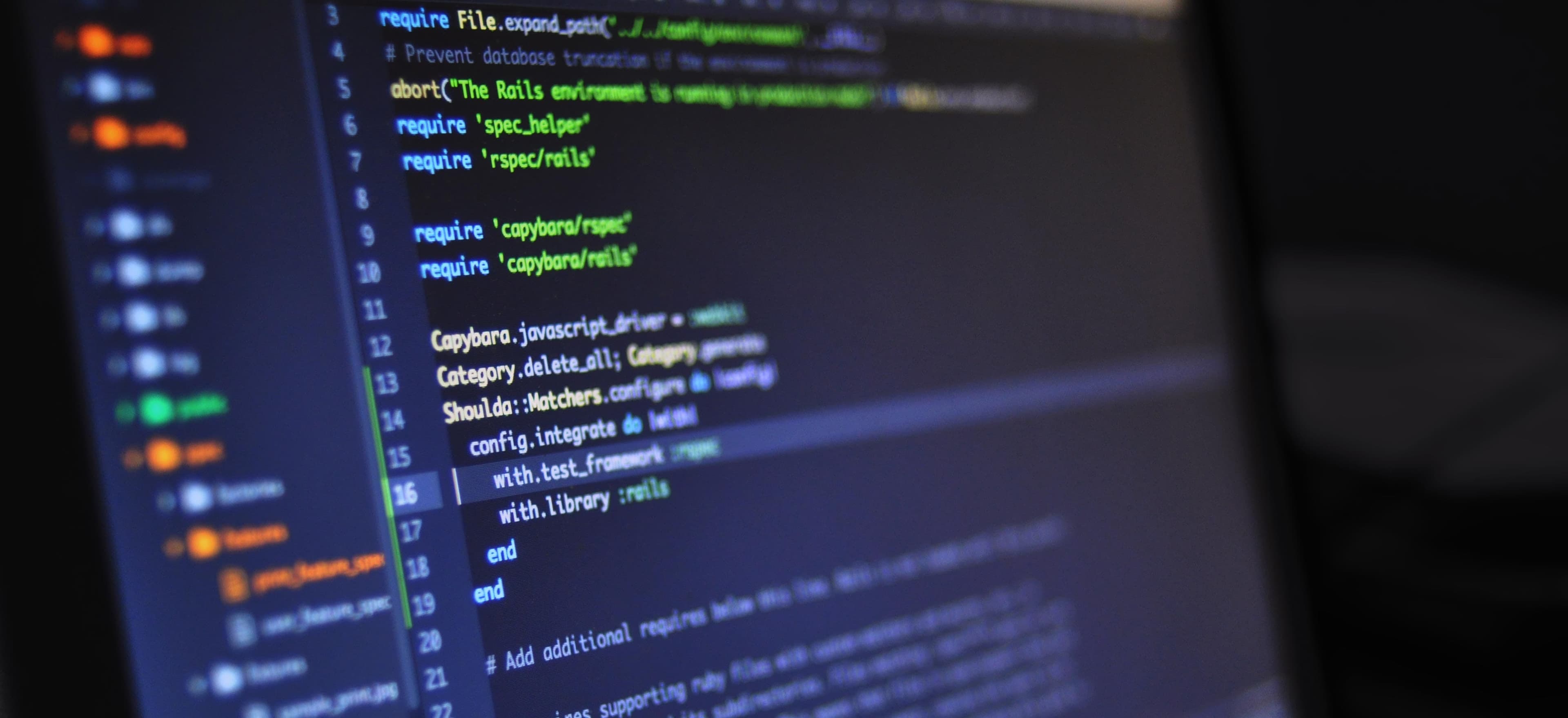
- Published on
Mastering Java Spoon: Overcoming Code Analysis Challenges
In the realm of code analysis and transformation, tools play a crucial role in enhancing productivity while maintaining code quality. Spoon stands out as a powerful library in Java that provides developers with the ability to analyze and transform Java code without compromising its structure.
This blog post aims to unveil the intricacies of Spoon, address common challenges developers face during code analysis, and provide solutions to overcome these potential roadblocks.
What is Spoon?
Spoon is an open-source library that enables the analysis and transformation of Java source code. It allows developers to create flexible code manipulations, making it easier to perform tasks such as code generation, refactoring, and even creating quality metrics.
For those interested in learning more about Spoon, you can visit their official GitHub repository.
Key Features of Spoon
-
Abstract Syntax Tree (AST) Representation: Spoon constructs an AST for your Java code, allowing developers to navigate and manipulate the code structure easily.
-
Simple API for Code Manipulation: The library provides a straightforward API, making it easier for developers to transform Java code without extensive overhead.
-
Support for Java Standards: Spoon fully supports Java language features, including generics, lambdas, and annotations.
Getting Started with Spoon
Before diving into advanced use cases, it is essential to set up your environment for Spoon. Here's a simple Maven configuration to include Spoon in your project:
<dependency>
<groupId>fr.inria.gova.hub</groupId>
<artifactId>spoon-core</artifactId>
<version>10.3.0</version> <!-- Replace with the latest version -->
</dependency>
Sample Project Structure
When working with Spoon, structure your project as follows:
your-project/
|-- src/
| |-- main/
| | |-- java/
| | | |-- Main.java
| | |-- resources/
|-- pom.xml
Basic Code Analysis with Spoon
The first step in making use of Spoon is to analyze existing Java classes. Here's a simple example of how to analyze a Java class and print all method names:
import spoon.Launcher;
import spoon.reflect.CtModel;
import spoon.reflect.code.CtMethod;
public class Main {
public static void main(String[] args) {
// Initializing the Spoon Launcher
Launcher launcher = new Launcher();
// Setting the source classpath
launcher.addInputResource("src/main/java");
// Building the model
CtModel model = launcher.buildModel();
// Iterating through all methods and printing their names
model.getAllElements()
.filter(el -> el instanceof CtMethod)
.forEach(method -> System.out.println(((CtMethod<?>) method).getSimpleName()));
}
}
Code Breakdown
- Launcher Instance: The
Launcher
class is crucial for defining the context for analysis. It configures the classpath and initializes the analysis. - Add Input Resource: This method specifies where Spoon should look for Java files.
- Building the Model: The
buildModel()
method constructs the AST for all the Java files in the input resource. - Filter Methods: We filter for elements of type
CtMethod
, which represents Java methods, and print their names.
Overcoming Common Challenges with Spoon
While Spoon provides a seamless experience for many use cases, developers may encounter challenges during code analysis. Here are some common issues along with suggested solutions.
1. Handling Large Codebases
Problem: Analyzing a vast codebase can be time-consuming and resource-intensive.
Solution: Utilize Spoon's selective analysis features. You can limit the analysis to specific packages or classes. Adjusting the scope considerably speeds up the analysis process.
launcher.addInputResource("src/main/java/com/example"); // Limiting to a specific package
2. Style and Formatting Issues
Problem: The default formatting of code generated or modified by Spoon may not adhere to your project's code standards.
Solution: Ensure to implement a formatting phase after the transformation. You can use Google Java Format or a similar library to maintain consistent style.
Example:
import com.google.googlejavaformat.java.Formatter;
import java.io.IOException;
public class CodeFormatter {
public static String formatCode(String code) throws IOException {
Formatter formatter = new Formatter();
return formatter.format(code);
}
}
3. Extending Spoon for Custom Analyses
Problem: Your project may require specific analyses that are not covered by default Spoon features.
Solution: Spoon allows the creation of custom processors. Here’s a simple example of a processor that counts method parameters:
import spoon.processing.AbstractProcessor;
import spoon.reflect.code.CtMethod;
public class ParameterCounterProcessor extends AbstractProcessor<CtMethod<?>> {
@Override
public void process(CtMethod<?> method) {
System.out.println("Method " + method.getSimpleName() + " has " + method.getParameters().size() + " parameters.");
}
}
// Usage
launcher.addProcessor(new ParameterCounterProcessor());
Advanced Transformations
Spoon is more than just an analysis tool. You can also apply modifications to the code. Here’s how to rename a method in a given class:
import spoon.reflect.code.CtMethod;
import spoon.reflect.declaration.CtClass;
public class RenameMethodProcessor extends AbstractProcessor<CtMethod<?>> {
@Override
public void process(CtMethod<?> method) {
if (method.getSimpleName().equals("oldMethodName")) {
method.setSimpleName("newMethodName");
System.out.println("Renamed method to " + method.getSimpleName());
}
}
}
// Usage
launcher.addProcessor(new RenameMethodProcessor());
Bringing It All Together
Mastering Spoon can significantly streamline both code analysis and transformation tasks in Java. While it may take time to navigate its features and overcome challenges, the benefits are undeniable. With the ability to analyze code structure, perform complex modifications, and integrate with existing projects, Spoon proves to be an invaluable tool for Java developers.
To stay updated with Spoon's latest features and best practices, you may want to check out the Spoon documentation or consider exploring additional resources such as the community forums.
By utilizing Spoon effectively, you are empowered to tackle code quality challenges head-on, maintaining a robust and efficient development workflow. Happy coding!
Checkout our other articles